Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Controls / ListBoxItem.cs / 1305600 / ListBoxItem.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Utility; using System.ComponentModel; using System.Diagnostics; using System.Windows.Threading; using System.Windows.Automation.Peers; using System.Windows.Media; using System.Windows.Input; using System.Windows.Controls.Primitives; using System.Windows.Shapes; using System; // Disable CS3001: Warning as Error: not CLS-compliant #pragma warning disable 3001 namespace System.Windows.Controls { ////// Control that implements a selectable item inside a ListBox. /// [DefaultEvent("Selected")] public class ListBoxItem : ContentControl { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Default DependencyObject constructor /// public ListBoxItem() : base() { } static ListBoxItem() { DefaultStyleKeyProperty.OverrideMetadata(typeof(ListBoxItem), new FrameworkPropertyMetadata(typeof(ListBoxItem))); _dType = DependencyObjectType.FromSystemTypeInternal(typeof(ListBoxItem)); KeyboardNavigation.DirectionalNavigationProperty.OverrideMetadata(typeof(ListBoxItem), new FrameworkPropertyMetadata(KeyboardNavigationMode.Once)); KeyboardNavigation.TabNavigationProperty.OverrideMetadata(typeof(ListBoxItem), new FrameworkPropertyMetadata(KeyboardNavigationMode.Local)); IsEnabledProperty.OverrideMetadata(typeof(ListBoxItem), new UIPropertyMetadata(new PropertyChangedCallback(OnVisualStatePropertyChanged))); IsMouseOverPropertyKey.OverrideMetadata(typeof(ListBoxItem), new UIPropertyMetadata(new PropertyChangedCallback(OnVisualStatePropertyChanged))); Selector.IsSelectionActivePropertyKey.OverrideMetadata(typeof(ListBoxItem), new FrameworkPropertyMetadata(new PropertyChangedCallback(OnVisualStatePropertyChanged))); } #endregion //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Public Properties ////// Indicates whether this ListBoxItem is selected. /// public static readonly DependencyProperty IsSelectedProperty = Selector.IsSelectedProperty.AddOwner(typeof(ListBoxItem), new FrameworkPropertyMetadata(BooleanBoxes.FalseBox, FrameworkPropertyMetadataOptions.BindsTwoWayByDefault | FrameworkPropertyMetadataOptions.Journal, new PropertyChangedCallback(OnIsSelectedChanged))); ////// Indicates whether this ListBoxItem is selected. /// [Bindable(true), Category("Appearance")] public bool IsSelected { get { return (bool) GetValue(IsSelectedProperty); } set { SetValue(IsSelectedProperty, BooleanBoxes.Box(value)); } } private static void OnIsSelectedChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ListBoxItem listItem = d as ListBoxItem; bool isSelected = (bool) e.NewValue; Selector parentSelector = listItem.ParentSelector; if (parentSelector != null) { parentSelector.RaiseIsSelectedChangedAutomationEvent(listItem, isSelected); } if (isSelected) { listItem.OnSelected(new RoutedEventArgs(Selector.SelectedEvent, listItem)); } else { listItem.OnUnselected(new RoutedEventArgs(Selector.UnselectedEvent, listItem)); } listItem.UpdateVisualState(); } ////// Event indicating that the IsSelected property is now true. /// /// Event arguments protected virtual void OnSelected(RoutedEventArgs e) { HandleIsSelectedChanged(true, e); } ////// Event indicating that the IsSelected property is now false. /// /// Event arguments protected virtual void OnUnselected(RoutedEventArgs e) { HandleIsSelectedChanged(false, e); } private void HandleIsSelectedChanged(bool newValue, RoutedEventArgs e) { RaiseEvent(e); } #endregion #region Events ////// Raised when the item's IsSelected property becomes true. /// public static readonly RoutedEvent SelectedEvent = Selector.SelectedEvent.AddOwner(typeof(ListBoxItem)); ////// Raised when the item's IsSelected property becomes true. /// public event RoutedEventHandler Selected { add { AddHandler(SelectedEvent, value); } remove { RemoveHandler(SelectedEvent, value); } } ////// Raised