Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / CultureInfoConverter.cs / 1305600 / CultureInfoConverter.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: CultureInfoConverter.cs // // Description: Contains the CultureInfoIetfLanguageTagConverter: TypeConverter for the CultureInfo class. // // History: // 01/12/2005 : niklasb - Initial implementation // 02/10/2006 : niklasb - Renamed from CulutureInfoConveter to CultureInfoIetfLanguageTagConverter // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Security; namespace System.Windows { ////// CultureInfoIetfLanguageTagConverter - Type converter for converting instances of other types to and from CultureInfo. /// ////// This class differs in two ways from System.ComponentModel.CultureInfoConverter, the default type converter /// for the CultureInfo class. First, it uses a string representation based on the IetfLanguageTag property /// rather than the Name property (i.e., RFC 3066 rather than RFC 1766). Second, when converting from a string, /// the properties of the resulting CultureInfo object depend only on the string and not on user overrides set /// in Control Panel. This makes it possible to create documents the appearance of which do not depend on /// local settings. /// public class CultureInfoIetfLanguageTagConverter : TypeConverter { //------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- #region Public Methods ////// CanConvertFrom - Returns whether or not this class can convert from a given type. /// ////// bool - True if this converter can convert from the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertFrom(ITypeDescriptorContext typeDescriptorContext, Type sourceType) { // We can only handle strings. return sourceType == typeof(string); } ////// CanConvertTo - Returns whether or not this class can convert to a given type. /// ////// bool - True if this converter can convert to the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertTo(ITypeDescriptorContext typeDescriptorContext, Type destinationType) { // We can convert to an InstanceDescriptor or to a string. return destinationType == typeof(InstanceDescriptor) || destinationType == typeof(string); } ////// ConvertFrom - Attempt to convert to a CultureInfo from the given object /// ////// A CultureInfo object based on the specified culture name. /// ////// An ArgumentNullException is thrown if the example object is null. /// ////// An ArgumentException is thrown if the example object is not null and is not a valid type /// which can be converted to a CultureInfo. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The object to convert to a CultureInfo. public override object ConvertFrom(ITypeDescriptorContext typeDescriptorContext, CultureInfo cultureInfo, object source) { string cultureName = source as string; if (cultureName != null) { return CultureInfo.GetCultureInfoByIetfLanguageTag(cultureName); } throw GetConvertFromException(source); } ////// ConvertTo - Attempt to convert a CultureInfo to the given type /// ////// The object which was constructed. /// ////// An ArgumentNullException is thrown if the example object is null. /// ////// An ArgumentException is thrown if the example object is not null and is not a CultureInfo, /// or if the destinationType isn't one of the valid destination types. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The double to convert. /// The type to which to convert the CultureInfo. ////// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for CultureInfo.GetCultureInfo, not an arbitrary class/method /// [SecurityCritical] public override object ConvertTo(ITypeDescriptorContext typeDescriptorContext, CultureInfo cultureInfo, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } CultureInfo culture = value as CultureInfo; if (culture != null) { if (destinationType == typeof(string)) { return culture.IetfLanguageTag; } else if (destinationType == typeof(InstanceDescriptor)) { MethodInfo method = typeof(CultureInfo).GetMethod( "GetCultureInfo", BindingFlags.Static | BindingFlags.InvokeMethod | BindingFlags.Public, null, // use default binder new Type[] { typeof(string) }, null // default binder doesn't use parameter modifiers ); return new InstanceDescriptor(method, new object[] { culture.Name }); } } throw GetConvertToException(value, destinationType); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: CultureInfoConverter.cs // // Description: Contains the CultureInfoIetfLanguageTagConverter: TypeConverter for the CultureInfo class. // // History: // 01/12/2005 : niklasb - Initial implementation // 02/10/2006 : niklasb - Renamed from CulutureInfoConveter to CultureInfoIetfLanguageTagConverter // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Security; namespace System.Windows { ////// CultureInfoIetfLanguageTagConverter - Type converter for converting instances of other types to and from CultureInfo. /// ////// This class differs in two ways from System.ComponentModel.CultureInfoConverter, the default type converter /// for the CultureInfo class. First, it uses a string representation based on the IetfLanguageTag property /// rather than the Name property (i.e., RFC 3066 rather than RFC 1766). Second, when converting from a string, /// the properties of the resulting CultureInfo object depend only on the string and not on user overrides set /// in Control Panel. This makes it possible to create documents the appearance of which do not depend on /// local settings. /// public class CultureInfoIetfLanguageTagConverter : TypeConverter { //------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- #region