Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Linq / Parallel / QueryOperators / Inlined / NullableLongAverageAggregationOperator.cs / 1305376 / NullableLongAverageAggregationOperator.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // NullableLongAverageAggregationOperator.cs // //[....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Threading; namespace System.Linq.Parallel { ////// An inlined average aggregation operator and its enumerator, for Nullable longs. /// internal sealed class NullableLongAverageAggregationOperator : InlinedAggregationOperator, double?> { //---------------------------------------------------------------------------------------- // Constructs a new instance of an average associative operator. // internal NullableLongAverageAggregationOperator(IEnumerable child) : base(child) { } //--------------------------------------------------------------------------------------- // Executes the entire query tree, and aggregates the intermediate results into the // final result based on the binary operators and final reduction. // // Return Value: // The single result of aggregation. // protected override double? InternalAggregate(ref Exception singularExceptionToThrow) { // Because the final reduction is typically much cheaper than the intermediate // reductions over the individual partitions, and because each parallel partition // will do a lot of work to produce a single output element, we prefer to turn off // pipelining, and process the final reductions serially. using (IEnumerator > enumerator = GetEnumerator(ParallelMergeOptions.FullyBuffered, true)) { // If the sequence was empty, return null right away. if (!enumerator.MoveNext()) { return null; } Pair result = enumerator.Current; // Simply add together the sums and totals. while (enumerator.MoveNext()) { checked { result.First += enumerator.Current.First; result.Second += enumerator.Current.Second; } } // And divide the sum by the total to obtain the final result. return (double)result.First / result.Second; } } //--------------------------------------------------------------------------------------- // Creates an enumerator that is used internally for the final aggregation step. // protected override QueryOperatorEnumerator , int> CreateEnumerator ( int index, int count, QueryOperatorEnumerator source, object sharedData, CancellationToken cancellationToken) { return new NullableLongAverageAggregationOperatorEnumerator (source, index, cancellationToken); } //--------------------------------------------------------------------------------------- // This enumerator type encapsulates the intermediary aggregation over the underlying // (possibly partitioned) data source. // private class NullableLongAverageAggregationOperatorEnumerator : InlinedAggregationOperatorEnumerator > { private QueryOperatorEnumerator m_source; // The source data. //---------------------------------------------------------------------------------------- // Instantiates a new aggregation operator. // internal NullableLongAverageAggregationOperatorEnumerator(QueryOperatorEnumerator source, int partitionIndex, CancellationToken cancellationToken) : base(partitionIndex, cancellationToken) { Contract.Assert(source != null); m_source = source; } //--------------------------------------------------------------------------------------- // Tallies up the average of the underlying data source, walking the entire thing the first // time MoveNext is called on this object. // protected override bool MoveNextCore(ref Pair currentElement) { // The temporary result contains the running sum and count, respectively. long sum = 0; long count = 0; QueryOperatorEnumerator source = m_source; long? current = default(long?); TKey keyUnused = default(TKey); int i = 0; while (source.MoveNext(ref current, ref keyUnused)) { if ((i++ & CancellationState.POLL_INTERVAL) == 0) CancellationState.ThrowIfCanceled(m_cancellationToken); if (current.HasValue) { sum += current.GetValueOrDefault(); count++; } } currentElement = new Pair (sum, count); return count > 0; } //---------------------------------------------------------------------------------------- // Dispose of resources associated with the underlying enumerator. // protected override void Dispose(bool disposing) { Contract.Assert(m_source != null); m_source.Dispose(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // NullableLongAverageAggregationOperator.cs // // [....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Threading; namespace System.Linq.Parallel { ////// An inlined average aggregation operator and its enumerator, for Nullable longs. /// internal sealed class NullableLongAverageAggregationOperator : InlinedAggregationOperator, double?