Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Description / ServiceMetadataExtension.cs / 2 / ServiceMetadataExtension.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Description { using System.Collections.Generic; using System.ServiceModel.Dispatcher; using System.Collections.ObjectModel; using System.Net; using System.ServiceModel; using System.ServiceModel.Channels; using System.Xml; using System.Xml.Schema; using System.Runtime.Serialization; using WsdlNS = System.Web.Services.Description; using System.Text; using System.Globalization; using System.IO; using System.Threading; using System.Diagnostics; using System.Reflection; using System.ServiceModel.Diagnostics; using System.ServiceModel.Activation; using System.ServiceModel.Configuration; // the description/metadata "mix-in" public class ServiceMetadataExtension : IExtension{ const string BaseAddressPattern = "{%BaseAddress%}"; static readonly Uri EmptyUri = new Uri(String.Empty, UriKind.Relative); static readonly Type[] httpGetSupportedChannels = new Type[] { typeof(IReplyChannel), }; ServiceMetadataBehavior.MetadataExtensionInitializer initializer; MetadataSet metadata; bool isInitialized = false; Uri externalMetadataLocation; ServiceHostBase owner; object syncRoot = new object(); bool mexEnabled = false; bool httpGetEnabled = false; bool httpsGetEnabled = false; bool httpHelpPageEnabled = false; bool httpsHelpPageEnabled = false; Uri mexUrl; Uri httpGetUrl; Uri httpsGetUrl; Uri httpHelpPageUrl; Uri httpsHelpPageUrl; Binding httpHelpPageBinding; Binding httpsHelpPageBinding; Binding httpGetBinding; Binding httpsGetBinding; public ServiceMetadataExtension() : this(null) { } internal ServiceMetadataExtension(ServiceMetadataBehavior.MetadataExtensionInitializer initializer) { this.initializer = initializer; } internal ServiceMetadataBehavior.MetadataExtensionInitializer Initializer { get { return this.initializer; } set { this.initializer = value; } } public MetadataSet Metadata { get { EnsureInitialized(); return this.metadata; } } internal Uri ExternalMetadataLocation { get { return this.externalMetadataLocation; } set { this.externalMetadataLocation = value; } } internal bool MexEnabled { get { return this.mexEnabled; } set { this.mexEnabled = value; } } internal bool HttpGetEnabled { get { return this.httpGetEnabled; } set { this.httpGetEnabled = value; } } internal bool HttpsGetEnabled { get { return this.httpsGetEnabled; } set { this.httpsGetEnabled = value; } } internal bool HelpPageEnabled { get { return this.httpHelpPageEnabled || this.httpsHelpPageEnabled; } } internal bool MetadataEnabled { get { return this.mexEnabled || this.httpGetEnabled || this.httpsGetEnabled; } } internal bool HttpHelpPageEnabled { get { return this.httpHelpPageEnabled; } set { this.httpHelpPageEnabled = value; } } internal bool HttpsHelpPageEnabled { get { return this.httpsHelpPageEnabled; } set { this.httpsHelpPageEnabled = value; } } internal Uri MexUrl { get { return this.mexUrl; } set { this.mexUrl = value; } } internal Uri HttpGetUrl { get { return this.httpGetUrl; } set { this.httpGetUrl = value; } } internal Uri HttpsGetUrl { get { return this.httpsGetUrl; } set { this.httpsGetUrl = value; } } internal Uri HttpHelpPageUrl { get { return this.httpHelpPageUrl; } set { this.httpHelpPageUrl = value; } } internal Uri HttpsHelpPageUrl { get { return this.httpsHelpPageUrl; } set { this.httpsHelpPageUrl = value; } } internal Binding HttpHelpPageBinding { get { return this.httpHelpPageBinding; } set { this.httpHelpPageBinding = value; } } internal Binding