Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Common / Utils / Boolean / Clause.cs / 1305376 / Clause.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Text; using System.Globalization; using System.Collections.ObjectModel; using System.Diagnostics; using System.Linq; namespace System.Data.Common.Utils.Boolean { ////// Base class for clauses, which are (constrained) combinations of literals. /// ///Type of normal form literal. internal abstract class Clause: NormalFormNode { private readonly Set > _literals; private readonly int _hashCode; /// /// Initialize a new clause. /// /// Literals contained in the clause. /// Type of expression tree to produce from literals. protected Clause(Set> literals, ExprType treeType) : base(ConvertLiteralsToExpr(literals, treeType)) { _literals = literals.AsReadOnly(); _hashCode = _literals.GetElementsHashCode(); } /// /// Gets the literals contained in this clause. /// internal Set> Literals { get { return _literals; } } // Given a collection of literals and a tree type, returns an expression of the given type. private static BoolExpr ConvertLiteralsToExpr(Set > literals, ExprType treeType) { bool isAnd = ExprType.And == treeType; Debug.Assert(isAnd || ExprType.Or == treeType); IEnumerable > literalExpressions = literals.Select( new Func , BoolExpr >(ConvertLiteralToExpression)); if (isAnd) { return new AndExpr (literalExpressions); } else { return new OrExpr (literalExpressions); } } // Given a literal, returns its logical equivalent expression. private static BoolExpr ConvertLiteralToExpression(Literal literal) { return literal.Expr; } public override string ToString() { StringBuilder builder = new StringBuilder(); builder.Append("Clause{"); builder.Append(_literals); return builder.Append("}").ToString(); } public override int GetHashCode() { return _hashCode; } public override bool Equals(object obj) { Debug.Fail("call typed Equals"); return base.Equals(obj); } } /// /// A DNF clause is of the form: /// /// Literal1 . Literal2 . ... /// /// Each literal is of the form: /// /// Term /// /// or /// /// !Term /// ///Type of normal form literal. internal sealed class DnfClause: Clause , IEquatable > { /// /// Initialize a DNF clause. /// /// Literals in clause. internal DnfClause(Set> literals) : base(literals, ExprType.And) { } public bool Equals(DnfClause other) { return null != other && other.Literals.SetEquals(Literals); } } /// /// A CNF clause is of the form: /// /// Literal1 + Literal2 . ... /// /// Each literal is of the form: /// /// Term /// /// or /// /// !Term /// ///Type of normal form literal. internal sealed class CnfClause: Clause , IEquatable > { /// /// Initialize a CNF clause. /// /// Literals in clause. internal CnfClause(Set> literals) : base(literals, ExprType.Or) { } public bool Equals(CnfClause other) { return null != other && other.Literals.SetEquals(Literals); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Text; using System.Globalization; using System.Collections.ObjectModel; using System.Diagnostics; using System.Linq; namespace System.Data.Common.Utils.Boolean { ////// Base class for clauses, which are (constrained) combinations of literals. /// ///Type of normal form literal. internal abstract class Clause: NormalFormNode { private readonly Set > _literals; private readonly int _hashCode; /// /// Initialize a new clause. /// /// Literals contained in the clause. /// Type of expression tree to produce from literals. protected Clause(Set> literals, ExprType treeType) : base(ConvertLiteralsToExpr(literals, treeType)) { _literals = literals.AsReadOnly(); _hashCode = _literals.GetElementsHashCode(); } /// /// Gets the literals contained in this clause. /// internal Set> Literals { get { return _literals; } } // Given a collection of literals and a tree type, returns an expression of the given type. private static BoolExpr ConvertLiteralsToExpr(Set > literals, ExprType treeType) { bool isAnd = ExprType.And == treeType; Debug.Assert(isAnd || ExprType.Or == treeType); IEnumerable > literalExpressions = literals.Select( new