Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / DataOracleClient / System / Data / OracleClient / UnsafeNativeMethods.cs / 1 / UnsafeNativeMethods.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Data.Common { using System; using System.Data.OracleClient; using System.Runtime.InteropServices; using System.Runtime.ConstrainedExecution; [ System.Security.SuppressUnmanagedCodeSecurityAttribute() ] sealed internal class UnsafeNativeMethods { private UnsafeNativeMethods() { } // [DllImport(ExternDll.Advapi32, SetLastError=true)] [return:MarshalAs(UnmanagedType.Bool)] static internal extern bool CheckTokenMembership (IntPtr tokenHandle, byte[] sidToCheck, out bool isMember); [DllImport(ExternDll.Advapi32, SetLastError=true)] [return:MarshalAs(UnmanagedType.Bool)] static internal extern bool ConvertSidToStringSidW(IntPtr sid, out IntPtr stringSid); [DllImport(ExternDll.Advapi32, EntryPoint="CreateWellKnownSid", SetLastError=true, CharSet=CharSet.Unicode)] static internal extern int CreateWellKnownSid( int sidType, byte[] domainSid, [Out] byte[] resultSid, ref uint resultSidLength ); [DllImport(ExternDll.Advapi32, SetLastError=true)] [return:MarshalAs(UnmanagedType.Bool)] static internal extern bool GetTokenInformation(IntPtr tokenHandle, uint token_class, IntPtr tokenStruct, uint tokenInformationLength, ref uint tokenString); [DllImport(ExternDll.Advapi32, SetLastError=true)] [return:MarshalAs(UnmanagedType.Bool)] static internal extern bool IsTokenRestricted(IntPtr tokenHandle); [DllImport(ExternDll.Kernel32, CharSet=CharSet.Ansi)] internal static extern int lstrlenA(IntPtr ptr); [DllImport(ExternDll.Kernel32, CharSet=CharSet.Unicode)] internal static extern int lstrlenW(IntPtr ptr); [DllImport(ExternDll.Kernel32)] static internal extern void SetLastError(int dwErrCode); ////////////////////////////// ///// END OF COMMON CODE STUFF ////////////////////////////// [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] [DllImport(ExternDll.OraMtsDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OraMTSEnlCtxGet ( [In, MarshalAs(UnmanagedType.LPArray)] byte[] lpUname, [In, MarshalAs(UnmanagedType.LPArray)] byte[] lpPsswd, [In, MarshalAs(UnmanagedType.LPArray)] byte[] lpDbnam, OciHandle pOCISvc, OciHandle pOCIErr, uint dwFlags, out IntPtr pCtxt // can't return a SafeHandle ); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [DllImport(ExternDll.OraMtsDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OraMTSEnlCtxRel ( IntPtr pCtxt ); [DllImport(ExternDll.OraMtsDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OraMTSOCIErrGet ( ref int dwErr, NativeBuffer lpcEMsg, ref int lpdLen ); [DllImport(ExternDll.OraMtsDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OraMTSJoinTxn ( OciEnlistContext pCtxt, System.Transactions.IDtcTransaction pTrans ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int oermsg ( short rcode, NativeBuffer buf ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIAttrGet ( OciHandle trgthndlp, [In, MarshalAs(UnmanagedType.U4)] OCI.HTYPE trghndltyp, OciHandle attributep, out uint sizep, [In, MarshalAs(UnmanagedType.U4)] OCI.ATTR attrtype, OciHandle errhp ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIAttrGet ( OciHandle trgthndlp, [In, MarshalAs(UnmanagedType.U4)] OCI.HTYPE trghndltyp, out int attributep, out uint sizep, [In, MarshalAs(UnmanagedType.U4)] OCI.ATTR attrtype, OciHandle errhp ); //--------------------------------------------------------------------- // WebData 107422 - This particular overload has some quirks where // in some cases Oracle wants to use the attributep // and sizep args, so we're passing them as REF // instead of OUT. [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIAttrGet ( OciHandle trgthndlp, [In, MarshalAs(UnmanagedType.U4)] OCI.HTYPE trghndltyp, ref IntPtr attributep, // can't return a SafeHandle ref uint sizep, [In, MarshalAs(UnmanagedType.U4)] OCI.ATTR attrtype, OciHandle errhp ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIAttrSet ( OciHandle trgthndlp, [In, MarshalAs(UnmanagedType.U4)] OCI.HTYPE trghndltyp, OciHandle attributep, uint size, [In, MarshalAs(UnmanagedType.U4)] OCI.ATTR attrtype, OciHandle errhp ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIAttrSet ( OciHandle trgthndlp, [In, MarshalAs(UnmanagedType.U4)] OCI.HTYPE trghndltyp, ref int attributep, uint size, [In, MarshalAs(UnmanagedType.U4)] OCI.ATTR attrtype, OciHandle errhp ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIAttrSet ( OciHandle trgthndlp, [In, MarshalAs(UnmanagedType.U4)] OCI.HTYPE trghndltyp, [In, MarshalAs(UnmanagedType.LPArray)] byte[] attributep, uint size, [In, MarshalAs(UnmanagedType.U4)] OCI.ATTR attrtype, OciHandle errhp ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIBindByName ( OciHandle stmtp, out IntPtr bindpp, // can't return a SafeHandle OciHandle errhp, [In, MarshalAs(UnmanagedType.LPArray)] byte[] placeholder, int placeh_len, IntPtr valuep, // must always addref the SafeHandle int value_sz, [In, MarshalAs(UnmanagedType.U2)] OCI.DATATYPE dty, IntPtr indp, // must always addref the SafeHandle IntPtr alenp, // must always addref the SafeHandle IntPtr rcodep, // must always addref the SafeHandle uint maxarr_len, IntPtr curelap, // must always addref the SafeHandle [In, MarshalAs(UnmanagedType.U4)] OCI.MODE mode ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCICharSetToUnicode ( OciHandle hndl, IntPtr dst, // always using pinned memory; IntPtr is OK. uint dstsz, IntPtr src, // always using pinned memory; IntPtr is OK. uint srcsz, out uint size ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIDateTimeFromArray ( OciHandle hndl, OciHandle err, [In, MarshalAs(UnmanagedType.LPArray)] byte[] inarray, uint len, byte type, // OCI.DATATYPE OciHandle datetime, OciHandle reftz, byte fsprec ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIDateTimeToArray ( OciHandle hndl, OciHandle err, OciHandle datetime, OciHandle reftz, [In, MarshalAs(UnmanagedType.LPArray)] byte[] outarray, ref uint len, byte fsprec ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIDateTimeGetTimeZoneOffset ( OciHandle hndl, OciHandle err, OciHandle datetime, out sbyte hour, out sbyte min ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIDefineArrayOfStruct ( OciHandle defnp, OciHandle errhp, uint pvskip, uint indskip, uint rlskip, uint rcskip ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIDefineByPos ( OciHandle stmtp, out IntPtr hndlpp, // can't return a SafeHandle OciHandle errhp, uint position, IntPtr valuep, // must always addref the SafeHandle int value_sz, [In, MarshalAs(UnmanagedType.U2)] OCI.DATATYPE dty, IntPtr indp, // must always addref the SafeHandle IntPtr alenp, // must always addref the SafeHandle IntPtr rcodep, // must always addref the SafeHandle [In, MarshalAs(UnmanagedType.U4)] OCI.MODE mode ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIDefineDynamic ( OciHandle defnp, OciHandle errhp, IntPtr octxp, // always passed as IntPtr.Zero OCI.Callback.OCICallbackDefine ocbfp ); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIDescriptorAlloc ( OciHandle parenth, out IntPtr descp, // can't return a SafeHandle [In, MarshalAs(UnmanagedType.U4)] OCI.HTYPE type, uint xtramem_sz, IntPtr usrmempp // technically, this is an in/out, but we always pass IntPtr.Zero ); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIDescriptorFree ( IntPtr hndlp, [In, MarshalAs(UnmanagedType.U4)] OCI.HTYPE type ); // NOTE: The OciNlsEnvironmentHandle and the OciEnvironmentHandle // classes are SafeHandles, and everything will be pre-jitted becase // the constructors occur inside a CER, and all the methods that the // constructors call have a reliability contract. The upshot of all // that is that this entire method fails with a can't find entry point // OCIEnvNlsCreate if you don't have Oracle92 on the machine. This can // be problematic. If you remove the reliability contracts on the // PInvokes, the problem is solved. [....] and WeiWenLu et al claim // that once the call is made, the out value (envhpp) will be written // to reliability, so the only potential issue is that we may not have // these methods already JITted and you'll get an out of memory situation, // but since this is the first handle for each connection that really // doesn't create a problem -- nothing will be allocated that we need to // free... [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIEnvCreate ( out IntPtr envhpp, // can't return a SafeHandle [In, MarshalAs(UnmanagedType.U4)] OCI.MODE mode, IntPtr ctxp, // context to pass to user-defined function, but we always pass IntPtr.Zero IntPtr malocfp, // pointer to user-defined malloc function, but we always pass IntPtr.Zero IntPtr ralocfp, // pointer to user-defined realloc function, but we always pass IntPtr.Zero IntPtr mfreefp, // pointer to user-defined free function, but we always pass IntPtr.Zero uint xtramemsz, IntPtr usrmempp // technically, this is an in/out, but we always pass IntPtr.Zero ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIEnvNlsCreate ( out IntPtr envhpp, // can't return a SafeHandle [In, MarshalAs(UnmanagedType.U4)] OCI.MODE mode, IntPtr ctxp, // context to pass to user-defined function, but we always pass IntPtr.Zero IntPtr malocfp, // pointer to user-defined malloc function, but we always pass IntPtr.Zero IntPtr ralocfp, // pointer to user-defined realloc function, but we always pass IntPtr.Zero IntPtr mfreefp, // pointer to user-defined free function, but we always pass IntPtr.Zero uint xtramemsz, IntPtr usrmempp, // technically, this is an in/out, but we always pass IntPtr.Zero ushort charset, ushort ncharset ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIErrorGet ( OciHandle hndlp, // The error handle, in most cases, or the environment handle uint recordno, IntPtr sqlstate, // always passed as IntPtr.Zero out int errcodep, NativeBuffer bufp, uint bufsiz, [In, MarshalAs(UnmanagedType.U4)] OCI.HTYPE type ); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIHandleAlloc ( OciHandle parenth, // environment handle out IntPtr hndlpp, // can't return a SafeHandle [In, MarshalAs(UnmanagedType.U4)] OCI.HTYPE type, uint xtramemsz, IntPtr usrmempp // technically, this is an in/out, but we always pass IntPtr.Zero ); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIHandleFree ( IntPtr hndlp, [In, MarshalAs(UnmanagedType.U4)] OCI.HTYPE type ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobAppend ( OciHandle svchp, // service context handle OciHandle errhp, // error handle OciHandle dst_locp, OciHandle src_locp ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobClose ( OciHandle svchp, // service context handle OciHandle errhp, // error handle OciHandle locp ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobCopy ( OciHandle svchp, // service context handle OciHandle errhp, // error handle OciHandle dst_locp, OciHandle src_locp, uint amount, uint dst_offset, uint src_offset ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobCopy2 ( IntPtr svchp, // service context handle IntPtr errhp, // error handle IntPtr dst_locp, IntPtr src_locp, ulong amount, ulong dst_offset, ulong src_offset ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobCreateTemporary ( OciHandle svchp, OciHandle errhp, OciHandle locp, ushort csid, [In, MarshalAs(UnmanagedType.U1)] OCI.CHARSETFORM csfrm, [In, MarshalAs(UnmanagedType.U1)] OCI.LOB_TYPE lobtype, int cache, [In, MarshalAs(UnmanagedType.U2)] OCI.DURATION duration ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobErase ( OciHandle svchp, OciHandle errhp, OciHandle locp, ref uint amount, uint offset ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobFileExists ( OciHandle svchp, OciHandle errhp, OciHandle locp, out int flag ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobFileGetName ( OciHandle envhp, // environment handle. OciHandle errhp, // error