HttpsHelpPageBinding { get { return this.httpsHelpPageBinding; } set { this.httpsHelpPageBinding = value; } } internal Binding HttpGetBinding { get { return this.httpGetBinding; } set { this.httpGetBinding = value; } } internal Binding HttpsGetBinding { get { return this.httpsGetBinding; } set { this.httpsGetBinding = value; } } void EnsureInitialized() { if (!this.isInitialized) { lock (this.syncRoot) { if (!this.isInitialized) { if (this.initializer != null) { // the following call will initialize this // it will use the Metadata property to do the initialization // this will call back into this method, but exit because isInitialized is set. // if other threads try to call these methods, they will block on the lock this.metadata = this.initializer.GenerateMetadata(); } if (this.metadata == null) { this.metadata = new MetadataSet(); } Thread.MemoryBarrier(); this.isInitialized = true; this.initializer = null; } } } } void IExtension .Attach(ServiceHostBase owner) { if (owner == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("owner")); if (this.owner != null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.TheServiceMetadataExtensionInstanceCouldNot2_0))); owner.ThrowIfClosedOrOpened(); this.owner = owner; } void IExtension .Detach(ServiceHostBase owner) { if (owner == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("owner"); if (this.owner == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.TheServiceMetadataExtensionInstanceCouldNot3_0))); if (this.owner != owner) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument("owner", SR.GetString(SR.TheServiceMetadataExtensionInstanceCouldNot4_0)); this.owner.ThrowIfClosedOrOpened(); this.owner = null; } static internal ServiceMetadataExtension EnsureServiceMetadataExtension(ServiceDescription description, ServiceHostBase host) { ServiceMetadataExtension mex = host.Extensions.Find (); if (mex == null) { mex = new ServiceMetadataExtension(); host.Extensions.Add(mex); } return mex; } internal ChannelDispatcher EnsureGetDispatcher(Uri listenUri) { ChannelDispatcher channelDispatcher = FindGetDispatcher(listenUri); if (channelDispatcher == null) { channelDispatcher = CreateGetDispatcher(listenUri); owner.ChannelDispatchers.Add(channelDispatcher); } return channelDispatcher; } internal ChannelDispatcher EnsureGetDispatcher(Uri listenUri, bool isServiceDebugBehavior) { ChannelDispatcher channelDispatcher = FindGetDispatcher(listenUri); Binding binding; if (channelDispatcher == null) { if (listenUri.Scheme == Uri.UriSchemeHttp) { if (isServiceDebugBehavior) { binding = this.httpHelpPageBinding ?? MetadataExchangeBindings.HttpGet; } else { binding = this.httpGetBinding ?? MetadataExchangeBindings.HttpGet; } } else if (listenUri.Scheme == Uri.UriSchemeHttps) { if (isServiceDebugBehavior) { binding = this.httpsHelpPageBinding ?? MetadataExchangeBindings.HttpsGet; } else { binding = this.httpsGetBinding ?? MetadataExchangeBindings.HttpsGet; } } else { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentException(SR.GetString(SR.SFxGetChannelDispatcherDoesNotSupportScheme, typeof(ChannelDispatcher).Name, Uri.UriSchemeHttp, Uri.UriSchemeHttps))); } channelDispatcher = CreateGetDispatcher(listenUri, binding); owner.ChannelDispatchers.Add(channelDispatcher); } return channelDispatcher; } private ChannelDispatcher FindGetDispatcher(Uri listenUri) { foreach (ChannelDispatcher channelDispatcher in owner.ChannelDispatchers) { if (channelDispatcher.Listener.Uri == listenUri) { if(channelDispatcher.Endpoints.Count==1 && channelDispatcher.Endpoints[0].DispatchRuntime.SingletonInstanceContext != null && channelDispatcher.Endpoints[0].DispatchRuntime.SingletonInstanceContext.UserObject is HttpGetImpl) { return channelDispatcher; } } } return null; } private ChannelDispatcher CreateGetDispatcher(Uri listenUri) { if (listenUri.Scheme == Uri.UriSchemeHttp) { return CreateGetDispatcher(listenUri, MetadataExchangeBindings.HttpGet); } else if (listenUri.Scheme == Uri.UriSchemeHttps) { return CreateGetDispatcher(listenUri, MetadataExchangeBindings.HttpsGet); } else { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentException(SR.GetString(SR.SFxGetChannelDispatcherDoesNotSupportScheme, typeof(ChannelDispatcher).Name, Uri.UriSchemeHttp, Uri.UriSchemeHttps))); } } private ChannelDispatcher CreateGetDispatcher(Uri listenUri, Binding binding) { EndpointAddress address = new EndpointAddress(listenUri); Uri listenUriBaseAddress = listenUri; string listenUriRelativeAddress = string.Empty; BindingParameterCollection parameters = GetBindingParameters(owner.Description); // find listener for HTTP GET IChannelListener listener = null; if (binding.CanBuildChannelListener (parameters)) { listener = binding.BuildChannelListener (listenUriBaseAddress, listenUriRelativeAddress, parameters); } else { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentException(SR.GetString(SR.SFxBindingNotSupportedForMetadataHttpGet))); } //create dispatchers ChannelDispatcher channelDispatcher = new ChannelDispatcher(listener, HttpGetImpl.MetadataHttpGetBinding, binding); channelDispatcher.MessageVersion = binding.MessageVersion; EndpointDispatcher dispatcher = new EndpointDispatcher(address, HttpGetImpl.ContractName, HttpGetImpl.ContractNamespace); //Add operation DispatchOperation operationDispatcher = new DispatchOperation(dispatcher.DispatchRuntime, HttpGetImpl.GetMethodName, HttpGetImpl.RequestAction, HttpGetImpl.ReplyAction); operationDispatcher.Formatter = MessageOperationFormatter.Instance; MethodInfo methodInfo = typeof(IHttpGetMetadata).GetMethod(HttpGetImpl.GetMethodName); operationDispatcher.Invoker = new SyncMethodInvoker(methodInfo); dispatcher.DispatchRuntime.Operations.Add(operationDispatcher); //wire up dispatchers HttpGetImpl impl = new HttpGetImpl(this, listener.Uri); dispatcher.DispatchRuntime.SingletonInstanceContext = new InstanceContext(owner, impl, false); dispatcher.DispatchRuntime.MessageInspectors.Add(impl); channelDispatcher.Endpoints.Add(dispatcher); dispatcher.ContractFilter = new MatchAllMessageFilter(); dispatcher.FilterPriority = 0; dispatcher.DispatchRuntime.InstanceContextProvider = InstanceContextProviderBase.GetProviderForMode(InstanceContextMode.Single, dispatcher.DispatchRuntime); channelDispatcher.ServiceThrottle = owner.ServiceThrottle; ServiceDebugBehavior sdb = owner.Description.Behaviors.Find (); if (sdb != null) channelDispatcher.IncludeExceptionDetailInFaults |= sdb.IncludeExceptionDetailInFaults; ServiceBehaviorAttribute sba = owner.Description.Behaviors.Find (); if (sba != null) channelDispatcher.IncludeExceptionDetailInFaults |= sba.IncludeExceptionDetailInFaults; return channelDispatcher; } static BindingParameterCollection GetBindingParameters(ServiceDescription description) { BindingParameterCollection c = new BindingParameterCollection(); foreach (IServiceBehavior b in description.Behaviors) { if (b is ServiceCredentials || b is ServiceSecurityAuditBehavior) { c.Add(b); } else if (b is HostedBindingBehavior) { c.Add(((HostedBindingBehavior)b).VirtualPathExtension); c.Add(new HostedMetadataProperty()); } } return c; } internal class