when the item's IsSelected property becomes false. /// public static readonly RoutedEvent UnselectedEvent = Selector.UnselectedEvent.AddOwner(typeof(ListBoxItem)); ////// Raised when the item's IsSelected property becomes false. /// public event RoutedEventHandler Unselected { add { AddHandler(UnselectedEvent, value); } remove { RemoveHandler(UnselectedEvent, value); } } #endregion //-------------------------------------------------------------------- // // Protected Methods // //-------------------------------------------------------------------- #region Protected Methods internal override void ChangeVisualState(bool useTransitions) { // Change to the correct state in the Interaction group if (!IsEnabled) { // [copied from SL code] // If our child is a control then we depend on it displaying a proper "disabled" state. If it is not a control // (ie TextBlock, Border, etc) then we will use our visuals to show a disabled state. VisualStateManager.GoToState(this, Content is Control ? VisualStates.StateNormal : VisualStates.StateDisabled, useTransitions); } else if (IsMouseOver) { VisualStateManager.GoToState(this, VisualStates.StateMouseOver, useTransitions); } else { VisualStateManager.GoToState(this, VisualStates.StateNormal, useTransitions); } // Change to the correct state in the Selection group if (IsSelected) { if (Selector.GetIsSelectionActive(this)) { VisualStateManager.GoToState(this, VisualStates.StateSelected, useTransitions); } else { VisualStates.GoToState(this, useTransitions, VisualStates.StateSelectedUnfocused, VisualStates.StateSelected); } } else { VisualStateManager.GoToState(this, VisualStates.StateUnselected, useTransitions); } if (IsKeyboardFocused) { VisualStateManager.GoToState(this, VisualStates.StateFocused, useTransitions); } else { VisualStateManager.GoToState(this, VisualStates.StateUnfocused, useTransitions); } base.ChangeVisualState(useTransitions); } ////// Creates AutomationPeer ( protected override AutomationPeer OnCreateAutomationPeer() { return new ListBoxItemWrapperAutomationPeer(this); } ///) /// /// This is the method that responds to the MouseButtonEvent event. /// /// Event arguments protected override void OnMouseLeftButtonDown(MouseButtonEventArgs e) { if (!e.Handled) { // e.Handled = true; HandleMouseButtonDown(MouseButton.Left); } base.OnMouseLeftButtonDown(e); } ////// This is the method that responds to the MouseButtonEvent event. /// /// Event arguments protected override void OnMouseRightButtonDown(MouseButtonEventArgs e) { if (!e.Handled) { // e.Handled = true; HandleMouseButtonDown(MouseButton.Right); } base.OnMouseRightButtonDown(e); } private void HandleMouseButtonDown(MouseButton mouseButton) { if (Selector.UiGetIsSelectable(this) && Focus()) { ListBox parent = ParentListBox; if (parent != null) { parent.NotifyListItemClicked(this, mouseButton); } } } ////// Called when IsMouseOver changes on this element. /// /// protected override void OnMouseEnter(MouseEventArgs e) { // abort any drag operation we have queued. if (parentNotifyDraggedOperation != null) { parentNotifyDraggedOperation.Abort(); parentNotifyDraggedOperation = null; } if (IsMouseOver) { ListBox parent = ParentListBox; if (parent != null && Mouse.LeftButton == MouseButtonState.Pressed) { parent.NotifyListItemMouseDragged(this); } } base.OnMouseEnter(e); } ////// Called when IsMouseOver changes on this element. /// /// protected override void OnMouseLeave(MouseEventArgs e) { // abort any drag operation we have queued. if (parentNotifyDraggedOperation != null) { parentNotifyDraggedOperation.Abort(); parentNotifyDraggedOperation = null; } base.OnMouseLeave(e); } ////// Called when the visual parent of this element changes. /// /// protected internal override void OnVisualParentChanged(DependencyObject oldParent) { ItemsControl oldItemsControl = null; if (VisualTreeHelper.GetParent(this) == null) { if (IsKeyboardFocusWithin) { // This ListBoxItem had focus but was removed from the tree. // The normal behavior is for focus to become null, but we would rather that // focus go to the parent ListBox. // Use the oldParent to get a reference to the ListBox that this ListBoxItem used to be in. // The oldParent's ItemsOwner should be the ListBox. oldItemsControl = ItemsControl.GetItemsOwner(oldParent); } } else { // When the item is added to the tree while grouping, the item will need to // update its IsSelected property. This is currently less optimal than the non-grouped // case where IsSelected is updated directly on the child because each selected // child here will have to search the selected items collection (but usually this // is a small number and will only happen when some style changed and caused everything // to be re-generated). Selector selector = ParentSelector; if (selector != null) { if ((selector.ItemContainerGenerator.GroupStyle != null) && !IsSelected) { object item = selector.ItemContainerGenerator.ItemFromContainer(this); if ((item != null) && selector._selectedItems.Contains(item)) { SetCurrentValueInternal(IsSelectedProperty, true); } } } } base.OnVisualParentChanged(oldParent); // If earlier, we decided to set focus to the old parent ListBox, do it here // after calling base so that the state for IsKeyboardFocusWithin is updated correctly. if (oldItemsControl != null) { oldItemsControl.Focus(); } } #endregion //------------------------------------------------------------------- // // Implementation // //-------------------------------------------------------------------- #region Implementation private ListBox ParentListBox { get { return ParentSelector as ListBox; } } internal Selector ParentSelector { get { return ItemsControl.ItemsControlFromItemContainer(this) as Selector; } } #endregion #if OLD_AUTOMATION // left here for reference only as it is still used by MonthCalendar ////// DependencyProperty for SelectionContainer property. /// internal static readonly DependencyProperty SelectionContainerProperty = DependencyProperty.RegisterAttached("SelectionContainer", typeof(UIElement), typeof(ListBoxItem), new FrameworkPropertyMetadata((UIElement)null)); #endif #region Private Fields DispatcherOperation parentNotifyDraggedOperation = null; #endregion #region DTypeThemeStyleKey // Returns the DependencyObjectType for the registered ThemeStyleKey's default // value. Controls will override this method to return approriate types. internal override DependencyObjectType DTypeThemeStyleKey { get { return _dType; } } private static DependencyObjectType _dType; #endregion DTypeThemeStyleKey } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Utility; using System.ComponentModel; using System.Diagnostics; using System.Windows.Threading; using System.Windows.Automation.Peers; using System.Windows.Media; using System.Windows.Input; using System.Windows.Controls.Primitives; using System.Windows.Shapes; using System; // Disable CS3001: Warning as Error: not CLS-compliant #pragma warning disable 3001 namespace System.Windows.Controls { ////// Control that implements a selectable item inside a ListBox. /// [DefaultEvent("Selected")] public class ListBoxItem : ContentControl { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Default DependencyObject constructor /// public ListBoxItem() : base() { } static ListBoxItem() { DefaultStyleKeyProperty.OverrideMetadata(typeof(ListBoxItem), new FrameworkPropertyMetadata(typeof(ListBoxItem))); _dType = DependencyObjectType.FromSystemTypeInternal(typeof(ListBoxItem)); KeyboardNavigation.DirectionalNavigationProperty.OverrideMetadata(typeof(ListBoxItem), new FrameworkPropertyMetadata(KeyboardNavigationMode.Once)); KeyboardNavigation.TabNavigationProperty.OverrideMetadata(typeof(ListBoxItem), new