Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / FaultCode.cs / 1 / FaultCode.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel { using System.Xml; using System.ServiceModel; using System.ServiceModel.Description; using System.Xml.Schema; using System.Xml.Serialization; using System.Runtime.Serialization; public class FaultCode { FaultCode subCode; string name; string ns; EnvelopeVersion version; public FaultCode(string name) : this(name, "", null) { } public FaultCode(string name, FaultCode subCode) : this(name, "", subCode) { } public FaultCode(string name, string ns) : this(name, ns, null) { } public FaultCode(string name, string ns, FaultCode subCode) { if (name == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("name")); if (name.Length == 0) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("name")); if (!string.IsNullOrEmpty(ns)) NamingHelper.CheckUriParameter(ns, "ns"); this.name = name; this.ns = ns; this.subCode = subCode; if (ns == Message12Strings.Namespace) this.version = EnvelopeVersion.Soap12; else if (ns == Message11Strings.Namespace) this.version = EnvelopeVersion.Soap11; else if (ns == MessageStrings.Namespace) this.version = EnvelopeVersion.None; else this.version = null; } public bool IsPredefinedFault { get { return ns.Length == 0 || version != null; } } public bool IsSenderFault { get { if (IsPredefinedFault) return name == (this.version ?? EnvelopeVersion.Soap12).SenderFaultName; return false; } } public bool IsReceiverFault { get { if (IsPredefinedFault) return name == (this.version ?? EnvelopeVersion.Soap12).ReceiverFaultName; return false; } } public string Namespace { get { return ns; } } public string Name { get { return name; } } public FaultCode SubCode { get { return subCode; } } public static FaultCode CreateSenderFaultCode(FaultCode subCode) { return new FaultCode("Sender", subCode); } public static FaultCode CreateSenderFaultCode(string name, string ns) { return CreateSenderFaultCode(new FaultCode(name, ns)); } public static FaultCode CreateReceiverFaultCode(FaultCode subCode) { if (subCode == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("subCode")); return new FaultCode("Receiver", subCode); } public static FaultCode CreateReceiverFaultCode(string name, string ns) { return CreateReceiverFaultCode(new FaultCode(name, ns)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
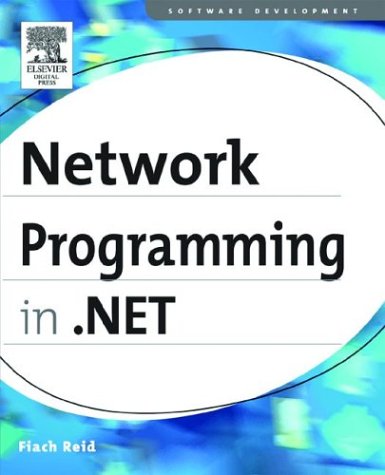
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UnsafeNativeMethods.cs
- EntityClientCacheKey.cs
- EncryptedKey.cs
- IncomingWebRequestContext.cs
- RenderDataDrawingContext.cs
- CodeConditionStatement.cs
- AccessibleObject.cs
- MetricEntry.cs
- CodeTypeOfExpression.cs
- PartitionedStreamMerger.cs
- DataReceivedEventArgs.cs
- DBDataPermissionAttribute.cs
- TemplateControl.cs
- HotSpotCollection.cs
- ToolStripSeparatorRenderEventArgs.cs
- FaultDesigner.cs
- CommandField.cs
- RemoteCryptoSignHashRequest.cs
- ColumnBinding.cs
- DataObjectEventArgs.cs
- FrameworkReadOnlyPropertyMetadata.cs
- MDIClient.cs
- HTMLTextWriter.cs
- AttributeProviderAttribute.cs
- FixedDSBuilder.cs
- EventSourceCreationData.cs
- TextSimpleMarkerProperties.cs
- EdmTypeAttribute.cs
- sitestring.cs
- RadioButton.cs
- CompilerParameters.cs
- TabletDevice.cs
- UnsafeNativeMethodsTablet.cs
- Color.cs
- CatalogZoneBase.cs
- AutoResizedEvent.cs
- ColorMatrix.cs
- autovalidator.cs
- WindowsListViewItemStartMenu.cs
- FormView.cs
- DbConnectionPoolOptions.cs
- DbMetaDataFactory.cs
- SelectionProviderWrapper.cs
- AnnotationHelper.cs
- Misc.cs
- TextTreeRootTextBlock.cs
- HtmlTitle.cs
- ToolStripOverflow.cs
- MailBnfHelper.cs
- TrustLevel.cs
- ContainerFilterService.cs
- RadioButtonStandardAdapter.cs
- FormViewDeleteEventArgs.cs
- Int16AnimationBase.cs
- MimeTypePropertyAttribute.cs
- ProcessHostFactoryHelper.cs
- ChannelListenerBase.cs
- MemoryRecordBuffer.cs
- FilteredDataSetHelper.cs
- NameSpaceExtractor.cs
- DbMetaDataCollectionNames.cs
- _NestedSingleAsyncResult.cs
- SmiEventSink_DeferedProcessing.cs
- CodeIterationStatement.cs
- EncodingDataItem.cs
- DoWorkEventArgs.cs
- InternalTransaction.cs
- Command.cs
- RegexCompilationInfo.cs
- SqlVersion.cs
- UIElementHelper.cs
- XmlAnyElementAttribute.cs
- AspNetHostingPermission.cs
- SoapFormatter.cs
- ListBoxItem.cs
- PageCatalogPartDesigner.cs
- ExpressionBuilderCollection.cs
- ServiceObjectContainer.cs
- Visual3D.cs
- PersonalizationState.cs
- TextDecorationUnitValidation.cs
- WithStatement.cs
- InheritanceContextChangedEventManager.cs
- DbConnectionStringCommon.cs
- Function.cs
- ClonableStack.cs
- ToolboxDataAttribute.cs
- VerticalAlignConverter.cs
- NameNode.cs
- EntityStoreSchemaGenerator.cs
- Label.cs
- WebPartTracker.cs
- EntityObject.cs
- PersonalizationAdministration.cs
- IgnoreSectionHandler.cs
- UpdateRecord.cs
- SecurityTokenTypes.cs
- InstanceDescriptor.cs
- XmlSchemaExporter.cs
- EncodingNLS.cs