Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / TriggerActionCollection.cs / 1 / TriggerActionCollection.cs
/****************************************************************************\ * * File: TriggerActionCollection.cs * * A collection of TriggerAction objects to be associated with a Trigger * object * * Copyright (C) by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System.Collections; // IList using System.Collections.Generic; // IListusing MS.Internal; using System.Diagnostics; namespace System.Windows { /// /// A set of TriggerAction for use in a Trigger object /// public sealed class TriggerActionCollection : IList, IList{ /////////////////////////////////////////////////////////////////////// // Public members /// /// Creates a TriggerActionCollection /// public TriggerActionCollection() { _rawList = new List(); } /// /// Creates a TriggerActionCollection starting at the given size /// public TriggerActionCollection(int initialSize) { _rawList = new List(initialSize); } /////////////////////////////////////////////////////////////////////// // Public non-type-specific properties and methods that satisfy // implementation requirements of both IList and IList /// /// ICollection.Count /// public int Count { get { return _rawList.Count; } } ////// IList.IsReadOnly /// public bool IsReadOnly { get { return _sealed; } } ////// IList.Clear /// public void Clear() { CheckSealed(); for (int i = _rawList.Count - 1; i >= 0; i--) { InheritanceContextHelper.RemoveContextFromObject(_owner, _rawList[i]); } _rawList.Clear(); } ////// IList.RemoveAt /// public void RemoveAt(int index) { CheckSealed(); TriggerAction oldValue = _rawList[index]; InheritanceContextHelper.RemoveContextFromObject(_owner, oldValue); _rawList.RemoveAt(index); } /////////////////////////////////////////////////////////////////////// // Strongly-typed implementations ////// IList.Add /// public void Add(TriggerAction value) { CheckSealed(); InheritanceContextHelper.ProvideContextForObject( _owner, value ); _rawList.Add(value); } ////// IList.Contains /// public bool Contains(TriggerAction value) { return _rawList.Contains(value); } ////// ICollection.CopyTo /// public void CopyTo( TriggerAction[] array, int index ) { _rawList.CopyTo(array, index); } ////// IList.IndexOf /// public int IndexOf(TriggerAction value) { return _rawList.IndexOf(value); } ////// IList.Insert /// public void Insert(int index, TriggerAction value) { CheckSealed(); InheritanceContextHelper.ProvideContextForObject(_owner, value ); _rawList.Insert(index, value); } ////// IList.Remove /// public bool Remove(TriggerAction value) { CheckSealed(); InheritanceContextHelper.RemoveContextFromObject(_owner, value); bool wasRemoved = _rawList.Remove(value); return wasRemoved; } ////// IList.Item /// public TriggerAction this[int index] { get { return _rawList[index]; } set { CheckSealed(); object oldValue = _rawList[index]; InheritanceContextHelper.RemoveContextFromObject(Owner, oldValue as DependencyObject); _rawList[index] = value; } } ////// IEnumerable.GetEnumerator /// [CLSCompliant(false)] public IEnumeratorGetEnumerator() { return _rawList.GetEnumerator(); } /////////////////////////////////////////////////////////////////////// // Object-based implementations that can be removed once Parser // has IList support for strong typing. int IList.Add(object value) { CheckSealed(); InheritanceContextHelper.ProvideContextForObject(_owner, value as DependencyObject); int index = ((IList) _rawList).Add(VerifyIsTriggerAction(value)); return index; } bool IList.Contains(object value) { return _rawList.Contains(VerifyIsTriggerAction(value)); } int IList.IndexOf(object value) { return _rawList.IndexOf(VerifyIsTriggerAction(value)); } void IList.Insert(int index, object value) { Insert(index, VerifyIsTriggerAction(value)); } bool IList.IsFixedSize { get { return _sealed; } } void IList.Remove(object value) { Remove(VerifyIsTriggerAction(value)); } object IList.this[int index] { get { return _rawList[index]; } set { this[index] = VerifyIsTriggerAction(value); } } void ICollection.CopyTo(Array array, int index) { ((ICollection)_rawList).CopyTo(array, index); } object ICollection.SyncRoot { get { return this; } } bool ICollection.IsSynchronized { get { return false; } } IEnumerator IEnumerable.GetEnumerator() { return ((IEnumerable)_rawList).GetEnumerator(); } /////////////////////////////////////////////////////////////////////// // Internal members internal void Seal(TriggerBase containingTrigger ) { for( int i = 0; i < _rawList.Count; i++ ) { _rawList[i].Seal(containingTrigger); } } // The event trigger that we're in internal DependencyObject Owner { get { return _owner; } set { Debug.Assert (Owner == null); _owner = value; } } /////////////////////////////////////////////////////////////////////// // Private members // Throw if a change is attempted at a time we're not allowing them private void CheckSealed() { if ( _sealed ) { throw new InvalidOperationException(SR.Get(SRID.CannotChangeAfterSealed, "TriggerActionCollection")); } } // Throw if the given object isn't a TriggerAction private TriggerAction VerifyIsTriggerAction(object value) { TriggerAction action = value as TriggerAction; if( action == null ) { if( value == null ) { throw new ArgumentNullException("value"); } else { throw new ArgumentException(SR.Get(SRID.MustBeTriggerAction)); } } return action; } // The actual underlying storage for our TriggerActions private List _rawList; // Whether we are allowing further changes to the collection private bool _sealed = false; // The event trigger that we're in private DependencyObject _owner = null; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /****************************************************************************\ * * File: TriggerActionCollection.cs * * A collection of TriggerAction objects to be associated with a Trigger * object * * Copyright (C) by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System.Collections; // IList using System.Collections.Generic; // IList using MS.Internal; using System.Diagnostics; namespace System.Windows { /// /// A set of TriggerAction for use in a Trigger object /// public sealed class TriggerActionCollection : IList, IList{ /////////////////////////////////////////////////////////////////////// // Public members /// /// Creates a TriggerActionCollection /// public TriggerActionCollection() { _rawList = new List(); } /// /// Creates a TriggerActionCollection starting at the given size /// public TriggerActionCollection(int initialSize) { _rawList = new List(initialSize); } /////////////////////////////////////////////////////////////////////// // Public non-type-specific properties and methods that satisfy // implementation requirements of both IList and IList /// /// ICollection.Count /// public int Count { get { return _rawList.Count; } } ////// IList.IsReadOnly /// public bool IsReadOnly { get { return _sealed; } } ////// IList.Clear /// public void Clear() { CheckSealed(); for (int i = _rawList.Count - 1; i >= 0; i--) { InheritanceContextHelper.RemoveContextFromObject(_owner, _rawList[i]); } _rawList.Clear(); } ////// IList.RemoveAt /// public void RemoveAt(int index) { CheckSealed(); TriggerAction oldValue = _rawList[index]; InheritanceContextHelper.RemoveContextFromObject(_owner, oldValue); _rawList.RemoveAt(index); } /////////////////////////////////////////////////////////////////////// // Strongly-typed implementations ////// IList.Add /// public void Add(TriggerAction value) { CheckSealed(); InheritanceContextHelper.ProvideContextForObject( _owner, value ); _rawList.Add(value); } ////// IList.Contains /// public bool Contains(TriggerAction value) { return _rawList.Contains(value); } ////// ICollection.CopyTo /// public void CopyTo( TriggerAction[] array, int index ) { _rawList.CopyTo(array, index); } ////// IList.IndexOf /// public int IndexOf(TriggerAction value) { return _rawList.IndexOf(value); } ////// IList.Insert /// public void Insert(int index, TriggerAction value) { CheckSealed(); InheritanceContextHelper.ProvideContextForObject(_owner, value ); _rawList.Insert(index, value); } ////// IList.Remove /// public bool Remove(TriggerAction value) { CheckSealed(); InheritanceContextHelper.RemoveContextFromObject(_owner, value); bool wasRemoved = _rawList.Remove(value); return wasRemoved; } ////// IList.Item /// public TriggerAction this[int index] { get { return _rawList[index]; } set { CheckSealed(); object oldValue = _rawList[index]; InheritanceContextHelper.RemoveContextFromObject(Owner, oldValue as DependencyObject); _rawList[index] = value; } } ////// IEnumerable.GetEnumerator /// [CLSCompliant(false)] public IEnumeratorGetEnumerator() { return _rawList.GetEnumerator(); } /////////////////////////////////////////////////////////////////////// // Object-based implementations that can be removed once Parser // has IList support for strong typing. int IList.Add(object value) { CheckSealed(); InheritanceContextHelper.ProvideContextForObject(_owner, value as DependencyObject); int index = ((IList) _rawList).Add(VerifyIsTriggerAction(value)); return index; } bool IList.Contains(object