FrameworkPropertyMetadata(KeyboardNavigationMode.Local)); IsEnabledProperty.OverrideMetadata(typeof(ListBoxItem), new UIPropertyMetadata(new PropertyChangedCallback(OnVisualStatePropertyChanged))); IsMouseOverPropertyKey.OverrideMetadata(typeof(ListBoxItem), new UIPropertyMetadata(new PropertyChangedCallback(OnVisualStatePropertyChanged))); Selector.IsSelectionActivePropertyKey.OverrideMetadata(typeof(ListBoxItem), new FrameworkPropertyMetadata(new PropertyChangedCallback(OnVisualStatePropertyChanged))); } #endregion //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Public Properties ////// Indicates whether this ListBoxItem is selected. /// public static readonly DependencyProperty IsSelectedProperty = Selector.IsSelectedProperty.AddOwner(typeof(ListBoxItem), new FrameworkPropertyMetadata(BooleanBoxes.FalseBox, FrameworkPropertyMetadataOptions.BindsTwoWayByDefault | FrameworkPropertyMetadataOptions.Journal, new PropertyChangedCallback(OnIsSelectedChanged))); ////// Indicates whether this ListBoxItem is selected. /// [Bindable(true), Category("Appearance")] public bool IsSelected { get { return (bool) GetValue(IsSelectedProperty); } set { SetValue(IsSelectedProperty, BooleanBoxes.Box(value)); } } private static void OnIsSelectedChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ListBoxItem listItem = d as ListBoxItem; bool isSelected = (bool) e.NewValue; Selector parentSelector = listItem.ParentSelector; if (parentSelector != null) { parentSelector.RaiseIsSelectedChangedAutomationEvent(listItem, isSelected); } if (isSelected) { listItem.OnSelected(new RoutedEventArgs(Selector.SelectedEvent, listItem)); } else { listItem.OnUnselected(new RoutedEventArgs(Selector.UnselectedEvent, listItem)); } listItem.UpdateVisualState(); } ////// Event indicating that the IsSelected property is now true. /// /// Event arguments protected virtual void OnSelected(RoutedEventArgs e) { HandleIsSelectedChanged(true, e); } ////// Event indicating that the IsSelected property is now false. /// /// Event arguments protected virtual void OnUnselected(RoutedEventArgs e) { HandleIsSelectedChanged(false, e); } private void HandleIsSelectedChanged(bool newValue, RoutedEventArgs e) { RaiseEvent(e); } #endregion #region Events ////// Raised when the item's IsSelected property becomes true. /// public static readonly RoutedEvent SelectedEvent = Selector.SelectedEvent.AddOwner(typeof(ListBoxItem)); ////// Raised when the item's IsSelected property becomes true. /// public event RoutedEventHandler Selected { add { AddHandler(SelectedEvent, value); } remove { RemoveHandler(SelectedEvent, value); } } ////// Raised when the item's IsSelected property becomes false. /// public static readonly RoutedEvent UnselectedEvent = Selector.UnselectedEvent.AddOwner(typeof(ListBoxItem)); ////// Raised when the item's IsSelected property becomes false. /// public event RoutedEventHandler Unselected { add { AddHandler(UnselectedEvent, value); } remove { RemoveHandler(UnselectedEvent, value); } } #endregion //-------------------------------------------------------------------- // // Protected Methods // //-------------------------------------------------------------------- #region Protected Methods internal override void ChangeVisualState(bool useTransitions) { // Change to the correct state in the Interaction group if (!IsEnabled) { // [copied from SL code] // If our child is a control then we depend on it displaying a proper "disabled" state. If it is not a control // (ie TextBlock, Border, etc) then we will use our visuals to show a disabled state. VisualStateManager.GoToState(this, Content is Control ? VisualStates.StateNormal : VisualStates.StateDisabled, useTransitions); } else if (IsMouseOver) { VisualStateManager.GoToState(this, VisualStates.StateMouseOver, useTransitions); } else { VisualStateManager.GoToState(this, VisualStates.StateNormal, useTransitions); } // Change