Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Base / MS / Internal / IO / Packaging / CompoundFile / UserUseLicenseDictionaryLoader.cs / 1 / UserUseLicenseDictionaryLoader.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // This class is a helper to load a set of associations between a user and the use license // granted to that user. // // History: // 04/19/2005: LGolding: Initial implementation. // 03/08/2006: IgorBel: switch from a dictionary to a Load helper // // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Text; using System.IO; using System.IO.Packaging; using System.Windows; using System.Security.RightsManagement; using MS.Internal; namespace MS.Internal.IO.Packaging.CompoundFile { internal class UserUseLicenseDictionaryLoader { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constructor. /// internal UserUseLicenseDictionaryLoader(RightsManagementEncryptionTransform rmet) { _dict = new Dictionary(ContentUser._contentUserComparer); // // This constructor is only called from RightsManagementEncryptionTransform // .GetEmbeddedUseLicenses. That method passes "this" as the parameter. // So it can't possibly be null. // Invariant.Assert(rmet != null); Load(rmet); } #endregion Constructors //------------------------------------------------------ // // Internal Properties // //----------------------------------------------------- #region Internal Properties internal Dictionary LoadedDictionary { get { return _dict; } } #endregion Internal Properties //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ #region Private Methods /// /// Load the contents of the dictionary from the compound file. /// /// /// The object that knows how to load use license data from the compound file. /// ////// If private void Load(RightsManagementEncryptionTransform rmet ) { rmet.EnumUseLicenseStreams( new RightsManagementEncryptionTransform.UseLicenseStreamCallback( this.AddUseLicenseFromStreamToDictionary ), null ); } ///is null. /// /// Callback function used by Load. Called once for each use license stream /// in the compound file. Extracts the user and use license from the specified /// stream. /// /// /// The object that knows how to extract license information from the compound file. /// /// /// The stream containing the user/user license pair to be added to the dictionary. /// /// /// Caller-supplied parameter to EnumUseLicenseStreams. Not used. /// /// /// Set to true if the callback function wants to stop the enumeration. This callback /// function never wants to stop the enumeration, so this parameter is not used. /// private void AddUseLicenseFromStreamToDictionary( RightsManagementEncryptionTransform rmet, StreamInfo si, object param, ref bool stop ) { ContentUser user; using (Stream stream = si.GetStream(FileMode.Open, FileAccess.Read)) { using(BinaryReader utf8Reader = new BinaryReader(stream, _utf8Encoding)) { UseLicense useLicense = rmet.LoadUseLicenseAndUserFromStream(utf8Reader, out user); _dict.Add(user, useLicense); } } } #endregion Private Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields // // The object that provides the implementation of the IDictionary methods. // private Dictionary_dict; // // Text encoding object used to read or write publish licenses and use licenses. // private UTF8Encoding _utf8Encoding = new UTF8Encoding(); #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // This class is a helper to load a set of associations between a user and the use license // granted to that user. // // History: // 04/19/2005: LGolding: Initial implementation. // 03/08/2006: IgorBel: switch from a dictionary to a Load helper // // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Text; using System.IO; using System.IO.Packaging; using System.Windows; using System.Security.RightsManagement; using MS.Internal; namespace MS.Internal.IO.Packaging.CompoundFile { internal class UserUseLicenseDictionaryLoader { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constructor. /// internal UserUseLicenseDictionaryLoader(RightsManagementEncryptionTransform rmet) { _dict = new Dictionary(ContentUser._contentUserComparer); // // This constructor is only called from RightsManagementEncryptionTransform // .GetEmbeddedUseLicenses. That method passes "this" as the parameter. // So it can't possibly be null. // Invariant.Assert(rmet != null); Load(rmet); } #endregion Constructors //------------------------------------------------------ // // Internal Properties // //----------------------------------------------------- #region