Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / PeerNodeAddress.cs / 1 / PeerNodeAddress.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel { using System.Collections.Generic; using System.Globalization; using System.Collections.ObjectModel; using System.Net; using System.Runtime.Serialization; using System.ServiceModel.Channels; [DataContract(Name = "PeerNodeAddress", Namespace = PeerStrings.Namespace)] [KnownType(typeof(IPAddress[]))] public sealed class PeerNodeAddress { [DataMember(Name = "EndpointAddress")] internal EndpointAddress10 InnerEPR { get { return this.endpointAddress == null ? null : EndpointAddress10.FromEndpointAddress(this.endpointAddress); } set { this.endpointAddress = (value == null ? null : value.ToEndpointAddress()); } } EndpointAddress endpointAddress; string servicePath; ReadOnlyCollectionipAddresses; [DataMember(Name = "IPAddresses")] internal IList ipAddressesDataMember { get { return ipAddresses; } set { ipAddresses = new ReadOnlyCollection ((value == null) ? new IPAddress[0] : value); } } //NOTE: if a default constructor is provided, make sure to review ServicePath property getter. public PeerNodeAddress(EndpointAddress endpointAddress, ReadOnlyCollection ipAddresses) { if (endpointAddress == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("endpointAddress")); if (ipAddresses == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("ipAddresses")); Initialize(endpointAddress, ipAddresses); } void Initialize(EndpointAddress endpointAddress, ReadOnlyCollection ipAddresses) { this.endpointAddress = endpointAddress; servicePath = this.endpointAddress.Uri.PathAndQuery.ToUpperInvariant(); this.ipAddresses = ipAddresses; } public EndpointAddress EndpointAddress { get { return this.endpointAddress; } } internal string ServicePath { get { if (this.servicePath == null) { this.servicePath = this.endpointAddress.Uri.PathAndQuery.ToUpperInvariant(); } return this.servicePath; } } public ReadOnlyCollection IPAddresses { get { if (this.ipAddresses == null) { this.ipAddresses = new ReadOnlyCollection (new IPAddress[0]); } return this.ipAddresses; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
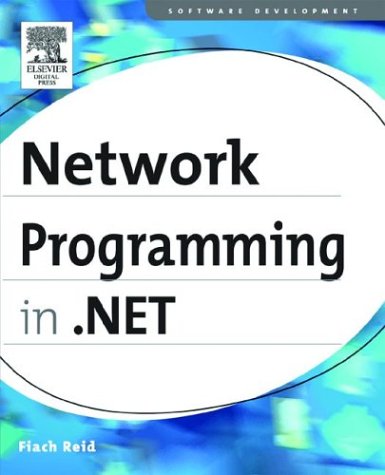
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProjectedSlot.cs
- InputScopeManager.cs
- JoinElimination.cs
- HttpRuntimeSection.cs
- ColorAnimation.cs
- WeakReference.cs
- SequenceNumber.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- MdiWindowListStrip.cs
- XmlBinaryWriter.cs
- AdornerDecorator.cs
- RepeaterItemEventArgs.cs
- PeerSecurityManager.cs
- SecurityMessageProperty.cs
- SQLDoubleStorage.cs
- PerspectiveCamera.cs
- Point3DValueSerializer.cs
- IdleTimeoutMonitor.cs
- ServiceObjectContainer.cs
- ContentPathSegment.cs
- DbInsertCommandTree.cs
- Separator.cs
- ReadOnlyDictionary.cs
- DataColumnCollection.cs
- TriggerActionCollection.cs
- SvcFileManager.cs
- ArcSegment.cs
- XmlArrayAttribute.cs
- Typeface.cs
- GradientSpreadMethodValidation.cs
- FindCriteria.cs
- CheckPair.cs
- MethodImplAttribute.cs
- EnumMemberAttribute.cs
- Thumb.cs
- localization.cs
- InvokeGenerator.cs
- SkipQueryOptionExpression.cs
- Parser.cs
- StylusPlugin.cs
- SQlBooleanStorage.cs
- DefaultPerformanceCounters.cs
- DataReaderContainer.cs
- ApplicationServicesHostFactory.cs
- HMAC.cs
- DataGridCell.cs
- OptimalTextSource.cs
- DataFieldEditor.cs
- WebBrowserContainer.cs
- LocalizabilityAttribute.cs
- TextTreeTextElementNode.cs
- ApplyImportsAction.cs
- DragDeltaEventArgs.cs
- TextBlock.cs
- Matrix3DConverter.cs
- DecimalAnimationBase.cs
- HttpRequestCacheValidator.cs
- URL.cs
- DropTarget.cs
- ListViewItem.cs
- CompressEmulationStream.cs
- ViewEventArgs.cs
- TextTreeRootNode.cs
- VisualState.cs
- SerialReceived.cs
- RemotingAttributes.cs
- DynamicRendererThreadManager.cs
- DisplayClaim.cs
- ColorKeyFrameCollection.cs
- ConfigurationSectionGroup.cs
- BaseHashHelper.cs
- Quad.cs
- XmlEnumAttribute.cs
- ActivationArguments.cs
- Root.cs
- CipherData.cs
- CompModHelpers.cs
- Processor.cs
- ConnectionConsumerAttribute.cs
- CultureTableRecord.cs
- DBNull.cs
- OletxDependentTransaction.cs
- ObjectSpanRewriter.cs
- ObjectStateEntry.cs
- X509AsymmetricSecurityKey.cs
- SafeNativeMethodsMilCoreApi.cs
- RemotingAttributes.cs
- XmlnsDictionary.cs
- UserMapPath.cs
- DurationConverter.cs
- LookupNode.cs
- InterleavedZipPartStream.cs
- DESCryptoServiceProvider.cs
- InfoCardClaimCollection.cs
- StatusStrip.cs
- ExitEventArgs.cs
- CommandPlan.cs
- Switch.cs
- DynamicILGenerator.cs
- StorageComplexTypeMapping.cs