Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / Net / System / Net / Mail / MimeMultiPart.cs / 1 / MimeMultiPart.cs
using System; using System.IO; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Globalization; namespace System.Net.Mime { ////// Summary description for MimeMultiPart. /// internal class MimeMultiPart:MimeBasePart { Collectionparts; static int boundary; AsyncCallback mimePartSentCallback; internal MimeMultiPart(MimeMultiPartType type) { MimeMultiPartType = type; } internal MimeMultiPartType MimeMultiPartType { /* // Consider removing. get { return GetPartType(); } */ set { if (value > MimeMultiPartType.Related || value < MimeMultiPartType.Mixed) { throw new NotSupportedException(value.ToString()); } SetType(value); } } void SetType(MimeMultiPartType type) { ContentType.MediaType = "multipart" + "/" + type.ToString().ToLower(CultureInfo.InvariantCulture); ContentType.Boundary = GetNextBoundary(); } /* // Consider removing. MimeMultiPartType GetPartType() { switch (ContentType.MediaType) { case "multipart/alternative": return MimeMultiPartType.Alternative; case "multipart/mixed": return MimeMultiPartType.Mixed; case "multipart/parallel": return MimeMultiPartType.Parallel; case "multipart/related": return MimeMultiPartType.Related; } return MimeMultiPartType.Unknown; } */ internal Collection Parts { get{ if (parts == null) { parts = new Collection (); } return parts; } } internal void Complete(IAsyncResult result, Exception e){ //if we already completed and we got called again, //it mean's that there was an exception in the callback and we //should just rethrow it. MimePartContext context = (MimePartContext)result.AsyncState; if (context.completed) { throw e; } try{ context.outputStream.Close(); } catch(Exception ex){ if (e == null) { e = ex; } } catch { if (e == null) { e = new Exception(SR.GetString(SR.net_nonClsCompliantException)); } } context.completed = true; context.result.InvokeCallback(e); } internal void MimeWriterCloseCallback(IAsyncResult result) { if (result.CompletedSynchronously ) { return; } ((MimePartContext)result.AsyncState).completedSynchronously = false; try{ MimeWriterCloseCallbackHandler(result); } catch (Exception e) { Complete(result,e); } catch { Complete(result, new Exception(SR.GetString(SR.net_nonClsCompliantException))); } } void MimeWriterCloseCallbackHandler(IAsyncResult result) { MimePartContext context = (MimePartContext)result.AsyncState; ((MimeWriter)context.writer).EndClose(result); Complete(result,null); } internal void MimePartSentCallback(IAsyncResult result) { if (result.CompletedSynchronously ) { return; } ((MimePartContext)result.AsyncState).completedSynchronously = false; try{ MimePartSentCallbackHandler(result); } catch (Exception e) { Complete(result,e); } catch { Complete(result, new Exception(SR.GetString(SR.net_nonClsCompliantException))); } } void MimePartSentCallbackHandler(IAsyncResult result) { MimePartContext context = (MimePartContext)result.AsyncState; MimeBasePart part = (MimeBasePart)context.partsEnumerator.Current; part.EndSend(result); if (context.partsEnumerator.MoveNext()) { part = (MimeBasePart)context.partsEnumerator.Current; IAsyncResult sendResult = part.BeginSend(context.writer, mimePartSentCallback, context); if (sendResult.CompletedSynchronously) { MimePartSentCallbackHandler(sendResult); } return; } else { IAsyncResult closeResult = ((MimeWriter)context.writer).BeginClose(new AsyncCallback(MimeWriterCloseCallback), context); if (closeResult.CompletedSynchronously) { MimeWriterCloseCallbackHandler(closeResult); } } } internal void ContentStreamCallback(IAsyncResult result) { if (result.CompletedSynchronously ) { return; } ((MimePartContext)result.AsyncState).completedSynchronously = false; try{ ContentStreamCallbackHandler(result); } catch (Exception e) { Complete(result,e); } catch { Complete(result, new Exception(SR.GetString(SR.net_nonClsCompliantException))); } } void ContentStreamCallbackHandler(IAsyncResult result) { MimePartContext context = (MimePartContext)result.AsyncState; context.outputStream = context.writer.EndGetContentStream(result); context.writer = new MimeWriter(context.outputStream, ContentType.Boundary); if (context.partsEnumerator.MoveNext()) { MimeBasePart part = (MimeBasePart)context.partsEnumerator.Current; mimePartSentCallback = new AsyncCallback(MimePartSentCallback); IAsyncResult sendResult = part.BeginSend(context.writer, mimePartSentCallback, context); if (sendResult.CompletedSynchronously) { MimePartSentCallbackHandler(sendResult); } return; } else { IAsyncResult closeResult = ((MimeWriter)context.writer).BeginClose(new AsyncCallback(MimeWriterCloseCallback),context); if (closeResult.CompletedSynchronously) { MimeWriterCloseCallbackHandler(closeResult); } } } internal override IAsyncResult BeginSend(BaseWriter writer, AsyncCallback callback, object state) { writer.WriteHeaders(Headers); MimePartAsyncResult result = new MimePartAsyncResult(this, state, callback); MimePartContext context = new MimePartContext(writer, result, Parts.GetEnumerator()); IAsyncResult contentResult = writer.BeginGetContentStream(new AsyncCallback(ContentStreamCallback), context); if (contentResult.CompletedSynchronously) { ContentStreamCallbackHandler(contentResult); } return result; } internal class MimePartContext { internal MimePartContext(BaseWriter writer, LazyAsyncResult result, IEnumerator partsEnumerator) { this.writer = writer; this.result = result; this.partsEnumerator = partsEnumerator; } internal IEnumerator partsEnumerator; internal Stream outputStream; internal LazyAsyncResult result; internal BaseWriter writer; internal bool completed; internal bool completedSynchronously = true; } internal override void Send(BaseWriter writer) { writer.WriteHeaders(Headers); Stream outputStream = writer.GetContentStream(); MimeWriter mimeWriter = new MimeWriter(outputStream, ContentType.Boundary); foreach (MimeBasePart part in Parts) { part.Send(mimeWriter); } mimeWriter.Close(); outputStream.Close(); } internal string GetNextBoundary() { string boundaryString = "--boundary_" + boundary.ToString(CultureInfo.InvariantCulture)+"_"+Guid.NewGuid().ToString(null, CultureInfo.InvariantCulture); boundary++; return boundaryString; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.IO; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Globalization; namespace System.Net.Mime { /// /// Summary description for MimeMultiPart. /// internal class MimeMultiPart:MimeBasePart { Collectionparts; static int boundary; AsyncCallback mimePartSentCallback; internal MimeMultiPart(MimeMultiPartType type) { MimeMultiPartType = type; } internal MimeMultiPartType MimeMultiPartType { /* // Consider removing. get { return GetPartType(); } */ set { if (value > MimeMultiPartType.Related || value < MimeMultiPartType.Mixed) { throw new NotSupportedException(value.ToString()); } SetType(value); } } void SetType(MimeMultiPartType type) { ContentType.MediaType = "multipart" + "/" + type.ToString().ToLower(CultureInfo.InvariantCulture); ContentType.Boundary = GetNextBoundary(); } /* // Consider removing. MimeMultiPartType GetPartType() { switch (ContentType.MediaType) { case "multipart/alternative": return MimeMultiPartType.Alternative; case "multipart/mixed": return MimeMultiPartType.Mixed; case "multipart/parallel": return MimeMultiPartType.Parallel; case "multipart/related": return MimeMultiPartType.Related; } return