Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WebForms / System / Web / UI / Design / WebControls / BaseDataBoundControlDesigner.cs / 1 / BaseDataBoundControlDesigner.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design.WebControls { using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Data; using System.Design; using System.Diagnostics; using System.Drawing; using System.Text; using System.Web; using System.Web.UI; using System.Web.UI.Design.Util; using System.Web.UI.WebControls; using System.Windows.Forms; using Control = System.Web.UI.Control; ////// /// BaseDataBoundControlDesigner is the designer associated with a /// BaseDataBoundControl. It provides the basic shared design-time experience /// for all data-bound controls. /// public abstract class BaseDataBoundControlDesigner : ControlDesigner { private bool _keepDataSourceBrowsable; ////// /// Implements the designer's version of the DataSource property. /// This is used to shadow the DataSource property of the /// runtime control. /// The data source is persisted by the designer as a data-binding /// expression on the control's tag. /// public string DataSource { get { DataBinding binding = DataBindings["DataSource"]; if (binding != null) { return binding.Expression; } return String.Empty; } set { if ((value == null) || (value.Length == 0)) { DataBindings.Remove("DataSource"); } else { DataBinding binding = DataBindings["DataSource"]; if (binding == null) { binding = new DataBinding("DataSource", typeof(IEnumerable), value); } else { binding.Expression = value; } DataBindings.Add(binding); } OnDataSourceChanged(true); OnBindingsCollectionChangedInternal("DataSource"); } } ////// /// Implements the designer's version of the DataSourceID property. /// This is used to shadow the DataSourceID property of the /// runtime control. /// public string DataSourceID { get { return ((BaseDataBoundControl)Component).DataSourceID; } set { if (value == DataSourceID) { return; } if (value == SR.GetString(SR.DataSourceIDChromeConverter_NewDataSource)) { CreateDataSource(); TypeDescriptor.Refresh(Component); return; } if (value == SR.GetString(SR.DataSourceIDChromeConverter_NoDataSource)) { value = String.Empty; } // Refresh must be called before componentchanged (invoked by PropertyDescriptor.SetValue) // so the property grid can update the actions properly. TypeDescriptor.Refresh(Component); // BaseDataBoundControl.DataSourceID = value; // Get the property descriptor with the explicit type so we don't get the designer's filtered property PropertyDescriptor propDesc = TypeDescriptor.GetProperties(typeof(BaseDataBoundControl))["DataSourceID"]; propDesc.SetValue(Component, value); OnDataSourceChanged(false); OnSchemaRefreshed(); } } ////// Performs the actions necessary to connect to the current data /// source. This typically involved unhooking events from the previous /// data source and then attaching new events to the new data source. /// protected abstract bool ConnectToDataSource(); ////// Creates a new data source for this data bound control. /// protected abstract void CreateDataSource(); ////// /// Performs the necessary actions to set up the data bound control /// such that its child controls will be created with the proper /// state so that when the design time HTML is retrieved the control /// will render properly. /// protected abstract void DataBind(BaseDataBoundControl dataBoundControl); ////// Performs the actions necessary to disconnect from the current data /// source. This typically involved unhooking events from the previous /// data source. /// protected abstract void DisconnectFromDataSource(); ///protected override void Dispose(bool disposing) { if (disposing) { if (Component != null && Component.Site != null) { // Detach from data source designer events DisconnectFromDataSource(); if (RootDesigner != null) { RootDesigner.LoadComplete -= new EventHandler(OnDesignerLoadComplete); } IComponentChangeService changeService = (IComponentChangeService)Component.Site.GetService(typeof(IComponentChangeService)); if (changeService != null) { changeService.ComponentAdded -= new ComponentEventHandler(this.OnComponentAdded); changeService.ComponentRemoving -= new ComponentEventHandler(this.OnComponentRemoving); changeService.ComponentRemoved -= new ComponentEventHandler(this.OnComponentRemoved); changeService.ComponentChanged -= new ComponentChangedEventHandler(this.OnAnyComponentChanged); } } } base.Dispose(disposing); } /// /// /// Provides the design-time HTML used to display the control /// at design-time. /// DataBoundControlDesigner retrieves sample data used for binding /// purposes at design-time before rendering the control. /// If the control