Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Base / System / Windows / DependencyObjectType.cs / 1 / DependencyObjectType.cs
using System; using System.Collections; using System.Diagnostics; using System.Runtime.CompilerServices; using System.Windows.Threading; using MS.Utility; using MS.Internal.WindowsBase; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings namespace System.Windows { ////// Type cache for all DependencyObject derived types /// ////// Every public class DependencyObjectType { ///stores a reference to its DependencyObjectType. /// This is an object that represents a specific system (CLR) Type. /// /// DTypes have 2 purposes: /// ///
///- /// More performant type operations (especially for Expressions that /// rely heavily on type inspection) ///
///- /// Forces static constructors of base types to always run first. This /// consistancy is necessary for components (such as Expressions) that /// rely on static construction order for correctness. ///
////// Retrieve a DependencyObjectType that represents a given system (CLR) type /// /// The system (CLR) type to convert ////// A DependencyObjectType that represents the system (CLR) type (will create /// a new one if doesn't exist) /// public static DependencyObjectType FromSystemType(Type systemType) { if (systemType == null) { throw new ArgumentNullException("systemType"); } if (!typeof(DependencyObject).IsAssignableFrom(systemType)) { #pragma warning suppress 6506 // systemType is obviously not null throw new ArgumentException(SR.Get(SRID.DTypeNotSupportForSystemType, systemType.Name)); } return FromSystemTypeInternal(systemType); } ////// Helper method for the public FromSystemType call but without /// the expensive IsAssignableFrom parameter validation. /// [FriendAccessAllowed] // Built into Base, also used by Framework. internal static DependencyObjectType FromSystemTypeInternal(Type systemType) { Debug.Assert(systemType != null && typeof(DependencyObject).IsAssignableFrom(systemType), "Invalid systemType argument"); DependencyObjectType retVal; lock(_lock) { // Recursive routine to (set up if necessary) and use the // DTypeFromCLRType hashtable that is used for the actual lookup. retVal = FromSystemTypeRecursive( systemType ); } return retVal; } // The caller must wrap this routine inside a locked block. // This recursive routine manipulates the static hashtable DTypeFromCLRType // and it must not be allowed to do this across multiple threads // simultaneously. private static DependencyObjectType FromSystemTypeRecursive(Type systemType) { DependencyObjectType dType; // Map a CLR Type to a DependencyObjectType dType = (DependencyObjectType)DTypeFromCLRType[systemType]; if (dType == null) { // No DependencyObjectType found, create dType = new DependencyObjectType(); // Store CLR type dType._systemType = systemType; // Store reverse mapping DTypeFromCLRType[systemType] = dType; // Establish base DependencyObjectType and base property count if (systemType != typeof(DependencyObject)) { // Get base type dType._baseDType = FromSystemTypeRecursive(systemType.BaseType); } // Store DependencyObjectType zero-based Id dType._id = DTypeCount++; } return dType; } ////// Zero-based unique identifier for constant-time array lookup operations /// ////// There is no guarantee on this value. It can vary between application runs. /// public int Id { get { return _id; } } ////// The system (CLR) type that this DependencyObjectType represents /// public Type SystemType { get { return _systemType; } } ////// The DependencyObjectType of the base class /// public DependencyObjectType BaseType { get { return _baseDType; } } ////// Returns the name of the represented system (CLR) type /// public string Name { get { return SystemType.Name; } } ////// Determines whether the specifed object is an instance of the current DependencyObjectType /// /// The object to compare with the current Type ////// true if the current DependencyObjectType is in the inheritance hierarchy of the /// object represented by the o parameter. false otherwise. /// public bool IsInstanceOfType(DependencyObject dependencyObject) { if (dependencyObject != null) { DependencyObjectType dType = dependencyObject.DependencyObjectType; do { if (dType.Id == Id) { return true; } dType = dType._baseDType; } while (dType != null); } return false; } ////// Determines whether the current DependencyObjectType derives from the /// specified DependencyObjectType /// /// The DependencyObjectType to compare /// with the current DependencyObjectType ////// true if the DependencyObjectType represented by the dType parameter and the /// current DependencyObjectType represent classes, and the class represented /// by the current DependencyObjectType derives from the class represented by /// c; otherwise, false. This