MimeMultiPartType.Unknown; } */ internal Collection Parts { get{ if (parts == null) { parts = new Collection (); } return parts; } } internal void Complete(IAsyncResult result, Exception e){ //if we already completed and we got called again, //it mean's that there was an exception in the callback and we //should just rethrow it. MimePartContext context = (MimePartContext)result.AsyncState; if (context.completed) { throw e; } try{ context.outputStream.Close(); } catch(Exception ex){ if (e == null) { e = ex; } } catch { if (e == null) { e = new Exception(SR.GetString(SR.net_nonClsCompliantException)); } } context.completed = true; context.result.InvokeCallback(e); } internal void MimeWriterCloseCallback(IAsyncResult result) { if (result.CompletedSynchronously ) { return; } ((MimePartContext)result.AsyncState).completedSynchronously = false; try{ MimeWriterCloseCallbackHandler(result); } catch (Exception e) { Complete(result,e); } catch { Complete(result, new Exception(SR.GetString(SR.net_nonClsCompliantException))); } } void MimeWriterCloseCallbackHandler(IAsyncResult result) { MimePartContext context = (MimePartContext)result.AsyncState; ((MimeWriter)context.writer).EndClose(result); Complete(result,null); } internal void MimePartSentCallback(IAsyncResult result) { if (result.CompletedSynchronously ) { return; } ((MimePartContext)result.AsyncState).completedSynchronously = false; try{ MimePartSentCallbackHandler(result); } catch (Exception e) { Complete(result,e); } catch { Complete(result, new Exception(SR.GetString(SR.net_nonClsCompliantException))); } } void MimePartSentCallbackHandler(IAsyncResult result) { MimePartContext context = (MimePartContext)result.AsyncState; MimeBasePart part = (MimeBasePart)context.partsEnumerator.Current; part.EndSend(result); if (context.partsEnumerator.MoveNext()) { part = (MimeBasePart)context.partsEnumerator.Current; IAsyncResult sendResult = part.BeginSend(context.writer, mimePartSentCallback, context); if (sendResult.CompletedSynchronously) { MimePartSentCallbackHandler(sendResult); } return; } else { IAsyncResult closeResult = ((MimeWriter)context.writer).BeginClose(new AsyncCallback(MimeWriterCloseCallback), context); if (closeResult.CompletedSynchronously) { MimeWriterCloseCallbackHandler(closeResult); } } } internal void ContentStreamCallback(IAsyncResult result) { if (result.CompletedSynchronously ) { return; } ((MimePartContext)result.AsyncState).completedSynchronously = false; try{ ContentStreamCallbackHandler(result); } catch (Exception e) { Complete(result,e); } catch { Complete(result, new Exception(SR.GetString(SR.net_nonClsCompliantException))); } } void ContentStreamCallbackHandler(IAsyncResult result) { MimePartContext context = (MimePartContext)result.AsyncState; context.outputStream = context.writer.EndGetContentStream(result); context.writer = new MimeWriter(context.outputStream, ContentType.Boundary); if (context.partsEnumerator.MoveNext()) { MimeBasePart part = (MimeBasePart)context.partsEnumerator.Current; mimePartSentCallback = new AsyncCallback(MimePartSentCallback); IAsyncResult sendResult = part.BeginSend(context.writer, mimePartSentCallback, context); if (sendResult.CompletedSynchronously) { MimePartSentCallbackHandler(sendResult); } return; } else { IAsyncResult closeResult = ((MimeWriter)context.writer).BeginClose(new AsyncCallback(MimeWriterCloseCallback),context); if (closeResult.CompletedSynchronously) { MimeWriterCloseCallbackHandler(closeResult); } } } internal override IAsyncResult BeginSend(BaseWriter writer, AsyncCallback callback, object state) { writer.WriteHeaders(Headers); MimePartAsyncResult result = new MimePartAsyncResult(this, state, callback); MimePartContext context = new