is not data-bound it calls GetEmptyDesignTimeHtml. /// If there is an error rendering the control, it calls /// GetErrorDesignTimeHtml. /// ////// The HTML used to render the control at design-time. /// public override string GetDesignTimeHtml() { string designTimeHtml = String.Empty; try { DataBind((BaseDataBoundControl)ViewControl); designTimeHtml = base.GetDesignTimeHtml(); } catch (Exception e) { designTimeHtml = GetErrorDesignTimeHtml(e); } return designTimeHtml; } ////// /// Provides the design-time HTML used to display the control /// at design-time if the control is empty or the dataSource can't be /// retrieved. /// BaseDataBoundControlDesigner retrieves sample data used for binding /// purposes at design-time before rendering the control. /// ////// The HTML used to render the control at design-time for an empty /// dataSource. /// protected override string GetEmptyDesignTimeHtml() { return CreatePlaceHolderDesignTimeHtml(null); } ////// /// Provides HTML to show in the designer when an error occurs. /// protected override string GetErrorDesignTimeHtml(Exception e) { return CreatePlaceHolderDesignTimeHtml(SR.GetString(SR.Control_ErrorRenderingShort) + "
" + e.Message); } ////// /// Initializes the component /// public override void Initialize(IComponent component) { VerifyInitializeArgument(component, typeof(BaseDataBoundControl)); base.Initialize(component); SetViewFlags(ViewFlags.DesignTimeHtmlRequiresLoadComplete, true); if (RootDesigner != null) { if (RootDesigner.IsLoading) { RootDesigner.LoadComplete += new EventHandler(OnDesignerLoadComplete); } else { OnDesignerLoadComplete(null, EventArgs.Empty); } } IComponentChangeService changeService = (IComponentChangeService)component.Site.GetService(typeof(IComponentChangeService)); if (changeService != null) { changeService.ComponentAdded += new ComponentEventHandler(this.OnComponentAdded); changeService.ComponentRemoving += new ComponentEventHandler(this.OnComponentRemoving); changeService.ComponentRemoved += new ComponentEventHandler(this.OnComponentRemoved); changeService.ComponentChanged += new ComponentChangedEventHandler(this.OnAnyComponentChanged); } } ////// /// Fires when a component is changing. This may be our DataSourceControl /// private void OnAnyComponentChanged(object sender, ComponentChangedEventArgs ce) { Control control = ce.Component as Control; if (control != null) { if (ce.Member != null && ce.Member.Name == "ID" && Component != null) { if((string)ce.OldValue == DataSourceID || (string)ce.NewValue == DataSourceID) { OnDataSourceChanged(false); } } } } ////// Fires when a component is added. This may be our DataSourceControl /// private void OnComponentAdded(object sender, ComponentEventArgs e) { Control control = e.Component as Control; if (control != null) { if (control.ID == DataSourceID) { OnDataSourceChanged(false); } } } ////// Fires when a component is being removed. This may be our DataSourceControl /// private void OnComponentRemoving(object sender, ComponentEventArgs e) { Control control = e.Component as Control; if (control != null && Component != null) { if (control.ID == DataSourceID) { DisconnectFromDataSource(); } } } ////// Fires when a component is removed. This may be our DataSourceControl /// private void OnComponentRemoved(object sender, ComponentEventArgs e) { Control control = e.Component as Control; if (control != null && Component != null) { if (control.ID == DataSourceID) { IDesignerHost designerHost = (IDesignerHost)this.GetService(typeof(IDesignerHost)); Debug.Assert(designerHost != null, "Did not get DesignerHost service."); if (designerHost != null && !designerHost.Loading) { OnDataSourceChanged(false); } } } } ////// /// Raised when the data source that this control is bound to has /// changed. Designers can override this to perform additional actions /// required. Make sure to call the base implementation. /// protected virtual void OnDataSourceChanged(bool forceUpdateView) { bool dataSourceChanged = ConnectToDataSource(); if (dataSourceChanged || forceUpdateView) { UpdateDesignTimeHtml(); } } private void OnDesignerLoadComplete(object sender, EventArgs e) { OnDataSourceChanged(false); } ////// Raised when the data source that this control is bound to has /// new schema. Designers can override this to perform additional /// actions required when new schema is available. /// protected virtual void OnSchemaRefreshed() { } ////// /// Overridden by the designer to shadow various runtime properties /// with corresponding properties that it implements. /// /// /// The properties to be filtered. /// protected override void PreFilterProperties(IDictionary properties) { base.PreFilterProperties(properties); PropertyDescriptor