Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / GrammarBuilding / itemelement.cs / 1 / itemelement.cs
//------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System.Collections.Generic; using System.Diagnostics; using System.Speech.Recognition; using System.Speech.Internal.SrgsParser; using System.Text; namespace System.Speech.Internal.GrammarBuilding { ////// /// #if VSCOMPILE [DebuggerDisplay ("{DebugSummary}")] #endif internal sealed class ItemElement : BuilderElements { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors ////// /// /// internal ItemElement (GrammarBuilderBase builder) : this (builder, 1, 1) { } ////// /// /// /// internal ItemElement (int minRepeat, int maxRepeat) : this ((GrammarBuilderBase) null, minRepeat, maxRepeat) { } ////// /// /// /// /// internal ItemElement (GrammarBuilderBase builder, int minRepeat, int maxRepeat) { if (builder != null) { Add (builder); } _minRepeat = minRepeat; _maxRepeat = maxRepeat; } ////// /// /// /// /// internal ItemElement (Listbuilders, int minRepeat, int maxRepeat) { foreach (GrammarBuilderBase builder in builders) { Items.Add (builder); } _minRepeat = minRepeat; _maxRepeat = maxRepeat; } /// /// /// /// internal ItemElement (GrammarBuilder builders) { foreach (GrammarBuilderBase builder in builders.InternalBuilder.Items) { Items.Add (builder); } } #endregion //******************************************************************** // // Public Methods // //******************************************************************* #region Public Methods /// TODOC <_include file='doc\SpeechAudioFormatInfo.uex' path='docs/doc[@for="SpeechAudioFormatInfo.Equals"]/*' /> public override bool Equals (object obj) { ItemElement refObj = obj as ItemElement; if (refObj == null) { return false; } if (!base.Equals (obj)) { return false; } return _minRepeat == refObj._minRepeat && _maxRepeat == refObj._maxRepeat; } /// TODOC <_include file='doc\SpeechAudioFormatInfo.uex' path='docs/doc[@for="SpeechAudioFormatInfo.GetHashCode"]/*' /> public override int GetHashCode () { return base.GetHashCode (); } #endregion //******************************************************************** // // Internal Methods // //******************************************************************** #region Internal Methods ////// /// ///internal override GrammarBuilderBase Clone () { ItemElement item = new ItemElement (_minRepeat, _maxRepeat); item.CloneItems (this); return item; } /// /// /// /// /// /// /// ///internal override IElement CreateElement (IElementFactory elementFactory, IElement parent, IRule rule, IdentifierCollection ruleIds) { // Create and return the real item (the item including the grammar) // for the current grammar IItem item = elementFactory.CreateItem (parent, rule, _minRepeat, _maxRepeat, 0.5f, 1f); // Create the children elements CreateChildrenElements (elementFactory, item, rule, ruleIds); return item; } #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Fields private readonly int _minRepeat = 1; private readonly int _maxRepeat = 1; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System.Collections.Generic; using System.Diagnostics; using System.Speech.Recognition; using System.Speech.Internal.SrgsParser; using System.Text; namespace System.Speech.Internal.GrammarBuilding { ////// /// #if VSCOMPILE [DebuggerDisplay ("{DebugSummary}")] #endif internal sealed class ItemElement : BuilderElements { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors ////// /// /// internal ItemElement (GrammarBuilderBase builder) : this (builder, 1, 1) { } ////// /// /// /// internal ItemElement (int minRepeat, int maxRepeat) : this ((GrammarBuilderBase) null, minRepeat, maxRepeat) { } ////// /// /// /// /// internal ItemElement (GrammarBuilderBase builder, int minRepeat, int maxRepeat) { if (builder != null) { Add (builder); } _minRepeat = minRepeat; _maxRepeat = maxRepeat; } ////// /// /// /// /// internal ItemElement (Listbuilders, int minRepeat, int maxRepeat) { foreach (GrammarBuilderBase builder in builders) { Items.Add (builder); } _minRepeat = minRepeat; _maxRepeat = maxRepeat; } /// /// /// /// internal ItemElement (GrammarBuilder builders) { foreach (GrammarBuilderBase builder in builders.InternalBuilder.Items) { Items.Add (builder); } } #endregion //******************************************************************** // // Public Methods // //******************************************************************* #region Public Methods /// TODOC <_include file='doc\SpeechAudioFormatInfo.uex' path='docs/doc[@for="SpeechAudioFormatInfo.Equals"]/*' /> public override bool Equals (object obj) { ItemElement refObj = obj as ItemElement; if (refObj == null) { return false; } if (!base.Equals (obj)) { return false; } return _minRepeat == refObj._minRepeat && _maxRepeat == refObj._maxRepeat; } /// TODOC <_include file='doc\SpeechAudioFormatInfo.uex' path='docs/doc[@for="SpeechAudioFormatInfo.GetHashCode"]/*' /> public override int GetHashCode () { return base.GetHashCode (); } #endregion //******************************************************************** // // Internal Methods // //******************************************************************** #region Internal Methods ////// /// ///internal override GrammarBuilderBase Clone () { ItemElement item = new ItemElement (_minRepeat, _maxRepeat); item.CloneItems (this); return item; } /// /// /// /// /// /// /// ///internal override IElement CreateElement (IElementFactory elementFactory, IElement parent, IRule rule, IdentifierCollection ruleIds) { // Create and return the real item (the item including the grammar) // for the current grammar IItem item = elementFactory.CreateItem (parent, rule, _minRepeat, _maxRepeat, 0.5f, 1f); // Create the children elements CreateChildrenElements (elementFactory, item, rule, ruleIds); return item; } #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Fields private readonly int _minRepeat = 1; private readonly int _maxRepeat = 1; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
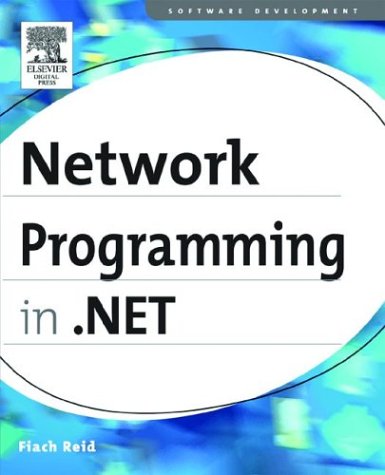
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WorkflowInstanceExtensionManager.cs
- GridViewRow.cs
- DeferredElementTreeState.cs
- Roles.cs
- FontStretchConverter.cs
- MetadataSet.cs
- OletxDependentTransaction.cs
- Documentation.cs
- ReadOnlyDataSourceView.cs
- util.cs
- SmtpAuthenticationManager.cs
- InstanceData.cs
- GPRECTF.cs
- DictionaryBase.cs
- Underline.cs
- MasterPageBuildProvider.cs
- DataGridViewToolTip.cs
- FocusChangedEventArgs.cs
- RijndaelManagedTransform.cs
- FormViewCommandEventArgs.cs
- GetPageCompletedEventArgs.cs
- OuterGlowBitmapEffect.cs
- TagNameToTypeMapper.cs
- DoubleStorage.cs
- InvalidateEvent.cs
- ClientUtils.cs
- ProtocolElementCollection.cs
- IgnoreSectionHandler.cs
- OdbcConnection.cs
- Application.cs
- HttpListenerException.cs
- LabelLiteral.cs
- localization.cs
- WebEventTraceProvider.cs
- ZipIOLocalFileDataDescriptor.cs
- VectorCollection.cs
- WebAdminConfigurationHelper.cs
- OpCodes.cs
- MappingSource.cs
- SignerInfo.cs
- AdornedElementPlaceholder.cs
- CommandID.cs
- Attributes.cs
- TextParagraphCache.cs
- ProjectionPath.cs
- safemediahandle.cs
- ItemsPanelTemplate.cs
- SqlClientFactory.cs
- DispatcherObject.cs
- ReferencedType.cs
- CacheSection.cs
- RemoteWebConfigurationHostStream.cs
- DataProviderNameConverter.cs
- TargetInvocationException.cs
- DecodeHelper.cs
- NumberFunctions.cs
- TdsParser.cs
- TargetException.cs
- ToolStripItemTextRenderEventArgs.cs
- InlinedLocationReference.cs
- TimelineClockCollection.cs
- CodeVariableReferenceExpression.cs
- FtpWebRequest.cs
- SecurityHelper.cs
- DesignerProperties.cs
- DataGridViewSortCompareEventArgs.cs
- TimeIntervalCollection.cs
- ReferenceEqualityComparer.cs
- Activator.cs
- ScriptReferenceEventArgs.cs
- DBBindings.cs
- Scene3D.cs
- StackSpiller.Bindings.cs
- CodePageUtils.cs
- ObjectListField.cs
- AdjustableArrowCap.cs
- HttpCachePolicyWrapper.cs
- DurableMessageDispatchInspector.cs
- SpellCheck.cs
- HybridDictionary.cs
- EventDescriptorCollection.cs
- SizeKeyFrameCollection.cs
- CodeAttributeArgument.cs
- ElementMarkupObject.cs
- ParallelTimeline.cs
- AutomationPropertyInfo.cs
- XNameConverter.cs
- StringSorter.cs
- TdsParserSafeHandles.cs
- EventHandlerService.cs
- Switch.cs
- PriorityRange.cs
- LoginView.cs
- MessageDecoder.cs
- SafeRightsManagementHandle.cs
- PageThemeCodeDomTreeGenerator.cs
- Material.cs
- LookupBindingPropertiesAttribute.cs
- RenderContext.cs
- Property.cs