Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / MainMenu.cs / 1305376 / MainMenu.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System; using System.ComponentModel; using System.Drawing; using Microsoft.Win32; using System.Security.Permissions; using System.Runtime.Versioning; ////// /// [ToolboxItemFilter("System.Windows.Forms.MainMenu")] public class MainMenu : Menu { internal Form form; internal Form ownerForm; // this is the form that created this menu, and is the only form allowed to dispose it. private RightToLeft rightToLeft = System.Windows.Forms.RightToLeft.Inherit; private EventHandler onCollapse; ////// Represents /// a menu structure for a form. ////// /// Creates a new MainMenu control. /// public MainMenu() : base(null) { } ////// /// public MainMenu(IContainer container) : this() { if (container == null) { throw new ArgumentNullException("container"); } container.Add(this); } ///Initializes a new instance of the ///class with the specified container. /// /// Creates a new MainMenu control with the given items to start /// with. /// public MainMenu(MenuItem[] items) : base(items) { } ////// /// [SRDescription(SR.MainMenuCollapseDescr)] public event EventHandler Collapse { add { onCollapse += value; } remove { onCollapse -= value; } } ///[To be supplied.] ////// /// This is used for international applications where the language /// is written from RightToLeft. When this property is true, /// text alignment and reading order will be from right to left. /// // VSWhidbey 94189: Add an AmbientValue attribute so that the Reset context menu becomes available in the Property Grid. [ Localizable(true), AmbientValue(RightToLeft.Inherit), SRDescription(SR.MenuRightToLeftDescr) ] public virtual RightToLeft RightToLeft { get { if (System.Windows.Forms.RightToLeft.Inherit == rightToLeft) { if (form != null) { return form.RightToLeft; } else { return RightToLeft.Inherit; } } else { return rightToLeft; } } set { //valid values are 0x0 to 0x2 if (!ClientUtils.IsEnumValid(value, (int)value, (int)RightToLeft.No, (int)RightToLeft.Inherit)){ throw new InvalidEnumArgumentException("RightToLeft", (int)value, typeof(RightToLeft)); } if (rightToLeft != value) { rightToLeft = value; UpdateRtl((value == System.Windows.Forms.RightToLeft.Yes)); } } } internal override bool RenderIsRightToLeft { get { return (RightToLeft == System.Windows.Forms.RightToLeft.Yes && (form == null || !form.IsMirrored)); } } ////// /// Creates a new MainMenu object which is a dupliate of this one. /// public virtual MainMenu CloneMenu() { MainMenu newMenu = new MainMenu(); newMenu.CloneMenu(this); return newMenu; } ////// /// ///[ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] protected override IntPtr CreateMenuHandle() { return UnsafeNativeMethods.CreateMenu(); } /// /// /// Clears out this MainMenu object and discards all of it's resources. /// If the menu is parented in a form, it is disconnected from that as /// well. /// protected override void Dispose(bool disposing) { if (disposing) { if (form != null && (ownerForm == null || form == ownerForm)) { form.Menu = null; } } base.Dispose(disposing); } ////// /// Indicates which form in which we are currently residing [if any] /// [UIPermission(SecurityAction.Demand, Window=UIPermissionWindow.AllWindows)] public Form GetForm() { return form; } internal Form GetFormUnsafe() { return form; } ////// /// ///internal override void ItemsChanged(int change) { base.ItemsChanged(change); if (form != null) form.MenuChanged(change, this); } /// /// /// ///internal virtual void ItemsChanged(int change, Menu menu) { if (form != null) form.MenuChanged(change, menu); } /// /// /// Fires the collapse event /// protected internal virtual void OnCollapse(EventArgs e) { if (onCollapse != null) { onCollapse(this, e); } } ////// /// Returns true if the RightToLeft should be persisted in code gen. /// internal virtual bool ShouldSerializeRightToLeft() { if (System.Windows.Forms.RightToLeft.Inherit == RightToLeft) { return false; } return true; } ////// /// Returns a string representation for this control. /// ///public override string ToString() { // VSWhidbey 495300: removing GetForm information return base.ToString(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System; using System.ComponentModel; using System.Drawing; using Microsoft.Win32; using System.Security.Permissions; using System.Runtime.Versioning; ////// /// [ToolboxItemFilter("System.Windows.Forms.MainMenu")] public class MainMenu : Menu { internal Form form; internal Form ownerForm; // this is the form that created this menu, and is the only form allowed to dispose it. private RightToLeft rightToLeft = System.Windows.Forms.RightToLeft.Inherit; private EventHandler onCollapse; ////// Represents /// a menu structure for a form. ////// /// Creates a new MainMenu control. /// public MainMenu() : base(null) { } ////// /// public MainMenu(IContainer container) : this() { if (container == null) { throw new ArgumentNullException("container"); } container.Add(this); } ///Initializes a new instance of the ///class with the specified container. /// /// Creates a new MainMenu control with the given items to start /// with. /// public MainMenu(MenuItem[] items) : base(items) { } ////// /// [SRDescription(SR.MainMenuCollapseDescr)] public event EventHandler Collapse { add { onCollapse += value; } remove { onCollapse -= value; } } ///[To be supplied.] ////// /// This is used for international applications where the language /// is written from RightToLeft. When this property is true, /// text alignment and reading order will be from right to left. /// // VSWhidbey 94189: Add an AmbientValue attribute so that the Reset context menu becomes available in the Property Grid. [ Localizable(true), AmbientValue(RightToLeft.Inherit), SRDescription(SR.MenuRightToLeftDescr) ] public virtual RightToLeft RightToLeft { get { if (System.Windows.Forms.RightToLeft.Inherit == rightToLeft) { if (form != null) { return form.RightToLeft; } else { return RightToLeft.Inherit; } } else { return rightToLeft; } } set { //valid values are 0x0 to 0x2 if (!ClientUtils.IsEnumValid(value, (int)value, (int)RightToLeft.No, (int)RightToLeft.Inherit)){ throw new InvalidEnumArgumentException("RightToLeft", (int)value, typeof(RightToLeft)); } if (rightToLeft != value) { rightToLeft = value; UpdateRtl((value == System.Windows.Forms.RightToLeft.Yes)); } } } internal override bool RenderIsRightToLeft { get { return (RightToLeft == System.Windows.Forms.RightToLeft.Yes && (form == null || !form.IsMirrored)); } } ////// /// Creates a new MainMenu object which is a dupliate of this one. /// public virtual MainMenu CloneMenu() { MainMenu newMenu = new MainMenu(); newMenu.CloneMenu(this); return newMenu; } ////// /// ///[ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] protected override IntPtr CreateMenuHandle() { return UnsafeNativeMethods.CreateMenu(); } /// /// /// Clears out this MainMenu object and discards all of it's resources. /// If the menu is parented in a form, it is disconnected from that as /// well. /// protected override void Dispose(bool disposing) { if (disposing) { if (form != null && (ownerForm == null || form == ownerForm)) { form.Menu = null; } } base.Dispose(disposing); } ////// /// Indicates which form in which we are currently residing [if any] /// [UIPermission(SecurityAction.Demand, Window=UIPermissionWindow.AllWindows)] public Form GetForm() { return form; } internal Form GetFormUnsafe() { return form; } ////// /// ///internal override void ItemsChanged(int change) { base.ItemsChanged(change); if (form != null) form.MenuChanged(change, this); } /// /// /// ///internal virtual void ItemsChanged(int change, Menu menu) { if (form != null) form.MenuChanged(change, menu); } /// /// /// Fires the collapse event /// protected internal virtual void OnCollapse(EventArgs e) { if (onCollapse != null) { onCollapse(this, e); } } ////// /// Returns true if the RightToLeft should be persisted in code gen. /// internal virtual bool ShouldSerializeRightToLeft() { if (System.Windows.Forms.RightToLeft.Inherit == RightToLeft) { return false; } return true; } ////// /// Returns a string representation for this control. /// ///public override string ToString() { // VSWhidbey 495300: removing GetForm information return base.ToString(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
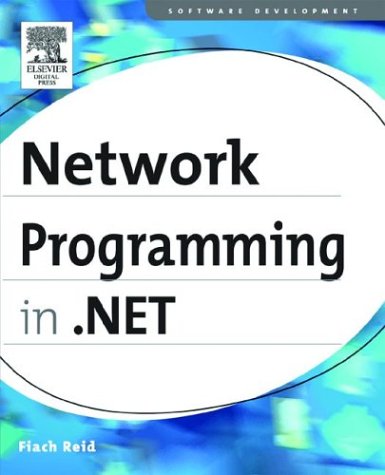
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PhysicalOps.cs
- ToolStripKeyboardHandlingService.cs
- FormatConvertedBitmap.cs
- TemplateBuilder.cs
- ImageMapEventArgs.cs
- DifferencingCollection.cs
- PersonalizableTypeEntry.cs
- CodeParameterDeclarationExpression.cs
- ContactManager.cs
- ResourceBinder.cs
- RegistrationServices.cs
- Number.cs
- ProcessHostFactoryHelper.cs
- AutomationPeer.cs
- Track.cs
- TreeViewCancelEvent.cs
- GuidelineSet.cs
- EditorPartChrome.cs
- CompileXomlTask.cs
- DesignerTransactionCloseEvent.cs
- List.cs
- QueryableDataSourceHelper.cs
- XmlSchemaComplexContentExtension.cs
- TypeRestriction.cs
- CommandID.cs
- WebBrowserDocumentCompletedEventHandler.cs
- StartFileNameEditor.cs
- XmlCharCheckingReader.cs
- HttpStreamXmlDictionaryReader.cs
- DataGridViewColumnCollectionDialog.cs
- IsolatedStorageFile.cs
- SqlUtils.cs
- NativeMethods.cs
- XmlCharacterData.cs
- StatementContext.cs
- BindToObject.cs
- SHA384.cs
- TypeExtensionConverter.cs
- BitSet.cs
- WebPartUtil.cs
- ElementHostAutomationPeer.cs
- InputProcessorProfilesLoader.cs
- CustomExpressionEventArgs.cs
- StandardOleMarshalObject.cs
- XmlSchemaAppInfo.cs
- UriSectionReader.cs
- InputGestureCollection.cs
- ColorMatrix.cs
- isolationinterop.cs
- XamlSerializerUtil.cs
- ModelService.cs
- SystemUdpStatistics.cs
- LinkDescriptor.cs
- XslVisitor.cs
- ConfigXmlSignificantWhitespace.cs
- XPathDocument.cs
- PointAnimation.cs
- WindowsNonControl.cs
- UserPersonalizationStateInfo.cs
- Rect3D.cs
- BrowsableAttribute.cs
- SqlUtils.cs
- CodeCompileUnit.cs
- FilteredAttributeCollection.cs
- LeftCellWrapper.cs
- GifBitmapDecoder.cs
- Timeline.cs
- KnownTypesHelper.cs
- ReliabilityContractAttribute.cs
- DataGridViewCellLinkedList.cs
- StyleHelper.cs
- querybuilder.cs
- TimeSpanOrInfiniteConverter.cs
- CodeMemberProperty.cs
- ListItemCollection.cs
- DetailsViewRowCollection.cs
- WebHttpElement.cs
- TreePrinter.cs
- FlowDocumentReaderAutomationPeer.cs
- DNS.cs
- AsyncSerializedWorker.cs
- WebPartUtil.cs
- HtmlTitle.cs
- SyntaxCheck.cs
- Monitor.cs
- SignedPkcs7.cs
- EventLogPermissionEntry.cs
- DoubleAverageAggregationOperator.cs
- RegexMatchCollection.cs
- FacetValueContainer.cs
- CompilerWrapper.cs
- HttpHandlersSection.cs
- PathFigureCollectionConverter.cs
- CryptoKeySecurity.cs
- Int64.cs
- StorageInfo.cs
- OneToOneMappingSerializer.cs
- Group.cs
- QilReference.cs
- GPPOINT.cs