prop; prop = (PropertyDescriptor)properties["DataSource"]; Debug.Assert(prop != null); // we can't create the designer DataSource property based on the runtime property since these // types do not match. Therefore, we have to copy over all the attributes from the runtime // and use them that way. // Set the BrowsableAttribute for DataSource to false if DataSource is empty // so the user isn't confused about which DataSource property to use System.ComponentModel.AttributeCollection runtimeAttributes = prop.Attributes; int browsableAttributeIndex = -1; int attributeCount; int runtimeAttributeCount = runtimeAttributes.Count; string dataSource = DataSource; bool hasDataSource = (dataSource != null) && (dataSource.Length > 0); if (hasDataSource) { _keepDataSourceBrowsable = true; } // find the position of the BrowsableAttribute for (int i = 0; i < runtimeAttributes.Count; i++ ) { if (runtimeAttributes[i] is BrowsableAttribute) { browsableAttributeIndex = i; break; } } // allocate the right sized array for attributes if (browsableAttributeIndex == -1 && !hasDataSource && !_keepDataSourceBrowsable) { attributeCount = runtimeAttributeCount + 1; } else { attributeCount = runtimeAttributeCount; } Attribute[] attrs = new Attribute[attributeCount]; runtimeAttributes.CopyTo(attrs, 0); // if DataSource is not empty and there was no BrowsableAttribute, add one. Otherwise, // change the one that's there to be false. if (!hasDataSource && !_keepDataSourceBrowsable) { if (browsableAttributeIndex == -1) { attrs[runtimeAttributeCount] = BrowsableAttribute.No; } else { attrs[browsableAttributeIndex] = BrowsableAttribute.No; } } prop = TypeDescriptor.CreateProperty(this.GetType(), "DataSource", typeof(string), attrs); properties["DataSource"] = prop; } public static DialogResult ShowCreateDataSourceDialog(ControlDesigner controlDesigner, Type dataSourceType, bool configure, out string dataSourceID) { CreateDataSourceDialog dialog = new CreateDataSourceDialog(controlDesigner, dataSourceType, configure); DialogResult result = UIServiceHelper.ShowDialog(controlDesigner.Component.Site, dialog); dataSourceID = dialog.DataSourceID; return result; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
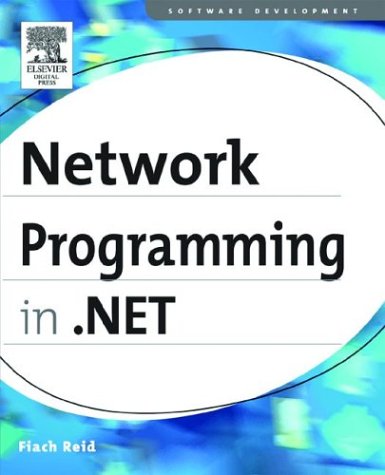
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PublisherMembershipCondition.cs
- SequenceNumber.cs
- MetafileHeader.cs
- TransformerConfigurationWizardBase.cs
- Range.cs
- CaseInsensitiveHashCodeProvider.cs
- StrokeNodeData.cs
- ExpandableObjectConverter.cs
- XmlSchemaAttributeGroupRef.cs
- TransformGroup.cs
- SrgsSubset.cs
- TypeUtil.cs
- AuthenticationModuleElementCollection.cs
- RelationshipWrapper.cs
- FormParameter.cs
- MetadataWorkspace.cs
- LinearGradientBrush.cs
- ItemsPresenter.cs
- EventRecord.cs
- SqlCacheDependencySection.cs
- Matrix3DValueSerializer.cs
- WSSecurityJan2004.cs
- VisualBasicDesignerHelper.cs
- AdapterDictionary.cs
- TagPrefixCollection.cs
- EndpointNotFoundException.cs
- XamlSerializer.cs
- PortCache.cs
- InvokerUtil.cs
- EventSourceCreationData.cs
- SectionUpdates.cs
- HtmlElement.cs
- StrokeNodeEnumerator.cs
- Debugger.cs
- EmptyEnumerable.cs
- MemberJoinTreeNode.cs
- BasicKeyConstraint.cs
- ReadOnlyDictionary.cs
- FactoryRecord.cs
- CroppedBitmap.cs
- Int32RectValueSerializer.cs
- ToolStripRendererSwitcher.cs
- TimerElapsedEvenArgs.cs
- SymbolUsageManager.cs
- NullToBooleanConverter.cs
- DialogBaseForm.cs
- LineUtil.cs
- DeploymentSectionCache.cs
- Int64Storage.cs
- RepeaterItemCollection.cs
- RenderDataDrawingContext.cs
- TextStore.cs
- XmlAnyElementAttributes.cs
- TextEditorParagraphs.cs
- WindowsRegion.cs
- CalculatedColumn.cs
- IssuedTokenServiceElement.cs
- SessionPageStatePersister.cs
- StrongNamePublicKeyBlob.cs
- ProfileService.cs
- EdgeModeValidation.cs
- TimerElapsedEvenArgs.cs
- UITypeEditors.cs
- ComIntegrationManifestGenerator.cs
- DbConnectionStringCommon.cs
- TextServicesContext.cs
- MultiBindingExpression.cs
- GeometryDrawing.cs
- BinarySecretSecurityToken.cs
- Parser.cs
- SignerInfo.cs
- ViewEventArgs.cs
- SocketInformation.cs
- CharAnimationUsingKeyFrames.cs
- ValueOfAction.cs
- Typography.cs
- GenericWebPart.cs
- ProvideValueServiceProvider.cs
- RecordBuilder.cs
- ListViewItem.cs
- Operator.cs
- ValidationVisibilityAttribute.cs
- LineGeometry.cs
- OAVariantLib.cs
- SymDocumentType.cs
- BooleanAnimationUsingKeyFrames.cs
- ObjectDataSourceFilteringEventArgs.cs
- FrameworkElementAutomationPeer.cs
- WeakReadOnlyCollection.cs
- WaitForChangedResult.cs
- COAUTHINFO.cs
- SqlCaseSimplifier.cs
- TabletDevice.cs
- ServerIdentity.cs
- Cursor.cs
- safex509handles.cs
- PersistenceException.cs
- GridItemPattern.cs
- MemoryResponseElement.cs
- EncodingTable.cs