MimePartContext(writer, result, Parts.GetEnumerator()); IAsyncResult contentResult = writer.BeginGetContentStream(new AsyncCallback(ContentStreamCallback), context); if (contentResult.CompletedSynchronously) { ContentStreamCallbackHandler(contentResult); } return result; } internal class MimePartContext { internal MimePartContext(BaseWriter writer, LazyAsyncResult result, IEnumerator partsEnumerator) { this.writer = writer; this.result = result; this.partsEnumerator = partsEnumerator; } internal IEnumerator partsEnumerator; internal Stream outputStream; internal LazyAsyncResult result; internal BaseWriter writer; internal bool completed; internal bool completedSynchronously = true; } internal override void Send(BaseWriter writer) { writer.WriteHeaders(Headers); Stream outputStream = writer.GetContentStream(); MimeWriter mimeWriter = new MimeWriter(outputStream, ContentType.Boundary); foreach (MimeBasePart part in Parts) { part.Send(mimeWriter); } mimeWriter.Close(); outputStream.Close(); } internal string GetNextBoundary() { string boundaryString = "--boundary_" + boundary.ToString(CultureInfo.InvariantCulture)+"_"+Guid.NewGuid().ToString(null, CultureInfo.InvariantCulture); boundary++; return boundaryString; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
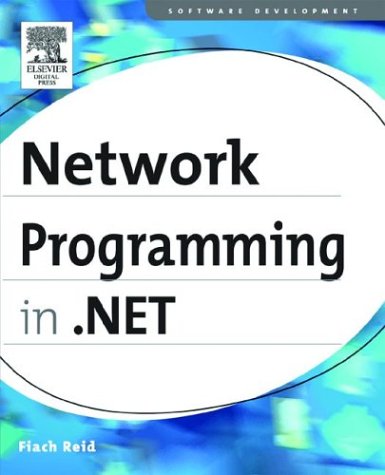
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TimeManager.cs
- UnaryQueryOperator.cs
- PrintPreviewGraphics.cs
- TdsValueSetter.cs
- ContentFilePart.cs
- GuidConverter.cs
- OutputScopeManager.cs
- ISCIIEncoding.cs
- TextParagraphProperties.cs
- CancellationHandler.cs
- KeyPressEvent.cs
- PointLight.cs
- AttributeProviderAttribute.cs
- Rule.cs
- Reference.cs
- CLSCompliantAttribute.cs
- FormatVersion.cs
- SqlServer2KCompatibilityCheck.cs
- ComponentDispatcher.cs
- ToolStrip.cs
- XmlConverter.cs
- DataGridViewDataConnection.cs
- CompareInfo.cs
- GlyphTypeface.cs
- GridPattern.cs
- URI.cs
- Options.cs
- CriticalFinalizerObject.cs
- SafeUserTokenHandle.cs
- MultilineStringConverter.cs
- PlainXmlSerializer.cs
- SqlDataSourceDesigner.cs
- SelectionPatternIdentifiers.cs
- GetPageCompletedEventArgs.cs
- RenamedEventArgs.cs
- FileVersionInfo.cs
- DataGridViewRowPostPaintEventArgs.cs
- XPathNavigator.cs
- TypedElement.cs
- WbemException.cs
- KeySplineConverter.cs
- StoreContentChangedEventArgs.cs
- XmlSequenceWriter.cs
- ListBoxChrome.cs
- SqlUserDefinedTypeAttribute.cs
- FrameDimension.cs
- SettingsPropertyValue.cs
- XmlSortKey.cs
- AsyncResult.cs
- SingleAnimationBase.cs
- Mappings.cs
- XmlAutoDetectWriter.cs
- DetailsViewRow.cs
- VirtualDirectoryMappingCollection.cs
- OleDbStruct.cs
- Int64.cs
- BindableAttribute.cs
- InstanceKeyCompleteException.cs
- TimeoutException.cs
- LOSFormatter.cs
- BinarySecretSecurityToken.cs
- WebEvents.cs
- SoapBinding.cs
- Win32Exception.cs
- WindowsImpersonationContext.cs
- JavaScriptObjectDeserializer.cs
- DataContractSerializerMessageContractImporter.cs
- DataGridViewAddColumnDialog.cs
- SqlClientMetaDataCollectionNames.cs
- HotSpotCollection.cs
- SocketAddress.cs
- DataFormats.cs
- StateItem.cs
- FontInfo.cs
- Attributes.cs
- ExtentCqlBlock.cs
- DataSourceCache.cs
- FormCollection.cs
- DoubleConverter.cs
- WebPartTransformerCollection.cs
- VectorCollectionConverter.cs
- Base64Encoder.cs
- PartialCachingAttribute.cs
- ObjectListFieldsPage.cs
- GridViewColumnCollectionChangedEventArgs.cs
- NodeLabelEditEvent.cs
- HandleCollector.cs
- CompiledQueryCacheEntry.cs
- EntityProviderServices.cs
- AdRotator.cs
- InstancePersistenceContext.cs
- Misc.cs
- GlobalId.cs
- PageAdapter.cs
- CompilerScopeManager.cs
- OperationContext.cs
- ParseChildrenAsPropertiesAttribute.cs
- HostProtectionPermission.cs
- controlskin.cs
- GridView.cs