Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / MIT / System / Web / UI / MobileControls / Adapters / XhtmlAdapters / XhtmlBasicCommandAdapter.cs / 1305376 / XhtmlBasicCommandAdapter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Collections.Specialized; using System.Security.Permissions; using System.Web; using System.Web.Mobile; using System.Web.UI.MobileControls; #if COMPILING_FOR_SHIPPED_SOURCE namespace System.Web.UI.MobileControls.ShippedAdapterSource.XhtmlAdapters #else namespace System.Web.UI.MobileControls.Adapters.XhtmlAdapters #endif { ///[AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] [Obsolete("The System.Web.Mobile.dll assembly has been deprecated and should no longer be used. For information about how to develop ASP.NET mobile applications, see http://go.microsoft.com/fwlink/?LinkId=157231.")] public class XhtmlCommandAdapter : XhtmlControlAdapter { /// protected new Command Control { get { return base.Control as Command; } } public override bool LoadPostData(String key, NameValueCollection data, Object controlPrivateData, out bool dataChanged) { dataChanged = false; // HTML input tags of type image postback with the coordinates // of the click rather than the name of the control. String name = Control.UniqueID; String postX = data[name + ".x"]; String postY = data[name + ".y"]; if (postX != null && postY != null && postX.Length > 0 && postY.Length > 0) { // set dataChannged to cause RaisePostDataChangedEvent() dataChanged = true; return true; } // For other command control, defer to default logic in control. return base.LoadPostData(key, data, controlPrivateData, out dataChanged); } /// public override void Render(XhtmlMobileTextWriter writer) { // Note: Since XHTML Basic and MP do not include the script element, we ignore the // Format==Link attribute as in CHTML. ConditionalClearPendingBreak(writer); string imageUrl = Control.ImageUrl; if (imageUrl != null && imageUrl.Length > 0 && Device.SupportsImageSubmit) { RenderAsInputTypeImage(writer); } else { RenderAsInputTypeSubmit(writer); } } private void RenderAsInputTypeImage(XhtmlMobileTextWriter writer) { ConditionalEnterStyle(writer, Style); writer.WriteBeginTag("input"); writer.WriteAttribute("type", "image"); writer.WriteAttribute("name", Control.UniqueID); writer.WriteAttribute("src", Control.ResolveUrl(Control.ImageUrl), true); writer.WriteAttribute("alt", Control.Text, true); ConditionalRenderClassAttribute(writer); ConditionalRenderCustomAttribute(writer, XhtmlConstants.AccessKeyCustomAttribute); writer.Write("/>"); // ConditionalSetPendingBreak should always be called *before* ConditionalExitStyle. // ConditionalExitStyle may render a block element and clear the pending break. ConditionalSetPendingBreakAfterInline(writer); ConditionalExitStyle(writer, Style); } private void RenderAsInputTypeSubmit(XhtmlMobileTextWriter writer){ ConditionalEnterStyle(writer, Style); writer.WriteBeginTag("input"); writer.WriteAttribute("type", "submit"); writer.WriteAttribute("name", Control.UniqueID); writer.WriteAttribute("value", Control.Text, true); ConditionalRenderClassAttribute(writer); ConditionalRenderCustomAttribute(writer, XhtmlConstants.AccessKeyCustomAttribute); writer.Write("/>"); // ConditionalSetPendingBreak should always be called *before* ConditionalExitStyle. // ConditionalExitStyle may render a block element and clear the pending break. ConditionalSetPendingBreakAfterInline(writer); ConditionalPopPhysicalCssClass(writer); ConditionalExitStyle(writer, Style); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Collections.Specialized; using System.Security.Permissions; using System.Web; using System.Web.Mobile; using System.Web.UI.MobileControls; #if COMPILING_FOR_SHIPPED_SOURCE namespace System.Web.UI.MobileControls.ShippedAdapterSource.XhtmlAdapters #else namespace System.Web.UI.MobileControls.Adapters.XhtmlAdapters #endif { ///[AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] [Obsolete("The System.Web.Mobile.dll assembly has been deprecated and should no longer be used. For information about how to develop ASP.NET mobile applications, see http://go.microsoft.com/fwlink/?LinkId=157231.")] public class XhtmlCommandAdapter : XhtmlControlAdapter { /// protected new Command Control { get { return base.Control as Command; } } public override bool LoadPostData(String key, NameValueCollection data, Object controlPrivateData, out bool dataChanged) { dataChanged = false; // HTML input tags of type image postback with the coordinates // of the click rather than the name of the control. String name = Control.UniqueID; String postX = data[name + ".x"]; String postY = data[name + ".y"]; if (postX != null && postY != null && postX.Length > 0 && postY.Length > 0) { // set dataChannged to cause RaisePostDataChangedEvent() dataChanged = true; return true; } // For other command control, defer to default logic in control. return base.LoadPostData(key, data, controlPrivateData, out dataChanged); } /// public override void Render(XhtmlMobileTextWriter writer) { // Note: Since XHTML Basic and MP do not include the script element, we ignore the // Format==Link attribute as in CHTML. ConditionalClearPendingBreak(writer); string imageUrl = Control.ImageUrl; if (imageUrl != null && imageUrl.Length > 0 && Device.SupportsImageSubmit) { RenderAsInputTypeImage(writer); } else { RenderAsInputTypeSubmit(writer); } } private void RenderAsInputTypeImage(XhtmlMobileTextWriter writer) { ConditionalEnterStyle(writer, Style); writer.WriteBeginTag("input"); writer.WriteAttribute("type", "image"); writer.WriteAttribute("name", Control.UniqueID); writer.WriteAttribute("src", Control.ResolveUrl(Control.ImageUrl), true); writer.WriteAttribute("alt", Control.Text, true); ConditionalRenderClassAttribute(writer); ConditionalRenderCustomAttribute(writer, XhtmlConstants.AccessKeyCustomAttribute); writer.Write("/>"); // ConditionalSetPendingBreak should always be called *before* ConditionalExitStyle. // ConditionalExitStyle may render a block element and clear the pending break. ConditionalSetPendingBreakAfterInline(writer); ConditionalExitStyle(writer, Style); } private void RenderAsInputTypeSubmit(XhtmlMobileTextWriter writer){ ConditionalEnterStyle(writer, Style); writer.WriteBeginTag("input"); writer.WriteAttribute("type", "submit"); writer.WriteAttribute("name", Control.UniqueID); writer.WriteAttribute("value", Control.Text, true); ConditionalRenderClassAttribute(writer); ConditionalRenderCustomAttribute(writer, XhtmlConstants.AccessKeyCustomAttribute); writer.Write("/>"); // ConditionalSetPendingBreak should always be called *before* ConditionalExitStyle. // ConditionalExitStyle may render a block element and clear the pending break. ConditionalSetPendingBreakAfterInline(writer); ConditionalPopPhysicalCssClass(writer); ConditionalExitStyle(writer, Style); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
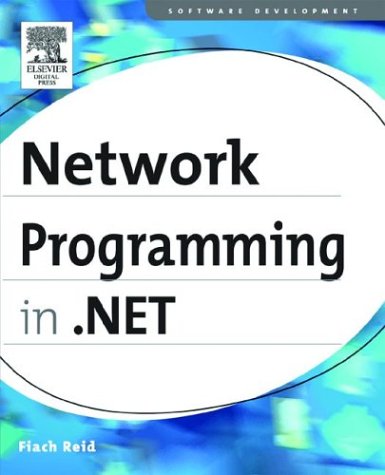
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- File.cs
- ListSortDescriptionCollection.cs
- DebugInfo.cs
- ListItemCollection.cs
- XmlSchemaRedefine.cs
- SubstitutionList.cs
- ZipIOBlockManager.cs
- ParserStreamGeometryContext.cs
- ParamArrayAttribute.cs
- DataTableTypeConverter.cs
- ContentHostHelper.cs
- Button.cs
- AnnotationAdorner.cs
- HashMembershipCondition.cs
- FileInfo.cs
- UpdateException.cs
- OrderByQueryOptionExpression.cs
- Delay.cs
- Win32MouseDevice.cs
- SolidColorBrush.cs
- WindowsFont.cs
- CryptographicAttribute.cs
- FloatUtil.cs
- DisplayInformation.cs
- OverflowException.cs
- LowerCaseStringConverter.cs
- HMACSHA256.cs
- EncryptedPackageFilter.cs
- TextElementCollection.cs
- ConfigXmlText.cs
- CodeAttachEventStatement.cs
- HasCopySemanticsAttribute.cs
- CompensationTokenData.cs
- TextEditorTyping.cs
- EventLogPermissionEntry.cs
- MetadataSource.cs
- HitTestWithGeometryDrawingContextWalker.cs
- SynchronousChannelMergeEnumerator.cs
- ProcessHostConfigUtils.cs
- ToggleButton.cs
- AppDomain.cs
- CommonDialog.cs
- SecurityTokenTypes.cs
- ConsoleEntryPoint.cs
- _ListenerRequestStream.cs
- ParseNumbers.cs
- ThrowHelper.cs
- CommandPlan.cs
- QilTargetType.cs
- SuspendDesigner.cs
- FullTrustAssembly.cs
- OleDbPermission.cs
- SortFieldComparer.cs
- FragmentNavigationEventArgs.cs
- XhtmlStyleClass.cs
- SoapReflectionImporter.cs
- IsolatedStoragePermission.cs
- Stylesheet.cs
- KoreanLunisolarCalendar.cs
- ApplicationProxyInternal.cs
- HighContrastHelper.cs
- PropertyChangedEventManager.cs
- GroupBoxAutomationPeer.cs
- GridViewItemAutomationPeer.cs
- ScrollPattern.cs
- EntityCommandExecutionException.cs
- RelationshipEntry.cs
- XmlSchemaFacet.cs
- CircleEase.cs
- TypedDataSourceCodeGenerator.cs
- FixedPosition.cs
- NativeMethods.cs
- safelink.cs
- DataSetUtil.cs
- ImageAutomationPeer.cs
- FontCacheLogic.cs
- UpdateInfo.cs
- Rect.cs
- DecimalAnimation.cs
- PathNode.cs
- UrlPath.cs
- GeneralTransform3DCollection.cs
- DescriptionAttribute.cs
- FormsAuthenticationCredentials.cs
- DayRenderEvent.cs
- FontDifferentiator.cs
- ItemCheckedEvent.cs
- DataServiceRequestOfT.cs
- BuilderPropertyEntry.cs
- FormatPage.cs
- TcpServerChannel.cs
- HMACSHA384.cs
- FaultReason.cs
- RegexCapture.cs
- WebPartTracker.cs
- StylusLogic.cs
- CheckBox.cs
- TextRangeEditLists.cs
- MarkupCompilePass2.cs
- TemplatePropertyEntry.cs