Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / FontStretchConverter.cs / 1 / FontStretchConverter.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: FontStretch type converter. // // History: // 01/25/2005 mleonov - Created. // //--------------------------------------------------------------------------- using System; using System.IO; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using System.Windows.Media; using System.Text; using System.Collections; using System.Globalization; using System.Runtime.InteropServices; using System.Security; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows { ////// FontStretchConverter class parses a font stretch string. /// public sealed class FontStretchConverter : TypeConverter { ////// CanConvertFrom /// public override bool CanConvertFrom(ITypeDescriptorContext td, Type t) { if (t == typeof(string)) { return true; } else { return false; } } ////// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert to ///true if conversion is possible public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor) || destinationType == typeof(string)) { return true; } return base.CanConvertTo(context, destinationType); } ////// ConvertFrom - attempt to convert to a FontStretch from the given object /// ////// A NotSupportedException is thrown if the example object is null or is not a valid type /// which can be converted to a FontStretch. /// public override object ConvertFrom(ITypeDescriptorContext td, CultureInfo ci, object value) { if (null == value) { throw GetConvertFromException(value); } String s = value as string; if (null == s) { throw new ArgumentException(SR.Get(SRID.General_BadType, "ConvertFrom"), "value"); } FontStretch fontStretch = new FontStretch(); if (!FontStretches.FontStretchStringToKnownStretch(s, ci, ref fontStretch)) throw new FormatException(SR.Get(SRID.Parsers_IllegalToken)); return fontStretch; } ////// TypeConverter method implementation. /// ////// An NotSupportedException is thrown if the example object is null or is not a FontStretch, /// or if the destinationType isn't one of the valid destination types. /// /// ITypeDescriptorContext /// current culture (see CLR specs) /// value to convert from /// Type to convert to ///converted value ////// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for FontStretch.FromOpenTypeStretch, not an arbitrary class/method /// [SecurityCritical] public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType != null && value is FontStretch) { if (destinationType == typeof(InstanceDescriptor)) { MethodInfo mi = typeof(FontStretch).GetMethod("FromOpenTypeStretch", new Type[]{typeof(int)}); FontStretch c = (FontStretch)value; return new InstanceDescriptor(mi, new object[]{c.ToOpenTypeStretch()}); } else if (destinationType == typeof(string)) { FontStretch c = (FontStretch)value; return ((IFormattable)c).ToString(null, culture); } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: FontStretch type converter. // // History: // 01/25/2005 mleonov - Created. // //--------------------------------------------------------------------------- using System; using System.IO; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using System.Windows.Media; using System.Text; using System.Collections; using System.Globalization; using System.Runtime.InteropServices; using System.Security; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows { ////// FontStretchConverter class parses a font stretch string. /// public sealed class FontStretchConverter : TypeConverter { ////// CanConvertFrom /// public override bool CanConvertFrom(ITypeDescriptorContext td, Type t) { if (t == typeof(string)) { return true; } else { return false; } } ////// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert to ///true if conversion is possible public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor) || destinationType == typeof(string)) { return true; } return base.CanConvertTo(context, destinationType); } ////// ConvertFrom - attempt to convert to a FontStretch from the given object /// ////// A NotSupportedException