Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / NetFx35 / System.ServiceModel.Web / System / ServiceModel / WebHttpBinding.cs / 1 / WebHttpBinding.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel { using System; using System.Configuration; using System.ServiceModel.Channels; using System.ServiceModel.Configuration; using System.ServiceModel.Description; using System.Text; using System.Xml; public class WebHttpBinding : Binding, IBindingRuntimePreferences { HttpsTransportBindingElement httpsTransportBindingElement; // private BindingElements HttpTransportBindingElement httpTransportBindingElement; WebHttpSecurity security = new WebHttpSecurity(); WebMessageEncodingBindingElement webMessageEncodingBindingElement; public WebHttpBinding() : this(WebHttpSecurityMode.None) { } public WebHttpBinding(string configurationName) : this() { ApplyConfiguration(configurationName); } public WebHttpBinding(WebHttpSecurityMode securityMode) : base() { Initialize(); this.security.Mode = securityMode; } public bool AllowCookies { get { return httpTransportBindingElement.AllowCookies; } set { httpTransportBindingElement.AllowCookies = value; httpsTransportBindingElement.AllowCookies = value; } } public bool BypassProxyOnLocal { get { return httpTransportBindingElement.BypassProxyOnLocal; } set { httpTransportBindingElement.BypassProxyOnLocal = value; httpsTransportBindingElement.BypassProxyOnLocal = value; } } public EnvelopeVersion EnvelopeVersion { get { return EnvelopeVersion.None; } } public HostNameComparisonMode HostNameComparisonMode { get { return httpTransportBindingElement.HostNameComparisonMode; } set { httpTransportBindingElement.HostNameComparisonMode = value; httpsTransportBindingElement.HostNameComparisonMode = value; } } public long MaxBufferPoolSize { get { return httpTransportBindingElement.MaxBufferPoolSize; } set { httpTransportBindingElement.MaxBufferPoolSize = value; httpsTransportBindingElement.MaxBufferPoolSize = value; } } public int MaxBufferSize { get { return httpTransportBindingElement.MaxBufferSize; } set { httpTransportBindingElement.MaxBufferSize = value; httpsTransportBindingElement.MaxBufferSize = value; } } public long MaxReceivedMessageSize { get { return httpTransportBindingElement.MaxReceivedMessageSize; } set { httpTransportBindingElement.MaxReceivedMessageSize = value; httpsTransportBindingElement.MaxReceivedMessageSize = value; } } public Uri ProxyAddress { get { return httpTransportBindingElement.ProxyAddress; } set { httpTransportBindingElement.ProxyAddress = value; httpsTransportBindingElement.ProxyAddress = value; } } public XmlDictionaryReaderQuotas ReaderQuotas { get { return webMessageEncodingBindingElement.ReaderQuotas; } set { if (value == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("value"); } value.CopyTo(webMessageEncodingBindingElement.ReaderQuotas); } } public override string Scheme { get { return GetTransport().Scheme; } } public WebHttpSecurity Security { get { return this.security; } } public TransferMode TransferMode { get { return httpTransportBindingElement.TransferMode; } set { httpTransportBindingElement.TransferMode = value; httpsTransportBindingElement.TransferMode = value; } } public bool UseDefaultWebProxy { get { return httpTransportBindingElement.UseDefaultWebProxy; } set { httpTransportBindingElement.UseDefaultWebProxy = value; httpsTransportBindingElement.UseDefaultWebProxy = value; } } public Encoding WriteEncoding { get { return webMessageEncodingBindingElement.WriteEncoding; } set { webMessageEncodingBindingElement.WriteEncoding = value; } } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1033:InterfaceMethodsShouldBeCallableByChildTypes")] // [....], This is the pattern we use on the standard bindings in Indigo V1 bool IBindingRuntimePreferences.ReceiveSynchronously { get { return false; } } public override BindingElementCollection CreateBindingElements() { // return collection of BindingElements BindingElementCollection bindingElements = new BindingElementCollection(); // order of BindingElements is important // add encoding bindingElements.Add(webMessageEncodingBindingElement); // add transport (http