Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / Microsoft / Scripting / Ast / LoopExpression.cs / 1305376 / LoopExpression.cs
/* **************************************************************************** * * Copyright (c) Microsoft Corporation. * * This source code is subject to terms and conditions of the Microsoft Public License. A * copy of the license can be found in the License.html file at the root of this distribution. If * you cannot locate the Microsoft Public License, please send an email to * dlr@microsoft.com. By using this source code in any fashion, you are agreeing to be bound * by the terms of the Microsoft Public License. * * You must not remove this notice, or any other, from this software. * * * ***************************************************************************/ using System.Diagnostics; using System.Dynamic.Utils; #if SILVERLIGHT using System.Core; #endif namespace System.Linq.Expressions { ////// Represents an infinite loop. It can be exited with "break". /// #if !SILVERLIGHT [DebuggerTypeProxy(typeof(Expression.LoopExpressionProxy))] #endif public sealed class LoopExpression : Expression { private readonly Expression _body; private readonly LabelTarget _break; private readonly LabelTarget _continue; internal LoopExpression(Expression body, LabelTarget @break, LabelTarget @continue) { _body = body; _break = @break; _continue = @continue; } ////// Gets the static type of the expression that this ///represents. /// The public sealed override Type Type { get { return _break == null ? typeof(void) : _break.Type; } } ///that represents the static type of the expression. /// Returns the node type of this Expression. Extension nodes should return /// ExpressionType.Extension when overriding this method. /// ///The public sealed override ExpressionType NodeType { get { return ExpressionType.Loop; } } ///of the expression. /// Gets the public Expression Body { get { return _body; } } ///that is the body of the loop. /// /// Gets the public LabelTarget BreakLabel { get { return _break; } } ///that is used by the loop body as a break statement target. /// /// Gets the public LabelTarget ContinueLabel { get { return _continue; } } ///that is used by the loop body as a continue statement target. /// /// Dispatches to the specific visit method for this node type. /// protected internal override Expression Accept(ExpressionVisitor visitor) { return visitor.VisitLoop(this); } ////// Creates a new expression that is like this one, but using the /// supplied children. If all of the children are the same, it will /// return this expression. /// /// Theproperty of the result. /// The property of the result. /// The property of the result. /// This expression if no children changed, or an expression with the updated children. public LoopExpression Update(LabelTarget breakLabel, LabelTarget continueLabel, Expression body) { if (breakLabel == BreakLabel && continueLabel == ContinueLabel && body == Body) { return this; } return Expression.Loop(body, breakLabel, continueLabel); } } public partial class Expression { ////// Creates a /// The body of the loop. ///with the given body. /// The created public static LoopExpression Loop(Expression body) { return Loop(body, null); } ///. /// Creates a /// The body of the loop. /// The break target used by the loop body. ///with the given body and break target. /// The created public static LoopExpression Loop(Expression body, LabelTarget @break) { return Loop(body, @break, null); } ///. /// Creates a /// The body of the loop. /// The break target used by the loop body. /// The continue target used by the loop body. ///with the given body. /// The created public static LoopExpression Loop(Expression body, LabelTarget @break, LabelTarget @continue) { RequiresCanRead(body, "body"); if (@continue != null && @continue.Type != typeof(void)) throw Error.LabelTypeMustBeVoid(); return new LoopExpression(body, @break, @continue); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved..
Link Menu
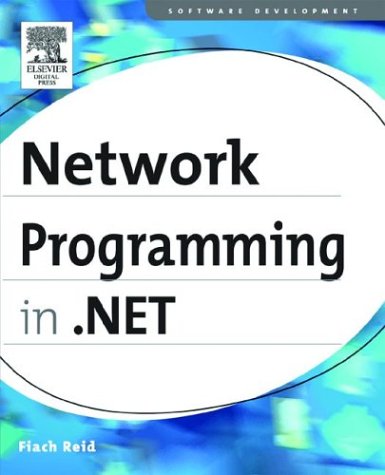
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BitmapEffectState.cs
- Error.cs
- TextBox.cs
- BaseDataBoundControl.cs
- DbDataSourceEnumerator.cs
- KeyBinding.cs
- WebPartUserCapability.cs
- OneOfConst.cs
- SafeNativeMethodsCLR.cs
- DynamicResourceExtension.cs
- FocusWithinProperty.cs
- SchemaSetCompiler.cs
- XDeferredAxisSource.cs
- PageMediaType.cs
- BufferAllocator.cs
- ReflectPropertyDescriptor.cs
- XamlRtfConverter.cs
- CompilerState.cs
- XPathAncestorIterator.cs
- ColorContextHelper.cs
- OleDbSchemaGuid.cs
- PropertyInfoSet.cs
- CellIdBoolean.cs
- RecognitionResult.cs
- Message.cs
- Atom10FormatterFactory.cs
- UnsettableComboBox.cs
- ToolStripRenderEventArgs.cs
- TablePattern.cs
- RectValueSerializer.cs
- ExceptionHandlers.cs
- SafeSecurityHelper.cs
- StreamWriter.cs
- WindowsRegion.cs
- Action.cs
- CodeDelegateCreateExpression.cs
- RowToFieldTransformer.cs
- storepermissionattribute.cs
- ClientRolePrincipal.cs
- WebProxyScriptElement.cs
- BinaryObjectInfo.cs
- SqlMethodTransformer.cs
- ExpressionNode.cs
- SchemaMapping.cs
- DataServiceConfiguration.cs
- LifetimeServices.cs
- TraceFilter.cs
- XmlIncludeAttribute.cs
- UnauthorizedWebPart.cs
- Attachment.cs
- PageParser.cs
- UInt32Storage.cs
- FontDriver.cs
- SerializationSectionGroup.cs
- PowerModeChangedEventArgs.cs
- CombinedGeometry.cs
- BrowsableAttribute.cs
- GridViewCancelEditEventArgs.cs
- TdsParserStateObject.cs
- GeneralTransform3DGroup.cs
- DynamicPropertyHolder.cs
- ImportDesigner.xaml.cs
- ImageListImage.cs
- XmlSchemaValidationException.cs
- _NTAuthentication.cs
- HostSecurityManager.cs
- HtmlControlPersistable.cs
- DataGridViewTextBoxCell.cs
- Int32EqualityComparer.cs
- LogLogRecord.cs
- DataGridViewUtilities.cs
- UrlPath.cs
- SuppressMessageAttribute.cs
- ToolStripCollectionEditor.cs
- SelectionPatternIdentifiers.cs
- Msec.cs
- CustomWebEventKey.cs
- TypeBuilderInstantiation.cs
- TypefaceMap.cs
- _StreamFramer.cs
- PathData.cs
- HMAC.cs
- WindowsListViewGroupHelper.cs
- HtmlControlPersistable.cs
- FormsAuthenticationTicket.cs
- AppearanceEditorPart.cs
- HtmlTextArea.cs
- SqlBooleanMismatchVisitor.cs
- HttpRequestTraceRecord.cs
- ProviderSettings.cs
- DataControlButton.cs
- CompoundFileStreamReference.cs
- ResourceCategoryAttribute.cs
- CharEnumerator.cs
- KeyboardDevice.cs
- CapabilitiesRule.cs
- CompiledQuery.cs
- RadioButtonRenderer.cs
- DataListItemCollection.cs
- SystemPens.cs