Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / Log / System / IO / Log / SequenceNumber.cs / 1305376 / SequenceNumber.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.IO.Log { // Add property for sequence number size using System; using System.Collections.Generic; [Serializable] public struct SequenceNumber : IComparable{ ulong sequenceNumberHigh; ulong sequenceNumberLow; public SequenceNumber(byte[] sequenceNumber) { if(sequenceNumber == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(Error.ArgumentNull("sequenceNumber")); } if ((sequenceNumber.Length != 8) && (sequenceNumber.Length != 16)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(Error.ArgumentInvalid(SR.Argument_SequenceNumberLength)); } this.sequenceNumberHigh = BitConverter.ToUInt64(sequenceNumber, 0); if (sequenceNumber.Length > 8) { this.sequenceNumberLow = BitConverter.ToUInt64(sequenceNumber, 8); } else { this.sequenceNumberLow = 0; } } internal SequenceNumber(ulong value) { this.sequenceNumberHigh = value; // Ensure that SequenceNumber.Invalid round-trips. // if (value == UInt64.MaxValue) { this.sequenceNumberLow = UInt64.MaxValue; } else { this.sequenceNumberLow = 0; } } internal SequenceNumber(ulong high, ulong low) { this.sequenceNumberHigh = high; this.sequenceNumberLow = low; } internal ulong High { get { return this.sequenceNumberHigh; } } internal ulong Low { get { return this.sequenceNumberLow; } } public static SequenceNumber Invalid { get { return new SequenceNumber(UInt64.MaxValue, UInt64.MaxValue); } } public static bool operator !=( SequenceNumber c1, SequenceNumber c2) { return (c1.CompareTo(c2) != 0); } public static bool operator <( SequenceNumber c1, SequenceNumber c2) { return (c1.CompareTo(c2) < 0); } public static bool operator <=(SequenceNumber c1, SequenceNumber c2) { return (c1.CompareTo(c2) <= 0); } public static bool operator ==( SequenceNumber c1, SequenceNumber c2) { return (c1.CompareTo(c2) == 0); } public static bool operator >( SequenceNumber c1, SequenceNumber c2) { return (c1.CompareTo(c2) > 0); } public static bool operator >=( SequenceNumber c1, SequenceNumber c2) { return (c1.CompareTo(c2) >= 0); } public int CompareTo(SequenceNumber other) { int result = this.sequenceNumberHigh.CompareTo(other.sequenceNumberHigh); if (result == 0) result = this.sequenceNumberLow.CompareTo(other.sequenceNumberLow); return result; } public bool Equals(SequenceNumber other) { return (this.CompareTo(other) == 0); } public override bool Equals(object other) { if (!(other is SequenceNumber)) return false; SequenceNumber otherSeq = (SequenceNumber)(other); return this.Equals(otherSeq); } public byte[] GetBytes() { byte[] bytes; if (this.sequenceNumberLow == 0) { bytes = new byte[8]; WriteUInt64(this.sequenceNumberHigh, bytes, 0); } else { bytes = new byte[16]; WriteUInt64(this.sequenceNumberHigh, bytes, 0); WriteUInt64(this.sequenceNumberLow, bytes, 8); } return bytes; } public override int GetHashCode() { return this.sequenceNumberHigh.GetHashCode() ^ this.sequenceNumberLow.GetHashCode(); } internal