handle OciHandle filep, IntPtr dir_alias, // NOTE: must addref the safehandle ref ushort d_length, IntPtr filename, // NOTE: must addref the safehandle ref ushort f_length ); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobFileSetName ( OciHandle envhp, OciHandle errhp, ref IntPtr filep, // can't return a SafeHandle [In, MarshalAs(UnmanagedType.LPArray)] byte[] dir_alias, ushort d_length, [In, MarshalAs(UnmanagedType.LPArray)] byte[] filename, ushort f_length ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobFreeTemporary ( OciHandle svchp, OciHandle errhp, OciHandle locp ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobGetChunkSize ( OciHandle svchp, OciHandle errhp, OciHandle locp, out uint lenp ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobGetLength ( OciHandle svchp, OciHandle errhp, OciHandle locp, out uint lenp ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobIsOpen ( OciHandle svchp, OciHandle errhp, OciHandle locp, out int flag ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobIsTemporary ( OciHandle envhp, OciHandle errhp, OciHandle locp, out int flag ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobLoadFromFile ( OciHandle svchp, OciHandle errhp, OciHandle dst_locp, OciHandle src_locp, uint amount, uint dst_offset, uint src_offset ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobOpen ( OciHandle svchp, OciHandle errhp, OciHandle locp, byte mode ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobRead ( OciHandle svchp, OciHandle errhp, OciHandle locp, ref uint amtp, uint offset, IntPtr bufp, // always using pinned memory, IntPtr is OK uint bufl, IntPtr ctxp, // context passed to callback function, but we always pass IntPtr.Zero IntPtr cbfp, // pointer to callback function to allow piecewise reading, but we always pass IntPtr.Zero ushort csid, [In, MarshalAs(UnmanagedType.U1)] OCI.CHARSETFORM csfrm ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobTrim ( OciHandle svchp, OciHandle errhp, OciHandle locp, uint newlen ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobWrite ( OciHandle svchp, OciHandle errhp, OciHandle locp, ref uint amtp, uint offset, IntPtr bufp, // always using pinned memory, IntPtr is OK uint buflen, byte piece, IntPtr ctxp, // context passed to callback function, but we always pass IntPtr.Zero IntPtr cbfp, // pointer to callback function to allow piecewise writing, but we always pass IntPtr.Zero ushort csid, [In, MarshalAs(UnmanagedType.U1)] OCI.CHARSETFORM csfrm ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberAbs ( OciHandle err, // error handle byte[] number, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberAdd ( OciHandle err, byte[] number1, byte[] number2, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberArcCos ( OciHandle err, byte[] number, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberArcSin ( OciHandle err, byte[] number, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberArcTan ( OciHandle err, byte[] number, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberArcTan2 ( OciHandle err, byte[] number1, byte[] number2, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberCeil ( OciHandle err, byte[] number, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberCmp ( OciHandle err, byte[] number1, byte[] number2, out int result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberCos ( OciHandle err, byte[] number, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberDiv ( OciHandle err, byte[] number1, byte[] number2, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberExp ( OciHandle err, byte[] p, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberFloor ( OciHandle err, byte[] number, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberFromInt ( OciHandle err, ref int inum, uint inum_length, OCI.SIGN inum_s_flag, byte[] number ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberFromInt ( OciHandle err, ref uint inum, uint