> { //---------------------------------------------------------------------------------------- // Constructs a new instance of an average associative operator. // internal NullableLongAverageAggregationOperator(IEnumerable child) : base(child) { } //--------------------------------------------------------------------------------------- // Executes the entire query tree, and aggregates the intermediate results into the // final result based on the binary operators and final reduction. // // Return Value: // The single result of aggregation. // protected override double? InternalAggregate(ref Exception singularExceptionToThrow) { // Because the final reduction is typically much cheaper than the intermediate // reductions over the individual partitions, and because each parallel partition // will do a lot of work to produce a single output element, we prefer to turn off // pipelining, and process the final reductions serially. using (IEnumerator > enumerator = GetEnumerator(ParallelMergeOptions.FullyBuffered, true)) { // If the sequence was empty, return null right away. if (!enumerator.MoveNext()) { return null; } Pair result = enumerator.Current; // Simply add together the sums and totals. while (enumerator.MoveNext()) { checked { result.First += enumerator.Current.First; result.Second += enumerator.Current.Second; } } // And divide the sum by the total to obtain the final result. return (double)result.First / result.Second; } } //--------------------------------------------------------------------------------------- // Creates an enumerator that is used internally for the final aggregation step. // protected override QueryOperatorEnumerator , int> CreateEnumerator ( int index, int count, QueryOperatorEnumerator source, object sharedData, CancellationToken cancellationToken) { return new NullableLongAverageAggregationOperatorEnumerator (source, index, cancellationToken); } //--------------------------------------------------------------------------------------- // This enumerator type encapsulates the intermediary aggregation over the underlying // (possibly partitioned) data source. // private class NullableLongAverageAggregationOperatorEnumerator : InlinedAggregationOperatorEnumerator > { private QueryOperatorEnumerator m_source; // The source data. //---------------------------------------------------------------------------------------- // Instantiates a new aggregation operator. // internal NullableLongAverageAggregationOperatorEnumerator(QueryOperatorEnumerator source, int partitionIndex, CancellationToken cancellationToken) : base(partitionIndex, cancellationToken) { Contract.Assert(source != null); m_source = source; } //--------------------------------------------------------------------------------------- // Tallies up the average of the underlying data source, walking the entire thing the first // time MoveNext is called on this object. // protected override bool MoveNextCore(ref Pair currentElement) { // The temporary result contains the running sum and count, respectively. long sum = 0; long count = 0; QueryOperatorEnumerator source = m_source; long? current = default(long?); TKey keyUnused = default(TKey); int i = 0; while (source.MoveNext(ref current, ref keyUnused)) { if ((i++ & CancellationState.POLL_INTERVAL) == 0) CancellationState.ThrowIfCanceled(m_cancellationToken); if (current.HasValue) { sum += current.GetValueOrDefault(); count++; } } currentElement = new Pair (sum, count); return count > 0; } //---------------------------------------------------------------------------------------- // Dispose of resources associated with the underlying enumerator. // protected override void Dispose(bool disposing) { Contract.Assert(m_source != null); m_source.Dispose(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
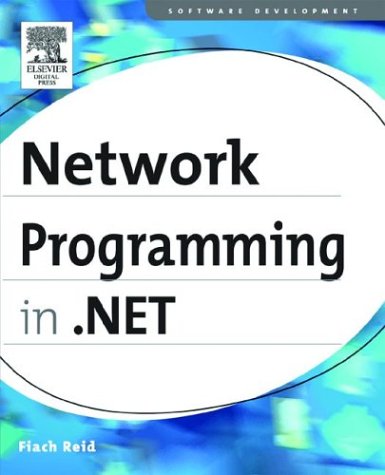
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BooleanAnimationBase.cs
- HwndSubclass.cs
- ActivityScheduledRecord.cs
- SqlClientPermission.cs
- InputReferenceExpression.cs
- ComboBoxDesigner.cs
- SqlRowUpdatedEvent.cs
- SimpleParser.cs
- CacheMode.cs
- SoundPlayerAction.cs
- ResourcesGenerator.cs
- XAMLParseException.cs
- SwitchElementsCollection.cs
- PropertyMetadata.cs
- UTF32Encoding.cs
- ExceptionAggregator.cs
- TextContainerChangedEventArgs.cs
- Double.cs
- StoreAnnotationsMap.cs
- BufferModesCollection.cs
- MdiWindowListStrip.cs
- TextBoxBase.cs
- ThreadLocal.cs
- CachingHintValidation.cs
- FormsAuthenticationModule.cs
- LinqDataSourceContextEventArgs.cs
- ExpressionBindingCollection.cs
- ISAPIApplicationHost.cs
- SiteMapDataSourceView.cs
- DataTableExtensions.cs
- Expander.cs
- GatewayDefinition.cs
- DES.cs
- HttpHandler.cs
- TableCell.cs
- MenuScrollingVisibilityConverter.cs
- WebPartDisplayMode.cs
- RoleManagerModule.cs
- ListGeneralPage.cs
- ListViewInsertEventArgs.cs
- Rect3DConverter.cs
- WindowsFormsDesignerOptionService.cs
- TextEditorLists.cs
- EdmValidator.cs
- BamlBinaryReader.cs
- SettingsPropertyCollection.cs
- DataListItemCollection.cs
- IIS7UserPrincipal.cs
- ExtractedStateEntry.cs
- SetStateEventArgs.cs
- SystemBrushes.cs
- ResourceAttributes.cs
- ConfigurationProperty.cs
- XmlWriterDelegator.cs
- WebControlParameterProxy.cs
- NavigatingCancelEventArgs.cs
- ScrollChrome.cs
- InputBindingCollection.cs
- TypeHelper.cs
- GridViewPageEventArgs.cs
- ValueUnavailableException.cs
- TypeResolver.cs
- DataGridViewAddColumnDialog.cs
- RegionInfo.cs
- SystemException.cs
- XmlQueryTypeFactory.cs
- CanExecuteRoutedEventArgs.cs
- FamilyTypefaceCollection.cs
- SerializationFieldInfo.cs
- SHA384.cs
- QueryOptionExpression.cs
- ListDictionaryInternal.cs
- ConfigXmlReader.cs
- PolicyAssertionCollection.cs
- DataMemberConverter.cs
- COM2Enum.cs
- PropertyValueChangedEvent.cs
- ListMarkerSourceInfo.cs
- TextRunProperties.cs
- TextDecorations.cs
- SimpleHandlerBuildProvider.cs
- SafeUserTokenHandle.cs
- InvalidCastException.cs
- SubMenuStyle.cs
- Message.cs
- XmlMemberMapping.cs
- CodeCompileUnit.cs
- XmlUtf8RawTextWriter.cs
- COMException.cs
- SqlParameterizer.cs
- RolePrincipal.cs
- ObjectSet.cs
- TableLayoutSettingsTypeConverter.cs
- Decorator.cs
- DataGridViewRowHeightInfoPushedEventArgs.cs
- RenderCapability.cs
- CryptoApi.cs
- ColorKeyFrameCollection.cs
- CodeSpit.cs
- ParameterModifier.cs