static void WriteUInt64(ulong value, byte[] bits, int offset) { bits[offset + 0] = (byte)((value >> 0) & 0xFF); bits[offset + 1] = (byte)((value >> 8) & 0xFF); bits[offset + 2] = (byte)((value >> 16) & 0xFF); bits[offset + 3] = (byte)((value >> 24) & 0xFF); bits[offset + 4] = (byte)((value >> 32) & 0xFF); bits[offset + 5] = (byte)((value >> 40) & 0xFF); bits[offset + 6] = (byte)((value >> 48) & 0xFF); bits[offset + 7] = (byte)((value >> 56) & 0xFF); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
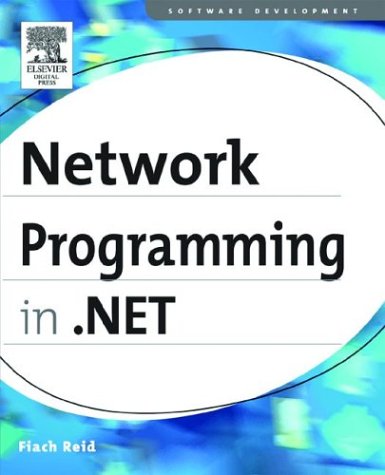
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SmtpDateTime.cs
- ControlOperationInvoker.cs
- NonBatchDirectoryCompiler.cs
- OperationValidationEventArgs.cs
- ProcessHost.cs
- DeadCharTextComposition.cs
- NativeMethods.cs
- Light.cs
- MetadataArtifactLoaderXmlReaderWrapper.cs
- LinearQuaternionKeyFrame.cs
- WebConfigManager.cs
- AuthenticationManager.cs
- ServiceOperationWrapper.cs
- EventMetadata.cs
- SqlMethodAttribute.cs
- ProgressBarAutomationPeer.cs
- TextEditorSpelling.cs
- OutOfMemoryException.cs
- Vector3D.cs
- PassportAuthentication.cs
- WpfWebRequestHelper.cs
- selecteditemcollection.cs
- UnicodeEncoding.cs
- XmlLanguageConverter.cs
- _HelperAsyncResults.cs
- StorageMappingItemLoader.cs
- ProviderSettings.cs
- PackagePart.cs
- Int64AnimationUsingKeyFrames.cs
- ConfigurationException.cs
- DynamicRenderer.cs
- Brush.cs
- PageCache.cs
- Models.cs
- SourceInterpreter.cs
- XmlDataSourceNodeDescriptor.cs
- SystemIPAddressInformation.cs
- TextServicesCompartmentEventSink.cs
- FontFamilyValueSerializer.cs
- ProcessThreadDesigner.cs
- InvokeMemberBinder.cs
- EntityDataSourceChangedEventArgs.cs
- CrossSiteScriptingValidation.cs
- Typeface.cs
- BitmapData.cs
- ConnectionConsumerAttribute.cs
- SamlConditions.cs
- EditorPartCollection.cs
- CallContext.cs
- DrawTreeNodeEventArgs.cs
- HandleCollector.cs
- TokenBasedSet.cs
- ToolStripPanelRow.cs
- CodeGenerator.cs
- SafeLibraryHandle.cs
- XmlNamespaceMapping.cs
- SqlAliaser.cs
- EntityViewGenerationAttribute.cs
- SelectionService.cs
- TypeDescriptionProvider.cs
- SendMailErrorEventArgs.cs
- ClassGenerator.cs
- ContextStack.cs
- SerializationException.cs
- RoutedEvent.cs
- XPathSingletonIterator.cs
- DeviceSpecificDesigner.cs
- DataGridViewCellValueEventArgs.cs
- EntityClientCacheKey.cs
- BoolLiteral.cs
- SqlCacheDependencyDatabase.cs
- ListItemViewControl.cs
- JoinElimination.cs
- CodeArrayCreateExpression.cs
- Graphics.cs
- PageThemeParser.cs
- assertwrapper.cs
- GeneralTransformGroup.cs
- DataRecordInternal.cs
- SqlCommandSet.cs
- ResolvedKeyFrameEntry.cs
- SHA256CryptoServiceProvider.cs
- ExecutionScope.cs
- GiveFeedbackEvent.cs
- RectAnimationClockResource.cs
- ValueUtilsSmi.cs
- AlphaSortedEnumConverter.cs
- DictionaryBase.cs
- SymDocumentType.cs
- CallSiteBinder.cs
- TrustSection.cs
- SqlTriggerContext.cs
- OperationCanceledException.cs
- sqlcontext.cs
- ping.cs
- RsaSecurityKey.cs
- ErrorWrapper.cs
- ListViewTableRow.cs
- SerialPort.cs
- RayMeshGeometry3DHitTestResult.cs