inum_length, [In, MarshalAs(UnmanagedType.U4)] OCI.SIGN inum_s_flag, byte[] number ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberFromInt ( OciHandle err, ref long inum, uint inum_length, [In, MarshalAs(UnmanagedType.U4)] OCI.SIGN inum_s_flag, byte[] number ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberFromInt ( OciHandle err, ref ulong inum, uint inum_length, [In, MarshalAs(UnmanagedType.U4)] OCI.SIGN inum_s_flag, byte[] number ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberFromReal ( OciHandle err, ref double rnum, uint rnum_length, byte[] number ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl, CharSet=CharSet.Ansi, BestFitMapping=false, ThrowOnUnmappableChar=true)] static internal extern int OCINumberFromText ( OciHandle err, [In, MarshalAs(UnmanagedType.LPStr)] // NOTE: Should be MultiByte, but UnmanagedType doesn't have it, and we don't pass user-data here. string str, uint str_length, [In, MarshalAs(UnmanagedType.LPStr)] // NOTE: Should be MultiByte, but UnmanagedType doesn't have it, and we don't pass user-data here. string fmt, uint fmt_length, IntPtr nls_params, // always passing IntPtr.Zero uint nls_p_length, byte[] number ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberHypCos ( OciHandle err, byte[] number, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberHypSin ( OciHandle err, byte[] number, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberHypTan ( OciHandle err, byte[] number, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberIntPower ( OciHandle err, byte[] baseNumber, int exponent, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberIsInt ( OciHandle err, byte[] number, out int result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberLn ( OciHandle err, byte[] number, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberLog ( OciHandle err, byte[] b, byte[] number, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberMod ( OciHandle err, byte[] number1, byte[] number2, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberMul ( OciHandle err, byte[] number1, byte[] number2, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberNeg ( OciHandle err, byte[] number, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberPower ( OciHandle err, byte[] baseNumber, byte[] exponent, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberRound ( OciHandle err, byte[] number, int decplace, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberShift ( OciHandle err, byte[] baseNumber, int nDig, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberSign ( OciHandle err, byte[] number, out int result ); #if GENERATENUMBERCONSTANTS [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern void OCINumberSetPi ( OciHandle err, byte[] number ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern void OCINumberSetZero ( OciHandle err, byte[] number ); #endif //GENERATENUMBERCONSTANTS [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberSin ( OciHandle err, byte[] number, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberSqrt ( OciHandle err, byte[] number, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberSub ( OciHandle err, byte[] number1, byte[] number2, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberTan ( OciHandle err, byte[] number, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberToInt ( OciHandle err, byte[] number, uint rsl_length, [In, MarshalAs(UnmanagedType.U4)] OCI.SIGN rsl_flag, out int rsl ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberToInt ( OciHandle err, byte[] number, uint rsl_length, [In, MarshalAs(UnmanagedType.U4)] OCI.SIGN rsl_flag, out uint rsl ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberToInt ( OciHandle err, byte[] number, uint rsl_length, [In, MarshalAs(UnmanagedType.U4)] OCI.SIGN rsl_flag, out long rsl ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberToInt ( OciHandle err, byte[] number, uint rsl_length, [In, MarshalAs(UnmanagedType.U4)] OCI.SIGN