Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Description / ServiceAuthorizationBehavior.cs / 2 / ServiceAuthorizationBehavior.cs
//---------------------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------------------- namespace System.ServiceModel.Description { using System; using System.ServiceModel.Channels; using System.ServiceModel.Dispatcher; using System.ServiceModel; using System.Runtime.Serialization; using System.ServiceModel.Security; using System.Web.Security; using System.Collections.ObjectModel; using System.Collections.Generic; using System.IdentityModel.Claims; using System.IdentityModel.Policy; using System.Runtime.CompilerServices; public sealed class ServiceAuthorizationBehavior : IServiceBehavior { internal const bool DefaultImpersonateCallerForAllOperations = false; internal const PrincipalPermissionMode DefaultPrincipalPermissionMode = PrincipalPermissionMode.UseWindowsGroups; bool impersonateCallerForAllOperations; ReadOnlyCollectionexternalAuthorizationPolicies; ServiceAuthorizationManager serviceAuthorizationManager; PrincipalPermissionMode principalPermissionMode; object roleProvider; bool isExternalPoliciesSet; bool isAuthorizationManagerSet; bool isReadOnly; public ServiceAuthorizationBehavior() { this.impersonateCallerForAllOperations = DefaultImpersonateCallerForAllOperations; this.principalPermissionMode = DefaultPrincipalPermissionMode; } ServiceAuthorizationBehavior(ServiceAuthorizationBehavior other) { this.impersonateCallerForAllOperations = other.impersonateCallerForAllOperations; this.principalPermissionMode = other.principalPermissionMode; this.roleProvider = other.roleProvider; this.isExternalPoliciesSet = other.isExternalPoliciesSet; this.isAuthorizationManagerSet = other.isAuthorizationManagerSet; if (other.isExternalPoliciesSet || other.isAuthorizationManagerSet) { CopyAuthorizationPoliciesAndManager(other); } this.isReadOnly = other.isReadOnly; } public ReadOnlyCollection ExternalAuthorizationPolicies { get { return this.externalAuthorizationPolicies; } set { ThrowIfImmutable(); this.isExternalPoliciesSet = true; this.externalAuthorizationPolicies = value; } } public ServiceAuthorizationManager ServiceAuthorizationManager { get { return this.serviceAuthorizationManager; } set { ThrowIfImmutable(); this.isAuthorizationManagerSet = true; this.serviceAuthorizationManager = value; } } public PrincipalPermissionMode PrincipalPermissionMode { get { return this.principalPermissionMode; } set { if (!PrincipalPermissionModeHelper.IsDefined(value)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("value")); ThrowIfImmutable(); this.principalPermissionMode = value; } } public RoleProvider RoleProvider { get { return (RoleProvider)this.roleProvider; } set { ThrowIfImmutable(); this.roleProvider = value; } } public bool ImpersonateCallerForAllOperations { get { return this.impersonateCallerForAllOperations; } set { ThrowIfImmutable(); this.impersonateCallerForAllOperations = value; } } [MethodImpl(MethodImplOptions.NoInlining)] void ApplyAuthorizationPoliciesAndManager(DispatchRuntime behavior) { if (this.externalAuthorizationPolicies != null) { behavior.ExternalAuthorizationPolicies = this.externalAuthorizationPolicies; } if (this.serviceAuthorizationManager != null) { behavior.ServiceAuthorizationManager = this.serviceAuthorizationManager; } } [MethodImpl(MethodImplOptions.NoInlining)] void CopyAuthorizationPoliciesAndManager(ServiceAuthorizationBehavior