WSMexImpl : IMetadataExchange { internal const string MetadataMexBinding = "ServiceMetadataBehaviorMexBinding"; internal const string ContractName = MetadataStrings.WSTransfer.Name; internal const string ContractNamespace = MetadataStrings.WSTransfer.Namespace; internal const string GetMethodName = "Get"; internal const string RequestAction = MetadataStrings.WSTransfer.GetAction; internal const string ReplyAction = MetadataStrings.WSTransfer.GetResponseAction; ServiceMetadataExtension parent; MetadataSet metadataLocationSet; TypedMessageConverter converter; bool isListeningOnHttps = false; internal WSMexImpl(ServiceMetadataExtension parent) { this.parent = parent; if (this.parent.ExternalMetadataLocation != null && this.parent.ExternalMetadataLocation != EmptyUri) { this.metadataLocationSet = new MetadataSet(); string location = this.GetLocationToReturn(); MetadataSection metadataLocationSection = new MetadataSection(MetadataSection.ServiceDescriptionDialect, null, new MetadataLocation(location)); this.metadataLocationSet.MetadataSections.Add(metadataLocationSection); } } internal bool IsListeningOnHttps { get { return this.isListeningOnHttps; } set { this.isListeningOnHttps = value; } } private string GetLocationToReturn() { Debug.Assert(this.parent.ExternalMetadataLocation != null); Uri location = this.parent.ExternalMetadataLocation; if (!location.IsAbsoluteUri) { Uri httpAddr = parent.owner.GetVia(Uri.UriSchemeHttp, location); Uri httpsAddr = parent.owner.GetVia(Uri.UriSchemeHttps, location); if (this.IsListeningOnHttps && httpsAddr != null) { location = httpsAddr; } else if (httpAddr != null) { location = httpAddr; } else { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument("ExternalMetadataLocation", SR.GetString(SR.SFxBadMetadataLocationNoAppropriateBaseAddress, this.parent.ExternalMetadataLocation.OriginalString)); } } return location.ToString(); } MetadataSet GatherMetadata(string dialect, string identifier) { if (metadataLocationSet != null) { return metadataLocationSet; } else { MetadataSet metadataSet = new MetadataSet(); foreach (MetadataSection document in parent.Metadata.MetadataSections) { if ((dialect == null || dialect == document.Dialect) && (identifier == null || identifier == document.Identifier)) metadataSet.MetadataSections.Add(document); } return metadataSet; } } public Message Get(Message request) { GetResponse response = new GetResponse(); response.Metadata = GatherMetadata(null, null); response.Metadata.WriteFilter = new LocationUpdatingWriter(BaseAddressPattern, null); if(converter == null) converter = TypedMessageConverter.Create(typeof(GetResponse), ReplyAction); return converter.ToMessage(response, request.Version); } public IAsyncResult BeginGet(Message request, AsyncCallback callback, object state) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } public Message EndGet(IAsyncResult result) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } } //If this contract is changed, you may need to change ServiceMetadataExtension.CreateHttpGetDispatcher() [ServiceContract] internal interface IHttpGetMetadata { [OperationContract(Action = MessageHeaders.WildcardAction, ReplyAction = MessageHeaders.WildcardAction)] Message Get(Message msg); } internal class HttpGetImpl : IHttpGetMetadata, IDispatchMessageInspector { const string DiscoToken = "disco token"; const string DiscoQueryString = "disco"; const string WsdlQueryString = "wsdl"; const string XsdQueryString = "xsd"; const string HtmlContentType = "text/html; charset=UTF-8"; const string XmlContentType = "text/xml; charset=UTF-8"; const int closeTimeoutInSeconds = 90; const int maxQueryStringChars = 2048; internal const string MetadataHttpGetBinding = "ServiceMetadataBehaviorHttpGetBinding"; internal const string ContractName = "IHttpGetHelpPageAndMetadataContract"; internal const string ContractNamespace = "http://schemas.microsoft.com/2006/04/http/metadata"; internal const string GetMethodName = "Get"; internal const string RequestAction = MessageHeaders.WildcardAction; internal const string ReplyAction = MessageHeaders.WildcardAction; internal const string HtmlBreak = "
"; static string[] NoQueries = new string[0]; ServiceMetadataExtension parent; object sync = new object(); InitializationData initData; Uri listenUri; bool helpPageEnabled = false; bool getWsdlEnabled = false; internal HttpGetImpl(ServiceMetadataExtension parent, Uri listenUri) { this.parent = parent; this.listenUri = listenUri; } public bool HelpPageEnabled { get { return this.helpPageEnabled; } set { this.helpPageEnabled = value; } } public bool GetWsdlEnabled { get { return this.getWsdlEnabled; } set { this.getWsdlEnabled = value; } } InitializationData GetInitData() { if (this.initData == null) { lock (this.sync) { if (this.initData == null) { this.initData = InitializationData.InitializeFrom(parent); } } } return this.initData; } string FindWsdlReference() { if (this.parent.ExternalMetadataLocation == null || this.parent.ExternalMetadataLocation == EmptyUri) { return null; } else { Uri location = this.parent.ExternalMetadataLocation; return ServiceHost.GetUri(this.listenUri, location).ToString(); } } bool TryHandleDocumentationRequest(Message httpGetRequest, string[] queries, out Message replyMessage) { replyMessage = null; if (!this.HelpPageEnabled) return false; if (parent.MetadataEnabled) { string discoUrl = null; string wsdlUrl = null; string httpGetUrl = null; bool linkMetadata = true; wsdlUrl = FindWsdlReference(); httpGetUrl = GetHttpGetUrl(); if (wsdlUrl == null && httpGetUrl != null) wsdlUrl = httpGetUrl + "?" + WsdlQueryString; if (httpGetUrl != null) discoUrl = httpGetUrl + "?" + DiscoQueryString; if (wsdlUrl == null) { wsdlUrl = GetMexUrl(); linkMetadata = false; } replyMessage = new MetadataOnHelpPageMessage(discoUrl, wsdlUrl, GetInitData().ServiceName, GetInitData().ClientName, linkMetadata); } else { replyMessage = new MetadataOffHelpPageMessage(GetInitData().ServiceName); } AddHttpProperty(replyMessage, HttpStatusCode.OK, HtmlContentType); return true; } private string GetHttpGetUrl() { if (this.listenUri.Scheme == Uri.UriSchemeHttp) { if (parent.HttpGetEnabled) return parent.HttpGetUrl.ToString(); else if (parent.HttpsGetEnabled) return parent.HttpsGetUrl.ToString(); } else { if (parent.HttpsGetEnabled) return parent.HttpsGetUrl.ToString(); else if (parent.HttpGetEnabled) return parent.HttpGetUrl.ToString(); } return null; } private string GetMexUrl() { if (parent.MexEnabled) return parent.MexUrl.ToString(); return null; } bool TryHandleMetadataRequest(Message httpGetRequest, string[] queries, out Message replyMessage) { replyMessage = null; if (!this.GetWsdlEnabled) return false; string query = FindQuery(queries); if (String.IsNullOrEmpty(query)) { //if the documentation page is not available return the default wsdl if it exists if (!this.helpPageEnabled && GetInitData().DefaultWsdl != null) { // use the default WSDL using (httpGetRequest) { replyMessage = new ServiceDescriptionMessage( GetInitData().DefaultWsdl, this.listenUri.ToString()); AddHttpProperty(replyMessage, HttpStatusCode.OK, XmlContentType); GetInitData().FixImportAddresses(); return