Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / Xml / System / Xml / schema / XmlSchemaExternal.cs / 1 / XmlSchemaExternal.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Schema { using System.Collections; using System.ComponentModel; using System.Xml.Serialization; ///public abstract class XmlSchemaExternal : XmlSchemaObject { string location; Uri baseUri; XmlSchema schema; string id; XmlAttribute[] moreAttributes; Compositor compositor; /// [XmlAttribute("schemaLocation", DataType="anyURI")] public string SchemaLocation { get { return location; } set { location = value; } } /// [XmlIgnore] public XmlSchema Schema { get { return schema; } set { schema = value; } } /// [XmlAttribute("id", DataType="ID")] public string Id { get { return id; } set { id = value; } } /// [XmlAnyAttribute] public XmlAttribute[] UnhandledAttributes { get { return moreAttributes; } set { moreAttributes = value; } } [XmlIgnore] internal Uri BaseUri { get { return baseUri; } set { baseUri = value; } } [XmlIgnore] internal override string IdAttribute { get { return Id; } set { Id = value; } } internal override void SetUnhandledAttributes(XmlAttribute[] moreAttributes) { this.moreAttributes = moreAttributes; } internal Compositor Compositor { get { return compositor; } set { compositor = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Schema { using System.Collections; using System.ComponentModel; using System.Xml.Serialization; ///public abstract class XmlSchemaExternal : XmlSchemaObject { string location; Uri baseUri; XmlSchema schema; string id; XmlAttribute[] moreAttributes; Compositor compositor; /// [XmlAttribute("schemaLocation", DataType="anyURI")] public string SchemaLocation { get { return location; } set { location = value; } } /// [XmlIgnore] public XmlSchema Schema { get { return schema; } set { schema = value; } } /// [XmlAttribute("id", DataType="ID")] public string Id { get { return id; } set { id = value; } } /// [XmlAnyAttribute] public XmlAttribute[] UnhandledAttributes { get { return moreAttributes; } set { moreAttributes = value; } } [XmlIgnore] internal Uri BaseUri { get { return baseUri; } set { baseUri = value; } } [XmlIgnore] internal override string IdAttribute { get { return Id; } set { Id = value; } } internal override void SetUnhandledAttributes(XmlAttribute[] moreAttributes) { this.moreAttributes = moreAttributes; } internal Compositor Compositor { get { return compositor; } set { compositor = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
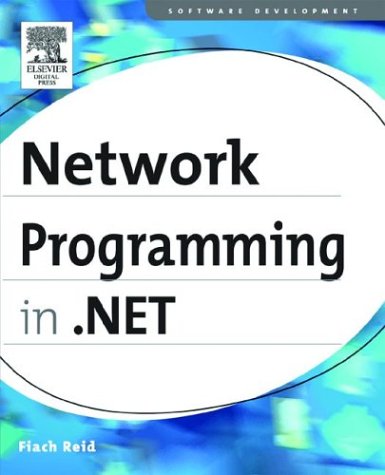
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PopupControlService.cs
- MetadataCollection.cs
- InfoCardRSAPKCS1KeyExchangeDeformatter.cs
- FloatSumAggregationOperator.cs
- OdbcInfoMessageEvent.cs
- Sql8ExpressionRewriter.cs
- ReadOnlyPropertyMetadata.cs
- ListMarkerLine.cs
- ISAPIWorkerRequest.cs
- ConfigurationManager.cs
- activationcontext.cs
- MouseGestureConverter.cs
- ConfigurationManagerInternalFactory.cs
- Label.cs
- SafeSecurityHelper.cs
- StandardCommands.cs
- ZipIOLocalFileDataDescriptor.cs
- UInt32Converter.cs
- AutoGeneratedFieldProperties.cs
- ExpressionNode.cs
- itemelement.cs
- MetabaseServerConfig.cs
- SortDescriptionCollection.cs
- StringInfo.cs
- SmiGettersStream.cs
- WizardPanelChangingEventArgs.cs
- DataSetMappper.cs
- ListBase.cs
- PropertyGridCommands.cs
- ADConnectionHelper.cs
- ZipIOExtraField.cs
- UnsafeNativeMethods.cs
- CodeSnippetCompileUnit.cs
- Opcode.cs
- MimeMapping.cs
- ToolStripDropDownClosingEventArgs.cs
- CircleEase.cs
- SynchronizationLockException.cs
- WindowsHyperlink.cs
- DictationGrammar.cs
- XmlNavigatorFilter.cs
- TabPanel.cs
- MetadataFile.cs
- CqlLexerHelpers.cs
- PropertyValueChangedEvent.cs
- DataGridViewMethods.cs
- Helpers.cs
- DecodeHelper.cs
- PeerTransportElement.cs
- GlobalizationAssembly.cs
- SettingsPropertyValue.cs
- KeySpline.cs
- ToolStripArrowRenderEventArgs.cs
- StartUpEventArgs.cs
- SqlTriggerAttribute.cs
- ComponentDispatcher.cs
- WebPartEventArgs.cs
- UpWmlPageAdapter.cs
- TextChange.cs
- TemplateBuilder.cs
- TextEvent.cs
- ReflectTypeDescriptionProvider.cs
- EventBuilder.cs
- MimeTextImporter.cs
- ValidationErrorCollection.cs
- Claim.cs
- SizeConverter.cs
- BuiltInPermissionSets.cs
- CustomAttribute.cs
- Interlocked.cs
- DBCommandBuilder.cs
- PropertyDescriptor.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- TextTreeObjectNode.cs
- RemotingAttributes.cs
- PositiveTimeSpanValidatorAttribute.cs
- InfoCardSchemas.cs
- HttpListenerPrefixCollection.cs
- ISSmlParser.cs
- SystemInformation.cs
- ParagraphResult.cs
- ResourceFallbackManager.cs
- PropertyCollection.cs
- HandleExceptionArgs.cs
- IssuedSecurityTokenProvider.cs
- OperatingSystem.cs
- AssemblyInfo.cs
- Blend.cs
- BuildTopDownAttribute.cs
- DbInsertCommandTree.cs
- WindowsListViewItem.cs
- EventWaitHandle.cs
- Substitution.cs
- Soap.cs
- SourceChangedEventArgs.cs
- unitconverter.cs
- ShaderRenderModeValidation.cs
- ServiceContractListItemList.cs
- ClosableStream.cs
- ByeOperation11AsyncResult.cs