Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / DataServiceQueryOfT.cs / 1305376 / DataServiceQueryOfT.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// query object // //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; using System.Collections.Generic; using System.Diagnostics; using System.IO; using System.Linq; using System.Linq.Expressions; #if !ASTORIA_LIGHT // Data.Services http stack using System.Net; #else using System.Data.Services.Http; #endif using System.Reflection; using System.Collections; ////// query object /// ///type of object to materialize [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1710:IdentifiersShouldHaveCorrectSuffix", Justification = "required for this feature")] public class DataServiceQuery: DataServiceQuery, IQueryable { #region Private fields. /// Linq Expression private readonly Expression queryExpression; ///Linq Query Provider private readonly DataServiceQueryProvider queryProvider; ///Uri, Projection, Version for translated query private QueryComponents queryComponents; #endregion Private fields. ////// query object /// /// expression for query /// query provider for query private DataServiceQuery(Expression expression, DataServiceQueryProvider provider) { Debug.Assert(null != provider.Context, "null context"); Debug.Assert(expression != null, "null expression"); Debug.Assert(provider is DataServiceQueryProvider, "Currently only support Web Query Provider"); this.queryExpression = expression; this.queryProvider = provider; } #region IQueryable implementation ////// Element Type /// public override Type ElementType { get { return typeof(TElement); } } ////// Linq Expression /// public override Expression Expression { get { return this.queryExpression; } } ////// Linq Query Provider /// public override IQueryProvider Provider { get { return this.queryProvider; } } #endregion ////// gets the URI for a the query /// public override Uri RequestUri { get { return this.Translate().Uri; } } ///The ProjectionPlan for the request (if precompiled in a previous page). internal override ProjectionPlan Plan { get { return null; } } ////// gets the UriTranslateResult for a the query /// internal override QueryComponents QueryComponents { get { return this.Translate(); } } ////// Begins an asynchronous request to an Internet resource. /// /// The AsyncCallback delegate. /// The state object for this request. ///An IAsyncResult that references the asynchronous request for a response. public new IAsyncResult BeginExecute(AsyncCallback callback, object state) { return base.BeginExecute(this, this.queryProvider.Context, callback, state); } ////// Ends an asynchronous request to an Internet resource. /// /// The pending request for a response. ///An IEnumerable that contains the response from the Internet resource. public new IEnumerableEndExecute(IAsyncResult asyncResult) { return DataServiceRequest.EndExecute (this, this.queryProvider.Context, asyncResult); } #if !ASTORIA_LIGHT // Synchronous methods not available /// /// Returns an IEnumerable from an Internet resource. /// ///An IEnumerable that contains the response from the Internet resource. public new IEnumerableExecute() { return this.Execute (this.queryProvider.Context, this.Translate()); } #endif /// /// Sets Expand option /// /// Path to expand ///New DataServiceQuery with expand option public DataServiceQueryExpand(string path) { Util.CheckArgumentNull(path, "path"); Util.CheckArgumentNotEmpty(path, "path"); MethodInfo mi = typeof(DataServiceQuery ).GetMethod("Expand"); return (DataServiceQuery )this.Provider.CreateQuery ( Expression.Call( Expression.Convert(this.Expression, typeof(DataServiceQuery .DataServiceOrderedQuery)), mi, new Expression[] { Expression.Constant(path) })); } /// /// Turn the inline counting option on for the query /// ///New DataServiceQuery with count option public DataServiceQueryIncludeTotalCount() { MethodInfo mi = typeof(DataServiceQuery ).GetMethod("IncludeTotalCount"); return (DataServiceQuery )this.Provider.CreateQuery ( Expression.Call( Expression.Convert(this.Expression, typeof(DataServiceQuery .DataServiceOrderedQuery)), mi)); } /// /// Sets user option /// /// name of value /// value of option ///New DataServiceQuery with expand option public DataServiceQueryAddQueryOption(string name, object value) { Util.CheckArgumentNull(name, "name"); Util.CheckArgumentNull(value, "value"); MethodInfo mi = typeof(DataServiceQuery ).GetMethod("AddQueryOption"); return (DataServiceQuery )this.Provider.CreateQuery ( Expression.Call( Expression.Convert(this.Expression, typeof(DataServiceQuery .DataServiceOrderedQuery)), mi, new Expression[] { Expression.Constant(name), Expression.Constant(value, typeof(object)) })); } /// /// get an enumerator materializes the objects the Uri request /// ///an enumerator #if !ASTORIA_LIGHT // Synchronous