method also returns false if dType and the /// current Type represent the same class. /// public bool IsSubclassOf(DependencyObjectType dependencyObjectType) { // Check for null and return false, since this type is never a subclass of null. if (dependencyObjectType != null) { // A DependencyObjectType isn't considered a subclass of itself, so start with base type DependencyObjectType dType = _baseDType; while (dType != null) { if (dType.Id == dependencyObjectType.Id) { return true; } dType = dType._baseDType; } } return false; } ////// Serves as a hash function for a particular type, suitable for use in /// hashing algorithms and data structures like a hash table /// ///The DependencyObjectType's Id public override int GetHashCode() { return _id; } // DTypes may not be constructed outside of FromSystemType private DependencyObjectType() { } private int _id; private Type _systemType; private DependencyObjectType _baseDType; // Synchronized: Covered by DispatcherLock private static Hashtable DTypeFromCLRType = new Hashtable(); // Synchronized: Covered by DispatcherLock private static int DTypeCount = 0; private static object _lock = new object(); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections; using System.Diagnostics; using System.Runtime.CompilerServices; using System.Windows.Threading; using MS.Utility; using MS.Internal.WindowsBase; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings namespace System.Windows { ////// Type cache for all DependencyObject derived types /// ////// Every public class DependencyObjectType { ///stores a reference to its DependencyObjectType. /// This is an object that represents a specific system (CLR) Type. /// /// DTypes have 2 purposes: /// ///
///- /// More performant type operations (especially for Expressions that /// rely heavily on type inspection) ///
///- /// Forces static constructors of base types to always run first. This /// consistancy is necessary for components (such as Expressions) that /// rely on static construction order for correctness. ///
////// Retrieve a DependencyObjectType that represents a given system (CLR) type /// /// The system (CLR) type to convert ////// A DependencyObjectType that represents the system (CLR) type (will create /// a new one if doesn't exist) /// public static DependencyObjectType FromSystemType(Type systemType) { if (systemType == null) { throw new ArgumentNullException("systemType"); } if (!typeof(DependencyObject).IsAssignableFrom(systemType)) { #pragma warning suppress 6506 // systemType is obviously not null throw new ArgumentException(SR.Get(SRID.DTypeNotSupportForSystemType, systemType.Name)); } return FromSystemTypeInternal(systemType); } ////// Helper method for the public FromSystemType call but without /// the expensive IsAssignableFrom parameter validation. /// [FriendAccessAllowed] // Built into Base, also used by Framework. internal static DependencyObjectType FromSystemTypeInternal(Type systemType) { Debug.Assert(systemType != null && typeof(DependencyObject).IsAssignableFrom(systemType), "Invalid systemType argument"); DependencyObjectType retVal; lock(_lock) { // Recursive routine to (set up if necessary) and use the // DTypeFromCLRType hashtable that is used for the actual lookup. retVal = FromSystemTypeRecursive( systemType ); } return retVal; } // The caller must wrap this routine inside a locked block. // This recursive routine manipulates the static hashtable DTypeFromCLRType // and it must not be allowed to do this across multiple threads // simultaneously. private static DependencyObjectType FromSystemTypeRecursive(Type systemType) { DependencyObjectType dType; // Map a CLR Type to a DependencyObjectType dType = (DependencyObjectType)DTypeFromCLRType[systemType]; if (dType == null) { // No DependencyObjectType found, create dType = new DependencyObjectType(); // Store CLR type dType._systemType = systemType; // Store reverse mapping DTypeFromCLRType[systemType] = dType; // Establish base DependencyObjectType and base property count if (systemType != typeof(DependencyObject)) { // Get base type dType._baseDType = FromSystemTypeRecursive(systemType.BaseType); } // Store DependencyObjectType zero-based Id dType._id = DTypeCount++; } return dType; } ////// Zero-based unique identifier for constant-time array lookup operations /// ////// There is no guarantee on this value. It can vary between application runs. /// public int Id { get { return _id; } } ////// The system (CLR) type that this DependencyObjectType represents /// public Type SystemType { get { return _systemType; } } ////// The DependencyObjectType of the base class /// public DependencyObjectType BaseType { get { return _baseDType; } } ////// Returns the name of the represented system (CLR) type /// public string Name { get { return