is thrown if the example object is null or is not a valid type /// which can be converted to a FontStretch. /// public override object ConvertFrom(ITypeDescriptorContext td, CultureInfo ci, object value) { if (null == value) { throw GetConvertFromException(value); } String s = value as string; if (null == s) { throw new ArgumentException(SR.Get(SRID.General_BadType, "ConvertFrom"), "value"); } FontStretch fontStretch = new FontStretch(); if (!FontStretches.FontStretchStringToKnownStretch(s, ci, ref fontStretch)) throw new FormatException(SR.Get(SRID.Parsers_IllegalToken)); return fontStretch; } ////// TypeConverter method implementation. /// ////// An NotSupportedException is thrown if the example object is null or is not a FontStretch, /// or if the destinationType isn't one of the valid destination types. /// /// ITypeDescriptorContext /// current culture (see CLR specs) /// value to convert from /// Type to convert to ///converted value ////// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for FontStretch.FromOpenTypeStretch, not an arbitrary class/method /// [SecurityCritical] public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType != null && value is FontStretch) { if (destinationType == typeof(InstanceDescriptor)) { MethodInfo mi = typeof(FontStretch).GetMethod("FromOpenTypeStretch", new Type[]{typeof(int)}); FontStretch c = (FontStretch)value; return new InstanceDescriptor(mi, new object[]{c.ToOpenTypeStretch()}); } else if (destinationType == typeof(string)) { FontStretch c = (FontStretch)value; return ((IFormattable)c).ToString(null, culture); } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
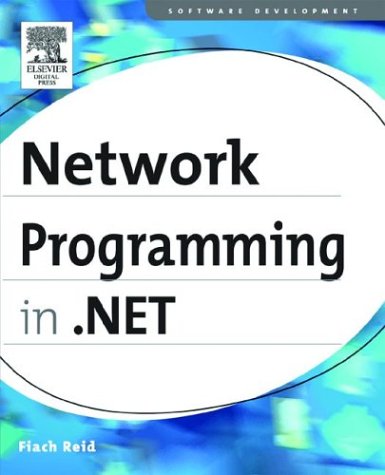
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SinglePageViewer.cs
- ParseElement.cs
- PropertyValueChangedEvent.cs
- TextEditorParagraphs.cs
- DtdParser.cs
- UriScheme.cs
- DataSetSchema.cs
- AdvancedBindingPropertyDescriptor.cs
- PublisherIdentityPermission.cs
- DateTimeFormatInfoScanner.cs
- Zone.cs
- InvalidOleVariantTypeException.cs
- ListMarkerLine.cs
- ApplicationDirectory.cs
- UnsafeNativeMethods.cs
- SqlUnionizer.cs
- ArrayExtension.cs
- Internal.cs
- SingleConverter.cs
- OutputScopeManager.cs
- HostingEnvironment.cs
- EnumValAlphaComparer.cs
- TreeNodeSelectionProcessor.cs
- DataGridViewTextBoxCell.cs
- SqlUtils.cs
- Matrix3D.cs
- PropertyRef.cs
- ManagementEventArgs.cs
- ObjectDataSourceStatusEventArgs.cs
- FilterElement.cs
- PassportAuthentication.cs
- GeometryModel3D.cs
- ConfigurationSettings.cs
- BoolExpression.cs
- FtpRequestCacheValidator.cs
- BrowserTree.cs
- ColorTransform.cs
- FontFamily.cs
- ConnectionPoolRegistry.cs
- XmlDataSource.cs
- SQLDoubleStorage.cs
- DoubleCollection.cs
- CommandBindingCollection.cs
- DataGridViewComboBoxCell.cs
- OleDbFactory.cs
- ExtendedPropertyCollection.cs
- PTProvider.cs
- ReaderContextStackData.cs
- Point4D.cs
- XmlNodeWriter.cs
- DNS.cs
- querybuilder.cs
- SortableBindingList.cs
- SaveFileDialog.cs
- GlobalEventManager.cs
- UpdatePanel.cs
- GPPOINT.cs
- SQLBytes.cs
- _TLSstream.cs
- Mutex.cs
- SchemaAttDef.cs
- AnimationStorage.cs
- DataSourceControl.cs
- Int32CollectionValueSerializer.cs
- ReadOnlyDataSourceView.cs
- XmlTextReaderImplHelpers.cs
- ExpressionBindingCollection.cs
- SchemaElement.cs
- BinaryParser.cs
- RuleSettingsCollection.cs
- Avt.cs
- DataGridItemEventArgs.cs
- EntityViewGenerationConstants.cs
- WebScriptEnablingBehavior.cs
- ShapingWorkspace.cs
- SemanticResolver.cs
- AutoCompleteStringCollection.cs
- WebPartConnectionsCloseVerb.cs
- ResourceType.cs
- DesignerWebPartChrome.cs
- TemplatedEditableDesignerRegion.cs
- WizardDesigner.cs
- LookupBindingPropertiesAttribute.cs
- SmtpFailedRecipientsException.cs
- TypeToken.cs
- FrameworkContentElement.cs
- BindingList.cs
- ConfigUtil.cs
- HtmlMobileTextWriter.cs
- DefaultShape.cs
- CatalogPartCollection.cs
- RtfFormatStack.cs
- ResponseBodyWriter.cs
- Graphics.cs
- ListViewGroupConverter.cs
- RuleInfoComparer.cs
- RichTextBoxConstants.cs
- ProviderIncompatibleException.cs
- KnownIds.cs
- LinqDataSourceSelectEventArgs.cs