Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / ComponentModel / COM2Interop / COM2Enum.cs / 1305376 / COM2Enum.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.ComponentModel.Com2Interop { using System.ComponentModel; using System.Diagnostics; using System; using System.Collections; using Microsoft.Win32; using System.Globalization; ////// /// This class mimics a clr enum that we can create at runtime. /// It associates an array of names with an array of values and converts /// between them. /// /// A note here: we compare string values when looking for the value of an item. /// Typically these aren't large lists and the perf is worth it. The reason stems /// from IPerPropertyBrowsing, which supplies a list of names and a list of /// variants to mimic enum functionality. If the actual property value is a DWORD, /// which translates to VT_UI4, and they specify their values as VT_I4 (which is a common /// mistake), they won't compare properly and values can't be updated. /// By comparing strings, we avoid this problem and add flexiblity to the system. /// internal class Com2Enum { ////// /// Our array of value string names /// private string[] names; ////// /// Our values /// private object[] values; ////// /// Our cached array of value.ToString()'s /// private string[] stringValues; ////// /// Should we allow values besides what's in the listbox? /// private bool allowUnknownValues; ////// /// Our one and only ctor /// public Com2Enum(string[] names, object[] values, bool allowUnknownValues) { this.allowUnknownValues = allowUnknownValues; // these have to be null and the same length if (names == null || values == null || names.Length != values.Length) { throw new ArgumentException(SR.GetString(SR.COM2NamesAndValuesNotEqual)); } PopulateArrays(names, values); } ////// /// Can this enum be values other than the strict enum? /// public bool IsStrictEnum { get { return !this.allowUnknownValues; } } ////// /// Retrieve a copy of the value array /// public virtual object[] Values { get { return(object[])this.values.Clone(); } } ////// /// Retrieve a copy of the nme array. /// public virtual string[] Names { get { return(string[])this.names.Clone(); } } ////// /// Associate a string to the appropriate value. /// public virtual object FromString(string s) { int bestMatch = -1; for (int i = 0; i < stringValues.Length; i++) { if (String.Compare(names[i], s, true, CultureInfo.InvariantCulture) == 0 || String.Compare(stringValues[i], s, true, CultureInfo.InvariantCulture) == 0) { return values[i]; } if (bestMatch == -1 && 0 == String.Compare(names[i], s, true, CultureInfo.InvariantCulture)) { bestMatch = i; } } if (bestMatch != -1) { return values[bestMatch]; } return allowUnknownValues ? s : null; } protected virtual void PopulateArrays(string[] names, object[] values) { // setup our values...since we have to walk through // them anyway to do the ToString, we just copy them here. this.names = new string[names.Length]; this.stringValues = new string[names.Length]; this.values = new object[names.Length]; for (int i = 0; i < names.Length; i++) { //Debug.WriteLine(names[i] + ": item " + i.ToString() + ",type=" + values[i].GetType().Name + ", value=" + values[i].ToString()); this.names[i] = names[i]; this.values[i] = values[i]; if (values[i] != null) { this.stringValues[i] = values[i].ToString(); } } } ////// /// Retrieves the string name of a given value. /// public virtual string ToString(object v) { if (v != null) { // in case this is a real enum...try to convert it. // if (values.Length > 0 && v.GetType() != values[0].GetType()) { try { v = Convert.ChangeType(v, values[0].GetType(), CultureInfo.InvariantCulture); } catch{ } } // we have to do this do compensate for small discrpencies // in a lot of objects in COM2 (DWORD -> VT_IU4, value we get is VT_I4, which // convert to Int32, UInt32 respectively string strVal = v.ToString(); for (int i = 0; i < values.Length; i++) { if (String.Compare(stringValues[i], strVal, true, CultureInfo.InvariantCulture) == 0) { return names[i]; } } if (allowUnknownValues) { return strVal; } } return ""; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
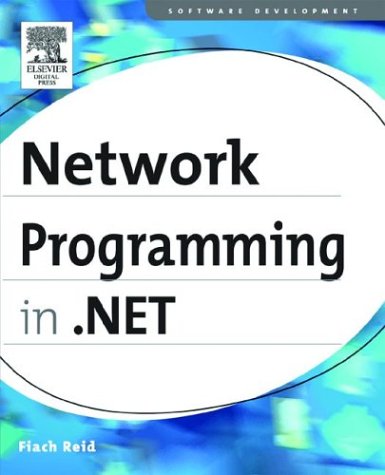
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LinqDataSourceSelectEventArgs.cs
- TerminatorSinks.cs
- SrgsElementList.cs
- DefaultSerializationProviderAttribute.cs
- QilParameter.cs
- WindowPatternIdentifiers.cs
- CurrentTimeZone.cs
- SpeechSynthesizer.cs
- EditorZoneBase.cs
- MissingSatelliteAssemblyException.cs
- DelegatingConfigHost.cs
- CodeMemberEvent.cs
- OuterGlowBitmapEffect.cs
- TextStore.cs
- ControlCollection.cs
- MexHttpsBindingCollectionElement.cs
- WebPartConnectionCollection.cs
- ExtendedProperty.cs
- CreatingCookieEventArgs.cs
- TabControlEvent.cs
- EventRouteFactory.cs
- KeyValuePair.cs
- ProtectedConfiguration.cs
- FontCacheLogic.cs
- TemplatedMailWebEventProvider.cs
- InfiniteIntConverter.cs
- XmlDataCollection.cs
- XsltException.cs
- Scanner.cs
- XPathNodePointer.cs
- jithelpers.cs
- UdpSocket.cs
- RoleManagerSection.cs
- XPathPatternBuilder.cs
- PropertyCondition.cs
- SqlBooleanizer.cs
- ToolStripTextBox.cs
- EventProviderWriter.cs
- ConsoleTraceListener.cs
- MDIClient.cs
- DocumentPageHost.cs
- ResourceExpressionBuilder.cs
- DataGridItemCollection.cs
- EventRouteFactory.cs
- JavaScriptSerializer.cs
- QilParameter.cs
- infer.cs
- DataSourceNameHandler.cs
- CorrelationManager.cs
- SocketElement.cs
- MemberPath.cs
- ImageField.cs
- HtmlAnchor.cs
- UrlParameterReader.cs
- DataGridViewColumnCollection.cs
- ModuleBuilder.cs
- NameTable.cs
- XmlEventCache.cs
- ISessionStateStore.cs
- EditingCoordinator.cs
- util.cs
- EmptyImpersonationContext.cs
- RangeValidator.cs
- Line.cs
- CssClassPropertyAttribute.cs
- ArrayExtension.cs
- CompModSwitches.cs
- WebPartExportVerb.cs
- Environment.cs
- StateDesigner.Layouts.cs
- XmlObjectSerializerReadContextComplexJson.cs
- StringFreezingAttribute.cs
- TextEditorSpelling.cs
- CancelRequestedRecord.cs
- TypeLibConverter.cs
- WebBrowserPermission.cs
- StringWriter.cs
- _ProxyRegBlob.cs
- RpcResponse.cs
- DataGridViewMethods.cs
- NativeMethods.cs
- BrowsableAttribute.cs
- RelationshipFixer.cs
- ConfigurationSection.cs
- DataServiceExpressionVisitor.cs
- DisplayNameAttribute.cs
- FontSource.cs
- GridViewSortEventArgs.cs
- OverlappedContext.cs
- DetailsViewPageEventArgs.cs
- FileSystemEventArgs.cs
- WebPartMovingEventArgs.cs
- CustomBindingCollectionElement.cs
- CompoundFileDeflateTransform.cs
- ListenerPerfCounters.cs
- ContentPlaceHolder.cs
- NamedElement.cs
- ConstNode.cs
- Baml2006ReaderSettings.cs
- FaultCode.cs