methods not available public IEnumeratorGetEnumerator() { return this.Execute().GetEnumerator(); } #else IEnumerator IEnumerable .GetEnumerator() { throw Error.NotSupported(Strings.DataServiceQuery_EnumerationNotSupportedInSL); } #endif /// /// gets the URI for the query /// ///a string with the URI public override string ToString() { try { return base.ToString(); } catch (NotSupportedException e) { return Strings.ALinq_TranslationError(e.Message); } } ////// get an enumerator materializes the objects the Uri request /// ///an enumerator System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator() { #if !ASTORIA_LIGHT // Synchronous methods not available return this.GetEnumerator(); #else throw Error.NotSupported(); #endif } #if !ASTORIA_LIGHT /// Synchronous methods not available ////// Returns an IEnumerable from an Internet resource. /// ///An IEnumerable that contains the response from the Internet resource. internal override IEnumerable ExecuteInternal() { return this.Execute(); } #endif ////// Begins an asynchronous request to an Internet resource. /// /// The AsyncCallback delegate. /// The state object for this request. ///An IAsyncResult that references the asynchronous request for a response. internal override IAsyncResult BeginExecuteInternal(AsyncCallback callback, object state) { return this.BeginExecute(callback, state); } ////// Ends an asynchronous request to an Internet resource. /// /// The pending request for a response. ///An IEnumerable that contains the response from the Internet resource. internal override IEnumerable EndExecuteInternal(IAsyncResult asyncResult) { return this.EndExecute(asyncResult); } ////// gets the query components for the query after translating /// ///QueryComponents for query private QueryComponents Translate() { if (this.queryComponents == null) { this.queryComponents = this.queryProvider.Translate(this.queryExpression); } return this.queryComponents; } ////// Ordered DataServiceQuery which implements IOrderedQueryable. /// internal class DataServiceOrderedQuery : DataServiceQuery, IOrderedQueryable , IOrderedQueryable { /// /// constructor /// /// expression for query /// query provider for query internal DataServiceOrderedQuery(Expression expression, DataServiceQueryProvider provider) : base(expression, provider) { } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
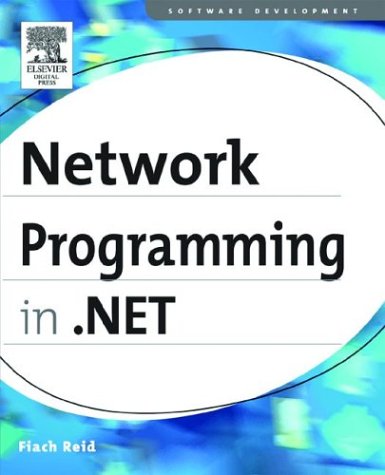
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GridViewAutomationPeer.cs
- DescendentsWalker.cs
- SettingsAttributes.cs
- MergeLocalizationDirectives.cs
- LinqDataSourceHelper.cs
- ASCIIEncoding.cs
- CookieHandler.cs
- XmlnsPrefixAttribute.cs
- RadioButton.cs
- ZoneIdentityPermission.cs
- RSACryptoServiceProvider.cs
- DataColumnPropertyDescriptor.cs
- BufferedGraphicsManager.cs
- HealthMonitoringSection.cs
- TargetInvocationException.cs
- LoadRetryConstantStrategy.cs
- ZipFileInfo.cs
- SimpleType.cs
- FrameworkElementFactory.cs
- ConfigXmlText.cs
- ProcessHostMapPath.cs
- SmtpDigestAuthenticationModule.cs
- JsonByteArrayDataContract.cs
- CommonObjectSecurity.cs
- NamespaceTable.cs
- SecurityHelper.cs
- StorageComplexPropertyMapping.cs
- DropSource.cs
- UserPreferenceChangedEventArgs.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- Font.cs
- CqlParserHelpers.cs
- QilScopedVisitor.cs
- ProtocolsSection.cs
- listitem.cs
- HuffmanTree.cs
- Solver.cs
- HotSpotCollection.cs
- RangeValidator.cs
- ItemCollection.cs
- MgmtConfigurationRecord.cs
- SpellerError.cs
- TreeNodeSelectionProcessor.cs
- FormsAuthenticationUser.cs
- ListChunk.cs
- UnaryNode.cs
- SecureUICommand.cs
- Site.cs
- QueryOperationResponseOfT.cs
- DesignBinding.cs
- ListControl.cs
- Stream.cs
- InheritanceRules.cs
- TreeView.cs
- ClientSession.cs
- QueryOpeningEnumerator.cs
- HttpHeaderCollection.cs
- StateMachineWorkflowInstance.cs
- DataAdapter.cs
- Switch.cs
- ThreadExceptionDialog.cs
- ThicknessAnimation.cs
- FileUtil.cs
- ParserStreamGeometryContext.cs
- IndentedTextWriter.cs
- DynamicPropertyHolder.cs
- _BaseOverlappedAsyncResult.cs
- TimeSpanValidator.cs
- InvalidPropValue.cs
- DatagridviewDisplayedBandsData.cs
- HuffmanTree.cs
- ColorTransformHelper.cs
- TypeElement.cs
- ScrollBarAutomationPeer.cs
- RightsManagementUser.cs
- Grid.cs
- TextContainerChangeEventArgs.cs
- GatewayIPAddressInformationCollection.cs
- COM2ColorConverter.cs
- TypeUnloadedException.cs
- SecuritySessionClientSettings.cs
- FormViewCommandEventArgs.cs
- DataTableTypeConverter.cs
- ExtendedPropertyCollection.cs
- SmiRequestExecutor.cs
- TextEditorMouse.cs
- Utilities.cs
- DocComment.cs
- EpmSourceTree.cs
- ProgressPage.cs
- PartBasedPackageProperties.cs
- HttpRuntimeSection.cs
- EraserBehavior.cs
- XmlAnyElementAttribute.cs
- AspProxy.cs
- FormsAuthenticationEventArgs.cs
- SaveFileDialog.cs
- AbstractSvcMapFileLoader.cs
- ProcessHostMapPath.cs
- Tuple.cs