Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / xsp / System / Web / IdleTimeoutMonitor.cs / 1 / IdleTimeoutMonitor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Request timeout manager -- implements the request timeout mechanism */ namespace System.Web { using System.Threading; using System.Collections; using System.Web.Hosting; using System.Web.Util; internal class IdleTimeoutMonitor { private TimeSpan _idleTimeout; // the timeout value private DateTime _lastEvent; // idle since this time private Timer _timer; private readonly TimeSpan _timerPeriod = new TimeSpan(0, 0, 30); // 30 secs internal IdleTimeoutMonitor(TimeSpan timeout) { _idleTimeout = timeout; _timer = new Timer(new TimerCallback(this.TimerCompletionCallback), null, _timerPeriod, _timerPeriod); _lastEvent = DateTime.UtcNow; } internal void Stop() { // stop the timer if (_timer != null) { lock (this) { if (_timer != null) { ((IDisposable)_timer).Dispose(); _timer = null; } } } } internal DateTime LastEvent { // thread-safe property get { DateTime t; lock (this) { t = _lastEvent; } return t; } set { lock (this) { _lastEvent = value; } } } private void TimerCompletionCallback(Object state) { // user idle timer to trim the free list of app instanced HttpApplicationFactory.TrimApplicationInstances(); // no idle timeout if (_idleTimeout == TimeSpan.MaxValue) return; // don't do idle timeout if already shutting down if (HostingEnvironment.ShutdownInitiated) return; // check if there are active requests if (HostingEnvironment.BusyCount != 0) return; // check if enough time passed if (DateTime.UtcNow <= LastEvent.Add(_idleTimeout)) return; // check if debugger is attached if (System.Diagnostics.Debugger.IsAttached) return; // shutdown HttpRuntime.SetShutdownReason(ApplicationShutdownReason.IdleTimeout, SR.GetString(SR.Hosting_Env_IdleTimeout)); HostingEnvironment.InitiateShutdown(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Request timeout manager -- implements the request timeout mechanism */ namespace System.Web { using System.Threading; using System.Collections; using System.Web.Hosting; using System.Web.Util; internal class IdleTimeoutMonitor { private TimeSpan _idleTimeout; // the timeout value private DateTime _lastEvent; // idle since this time private Timer _timer; private readonly TimeSpan _timerPeriod = new TimeSpan(0, 0, 30); // 30 secs internal IdleTimeoutMonitor(TimeSpan timeout) { _idleTimeout = timeout; _timer = new Timer(new TimerCallback(this.TimerCompletionCallback), null, _timerPeriod, _timerPeriod); _lastEvent = DateTime.UtcNow; } internal void Stop() { // stop the timer if (_timer != null) { lock (this) { if (_timer != null) { ((IDisposable)_timer).Dispose(); _timer = null; } } } } internal DateTime LastEvent { // thread-safe property get { DateTime t; lock (this) { t = _lastEvent; } return t; } set { lock (this) { _lastEvent = value; } } } private void TimerCompletionCallback(Object state) { // user idle timer to trim the free list of app instanced HttpApplicationFactory.TrimApplicationInstances(); // no idle timeout if (_idleTimeout == TimeSpan.MaxValue) return; // don't do idle timeout if already shutting down if (HostingEnvironment.ShutdownInitiated) return; // check if there are active requests if (HostingEnvironment.BusyCount != 0) return; // check if enough time passed if (DateTime.UtcNow <= LastEvent.Add(_idleTimeout)) return; // check if debugger is attached if (System.Diagnostics.Debugger.IsAttached) return; // shutdown HttpRuntime.SetShutdownReason(ApplicationShutdownReason.IdleTimeout, SR.GetString(SR.Hosting_Env_IdleTimeout)); HostingEnvironment.InitiateShutdown(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
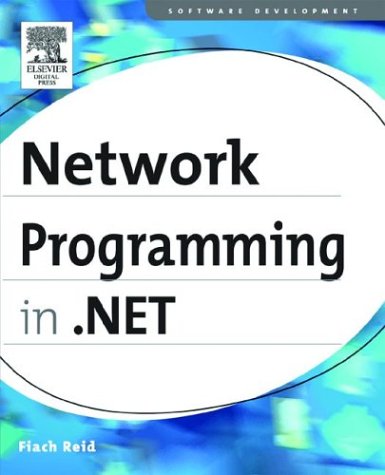
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Errors.cs
- SoundPlayerAction.cs
- EntityClientCacheKey.cs
- XmlBoundElement.cs
- Binding.cs
- IndentedWriter.cs
- WebPartDisplayMode.cs
- DropDownList.cs
- BinaryExpression.cs
- DataObjectSettingDataEventArgs.cs
- SqlDuplicator.cs
- FormatException.cs
- FormatVersion.cs
- Int32Rect.cs
- QuaternionRotation3D.cs
- Monitor.cs
- CodeGeneratorOptions.cs
- SoapProtocolImporter.cs
- CodeVariableReferenceExpression.cs
- DirectoryInfo.cs
- ToolStripMenuItem.cs
- StickyNoteAnnotations.cs
- WebPartsSection.cs
- ChangeBlockUndoRecord.cs
- StateFinalizationDesigner.cs
- XPathAncestorIterator.cs
- KeyPullup.cs
- CodeDirectoryCompiler.cs
- TargetParameterCountException.cs
- Stream.cs
- HttpRequestCacheValidator.cs
- InputBinding.cs
- ContextMenuAutomationPeer.cs
- InstallerTypeAttribute.cs
- ConfigPathUtility.cs
- AuthenticationService.cs
- CheckBoxFlatAdapter.cs
- GlobalizationSection.cs
- SecurityUtils.cs
- HistoryEventArgs.cs
- CodeSubDirectoriesCollection.cs
- NotImplementedException.cs
- Single.cs
- _ListenerRequestStream.cs
- CfgParser.cs
- FragmentQueryProcessor.cs
- SerializationSectionGroup.cs
- CustomCredentialPolicy.cs
- DataReceivedEventArgs.cs
- XmlTextEncoder.cs
- dataobject.cs
- KeyedHashAlgorithm.cs
- ExpressionTextBoxAutomationPeer.cs
- HostVisual.cs
- WebHttpEndpoint.cs
- SetterBase.cs
- ScrollPattern.cs
- FieldNameLookup.cs
- TextDecorationCollection.cs
- MatrixCamera.cs
- DetailsViewRow.cs
- ListViewInsertEventArgs.cs
- HttpCapabilitiesEvaluator.cs
- ValueOfAction.cs
- IIS7WorkerRequest.cs
- RuntimeConfigLKG.cs
- XamlSerializerUtil.cs
- DbModificationClause.cs
- DriveNotFoundException.cs
- SettingsPropertyValue.cs
- GridViewPageEventArgs.cs
- TTSEvent.cs
- StylusPointPropertyId.cs
- MdiWindowListItemConverter.cs
- WorkflowMessageEventHandler.cs
- RemotingConfigParser.cs
- DebugHandleTracker.cs
- LinqDataSourceStatusEventArgs.cs
- CopyAttributesAction.cs
- AnnotationComponentManager.cs
- UriTemplateMatchException.cs
- AppDomainManager.cs
- HtmlWindow.cs
- SubMenuStyleCollection.cs
- ImageCreator.cs
- IdentityReference.cs
- HtmlImage.cs
- TreeWalker.cs
- SqlDataSourceView.cs
- DeferredElementTreeState.cs
- WebSysDisplayNameAttribute.cs
- XamlTemplateSerializer.cs
- VectorAnimationBase.cs
- Utilities.cs
- ILGenerator.cs
- OverflowException.cs
- DefaultValueAttribute.cs
- KnownTypesHelper.cs
- HScrollBar.cs
- StoryFragments.cs