to the correct state in the Selection group if (IsSelected) { if (Selector.GetIsSelectionActive(this)) { VisualStateManager.GoToState(this, VisualStates.StateSelected, useTransitions); } else { VisualStates.GoToState(this, useTransitions, VisualStates.StateSelectedUnfocused, VisualStates.StateSelected); } } else { VisualStateManager.GoToState(this, VisualStates.StateUnselected, useTransitions); } if (IsKeyboardFocused) { VisualStateManager.GoToState(this, VisualStates.StateFocused, useTransitions); } else { VisualStateManager.GoToState(this, VisualStates.StateUnfocused, useTransitions); } base.ChangeVisualState(useTransitions); } ////// Creates AutomationPeer ( protected override AutomationPeer OnCreateAutomationPeer() { return new ListBoxItemWrapperAutomationPeer(this); } ///) /// /// This is the method that responds to the MouseButtonEvent event. /// /// Event arguments protected override void OnMouseLeftButtonDown(MouseButtonEventArgs e) { if (!e.Handled) { // e.Handled = true; HandleMouseButtonDown(MouseButton.Left); } base.OnMouseLeftButtonDown(e); } ////// This is the method that responds to the MouseButtonEvent event. /// /// Event arguments protected override void OnMouseRightButtonDown(MouseButtonEventArgs e) { if (!e.Handled) { // e.Handled = true; HandleMouseButtonDown(MouseButton.Right); } base.OnMouseRightButtonDown(e); } private void HandleMouseButtonDown(MouseButton mouseButton) { if (Selector.UiGetIsSelectable(this) && Focus()) { ListBox parent = ParentListBox; if (parent != null) { parent.NotifyListItemClicked(this, mouseButton); } } } ////// Called when IsMouseOver changes on this element. /// /// protected override void OnMouseEnter(MouseEventArgs e) { // abort any drag operation we have queued. if (parentNotifyDraggedOperation != null) { parentNotifyDraggedOperation.Abort(); parentNotifyDraggedOperation = null; } if (IsMouseOver) { ListBox parent = ParentListBox; if (parent != null && Mouse.LeftButton == MouseButtonState.Pressed) { parent.NotifyListItemMouseDragged(this); } } base.OnMouseEnter(e); } ////// Called when IsMouseOver changes on this element. /// /// protected override void OnMouseLeave(MouseEventArgs e) { // abort any drag operation we have queued. if (parentNotifyDraggedOperation != null) { parentNotifyDraggedOperation.Abort(); parentNotifyDraggedOperation = null; } base.OnMouseLeave(e); } ////// Called when the visual parent of this element changes. /// /// protected internal override void OnVisualParentChanged(DependencyObject oldParent) { ItemsControl oldItemsControl = null; if (VisualTreeHelper.GetParent(this) == null) { if (IsKeyboardFocusWithin) { // This ListBoxItem had focus but was removed from the tree. // The normal behavior is for focus to become null, but we would rather that // focus go to the parent ListBox. // Use the oldParent to get a reference to the ListBox that this ListBoxItem used to be in. // The oldParent's ItemsOwner should be the ListBox. oldItemsControl = ItemsControl.GetItemsOwner(oldParent); } } else { // When the item is added to the tree while grouping, the item will need to // update its IsSelected property. This is currently less optimal than the non-grouped // case where IsSelected is updated directly on the child because each selected // child here will have to search the selected items collection (but usually this // is a small number and will only happen when some style changed and caused everything // to be re-generated). Selector selector = ParentSelector; if (selector != null) { if ((selector.ItemContainerGenerator.GroupStyle != null) && !IsSelected) { object item = selector.ItemContainerGenerator.ItemFromContainer(this); if ((item != null) && selector._selectedItems.Contains(item)) { SetCurrentValueInternal(IsSelectedProperty, true); } } } } base.OnVisualParentChanged(oldParent); // If earlier, we decided to set focus