Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / Configuration / FormsAuthenticationUser.cs / 1305376 / FormsAuthenticationUser.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /***************************************************************************** From machine.config******************************************************************************/ namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.Globalization; using System.IO; using System.Text; using System.Web.Util; using System.ComponentModel; using System.Security.Permissions; public sealed class FormsAuthenticationUser : ConfigurationElement { private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propName = new ConfigurationProperty("name", typeof(string), "", new LowerCaseStringConverter(), null, ConfigurationPropertyOptions.IsRequired | ConfigurationPropertyOptions.IsKey); private static readonly ConfigurationProperty _propPassword = new ConfigurationProperty("password", typeof(string), "", ConfigurationPropertyOptions.IsRequired); static FormsAuthenticationUser() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propName); _properties.Add(_propPassword); } internal FormsAuthenticationUser() { } public FormsAuthenticationUser(String name, String password) : this() { Name = name.ToLower(CultureInfo.InvariantCulture); Password = password; } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("name", IsRequired = true, IsKey = true, DefaultValue = "")] [TypeConverter(typeof(LowerCaseStringConverter))] [StringValidator()] public string Name { get { return (string)base[_propName]; } set { base[_propName] = value; } } [ConfigurationProperty("password", IsRequired = true, DefaultValue = "")] [StringValidator()] public string Password { get { return (string)base[_propPassword]; } set { base[_propPassword] = value; } } } // class FormsAuthenticationUser } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
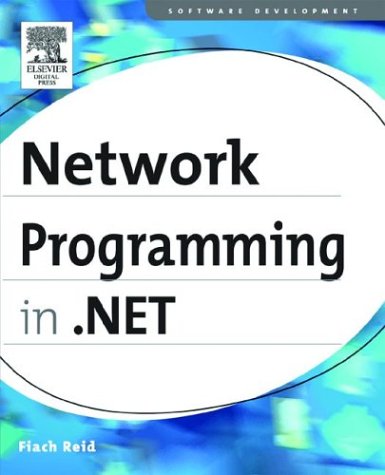
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UIElementAutomationPeer.cs
- GraphicsContainer.cs
- StylusPointPropertyId.cs
- SqlInfoMessageEvent.cs
- DateTimeConstantAttribute.cs
- Canvas.cs
- ResourceLoader.cs
- MachineKeyValidationConverter.cs
- EntityDataSourceSelectedEventArgs.cs
- TrackBarDesigner.cs
- FlowLayoutPanel.cs
- FixedPageAutomationPeer.cs
- DebugController.cs
- CapabilitiesRule.cs
- ScrollItemProviderWrapper.cs
- HttpCapabilitiesSectionHandler.cs
- HtmlForm.cs
- SqlBooleanMismatchVisitor.cs
- AggregationMinMaxHelpers.cs
- TransformPattern.cs
- EntityStoreSchemaFilterEntry.cs
- AstTree.cs
- DBPropSet.cs
- HttpRequestCacheValidator.cs
- InkPresenter.cs
- AsyncDataRequest.cs
- SimpleWebHandlerParser.cs
- SpecialNameAttribute.cs
- Choices.cs
- AssociationTypeEmitter.cs
- Point4D.cs
- ClientSideQueueItem.cs
- ComponentResourceKey.cs
- OperationExecutionFault.cs
- FileChangesMonitor.cs
- ActivationService.cs
- InstanceKey.cs
- ImpersonateTokenRef.cs
- JsonByteArrayDataContract.cs
- MasterPage.cs
- List.cs
- OdbcConnectionHandle.cs
- VectorAnimation.cs
- XmlLanguageConverter.cs
- OdbcEnvironment.cs
- ScrollItemPatternIdentifiers.cs
- ProgressBarAutomationPeer.cs
- HttpProfileBase.cs
- Grid.cs
- EntityContainerEntitySet.cs
- SortDescription.cs
- HttpServerProtocol.cs
- WebPartDescription.cs
- BitmapEffectCollection.cs
- ApplicationHost.cs
- ZipIOExtraField.cs
- VirtualPathProvider.cs
- TabItemAutomationPeer.cs
- OdbcHandle.cs
- DeclarativeCatalogPart.cs
- SqlTransaction.cs
- Rect3D.cs
- ObjectHandle.cs
- VBIdentifierName.cs
- DomainUpDown.cs
- IsolatedStorageFilePermission.cs
- DataGridViewEditingControlShowingEventArgs.cs
- WebPartManager.cs
- SqlTriggerAttribute.cs
- XmlIncludeAttribute.cs
- XmlDataContract.cs
- SettingsAttributeDictionary.cs
- SqlAggregateChecker.cs
- UdpTransportSettings.cs
- SessionStateModule.cs
- WeakReference.cs
- EventDrivenDesigner.cs
- SortedList.cs
- PropertyConverter.cs
- PerspectiveCamera.cs
- PagerSettings.cs
- DataGridViewSelectedRowCollection.cs
- TimelineGroup.cs
- AnnotationHighlightLayer.cs
- ResourcePart.cs
- JournalEntryStack.cs
- MSAANativeProvider.cs
- TimeSpanSecondsOrInfiniteConverter.cs
- ISCIIEncoding.cs
- InstanceLockLostException.cs
- FontInfo.cs
- ViewBase.cs
- HealthMonitoringSectionHelper.cs
- ComboBoxAutomationPeer.cs
- DetailsViewUpdatedEventArgs.cs
- InkPresenter.cs
- ParentQuery.cs
- CharEnumerator.cs
- SqlError.cs
- ActivityInstanceMap.cs