or https) bindingElements.Add(GetTransport()); return bindingElements.Clone(); } void ApplyConfiguration(string configurationName) { WebHttpBindingCollectionElement section = WebHttpBindingCollectionElement.GetBindingCollectionElement(); WebHttpBindingElement element = section.Bindings[configurationName]; if (element == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ConfigurationErrorsException( SR2.GetString(SR2.ConfigInvalidBindingConfigurationName, configurationName, WebHttpBindingConfigurationStrings.WebHttpBindingCollectionElementName))); } else { element.ApplyConfiguration(this); } } TransportBindingElement GetTransport() { if (security.Mode == WebHttpSecurityMode.Transport) { security.EnableTransportSecurity(httpsTransportBindingElement); return httpsTransportBindingElement; } else if (security.Mode == WebHttpSecurityMode.TransportCredentialOnly) { security.EnableTransportAuthentication(httpTransportBindingElement); return httpTransportBindingElement; } else { // ensure that there is no transport security security.DisableTransportAuthentication(httpTransportBindingElement); return httpTransportBindingElement; } } void Initialize() { httpTransportBindingElement = new HttpTransportBindingElement(); httpsTransportBindingElement = new HttpsTransportBindingElement(); httpTransportBindingElement.ManualAddressing = true; httpsTransportBindingElement.ManualAddressing = true; webMessageEncodingBindingElement = new WebMessageEncodingBindingElement(); webMessageEncodingBindingElement.MessageVersion = MessageVersion.None; } internal static class WebHttpBindingConfigurationStrings { internal const string WebHttpBindingCollectionElementName = "webHttpBinding"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
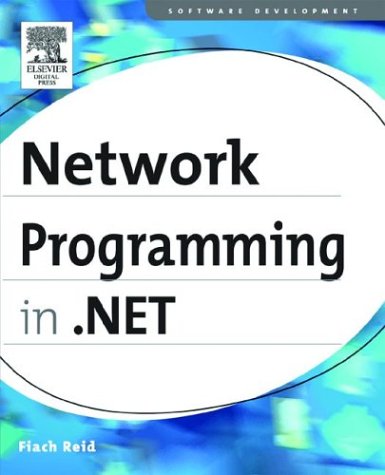
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlEventCache.cs
- DateTimeUtil.cs
- SqlNotificationRequest.cs
- ErrorTableItemStyle.cs
- MutexSecurity.cs
- CaseInsensitiveHashCodeProvider.cs
- SerializationSectionGroup.cs
- shaperfactory.cs
- MailWebEventProvider.cs
- ServiceObjectContainer.cs
- RenderingBiasValidation.cs
- Rect3DValueSerializer.cs
- WebPartConnectVerb.cs
- BaseHashHelper.cs
- StorageModelBuildProvider.cs
- CharStorage.cs
- ObjectTypeMapping.cs
- WebColorConverter.cs
- QuaternionConverter.cs
- TypeToTreeConverter.cs
- WebColorConverter.cs
- DateTimeFormatInfo.cs
- NativeWindow.cs
- LayoutTable.cs
- RijndaelCryptoServiceProvider.cs
- ThreadAttributes.cs
- BulletChrome.cs
- Matrix3D.cs
- RemotingConfigParser.cs
- FileController.cs
- BaseParagraph.cs
- TransportChannelFactory.cs
- ControlIdConverter.cs
- DataGridViewCellValidatingEventArgs.cs
- CommandEventArgs.cs
- DeflateStream.cs
- CopyOfAction.cs
- WebResourceAttribute.cs
- DataSourceHelper.cs
- OleDbCommandBuilder.cs
- TimeEnumHelper.cs
- InspectionWorker.cs
- ThreadSafeList.cs
- AttributeEmitter.cs
- AdornerDecorator.cs
- MyContact.cs
- BitConverter.cs
- StatusBar.cs
- BitmapEffect.cs
- QueryParameter.cs
- OpenTypeLayout.cs
- FormsAuthenticationConfiguration.cs
- Literal.cs
- SchemaTypeEmitter.cs
- RelatedCurrencyManager.cs
- XMLSyntaxException.cs
- InputLanguageEventArgs.cs
- DataTableTypeConverter.cs
- DBConnection.cs
- TableHeaderCell.cs
- ProxyHelper.cs
- FlowLayout.cs
- SafePointer.cs
- TargetException.cs
- CachedCompositeFamily.cs
- TranslateTransform3D.cs
- XmlSchemaAttributeGroup.cs
- HostProtectionException.cs
- ImageCodecInfo.cs
- InstanceLockTracking.cs
- NotifyIcon.cs
- SortedDictionary.cs
- FontUnitConverter.cs
- HtmlTernaryTree.cs
- NoClickablePointException.cs
- PopOutPanel.cs
- PropertyMappingExceptionEventArgs.cs
- SerializationObjectManager.cs
- ControlBuilderAttribute.cs
- UIntPtr.cs
- AuthenticatingEventArgs.cs
- SBCSCodePageEncoding.cs
- DetailsViewUpdateEventArgs.cs
- HostedElements.cs
- BookmarkUndoUnit.cs
- SqlDataSourceSelectingEventArgs.cs
- ComboBox.cs
- HwndSourceParameters.cs
- CheckBox.cs
- ProviderIncompatibleException.cs
- SessionEndingEventArgs.cs
- ActiveXSite.cs
- SoapCodeExporter.cs
- UriSectionReader.cs
- EventWaitHandle.cs
- SQLDecimalStorage.cs
- AutomationIdentifier.cs
- SqlWriter.cs
- SimpleWorkerRequest.cs
- HtmlInputButton.cs