rsl_flag, out ulong rsl ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberToReal ( OciHandle err, byte[] number, uint rsl_length, out double rsl ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl, CharSet=CharSet.Ansi, BestFitMapping=false, ThrowOnUnmappableChar=true)] static internal extern int OCINumberToText ( OciHandle err, byte[] number, [In, MarshalAs(UnmanagedType.LPStr)] // NOTE: Should be MultiByte, but UnmanagedType doesn't have it, and we don't pass user-data here. string fmt, int fmt_length, IntPtr nls_params, // always passing IntPtr.Zero uint nls_p_length, ref uint buf_size, [In, Out, MarshalAs(UnmanagedType.LPArray)] byte[] buffer ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberTrunc ( OciHandle err, byte[] number, int decplace, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIParamGet ( OciHandle hndlp, [In, MarshalAs(UnmanagedType.U4)] OCI.HTYPE htype, OciHandle errhp, out IntPtr paramdpp, // can't return a SafeHandle uint pos ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIRowidToChar ( OciHandle rowidDesc, NativeBuffer outbfp, ref ushort outbflp, OciHandle errhp ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIServerAttach ( OciHandle srvhp, // server handle OciHandle errhp, [In, MarshalAs(UnmanagedType.LPArray)] byte[] dblink, int dblink_len, [In, MarshalAs(UnmanagedType.U4)] OCI.MODE mode // Must always be OCI_DEFAULT ); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIServerDetach ( IntPtr srvhp, IntPtr errhp, [In, MarshalAs(UnmanagedType.U4)] OCI.MODE mode ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIServerVersion ( OciHandle hndlp, OciHandle errhp, NativeBuffer bufp, uint bufsz, [In, MarshalAs(UnmanagedType.U1)] byte hndltype ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCISessionBegin ( OciHandle svchp, OciHandle errhp, OciHandle usrhp, [In, MarshalAs(UnmanagedType.U4)] OCI.CRED credt, [In, MarshalAs(UnmanagedType.U4)] OCI.MODE mode ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCISessionEnd ( IntPtr svchp, IntPtr errhp, IntPtr usrhp, [In, MarshalAs(UnmanagedType.U4)] OCI.MODE mode ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIStmtExecute ( OciHandle svchp, // service context handle OciHandle stmtp, // statement handle OciHandle errhp, // error handle uint iters, uint rowoff, IntPtr snap_in, // always passing IntPtr.Zero IntPtr snap_out, // always passing IntPtr.Zero [In, MarshalAs(UnmanagedType.U4)] OCI.MODE mode ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIStmtFetch ( OciHandle stmtp, OciHandle errhp, uint nrows, [In, MarshalAs(UnmanagedType.U2)] OCI.FETCH orientation, [In, MarshalAs(UnmanagedType.U4)] OCI.MODE mode ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIStmtPrepare ( OciHandle stmtp, OciHandle errhp, [In, MarshalAs(UnmanagedType.LPArray)] byte[] stmt, uint stmt_len, [In, MarshalAs(UnmanagedType.U4)] OCI.SYNTAX language, [In, MarshalAs(UnmanagedType.U4)] OCI.MODE mode ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCITransCommit ( OciHandle svchp, OciHandle errhp, [In, MarshalAs(UnmanagedType.U4)] OCI.MODE flags ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCITransRollback ( OciHandle svchp, OciHandle errhp, [In, MarshalAs(UnmanagedType.U4)] OCI.MODE flags ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIUnicodeToCharSet ( OciHandle hndl, IntPtr dst, // Using pinned memory; IntPtr is OK. uint dstsz, IntPtr src, // Using pinned memory; IntPtr is OK. uint srcsz, out uint size ); }; } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Data.Common { using System; using System.Data.OracleClient; using System.Runtime.InteropServices; using System.Runtime.ConstrainedExecution; [ System.Security.SuppressUnmanagedCodeSecurityAttribute() ] sealed internal class UnsafeNativeMethods { private UnsafeNativeMethods() { } // [DllImport(ExternDll.Advapi32, SetLastError=true)] [return:MarshalAs(UnmanagedType.Bool)] static internal extern bool CheckTokenMembership (IntPtr