Internal Properties internal Dictionary LoadedDictionary { get { return _dict; } } #endregion Internal Properties //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ #region Private Methods /// /// Load the contents of the dictionary from the compound file. /// /// /// The object that knows how to load use license data from the compound file. /// ////// If private void Load(RightsManagementEncryptionTransform rmet ) { rmet.EnumUseLicenseStreams( new RightsManagementEncryptionTransform.UseLicenseStreamCallback( this.AddUseLicenseFromStreamToDictionary ), null ); } ///is null. /// /// Callback function used by Load. Called once for each use license stream /// in the compound file. Extracts the user and use license from the specified /// stream. /// /// /// The object that knows how to extract license information from the compound file. /// /// /// The stream containing the user/user license pair to be added to the dictionary. /// /// /// Caller-supplied parameter to EnumUseLicenseStreams. Not used. /// /// /// Set to true if the callback function wants to stop the enumeration. This callback /// function never wants to stop the enumeration, so this parameter is not used. /// private void AddUseLicenseFromStreamToDictionary( RightsManagementEncryptionTransform rmet, StreamInfo si, object param, ref bool stop ) { ContentUser user; using (Stream stream = si.GetStream(FileMode.Open, FileAccess.Read)) { using(BinaryReader utf8Reader = new BinaryReader(stream, _utf8Encoding)) { UseLicense useLicense = rmet.LoadUseLicenseAndUserFromStream(utf8Reader, out user); _dict.Add(user, useLicense); } } } #endregion Private Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields // // The object that provides the implementation of the IDictionary methods. // private Dictionary_dict; // // Text encoding object used to read or write publish licenses and use licenses. // private UTF8Encoding _utf8Encoding = new UTF8Encoding(); #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
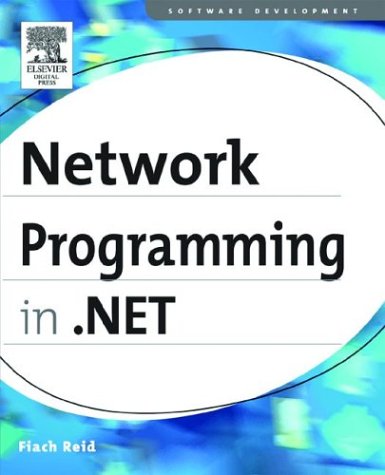
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BaseValidator.cs
- ScriptResourceMapping.cs
- SQLChars.cs
- XmlAttributeProperties.cs
- IteratorDescriptor.cs
- Emitter.cs
- ObjectStateManagerMetadata.cs
- SectionXmlInfo.cs
- TreeView.cs
- webclient.cs
- StrongNameKeyPair.cs
- EnumMember.cs
- Label.cs
- EventWaitHandleSecurity.cs
- GridViewUpdateEventArgs.cs
- JsonWriterDelegator.cs
- TdsRecordBufferSetter.cs
- InputReport.cs
- RectAnimationBase.cs
- ListControl.cs
- ThreadStartException.cs
- SessionStateSection.cs
- AlternateView.cs
- WhitespaceReader.cs
- FormatStringEditor.cs
- WindowsFormsHostPropertyMap.cs
- RtfControls.cs
- CodeDomConfigurationHandler.cs
- DataBoundLiteralControl.cs
- EntityViewContainer.cs
- InvokeSchedule.cs
- Code.cs
- ManualResetEventSlim.cs
- PeerOutputChannel.cs
- TypeTypeConverter.cs
- NativeMethods.cs
- ShortcutKeysEditor.cs
- DataKey.cs
- Authorization.cs
- AttributeQuery.cs
- ManagementObjectCollection.cs
- DesignerWidgets.cs
- ElementFactory.cs
- StringFreezingAttribute.cs
- InheritanceContextChangedEventManager.cs
- ValidatingPropertiesEventArgs.cs
- UnauthorizedAccessException.cs
- ValidationHelper.cs
- XmlSchemaObjectTable.cs
- XmlCharacterData.cs
- MatrixStack.cs
- Point3DCollection.cs
- CompilerError.cs
- TreeNodeClickEventArgs.cs
- HtmlControlPersistable.cs
- ListViewInsertedEventArgs.cs
- Size3D.cs
- HttpProtocolImporter.cs
- TrackingStringDictionary.cs
- ListViewGroupConverter.cs
- SequentialOutput.cs
- TextDecorationCollectionConverter.cs
- PropertyGrid.cs
- MessageQueueAccessControlEntry.cs
- RowSpanVector.cs
- IntSecurity.cs
- UserControlBuildProvider.cs
- XmlObjectSerializerWriteContextComplexJson.cs
- SoapExtensionTypeElement.cs
- PropertyGridDesigner.cs
- LocationUpdates.cs
- DataControlReference.cs
- GridViewEditEventArgs.cs
- BamlRecords.cs
- NetTcpSecurity.cs
- PersonalizationProvider.cs
- Camera.cs
- ObjRef.cs
- CodeSnippetCompileUnit.cs
- DependencyObject.cs
- EventLevel.cs
- WebPartEditorOkVerb.cs
- ObjectQueryProvider.cs
- MessagingDescriptionAttribute.cs
- ListViewGroupItemCollection.cs
- ClrProviderManifest.cs
- ToolStripComboBox.cs
- Identifier.cs
- EventWaitHandle.cs
- MetaTableHelper.cs
- EditorPartCollection.cs
- COM2IManagedPerPropertyBrowsingHandler.cs
- ValidationErrorCollection.cs
- FormViewCommandEventArgs.cs
- RepeaterItemEventArgs.cs
- DataObjectMethodAttribute.cs
- BindingMemberInfo.cs
- TransformGroup.cs
- TableLayoutRowStyleCollection.cs
- base64Transforms.cs