Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / LinkDescriptor.cs / 4 / LinkDescriptor.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// represents the association between two entities // //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System.Diagnostics; ////// represents the association between two entities /// public sealed class LinkDescriptor : Descriptor { ///equivalence comparer internal static readonly System.Collections.Generic.IEqualityComparerEquivalentComparer = new Equivalent(); /// source entity private readonly object source; ///name of property on source entity that references the target entity private readonly string sourceProperty; ///target entity private readonly object target; ////// constructor /// /// source entity /// name of property on source entity that references the target entity /// target entity /// link state internal LinkDescriptor(object source, string sourceProperty, object target, EntityStates state) : base(state) { this.source = source; this.sourceProperty = sourceProperty; this.target = target; Debug.Assert(null != source, "null != source"); Debug.Assert(!string.IsNullOrEmpty(sourceProperty), "null != sourceProperty"); Debug.Assert(null != target || (EntityStates.Unchanged == state) || (EntityStates.Modified == state) || (EntityStates.Detached == state), "null != target"); Debug.Assert( EntityStates.Added == state || EntityStates.Modified == state || EntityStates.Deleted == state || EntityStates.Unchanged == state || EntityStates.Detached == state, "state"); } ///target entity public object Target { get { return this.target; } } ///source entity public object Source { get { return this.source; } } ///name of property on source entity that references the target entity public string SourceProperty { get { return this.sourceProperty; } } ///equivalence comparer private sealed class Equivalent : System.Collections.Generic.IEqualityComparer{ /// are two LinkDescriptors equivalent, ignore state /// link descriptor x /// link descriptor y ///true if equivalent public bool Equals(LinkDescriptor x, LinkDescriptor y) { return (x.Source == y.Source) && (x.Target == y.Target) && (x.SourceProperty == y.SourceProperty); } ///compute hashcode for LinkDescriptor /// link descriptor ///hashcode public int GetHashCode(LinkDescriptor obj) { return obj.Source.GetHashCode() ^ ((null != obj.Target) ? obj.Target.GetHashCode() : 0) ^ obj.SourceProperty.GetHashCode(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// represents the association between two entities // //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System.Diagnostics; ////// represents the association between two entities /// public sealed class LinkDescriptor : Descriptor { ///equivalence comparer internal static readonly System.Collections.Generic.IEqualityComparerEquivalentComparer = new Equivalent(); /// source entity private readonly object source; ///name of property on source entity that references the target entity private readonly string sourceProperty; ///target entity private readonly object target; ////// constructor /// /// source entity /// name of property on source entity that references the target entity /// target entity /// link state internal LinkDescriptor(object source, string sourceProperty, object target, EntityStates state) : base(state) { this.source = source; this.sourceProperty = sourceProperty; this.target = target; Debug.Assert(null != source, "null != source"); Debug.Assert(!string.IsNullOrEmpty(sourceProperty), "null != sourceProperty"); Debug.Assert(null != target || (EntityStates.Unchanged == state) || (EntityStates.Modified == state) || (EntityStates.Detached == state), "null != target"); Debug.Assert( EntityStates.Added == state || EntityStates.Modified == state || EntityStates.Deleted == state || EntityStates.Unchanged == state || EntityStates.Detached == state, "state"); } ///target entity public object Target { get { return this.target; } } ///source entity public object Source { get { return this.source; } } ///name of property on source entity that references the target entity public string SourceProperty { get { return this.sourceProperty; } } ///equivalence comparer private sealed class Equivalent : System.Collections.Generic.IEqualityComparer{ /// are two LinkDescriptors equivalent, ignore state /// link descriptor x /// link descriptor y ///true if equivalent public bool Equals(LinkDescriptor x, LinkDescriptor y) { return (x.Source == y.Source) && (x.Target == y.Target) && (x.SourceProperty == y.SourceProperty); } ///compute hashcode for LinkDescriptor /// link descriptor ///hashcode public int GetHashCode(LinkDescriptor obj) { return obj.Source.GetHashCode() ^ ((null != obj.Target) ? obj.Target.GetHashCode() : 0) ^ obj.SourceProperty.GetHashCode(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
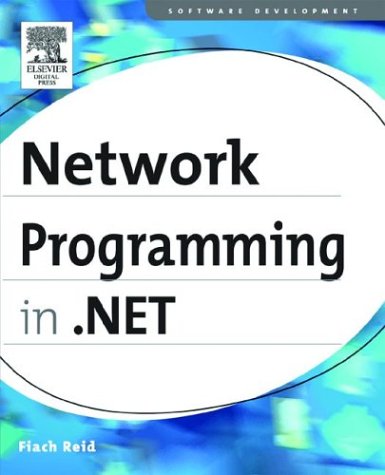
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlDataSourceQueryConverter.cs
- InternalSafeNativeMethods.cs
- TypeProvider.cs
- CodeAttachEventStatement.cs
- TypeDescriptorContext.cs
- AssemblyGen.cs
- OrderedDictionaryStateHelper.cs
- ArcSegment.cs
- SQLDateTimeStorage.cs
- GcHandle.cs
- EmbossBitmapEffect.cs
- MetabaseServerConfig.cs
- DataTableMappingCollection.cs
- MILUtilities.cs
- WpfMemberInvoker.cs
- ExplicitDiscriminatorMap.cs
- SynchronizedInputProviderWrapper.cs
- AttachedPropertyBrowsableAttribute.cs
- UnknownBitmapEncoder.cs
- SQLSingle.cs
- BulletedListEventArgs.cs
- Menu.cs
- StoreItemCollection.cs
- CodeMemberEvent.cs
- DataGridViewCellToolTipTextNeededEventArgs.cs
- DataGridCellInfo.cs
- TypeContext.cs
- UpdateProgress.cs
- TypeToken.cs
- ObjectStateEntryOriginalDbUpdatableDataRecord.cs
- VirtualPathProvider.cs
- DataGridAutomationPeer.cs
- GridErrorDlg.cs
- SoapReflectionImporter.cs
- DropDownButton.cs
- Constants.cs
- DocumentPageView.cs
- UpdatePanel.cs
- PartialList.cs
- WorkingDirectoryEditor.cs
- RemotingConfiguration.cs
- CodeTypeConstructor.cs
- FileUpload.cs
- CqlQuery.cs
- ExtensionSimplifierMarkupObject.cs
- WaitHandleCannotBeOpenedException.cs
- BamlCollectionHolder.cs
- VisualTarget.cs
- AssemblyBuilder.cs
- BufferedStream2.cs
- BackStopAuthenticationModule.cs
- namescope.cs
- Rijndael.cs
- SqlMethods.cs
- FormsIdentity.cs
- recordstatescratchpad.cs
- IpcClientManager.cs
- XmlSchemaExternal.cs
- DataGridViewCellStyle.cs
- LoginDesigner.cs
- RectangleConverter.cs
- parserscommon.cs
- TextClipboardData.cs
- ComplexLine.cs
- _IPv6Address.cs
- UriSectionData.cs
- RSAPKCS1SignatureFormatter.cs
- RegexParser.cs
- NeutralResourcesLanguageAttribute.cs
- SQLInt16.cs
- PixelFormatConverter.cs
- ErrorHandlingReceiver.cs
- DetailsViewActionList.cs
- PrintingPermission.cs
- X509RecipientCertificateClientElement.cs
- Popup.cs
- RelatedView.cs
- ImportStoreException.cs
- WebServiceEnumData.cs
- InheritablePropertyChangeInfo.cs
- ControlPropertyNameConverter.cs
- Stylesheet.cs
- NameScopePropertyAttribute.cs
- AlphaSortedEnumConverter.cs
- FileClassifier.cs
- SqlGatherProducedAliases.cs
- ClientCultureInfo.cs
- ItemsControlAutomationPeer.cs
- RegexWorker.cs
- DataGridViewColumnStateChangedEventArgs.cs
- PageThemeCodeDomTreeGenerator.cs
- HashMembershipCondition.cs
- SizeKeyFrameCollection.cs
- ResourceExpression.cs
- InternalDuplexChannelFactory.cs
- ProviderCollection.cs
- Matrix.cs
- ZipQueryOperator.cs
- GeneralTransform3DGroup.cs
- EventProperty.cs