other) { this.externalAuthorizationPolicies = other.externalAuthorizationPolicies; this.serviceAuthorizationManager = other.serviceAuthorizationManager; } [MethodImpl(MethodImplOptions.NoInlining)] void ApplyRoleProvider(DispatchRuntime behavior) { behavior.RoleProvider = (RoleProvider)this.roleProvider; } void IServiceBehavior.Validate(ServiceDescription description, ServiceHostBase serviceHostBase) { } void IServiceBehavior.AddBindingParameters(ServiceDescription description, ServiceHostBase serviceHostBase, Collection endpoints, BindingParameterCollection parameters) { } void IServiceBehavior.ApplyDispatchBehavior(ServiceDescription description, ServiceHostBase serviceHostBase) { if (description == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("description")); if (serviceHostBase == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("serviceHostBase")); for (int i=0; i
Link Menu
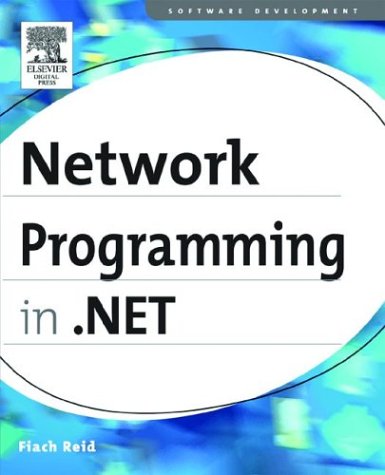
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ContextMenu.cs
- TextMarkerSource.cs
- MultilineStringConverter.cs
- DispatchChannelSink.cs
- TreeNodeCollectionEditor.cs
- MemoryRecordBuffer.cs
- IPCCacheManager.cs
- InputLanguageCollection.cs
- XmlSchemaExternal.cs
- SRDisplayNameAttribute.cs
- NativeCompoundFileAPIs.cs
- GeometryCombineModeValidation.cs
- RegisteredHiddenField.cs
- ComNativeDescriptor.cs
- MenuAutomationPeer.cs
- CatalogZoneBase.cs
- DataObjectEventArgs.cs
- Guid.cs
- EncryptedPackageFilter.cs
- BinarySerializer.cs
- ValidationSummaryDesigner.cs
- XmlSchemaException.cs
- AtomServiceDocumentSerializer.cs
- HijriCalendar.cs
- IERequestCache.cs
- PlainXmlDeserializer.cs
- WriteFileContext.cs
- ErrorFormatter.cs
- _FtpDataStream.cs
- WebPartConnection.cs
- BrowserInteropHelper.cs
- TextProviderWrapper.cs
- RegistrationServices.cs
- BrushMappingModeValidation.cs
- XmlFormatMapping.cs
- TypeTypeConverter.cs
- ImpersonationContext.cs
- AsyncCallback.cs
- WebScriptMetadataFormatter.cs
- UpdateCommandGenerator.cs
- SynchronizationContext.cs
- DbConnectionStringCommon.cs
- PropagatorResult.cs
- ForwardPositionQuery.cs
- ThreadSafeList.cs
- PropertyTab.cs
- SystemIPv4InterfaceProperties.cs
- EpmContentSerializerBase.cs
- ComplexTypeEmitter.cs
- UpdateCompiler.cs
- GlobalizationSection.cs
- FeatureSupport.cs
- SharedPersonalizationStateInfo.cs
- Tool.cs
- Interop.cs
- DmlSqlGenerator.cs
- SqlVersion.cs
- AssemblyUtil.cs
- AutomationPatternInfo.cs
- ObjectAnimationBase.cs
- AudioLevelUpdatedEventArgs.cs
- TreeNodeCollection.cs
- TrackBarRenderer.cs
- ParameterBuilder.cs
- EventLogger.cs
- ToolStripRendererSwitcher.cs
- PassportAuthenticationModule.cs
- ObjectSecurity.cs
- MemberAssignmentAnalysis.cs
- ParserExtension.cs
- MouseGestureValueSerializer.cs
- XamlPathDataSerializer.cs
- WaitHandleCannotBeOpenedException.cs
- SystemEvents.cs
- RepeaterItemEventArgs.cs
- MsmqException.cs
- DataSetUtil.cs
- WpfPayload.cs
- ColumnCollectionEditor.cs
- MailWriter.cs
- ViewgenGatekeeper.cs
- ZoneMembershipCondition.cs
- RelationshipNavigation.cs
- WmlImageAdapter.cs
- Model3DGroup.cs
- ComponentChangedEvent.cs
- TypeGenericEnumerableViewSchema.cs
- DispatcherObject.cs
- HealthMonitoringSection.cs
- DynamicFilterExpression.cs
- UInt16.cs
- XsdValidatingReader.cs
- EntryPointNotFoundException.cs
- SoapClientMessage.cs
- Geometry.cs
- WsrmFault.cs
- PlanCompilerUtil.cs
- CharConverter.cs
- Int64.cs
- CheckBoxAutomationPeer.cs