to the old parent ListBox, do it here // after calling base so that the state for IsKeyboardFocusWithin is updated correctly. if (oldItemsControl != null) { oldItemsControl.Focus(); } } #endregion //------------------------------------------------------------------- // // Implementation // //-------------------------------------------------------------------- #region Implementation private ListBox ParentListBox { get { return ParentSelector as ListBox; } } internal Selector ParentSelector { get { return ItemsControl.ItemsControlFromItemContainer(this) as Selector; } } #endregion #if OLD_AUTOMATION // left here for reference only as it is still used by MonthCalendar ////// DependencyProperty for SelectionContainer property. /// internal static readonly DependencyProperty SelectionContainerProperty = DependencyProperty.RegisterAttached("SelectionContainer", typeof(UIElement), typeof(ListBoxItem), new FrameworkPropertyMetadata((UIElement)null)); #endif #region Private Fields DispatcherOperation parentNotifyDraggedOperation = null; #endregion #region DTypeThemeStyleKey // Returns the DependencyObjectType for the registered ThemeStyleKey's default // value. Controls will override this method to return approriate types. internal override DependencyObjectType DTypeThemeStyleKey { get { return _dType; } } private static DependencyObjectType _dType; #endregion DTypeThemeStyleKey } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
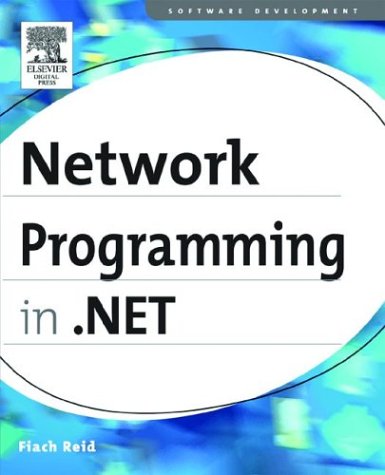
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BordersPage.cs
- XmlSchemaExternal.cs
- _emptywebproxy.cs
- IncrementalReadDecoders.cs
- complextypematerializer.cs
- FlatButtonAppearance.cs
- TraceHandlerErrorFormatter.cs
- MultiBinding.cs
- ComplexType.cs
- SkewTransform.cs
- TdsParserSafeHandles.cs
- AttachedPropertyBrowsableAttribute.cs
- RowUpdatedEventArgs.cs
- ScriptResourceInfo.cs
- CodeSubDirectory.cs
- OptimizedTemplateContent.cs
- Debug.cs
- AttachmentService.cs
- ContentElementAutomationPeer.cs
- WindowsIdentity.cs
- DescriptionAttribute.cs
- processwaithandle.cs
- ObjectDataSourceView.cs
- BatchParser.cs
- DataGridGeneralPage.cs
- IApplicationTrustManager.cs
- Geometry.cs
- ResourceLoader.cs
- ColumnClickEvent.cs
- ManagementEventWatcher.cs
- Image.cs
- UndirectedGraph.cs
- WebPartHeaderCloseVerb.cs
- PublishLicense.cs
- SqlBinder.cs
- ObjectHandle.cs
- CodeIndexerExpression.cs
- WebPartCancelEventArgs.cs
- Oid.cs
- TimeZoneNotFoundException.cs
- FileEnumerator.cs
- ExecutionEngineException.cs
- SqlStatistics.cs
- FaultReason.cs
- Maps.cs
- SignedInfo.cs
- ValidationManager.cs
- Comparer.cs
- mongolianshape.cs
- OleDbEnumerator.cs
- SqlFunctionAttribute.cs
- MaskedTextBoxDesignerActionList.cs
- BackgroundWorker.cs
- AQNBuilder.cs
- WpfWebRequestHelper.cs
- RequestResponse.cs
- Rotation3DAnimationUsingKeyFrames.cs
- MemberHolder.cs
- EventManager.cs
- SessionChannels.cs
- PrinterUnitConvert.cs
- httpstaticobjectscollection.cs
- BamlRecordReader.cs
- SplineKeyFrames.cs
- ClientFormsAuthenticationCredentials.cs
- PageStatePersister.cs
- ConfigXmlComment.cs
- CornerRadius.cs
- ToolStripSeparator.cs
- EventSetter.cs
- DataFieldCollectionEditor.cs
- InternalConfigSettingsFactory.cs
- GiveFeedbackEvent.cs
- Error.cs
- TreeViewImageIndexConverter.cs
- Win32.cs
- CatalogPart.cs
- RadioButtonPopupAdapter.cs
- SamlSubject.cs
- SqlBuffer.cs
- OneWayBindingElement.cs
- WebBrowserHelper.cs
- PropagationProtocolsTracing.cs
- UncommonField.cs
- JsonByteArrayDataContract.cs
- XsdDateTime.cs
- CacheEntry.cs
- TakeQueryOptionExpression.cs
- ObjectDataSourceView.cs
- ContextItem.cs
- TimeSpanSecondsConverter.cs
- InternalEnumValidator.cs
- HwndAppCommandInputProvider.cs
- GroupByQueryOperator.cs
- ProtocolElement.cs
- Sql8ExpressionRewriter.cs
- CacheOutputQuery.cs
- ValueUtilsSmi.cs
- DataServiceResponse.cs
- TripleDESCryptoServiceProvider.cs