true; } } return false; } // try to look the document up in the query table object doc; if (GetInitData().TryQueryLookup(query, out doc)) { using (httpGetRequest) { if (doc is WsdlNS.ServiceDescription) { replyMessage = new ServiceDescriptionMessage( (WsdlNS.ServiceDescription)doc, this.listenUri.ToString()); } else if (doc is XmlSchema) { replyMessage = new XmlSchemaMessage( ((XmlSchema)doc), this.listenUri.ToString()); } else if (doc is string) { if (((string)doc) == DiscoToken) { replyMessage = CreateDiscoMessage(); } else { DiagnosticUtility.DebugAssert("Bad object in HttpGetImpl docFromQuery table"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(String.Format(CultureInfo.InvariantCulture, "Bad object in HttpGetImpl docFromQuery table"))); } } else { DiagnosticUtility.DebugAssert("Bad object in HttpGetImpl docFromQuery table"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(String.Format(CultureInfo.InvariantCulture, "Bad object in HttpGetImpl docFromQuery table"))); } AddHttpProperty(replyMessage, HttpStatusCode.OK, XmlContentType); GetInitData().FixImportAddresses(); return true; } } // otherwise see if they just wanted ?WSDL if (String.Compare(query, WsdlQueryString, StringComparison.OrdinalIgnoreCase) == 0) { if (GetInitData().DefaultWsdl != null) { // use the default WSDL using (httpGetRequest) { replyMessage = new ServiceDescriptionMessage( GetInitData().DefaultWsdl, this.listenUri.ToString()); AddHttpProperty(replyMessage, HttpStatusCode.OK, XmlContentType); GetInitData().FixImportAddresses(); return true; } } // or redirect to an external WSDL string wsdlReference = FindWsdlReference(); if (wsdlReference != null) { replyMessage = CreateRedirectMessage(wsdlReference); return true; } } // we weren't able to handle the request -- return the documentation page if available return false; } Message CreateDiscoMessage() { string wsdlUrl = this.listenUri.ToString() + "?" + WsdlQueryString; string docUrl = null; if (this.listenUri.Scheme == Uri.UriSchemeHttp) { if (parent.HttpHelpPageEnabled) docUrl = parent.HttpHelpPageUrl.ToString(); else if (parent.HttpsHelpPageEnabled) docUrl = parent.HttpsGetUrl.ToString(); } else { if (parent.HttpsHelpPageEnabled) docUrl = parent.HttpsHelpPageUrl.ToString(); else if (parent.HttpHelpPageEnabled) docUrl = parent.HttpGetUrl.ToString(); } return new DiscoMessage(wsdlUrl, docUrl); } private string FindQuery(string[] queries) { string query = null; foreach (string q in queries) { int start = (q.Length > 0 && q[0] == '?') ? 1 : 0; if (String.Compare(q, start, WsdlQueryString, 0, WsdlQueryString.Length, StringComparison.OrdinalIgnoreCase) == 0) query = q; else if (String.Compare(q, start, XsdQueryString, 0, XsdQueryString.Length, StringComparison.OrdinalIgnoreCase) == 0) query = q; else if (parent.HelpPageEnabled && (String.Compare(q, start, DiscoQueryString, 0, DiscoQueryString.Length, StringComparison.OrdinalIgnoreCase) == 0)) query = q; } return query; } Message ProcessHttpRequest(Message httpGetRequest) { string queryString = httpGetRequest.Properties.Via.Query; if (queryString.Length > maxQueryStringChars) return CreateHttpResponseMessage(HttpStatusCode.RequestUriTooLong); if (queryString.StartsWith("?", StringComparison.OrdinalIgnoreCase)) queryString = queryString.Substring(1); string[] queries = queryString.Length > 0 ? queryString.Split('&') : NoQueries; Message replyMessage = null; if (TryHandleMetadataRequest(httpGetRequest, queries, out replyMessage)) return replyMessage; if (TryHandleDocumentationRequest(httpGetRequest, queries, out