Public Methods ////// CanConvertFrom - Returns whether or not this class can convert from a given type. /// ////// bool - True if this converter can convert from the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertFrom(ITypeDescriptorContext typeDescriptorContext, Type sourceType) { // We can only handle strings. return sourceType == typeof(string); } ////// CanConvertTo - Returns whether or not this class can convert to a given type. /// ////// bool - True if this converter can convert to the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertTo(ITypeDescriptorContext typeDescriptorContext, Type destinationType) { // We can convert to an InstanceDescriptor or to a string. return destinationType == typeof(InstanceDescriptor) || destinationType == typeof(string); } ////// ConvertFrom - Attempt to convert to a CultureInfo from the given object /// ////// A CultureInfo object based on the specified culture name. /// ////// An ArgumentNullException is thrown if the example object is null. /// ////// An ArgumentException is thrown if the example object is not null and is not a valid type /// which can be converted to a CultureInfo. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The object to convert to a CultureInfo. public override object ConvertFrom(ITypeDescriptorContext typeDescriptorContext, CultureInfo cultureInfo, object source) { string cultureName = source as string; if (cultureName != null) { return CultureInfo.GetCultureInfoByIetfLanguageTag(cultureName); } throw GetConvertFromException(source); } ////// ConvertTo - Attempt to convert a CultureInfo to the given type /// ////// The object which was constructed. /// ////// An ArgumentNullException is thrown if the example object is null. /// ////// An ArgumentException is thrown if the example object is not null and is not a CultureInfo, /// or if the destinationType isn't one of the valid destination types. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The double to convert. /// The type to which to convert the CultureInfo. ////// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for CultureInfo.GetCultureInfo, not an arbitrary class/method /// [SecurityCritical] public override object ConvertTo(ITypeDescriptorContext typeDescriptorContext, CultureInfo cultureInfo, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } CultureInfo culture = value as CultureInfo; if (culture != null) { if (destinationType == typeof(string)) { return culture.IetfLanguageTag; } else if (destinationType == typeof(InstanceDescriptor)) { MethodInfo method = typeof(CultureInfo).GetMethod( "GetCultureInfo", BindingFlags.Static | BindingFlags.InvokeMethod | BindingFlags.Public, null, // use default binder new Type[] { typeof(string) }, null // default binder doesn't use parameter modifiers ); return new InstanceDescriptor(method, new object[] { culture.Name }); } } throw GetConvertToException(value, destinationType); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
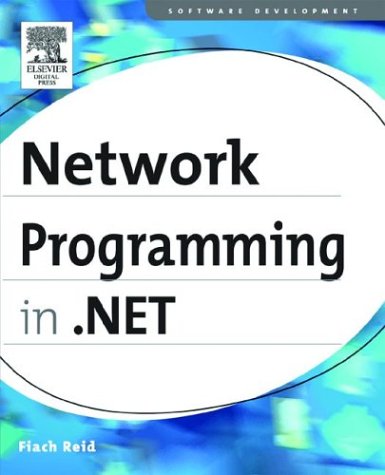
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ObjectQuery_EntitySqlExtensions.cs
- IpcChannelHelper.cs
- CultureTableRecord.cs
- ProfileServiceManager.cs
- CheckableControlBaseAdapter.cs
- FailedToStartupUIException.cs
- SqlRemoveConstantOrderBy.cs
- GeneratedCodeAttribute.cs
- DiagnosticTraceSource.cs
- IndexingContentUnit.cs
- UserUseLicenseDictionaryLoader.cs
- XAMLParseException.cs
- GcHandle.cs
- BitmapDownload.cs
- ListViewSelectEventArgs.cs
- GridViewEditEventArgs.cs
- SystemKeyConverter.cs
- CacheChildrenQuery.cs
- FillRuleValidation.cs
- Matrix3DStack.cs
- QuaternionKeyFrameCollection.cs
- WorkflowView.cs
- OracleCommand.cs
- RequestCachePolicyConverter.cs
- InputLangChangeRequestEvent.cs
- ConfigPathUtility.cs
- SystemTcpStatistics.cs
- ExcCanonicalXml.cs
- RefreshPropertiesAttribute.cs
- ObjectStateEntryBaseUpdatableDataRecord.cs
- SplitterCancelEvent.cs
- AssemblyName.cs
- EmptyCollection.cs
- SafeNativeMethods.cs
- GridViewItemAutomationPeer.cs
- CommandField.cs
- Registry.cs
- CryptoProvider.cs
- ToolBar.cs
- SQLMoneyStorage.cs
- _ListenerResponseStream.cs
- Activity.cs
- TextTreeInsertUndoUnit.cs
- PeerCollaborationPermission.cs
- TextFormatterContext.cs
- AsyncDataRequest.cs
- Mapping.cs
- TreeView.cs
- PartialTrustVisibleAssemblyCollection.cs
- ProviderConnectionPointCollection.cs
- MemberDomainMap.cs
- UrlMappingCollection.cs
- HttpPostedFile.cs
- XPathSingletonIterator.cs
- ComplexBindingPropertiesAttribute.cs
- PocoEntityKeyStrategy.cs
- GeometryHitTestParameters.cs
- AnimatedTypeHelpers.cs
- RegexCapture.cs
- ValuePatternIdentifiers.cs
- MobileComponentEditorPage.cs
- OleDbReferenceCollection.cs
- RadioButtonAutomationPeer.cs
- CodeMemberProperty.cs
- EndOfStreamException.cs
- RequestTimeoutManager.cs
- TimerEventSubscription.cs
- BamlLocalizer.cs
- StyleSheetDesigner.cs
- EntityViewGenerationAttribute.cs
- BasicExpandProvider.cs
- ActivationServices.cs
- Encoder.cs
- AnimationStorage.cs
- HttpClientProtocol.cs
- SmtpTransport.cs
- MDIControlStrip.cs
- IdentityManager.cs
- FigureParaClient.cs
- ObjectIDGenerator.cs
- Clock.cs
- ClassDataContract.cs
- UniformGrid.cs
- ObsoleteAttribute.cs
- SymmetricKey.cs
- XmlDigitalSignatureProcessor.cs
- XmlAttributeAttribute.cs
- CapabilitiesPattern.cs
- ApplyTemplatesAction.cs
- NavigationExpr.cs
- WorkflowInstance.cs
- CatalogZoneBase.cs
- XPathNodeHelper.cs
- ResourceKey.cs
- CategoryValueConverter.cs
- FontClient.cs
- ExpressionBindings.cs
- XmlChildEnumerator.cs
- VerificationException.cs
- MulticastNotSupportedException.cs