SystemType.Name; } } ////// Determines whether the specifed object is an instance of the current DependencyObjectType /// /// The object to compare with the current Type ////// true if the current DependencyObjectType is in the inheritance hierarchy of the /// object represented by the o parameter. false otherwise. /// public bool IsInstanceOfType(DependencyObject dependencyObject) { if (dependencyObject != null) { DependencyObjectType dType = dependencyObject.DependencyObjectType; do { if (dType.Id == Id) { return true; } dType = dType._baseDType; } while (dType != null); } return false; } ////// Determines whether the current DependencyObjectType derives from the /// specified DependencyObjectType /// /// The DependencyObjectType to compare /// with the current DependencyObjectType ////// true if the DependencyObjectType represented by the dType parameter and the /// current DependencyObjectType represent classes, and the class represented /// by the current DependencyObjectType derives from the class represented by /// c; otherwise, false. This method also returns false if dType and the /// current Type represent the same class. /// public bool IsSubclassOf(DependencyObjectType dependencyObjectType) { // Check for null and return false, since this type is never a subclass of null. if (dependencyObjectType != null) { // A DependencyObjectType isn't considered a subclass of itself, so start with base type DependencyObjectType dType = _baseDType; while (dType != null) { if (dType.Id == dependencyObjectType.Id) { return true; } dType = dType._baseDType; } } return false; } ////// Serves as a hash function for a particular type, suitable for use in /// hashing algorithms and data structures like a hash table /// ///The DependencyObjectType's Id public override int GetHashCode() { return _id; } // DTypes may not be constructed outside of FromSystemType private DependencyObjectType() { } private int _id; private Type _systemType; private DependencyObjectType _baseDType; // Synchronized: Covered by DispatcherLock private static Hashtable DTypeFromCLRType = new Hashtable(); // Synchronized: Covered by DispatcherLock private static int DTypeCount = 0; private static object _lock = new object(); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
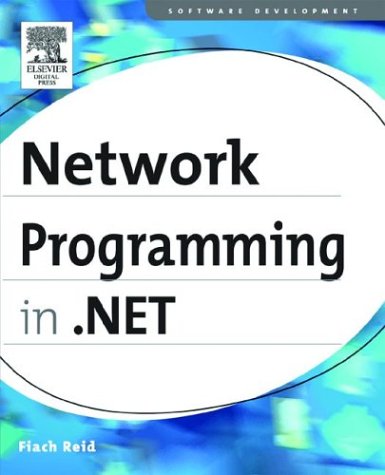
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PageThemeCodeDomTreeGenerator.cs
- LinqDataSourceContextEventArgs.cs
- ClientTarget.cs
- SqlClientPermission.cs
- MediaEntryAttribute.cs
- SessionEndedEventArgs.cs
- _LocalDataStoreMgr.cs
- ListenerBinder.cs
- DbModificationClause.cs
- ApplicationId.cs
- SiteMapSection.cs
- WebControl.cs
- UIElementIsland.cs
- BitmapScalingModeValidation.cs
- DocumentViewerBase.cs
- LabelDesigner.cs
- Win32Exception.cs
- PrimarySelectionAdorner.cs
- GrammarBuilderDictation.cs
- TCEAdapterGenerator.cs
- Point3DAnimationBase.cs
- TcpHostedTransportConfiguration.cs
- RuntimeArgumentHandle.cs
- ConfigurationCollectionAttribute.cs
- DataRowExtensions.cs
- ProfileParameter.cs
- CustomErrorsSection.cs
- TransformerConfigurationWizardBase.cs
- ButtonBase.cs
- StaticSiteMapProvider.cs
- ThreadPool.cs
- ListViewSortEventArgs.cs
- HeaderLabel.cs
- FixedSOMPageConstructor.cs
- ParserOptions.cs
- ProxyManager.cs
- DataGridBoolColumn.cs
- OutputWindow.cs
- _ChunkParse.cs
- SqlVersion.cs
- assemblycache.cs
- LinearGradientBrush.cs
- DataGridViewCellValidatingEventArgs.cs
- StateMachineDesignerPaint.cs
- Message.cs
- TextRunTypographyProperties.cs
- Binding.cs
- FormatSettings.cs
- ListViewPagedDataSource.cs
- CompilationLock.cs
- BinaryCommonClasses.cs
- EditorAttribute.cs
- IsolatedStorageFilePermission.cs
- EntityContainerRelationshipSetEnd.cs
- StrongNameMembershipCondition.cs
- TableLayoutSettingsTypeConverter.cs
- WhereQueryOperator.cs
- ListItemCollection.cs
- ClientTargetCollection.cs
- ResourceDisplayNameAttribute.cs
- SettingsAttributeDictionary.cs
- XmlAttributes.cs
- TransactionState.cs
- ObjectDataSourceSelectingEventArgs.cs
- ClockGroup.cs
- TraceRecords.cs
- FieldInfo.cs
- IndexingContentUnit.cs
- OleDbSchemaGuid.cs
- SerializationSectionGroup.cs
- PageCodeDomTreeGenerator.cs
- BinHexEncoder.cs
- rsa.cs
- VectorAnimationUsingKeyFrames.cs
- AncestorChangedEventArgs.cs
- PackagePart.cs
- SortableBindingList.cs
- ExpressionBinding.cs
- StringBuilder.cs
- TreeViewItem.cs
- DefaultTextStore.cs
- SchemaImporterExtensionElement.cs
- SignerInfo.cs
- DbgCompiler.cs
- ColumnHeader.cs
- Site.cs
- PartitionedStream.cs
- DataGridSortCommandEventArgs.cs
- CommandEventArgs.cs
- MessageQueueInstaller.cs
- SoapRpcServiceAttribute.cs
- SimpleApplicationHost.cs
- SessionState.cs
- XmlLanguage.cs
- DBCSCodePageEncoding.cs
- WindowsFormsSectionHandler.cs
- __ComObject.cs
- UpdateRecord.cs
- HatchBrush.cs
- ExportException.cs