replyMessage)) return replyMessage; return CreateHttpResponseMessage(HttpStatusCode.MethodNotAllowed); } public object AfterReceiveRequest(ref Message request, IClientChannel channel, InstanceContext instanceContext) { return request.Version; } public void BeforeSendReply(ref Message reply, object correlationState) { if ((reply != null) && reply.IsFault) { string error = SR.GetString(SR.SFxInternalServerError); ExceptionDetail exceptionDetail = null; MessageFault fault = MessageFault.CreateFault(reply, /* maxBufferSize */ 64 * 1024); if (fault.HasDetail) { exceptionDetail = fault.GetDetail(); if (exceptionDetail != null) { error = SR.GetString(SR.SFxDocExt_Error); } } reply = new MetadataOnHelpPageMessage(error, exceptionDetail); AddHttpProperty(reply, HttpStatusCode.InternalServerError, HtmlContentType); } } public Message Get(Message message) { return ProcessHttpRequest(message); } class InitializationData { readonly Dictionary docFromQuery; readonly Dictionary
Link Menu
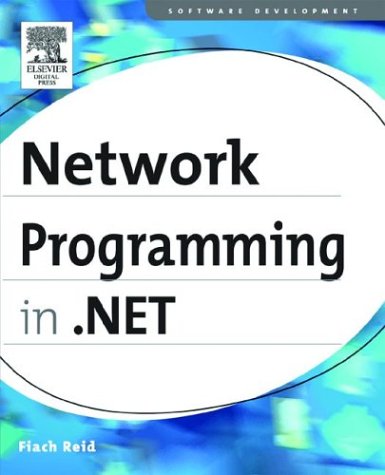
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebPartDescriptionCollection.cs
- AuthorizationSection.cs
- DecoderFallbackWithFailureFlag.cs
- QuotedPrintableStream.cs
- basenumberconverter.cs
- activationcontext.cs
- _ConnectStream.cs
- PartitionResolver.cs
- CodeAssignStatement.cs
- XslVisitor.cs
- AssemblyCache.cs
- SystemNetHelpers.cs
- AttributeInfo.cs
- Point4D.cs
- XmlSchemaInferenceException.cs
- StateDesigner.Helpers.cs
- BookmarkEventArgs.cs
- ManagedWndProcTracker.cs
- JsonGlobals.cs
- PeerMaintainer.cs
- XmlSchemaSimpleType.cs
- SmiRequestExecutor.cs
- ContentOperations.cs
- DataGridViewCellStyleChangedEventArgs.cs
- TimeoutValidationAttribute.cs
- SplitterEvent.cs
- TemplateBamlRecordReader.cs
- ExpanderAutomationPeer.cs
- PerspectiveCamera.cs
- SqlDependencyListener.cs
- PriorityQueue.cs
- WindowsImpersonationContext.cs
- HtmlTextArea.cs
- CharAnimationUsingKeyFrames.cs
- UInt16.cs
- EventLogTraceListener.cs
- SocketPermission.cs
- ApplicationId.cs
- PolicyUnit.cs
- WmlImageAdapter.cs
- DataException.cs
- ReservationNotFoundException.cs
- OletxTransactionFormatter.cs
- RowToFieldTransformer.cs
- PanelStyle.cs
- SelectingProviderEventArgs.cs
- MenuItemBindingCollection.cs
- SimpleWorkerRequest.cs
- CompoundFileReference.cs
- OutputScopeManager.cs
- StreamResourceInfo.cs
- StorageSetMapping.cs
- PathSegmentCollection.cs
- GridViewCancelEditEventArgs.cs
- AuthorizationRuleCollection.cs
- ConnectionStringSettings.cs
- OutputCacheSettings.cs
- EnumValAlphaComparer.cs
- QEncodedStream.cs
- IndicCharClassifier.cs
- RecipientIdentity.cs
- EnumBuilder.cs
- BindUriHelper.cs
- SafeViewOfFileHandle.cs
- SingleStorage.cs
- ActiveDocumentEvent.cs
- WebDisplayNameAttribute.cs
- Propagator.JoinPropagator.cs
- RenderOptions.cs
- GridViewColumnCollectionChangedEventArgs.cs
- AsyncSerializedWorker.cs
- DataKeyArray.cs
- HiddenFieldPageStatePersister.cs
- SymbolMethod.cs
- EnumValAlphaComparer.cs
- IsolatedStorage.cs
- RegistryKey.cs
- Border.cs
- SynthesizerStateChangedEventArgs.cs
- EllipseGeometry.cs
- AdornerPresentationContext.cs
- LogLogRecordHeader.cs
- PictureBox.cs
- DispatcherEventArgs.cs
- UdpChannelListener.cs
- ZipFileInfo.cs
- SecurityRuntime.cs
- XPathItem.cs
- HttpResponse.cs
- MultiDataTrigger.cs
- FloatUtil.cs
- WebPartsPersonalization.cs
- SafeRightsManagementPubHandle.cs
- HttpServerUtilityWrapper.cs
- ParameterReplacerVisitor.cs
- CurrentChangedEventManager.cs
- ProxyManager.cs
- DataGridCellEditEndingEventArgs.cs
- ParenthesizePropertyNameAttribute.cs
- SafeRegistryKey.cs