Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Xml / System / Xml / XPath / Internal / AttributeQuery.cs / 1305376 / AttributeQuery.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace MS.Internal.Xml.XPath { using System; using System.Xml; using System.Xml.XPath; using System.Diagnostics; using System.Globalization; internal sealed class AttributeQuery : BaseAxisQuery { private bool onAttribute = false; public AttributeQuery(Query qyParent, string Name, string Prefix, XPathNodeType Type) : base(qyParent, Name, Prefix, Type) {} private AttributeQuery(AttributeQuery other) : base(other) { this.onAttribute = other.onAttribute; } public override void Reset() { onAttribute = false; base.Reset(); } public override XPathNavigator Advance() { while (true) { if (! onAttribute) { currentNode = qyInput.Advance(); if (currentNode == null) { return null; } position = 0; currentNode = currentNode.Clone(); onAttribute = currentNode.MoveToFirstAttribute(); } else { onAttribute = currentNode.MoveToNextAttribute(); } if (onAttribute) { Debug.Assert(! currentNode.NamespaceURI.Equals(XmlReservedNs.NsXmlNs)); if (matches(currentNode)) { position++; return currentNode; } } } // while } public override XPathNavigator MatchNode(XPathNavigator context) { if (context != null) { if (context.NodeType == XPathNodeType.Attribute && matches(context)) { XPathNavigator temp = context.Clone(); if (temp.MoveToParent()) { return qyInput.MatchNode(temp); } } } return null; } public override XPathNodeIterator Clone() { return new AttributeQuery(this); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace MS.Internal.Xml.XPath { using System; using System.Xml; using System.Xml.XPath; using System.Diagnostics; using System.Globalization; internal sealed class AttributeQuery : BaseAxisQuery { private bool onAttribute = false; public AttributeQuery(Query qyParent, string Name, string Prefix, XPathNodeType Type) : base(qyParent, Name, Prefix, Type) {} private AttributeQuery(AttributeQuery other) : base(other) { this.onAttribute = other.onAttribute; } public override void Reset() { onAttribute = false; base.Reset(); } public override XPathNavigator Advance() { while (true) { if (! onAttribute) { currentNode = qyInput.Advance(); if (currentNode == null) { return null; } position = 0; currentNode = currentNode.Clone(); onAttribute = currentNode.MoveToFirstAttribute(); } else { onAttribute = currentNode.MoveToNextAttribute(); } if (onAttribute) { Debug.Assert(! currentNode.NamespaceURI.Equals(XmlReservedNs.NsXmlNs)); if (matches(currentNode)) { position++; return currentNode; } } } // while } public override XPathNavigator MatchNode(XPathNavigator context) { if (context != null) { if (context.NodeType == XPathNodeType.Attribute && matches(context)) { XPathNavigator temp = context.Clone(); if (temp.MoveToParent()) { return qyInput.MatchNode(temp); } } } return null; } public override XPathNodeIterator Clone() { return new AttributeQuery(this); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
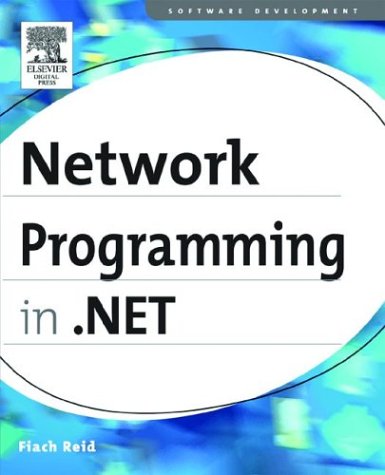
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- URLMembershipCondition.cs
- XmlDataSourceDesigner.cs
- WriteTimeStream.cs
- WMIInterop.cs
- HashHelper.cs
- ZipIOModeEnforcingStream.cs
- WebPartUserCapability.cs
- IconConverter.cs
- XPathExpr.cs
- SiteOfOriginContainer.cs
- Root.cs
- DiscoveryClientRequestChannel.cs
- DataRowView.cs
- WebBrowserSiteBase.cs
- SizeConverter.cs
- ProfessionalColorTable.cs
- DefaultBindingPropertyAttribute.cs
- DrawingCollection.cs
- XmlSchemaAttributeGroupRef.cs
- ConvertEvent.cs
- AgileSafeNativeMemoryHandle.cs
- ListView.cs
- xmlsaver.cs
- Vector3D.cs
- PropertyInfoSet.cs
- ActivityPreviewDesigner.cs
- assemblycache.cs
- SignerInfo.cs
- RegionData.cs
- ScaleTransform3D.cs
- RepeaterItem.cs
- ConfigurationStrings.cs
- CollectionContainer.cs
- dataobject.cs
- PeerContact.cs
- InvokePattern.cs
- MsmqActivation.cs
- IntegerValidatorAttribute.cs
- ClientSideQueueItem.cs
- RowParagraph.cs
- MessageQueueEnumerator.cs
- SerialErrors.cs
- TimeoutValidationAttribute.cs
- _RequestCacheProtocol.cs
- FilterQueryOptionExpression.cs
- OuterGlowBitmapEffect.cs
- DesignerGeometryHelper.cs
- SmtpCommands.cs
- ObjectConverter.cs
- EpmSourceTree.cs
- SingleObjectCollection.cs
- DuplexChannelBinder.cs
- MetaTableHelper.cs
- ExtensionQuery.cs
- ApplicationSecurityInfo.cs
- CollectionTypeElement.cs
- TrustLevelCollection.cs
- DPTypeDescriptorContext.cs
- MatrixAnimationBase.cs
- SimpleMailWebEventProvider.cs
- XmlElementList.cs
- UpdateCommand.cs
- DataGridViewLinkCell.cs
- MailBnfHelper.cs
- HelpInfo.cs
- WindowsListViewGroup.cs
- Vector3DCollectionConverter.cs
- EntityViewGenerationAttribute.cs
- PageThemeParser.cs
- XsltException.cs
- ObjectItemAssemblyLoader.cs
- SynchronizationLockException.cs
- DataBindingCollection.cs
- DataGridCellsPresenter.cs
- XmlSchemaIdentityConstraint.cs
- ApplyTemplatesAction.cs
- WriteFileContext.cs
- ParseHttpDate.cs
- UrlPath.cs
- SessionParameter.cs
- TimeoutStream.cs
- StyleSelector.cs
- ExpressionBindingsDialog.cs
- PathFigure.cs
- XmlElementAttribute.cs
- SingleSelectRootGridEntry.cs
- SchemaTypeEmitter.cs
- HttpConfigurationContext.cs
- PDBReader.cs
- DataGridHelper.cs
- Win32Native.cs
- MobileUserControl.cs
- CircleEase.cs
- VariantWrapper.cs
- ElementMarkupObject.cs
- OperationPickerDialog.designer.cs
- MediaElement.cs
- SimpleBitVector32.cs
- ClientConvert.cs
- DataGridViewCellStyleChangedEventArgs.cs