value) { return _rawList.Contains(VerifyIsTriggerAction(value)); } int IList.IndexOf(object value) { return _rawList.IndexOf(VerifyIsTriggerAction(value)); } void IList.Insert(int index, object value) { Insert(index, VerifyIsTriggerAction(value)); } bool IList.IsFixedSize { get { return _sealed; } } void IList.Remove(object value) { Remove(VerifyIsTriggerAction(value)); } object IList.this[int index] { get { return _rawList[index]; } set { this[index] = VerifyIsTriggerAction(value); } } void ICollection.CopyTo(Array array, int index) { ((ICollection)_rawList).CopyTo(array, index); } object ICollection.SyncRoot { get { return this; } } bool ICollection.IsSynchronized { get { return false; } } IEnumerator IEnumerable.GetEnumerator() { return ((IEnumerable)_rawList).GetEnumerator(); } /////////////////////////////////////////////////////////////////////// // Internal members internal void Seal(TriggerBase containingTrigger ) { for( int i = 0; i < _rawList.Count; i++ ) { _rawList[i].Seal(containingTrigger); } } // The event trigger that we're in internal DependencyObject Owner { get { return _owner; } set { Debug.Assert (Owner == null); _owner = value; } } /////////////////////////////////////////////////////////////////////// // Private members // Throw if a change is attempted at a time we're not allowing them private void CheckSealed() { if ( _sealed ) { throw new InvalidOperationException(SR.Get(SRID.CannotChangeAfterSealed, "TriggerActionCollection")); } } // Throw if the given object isn't a TriggerAction private TriggerAction VerifyIsTriggerAction(object value) { TriggerAction action = value as TriggerAction; if( action == null ) { if( value == null ) { throw new ArgumentNullException("value"); } else { throw new ArgumentException(SR.Get(SRID.MustBeTriggerAction)); } } return action; } // The actual underlying storage for our TriggerActions private List _rawList; // Whether we are allowing further changes to the collection private bool _sealed = false; // The event trigger that we're in private DependencyObject _owner = null; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
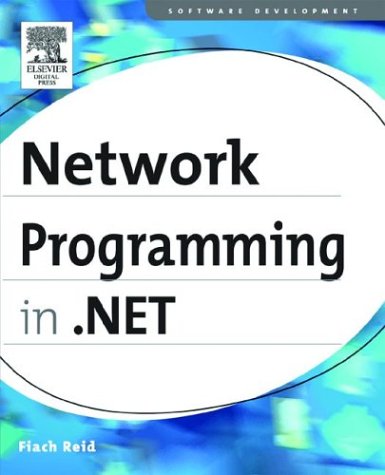
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SslStream.cs
- EntityContainerEmitter.cs
- AttributeQuery.cs
- ProcessModuleCollection.cs
- XmlExpressionDumper.cs
- CompositeControl.cs
- LOSFormatter.cs
- CombinedGeometry.cs
- GregorianCalendarHelper.cs
- ExceptionDetail.cs
- ToggleButtonAutomationPeer.cs
- TransactionState.cs
- entitydatasourceentitysetnameconverter.cs
- DbgCompiler.cs
- ExtendedProtectionPolicyElement.cs
- MultiView.cs
- DispatcherSynchronizationContext.cs
- Attributes.cs
- StringValidator.cs
- PersonalizationProviderCollection.cs
- ListViewInsertedEventArgs.cs
- DataServiceHostFactory.cs
- OutputCacheModule.cs
- WebConfigurationHostFileChange.cs
- SqlDataSourceView.cs
- PolyBezierSegment.cs
- MetaModel.cs
- sqlinternaltransaction.cs
- BevelBitmapEffect.cs
- BooleanToSelectiveScrollingOrientationConverter.cs
- DependentList.cs
- DocumentXPathNavigator.cs
- PrincipalPermission.cs
- BamlVersionHeader.cs
- CustomTypeDescriptor.cs
- EraserBehavior.cs
- TableLayoutSettingsTypeConverter.cs
- HGlobalSafeHandle.cs
- LifetimeServices.cs
- WindowsNonControl.cs
- CreateUserErrorEventArgs.cs
- FormViewUpdatedEventArgs.cs
- CatalogPart.cs
- EditingScopeUndoUnit.cs
- TypeContext.cs
- SignedXmlDebugLog.cs
- InvalidComObjectException.cs
- ListParagraph.cs
- Merger.cs
- CommonDialog.cs
- SHA256.cs
- MorphHelper.cs
- Span.cs
- DbProviderFactories.cs
- ipaddressinformationcollection.cs
- MaterialGroup.cs
- XmlSchemaGroup.cs
- ImageField.cs
- KoreanCalendar.cs
- BindingMAnagerBase.cs
- Renderer.cs
- TextContainer.cs
- RSACryptoServiceProvider.cs
- CommonRemoteMemoryBlock.cs
- PackageDocument.cs
- CatalogPartChrome.cs
- DataKeyArray.cs
- TextBreakpoint.cs
- SkipQueryOptionExpression.cs
- HttpCapabilitiesBase.cs
- ApplicationDirectoryMembershipCondition.cs
- SerializeAbsoluteContext.cs
- ZoneButton.cs
- SemaphoreSlim.cs
- CardSpacePolicyElement.cs
- KeyValuePairs.cs
- CollectionViewSource.cs
- HttpCacheVary.cs
- ObjectTag.cs
- SrgsDocumentParser.cs
- ProcessHostMapPath.cs
- DataBoundControlHelper.cs
- TraceUtility.cs
- TraceContextRecord.cs
- ServicePointManager.cs
- DataGridViewLinkColumn.cs
- Hash.cs
- SqlEnums.cs
- RewritingValidator.cs
- ZipIOBlockManager.cs
- nulltextnavigator.cs
- SoapAttributeOverrides.cs
- JpegBitmapDecoder.cs
- RecordConverter.cs
- _HelperAsyncResults.cs
- KnownTypes.cs
- ExpressionWriter.cs
- BaseAsyncResult.cs
- CredentialCache.cs
- httpapplicationstate.cs