Func , BoolExpr >(ConvertLiteralToExpression)); if (isAnd) { return new AndExpr (literalExpressions); } else { return new OrExpr (literalExpressions); } } // Given a literal, returns its logical equivalent expression. private static BoolExpr ConvertLiteralToExpression(Literal literal) { return literal.Expr; } public override string ToString() { StringBuilder builder = new StringBuilder(); builder.Append("Clause{"); builder.Append(_literals); return builder.Append("}").ToString(); } public override int GetHashCode() { return _hashCode; } public override bool Equals(object obj) { Debug.Fail("call typed Equals"); return base.Equals(obj); } } /// /// A DNF clause is of the form: /// /// Literal1 . Literal2 . ... /// /// Each literal is of the form: /// /// Term /// /// or /// /// !Term /// ///Type of normal form literal. internal sealed class DnfClause: Clause , IEquatable > { /// /// Initialize a DNF clause. /// /// Literals in clause. internal DnfClause(Set> literals) : base(literals, ExprType.And) { } public bool Equals(DnfClause other) { return null != other && other.Literals.SetEquals(Literals); } } /// /// A CNF clause is of the form: /// /// Literal1 + Literal2 . ... /// /// Each literal is of the form: /// /// Term /// /// or /// /// !Term /// ///Type of normal form literal. internal sealed class CnfClause: Clause , IEquatable > { /// /// Initialize a CNF clause. /// /// Literals in clause. internal CnfClause(Set> literals) : base(literals, ExprType.Or) { } public bool Equals(CnfClause other) { return null != other && other.Literals.SetEquals(Literals); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
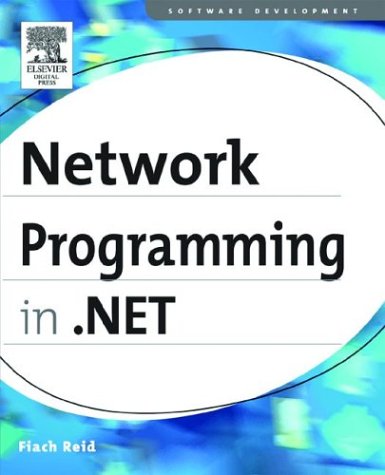
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Image.cs
- BamlStream.cs
- CommandSet.cs
- CoreSwitches.cs
- XmlCodeExporter.cs
- pingexception.cs
- InProcStateClientManager.cs
- ResXFileRef.cs
- TraceHandler.cs
- RuntimeCompatibilityAttribute.cs
- Debug.cs
- HashAlgorithm.cs
- SqlMetaData.cs
- printdlgexmarshaler.cs
- Condition.cs
- BroadcastEventHelper.cs
- WinEventHandler.cs
- Aggregates.cs
- OneOf.cs
- TextEncodedRawTextWriter.cs
- WindowInteropHelper.cs
- ConditionalAttribute.cs
- PreservationFileWriter.cs
- XMLDiffLoader.cs
- DbgCompiler.cs
- DesignOnlyAttribute.cs
- ReadOnlyDataSource.cs
- _UriTypeConverter.cs
- BinaryNode.cs
- MsmqAuthenticationMode.cs
- SHA384Managed.cs
- xsdvalidator.cs
- ValueHandle.cs
- DataBoundLiteralControl.cs
- RemoveStoryboard.cs
- Int16Storage.cs
- CurrencyManager.cs
- ProfilePropertySettingsCollection.cs
- PerspectiveCamera.cs
- DoWorkEventArgs.cs
- BeginStoryboard.cs
- EditableLabelControl.cs
- Mutex.cs
- XmlAttributeProperties.cs
- SystemDiagnosticsSection.cs
- RoleGroup.cs
- ReachPrintTicketSerializer.cs
- GatewayIPAddressInformationCollection.cs
- MissingSatelliteAssemblyException.cs
- DataGridState.cs
- DataDocumentXPathNavigator.cs
- ViewManager.cs
- PerformanceCountersElement.cs
- SuppressIldasmAttribute.cs
- _UriSyntax.cs
- KeyValueConfigurationElement.cs
- HyperLink.cs
- UntypedNullExpression.cs
- StylusSystemGestureEventArgs.cs
- BaseDataBoundControlDesigner.cs
- PrintDialog.cs
- NameObjectCollectionBase.cs
- RetriableClipboard.cs
- QuotedPrintableStream.cs
- ChameleonKey.cs
- DllNotFoundException.cs
- OneOfElement.cs
- Calendar.cs
- XmlText.cs
- PlatformCulture.cs
- NavigationProgressEventArgs.cs
- XamlStyleSerializer.cs
- XmlSchemaSimpleContentRestriction.cs
- NavigatingCancelEventArgs.cs
- CodeConditionStatement.cs
- BindingMemberInfo.cs
- AttachedAnnotation.cs
- NativeMethods.cs
- MediaEntryAttribute.cs
- ControlIdConverter.cs
- BackgroundWorker.cs
- FontEmbeddingManager.cs
- ActivityCodeGenerator.cs
- DataSourceSerializationException.cs
- ArgumentOutOfRangeException.cs
- StorageScalarPropertyMapping.cs
- ComPlusContractBehavior.cs
- PackagePartCollection.cs
- XmlSchemaProviderAttribute.cs
- _CommandStream.cs
- PartialList.cs
- RootBuilder.cs
- ZipFileInfo.cs
- List.cs
- UntypedNullExpression.cs
- SoapSchemaImporter.cs
- ProcessHostMapPath.cs
- autovalidator.cs
- HistoryEventArgs.cs
- Table.cs