Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / Common / AuthoringOM / Design / WinOEToolBoxItem.cs / 1305376 / WinOEToolBoxItem.cs
namespace System.Workflow.ComponentModel.Design { using System; using System.Collections; using System.Drawing; using System.Drawing.Design; using System.ComponentModel; using System.Runtime.Serialization; using System.ComponentModel.Design; using System.Security.Permissions; using System.Reflection; [Serializable] [PermissionSet(SecurityAction.InheritanceDemand, Name = "FullTrust")] [PermissionSet(SecurityAction.LinkDemand, Name = "FullTrust")] public class ActivityToolboxItem: ToolboxItem { private const String ActivitySuffix = "Activity"; public ActivityToolboxItem() { } public ActivityToolboxItem(Type type):base(type) { // if (type != null) { if (type.Name != null) { string name = type.Name; if ((type.Assembly == Assembly.GetExecutingAssembly() || type.Assembly != null && type.Assembly.FullName != null && type.Assembly.FullName.Equals(AssemblyRef.ActivitiesAssemblyRef, StringComparison.OrdinalIgnoreCase)) && type.Name.EndsWith(ActivitySuffix, StringComparison.Ordinal) && !type.Name.Equals(ActivitySuffix, StringComparison.Ordinal)) { name = type.Name.Substring(0, type.Name.Length - ActivitySuffix.Length); } base.DisplayName = name; } base.Description = ActivityDesigner.GetActivityDescription(type); } } protected ActivityToolboxItem(SerializationInfo info, StreamingContext context) { Deserialize(info, context); } public virtual IComponent[] CreateComponentsWithUI(IDesignerHost host) { return CreateComponentsCore(host); } // protected override IComponent[] CreateComponentsCore(IDesignerHost host) { Type typeOfComponent = GetType(host, AssemblyName, TypeName, true); if (typeOfComponent == null && host != null) typeOfComponent = host.GetType(TypeName); if (typeOfComponent == null) { ITypeProviderCreator tpc = null; if (host != null) tpc = (ITypeProviderCreator)host.GetService(typeof(ITypeProviderCreator)); if (tpc != null) { System.Reflection.Assembly assembly = tpc.GetTransientAssembly(this.AssemblyName); if (assembly != null) typeOfComponent = assembly.GetType(this.TypeName); } if (typeOfComponent == null) typeOfComponent = GetType(host, AssemblyName, TypeName, true); } ArrayList comps = new ArrayList(); if (typeOfComponent != null) { if (typeof(IComponent).IsAssignableFrom(typeOfComponent)) comps.Add(TypeDescriptor.CreateInstance(null, typeOfComponent, null, null)); } IComponent[] temp = new IComponent[comps.Count]; comps.CopyTo(temp, 0); return temp; } public static Image GetToolboxImage(Type activityType) { if (activityType == null) throw new ArgumentNullException("activityType"); Image toolBoxImage = null; if (activityType != null) { object[] attribs = activityType.GetCustomAttributes(typeof(ToolboxBitmapAttribute), false); if (attribs != null && attribs.GetLength(0) == 0) attribs = activityType.GetCustomAttributes(typeof(ToolboxBitmapAttribute), true); ToolboxBitmapAttribute toolboxBitmapAttribute = (attribs != null && attribs.GetLength(0) > 0) ? attribs[0] as ToolboxBitmapAttribute : null; if (toolboxBitmapAttribute != null) toolBoxImage = toolboxBitmapAttribute.GetImage(activityType); } return toolBoxImage; } public static string GetToolboxDisplayName(Type activityType) { if (activityType == null) throw new ArgumentNullException("activityType"); string displayName = activityType.Name; object[] toolboxItemAttributes = activityType.GetCustomAttributes(typeof(ToolboxItemAttribute), true); if (toolboxItemAttributes != null && toolboxItemAttributes.Length > 0) { ToolboxItemAttribute toolboxItemAttrib = toolboxItemAttributes[0] as ToolboxItemAttribute; if (toolboxItemAttrib != null && toolboxItemAttrib.ToolboxItemType != null) { try { ToolboxItem item = Activator.CreateInstance(toolboxItemAttrib.ToolboxItemType, new object[] { activityType }) as ToolboxItem; if (item != null) displayName = item.DisplayName; } catch { } } } if (activityType.Assembly != null && activityType.Assembly.FullName != null) { if ((activityType.Assembly.FullName.Equals(AssemblyRef.ActivitiesAssemblyRef, StringComparison.OrdinalIgnoreCase) || activityType.Assembly.FullName.Equals(Assembly.GetExecutingAssembly().FullName, StringComparison.OrdinalIgnoreCase)) && displayName.EndsWith(ActivitySuffix, StringComparison.Ordinal) && !displayName.Equals(ActivitySuffix, StringComparison.Ordinal)) { displayName = displayName.Substring(0, displayName.Length - ActivitySuffix.Length); } } return displayName; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace System.Workflow.ComponentModel.Design { using System; using System.Collections; using System.Drawing; using System.Drawing.Design; using System.ComponentModel; using System.Runtime.Serialization; using System.ComponentModel.Design; using System.Security.Permissions; using System.Reflection; [Serializable] [PermissionSet(SecurityAction.InheritanceDemand, Name = "FullTrust")] [PermissionSet(SecurityAction.LinkDemand, Name = "FullTrust")] public class ActivityToolboxItem: ToolboxItem { private const String ActivitySuffix = "Activity"; public ActivityToolboxItem() { } public ActivityToolboxItem(Type type):base(type) { // if (type != null) { if (type.Name != null) { string name = type.Name; if ((type.Assembly == Assembly.GetExecutingAssembly() || type.Assembly != null && type.Assembly.FullName != null && type.Assembly.FullName.Equals(AssemblyRef.ActivitiesAssemblyRef, StringComparison.OrdinalIgnoreCase)) && type.Name.EndsWith(ActivitySuffix, StringComparison.Ordinal) && !type.Name.Equals(ActivitySuffix, StringComparison.Ordinal)) { name = type.Name.Substring(0, type.Name.Length - ActivitySuffix.Length); } base.DisplayName = name; } base.Description = ActivityDesigner.GetActivityDescription(type); } } protected ActivityToolboxItem(SerializationInfo info, StreamingContext context) { Deserialize(info, context); } public virtual IComponent[] CreateComponentsWithUI(IDesignerHost host) { return CreateComponentsCore(host); } // protected override IComponent[] CreateComponentsCore(IDesignerHost host) { Type typeOfComponent = GetType(host, AssemblyName, TypeName, true); if (typeOfComponent == null && host != null) typeOfComponent = host.GetType(TypeName); if (typeOfComponent == null) { ITypeProviderCreator tpc = null; if (host != null) tpc = (ITypeProviderCreator)host.GetService(typeof(ITypeProviderCreator)); if (tpc != null) { System.Reflection.Assembly assembly = tpc.GetTransientAssembly(this.AssemblyName); if (assembly != null) typeOfComponent = assembly.GetType(this.TypeName); } if (typeOfComponent == null) typeOfComponent = GetType(host, AssemblyName, TypeName, true); } ArrayList comps = new ArrayList(); if (typeOfComponent != null) { if (typeof(IComponent).IsAssignableFrom(typeOfComponent)) comps.Add(TypeDescriptor.CreateInstance(null, typeOfComponent, null, null)); } IComponent[] temp = new IComponent[comps.Count]; comps.CopyTo(temp, 0); return temp; } public static Image GetToolboxImage(Type activityType) { if (activityType == null) throw new ArgumentNullException("activityType"); Image toolBoxImage = null; if (activityType != null) { object[] attribs = activityType.GetCustomAttributes(typeof(ToolboxBitmapAttribute), false); if (attribs != null && attribs.GetLength(0) == 0) attribs = activityType.GetCustomAttributes(typeof(ToolboxBitmapAttribute), true); ToolboxBitmapAttribute toolboxBitmapAttribute = (attribs != null && attribs.GetLength(0) > 0) ? attribs[0] as ToolboxBitmapAttribute : null; if (toolboxBitmapAttribute != null) toolBoxImage = toolboxBitmapAttribute.GetImage(activityType); } return toolBoxImage; } public static string GetToolboxDisplayName(Type activityType) { if (activityType == null) throw new ArgumentNullException("activityType"); string displayName = activityType.Name; object[] toolboxItemAttributes = activityType.GetCustomAttributes(typeof(ToolboxItemAttribute), true); if (toolboxItemAttributes != null && toolboxItemAttributes.Length > 0) { ToolboxItemAttribute toolboxItemAttrib = toolboxItemAttributes[0] as ToolboxItemAttribute; if (toolboxItemAttrib != null && toolboxItemAttrib.ToolboxItemType != null) { try { ToolboxItem item = Activator.CreateInstance(toolboxItemAttrib.ToolboxItemType, new object[] { activityType }) as ToolboxItem; if (item != null) displayName = item.DisplayName; } catch { } } } if (activityType.Assembly != null && activityType.Assembly.FullName != null) { if ((activityType.Assembly.FullName.Equals(AssemblyRef.ActivitiesAssemblyRef, StringComparison.OrdinalIgnoreCase) || activityType.Assembly.FullName.Equals(Assembly.GetExecutingAssembly().FullName, StringComparison.OrdinalIgnoreCase)) && displayName.EndsWith(ActivitySuffix, StringComparison.Ordinal) && !displayName.Equals(ActivitySuffix, StringComparison.Ordinal)) { displayName = displayName.Substring(0, displayName.Length - ActivitySuffix.Length); } } return displayName; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
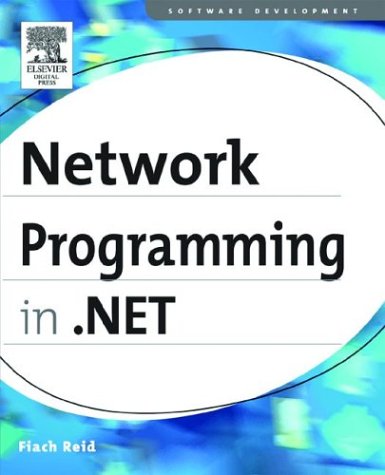
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DesigntimeLicenseContext.cs
- DataDesignUtil.cs
- CanExecuteRoutedEventArgs.cs
- Validator.cs
- Section.cs
- TextSelection.cs
- DSGeneratorProblem.cs
- NamedPipeProcessProtocolHandler.cs
- MailWriter.cs
- ClientRuntimeConfig.cs
- ExitEventArgs.cs
- BaseParagraph.cs
- Graphics.cs
- TextElementEnumerator.cs
- DataGridViewRowConverter.cs
- FileRecordSequenceCompletedAsyncResult.cs
- SamlSubject.cs
- TextServicesHost.cs
- ComponentRenameEvent.cs
- LowerCaseStringConverter.cs
- SqlParameter.cs
- ContainerSelectorBehavior.cs
- ExpandableObjectConverter.cs
- SHA1.cs
- XmlEntity.cs
- SqlGenericUtil.cs
- ExpressionParser.cs
- SplitterPanel.cs
- StylusEditingBehavior.cs
- QueryExpression.cs
- TrackBar.cs
- DrawItemEvent.cs
- User.cs
- safePerfProviderHandle.cs
- ConnectionManagementElement.cs
- ReadOnlyHierarchicalDataSourceView.cs
- _LocalDataStoreMgr.cs
- AssemblyUtil.cs
- DataGrid.cs
- DateTimePicker.cs
- DataGridViewRowPrePaintEventArgs.cs
- ISAPIWorkerRequest.cs
- ConfigurationStrings.cs
- EmptyElement.cs
- PersonalizationStateInfo.cs
- GeometryModel3D.cs
- SourceSwitch.cs
- ListBoxAutomationPeer.cs
- TemplatedWizardStep.cs
- ObjectResult.cs
- ColumnMap.cs
- FormClosedEvent.cs
- ByteStack.cs
- ComponentCollection.cs
- LogLogRecord.cs
- MatrixKeyFrameCollection.cs
- CatalogZone.cs
- SQLDouble.cs
- MetricEntry.cs
- X509ChainPolicy.cs
- DeviceSpecificChoice.cs
- DataQuery.cs
- FloaterBaseParagraph.cs
- Pens.cs
- ReadOnlyNameValueCollection.cs
- AsymmetricAlgorithm.cs
- MetadataSet.cs
- XmlAutoDetectWriter.cs
- Symbol.cs
- BindingList.cs
- ShapingWorkspace.cs
- IdnMapping.cs
- CollectionsUtil.cs
- DataControlImageButton.cs
- TreeViewItemAutomationPeer.cs
- OletxDependentTransaction.cs
- XmlSchemaSimpleTypeRestriction.cs
- EventLogTraceListener.cs
- TextBoxBase.cs
- StringResourceManager.cs
- ObjectViewListener.cs
- DataGridColumnCollection.cs
- XmlTextReaderImpl.cs
- RecordsAffectedEventArgs.cs
- MemberPathMap.cs
- XmlUtil.cs
- MbpInfo.cs
- Config.cs
- AttributeXamlType.cs
- DataGridViewButtonColumn.cs
- OracleBinary.cs
- Types.cs
- IntersectQueryOperator.cs
- DocumentViewer.cs
- TracingConnectionInitiator.cs
- PlainXmlSerializer.cs
- Matrix3D.cs
- RowUpdatedEventArgs.cs
- WasEndpointConfigContainer.cs
- Matrix3D.cs