tokenHandle, byte[] sidToCheck, out bool isMember); [DllImport(ExternDll.Advapi32, SetLastError=true)] [return:MarshalAs(UnmanagedType.Bool)] static internal extern bool ConvertSidToStringSidW(IntPtr sid, out IntPtr stringSid); [DllImport(ExternDll.Advapi32, EntryPoint="CreateWellKnownSid", SetLastError=true, CharSet=CharSet.Unicode)] static internal extern int CreateWellKnownSid( int sidType, byte[] domainSid, [Out] byte[] resultSid, ref uint resultSidLength ); [DllImport(ExternDll.Advapi32, SetLastError=true)] [return:MarshalAs(UnmanagedType.Bool)] static internal extern bool GetTokenInformation(IntPtr tokenHandle, uint token_class, IntPtr tokenStruct, uint tokenInformationLength, ref uint tokenString); [DllImport(ExternDll.Advapi32, SetLastError=true)] [return:MarshalAs(UnmanagedType.Bool)] static internal extern bool IsTokenRestricted(IntPtr tokenHandle); [DllImport(ExternDll.Kernel32, CharSet=CharSet.Ansi)] internal static extern int lstrlenA(IntPtr ptr); [DllImport(ExternDll.Kernel32, CharSet=CharSet.Unicode)] internal static extern int lstrlenW(IntPtr ptr); [DllImport(ExternDll.Kernel32)] static internal extern void SetLastError(int dwErrCode); ////////////////////////////// ///// END OF COMMON CODE STUFF ////////////////////////////// [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] [DllImport(ExternDll.OraMtsDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OraMTSEnlCtxGet ( [In, MarshalAs(UnmanagedType.LPArray)] byte[] lpUname, [In, MarshalAs(UnmanagedType.LPArray)] byte[] lpPsswd, [In, MarshalAs(UnmanagedType.LPArray)] byte[] lpDbnam, OciHandle pOCISvc, OciHandle pOCIErr, uint dwFlags, out IntPtr pCtxt // can't return a SafeHandle ); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [DllImport(ExternDll.OraMtsDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OraMTSEnlCtxRel ( IntPtr pCtxt ); [DllImport(ExternDll.OraMtsDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OraMTSOCIErrGet ( ref int dwErr, NativeBuffer lpcEMsg, ref int lpdLen ); [DllImport(ExternDll.OraMtsDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OraMTSJoinTxn ( OciEnlistContext pCtxt, System.Transactions.IDtcTransaction pTrans ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int oermsg ( short rcode, NativeBuffer buf ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIAttrGet ( OciHandle trgthndlp, [In, MarshalAs(UnmanagedType.U4)] OCI.HTYPE trghndltyp, OciHandle attributep, out uint sizep, [In, MarshalAs(UnmanagedType.U4)] OCI.ATTR attrtype, OciHandle errhp ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIAttrGet ( OciHandle trgthndlp, [In, MarshalAs(UnmanagedType.U4)] OCI.HTYPE trghndltyp, out int attributep, out uint sizep, [In, MarshalAs(UnmanagedType.U4)] OCI.ATTR attrtype, OciHandle errhp ); //--------------------------------------------------------------------- // WebData 107422 - This particular overload has some quirks where // in some cases Oracle wants to use the attributep // and sizep args, so we're passing them as REF // instead of OUT. [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIAttrGet ( OciHandle trgthndlp, [In, MarshalAs(UnmanagedType.U4)] OCI.HTYPE trghndltyp, ref IntPtr attributep, // can't return a SafeHandle ref uint sizep, [In, MarshalAs(UnmanagedType.U4)] OCI.ATTR attrtype, OciHandle errhp ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIAttrSet ( OciHandle trgthndlp, [In, MarshalAs(UnmanagedType.U4)] OCI.HTYPE trghndltyp, OciHandle attributep, uint size, [In, MarshalAs(UnmanagedType.U4)] OCI.ATTR attrtype, OciHandle errhp ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIAttrSet ( OciHandle trgthndlp, [In, MarshalAs(UnmanagedType.U4)] OCI.HTYPE trghndltyp, ref int attributep, uint size, [In, MarshalAs(UnmanagedType.U4)] OCI.ATTR attrtype, OciHandle errhp ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIAttrSet ( OciHandle trgthndlp, [In, MarshalAs(UnmanagedType.U4)] OCI.HTYPE trghndltyp, [In, MarshalAs(UnmanagedType.LPArray)] byte[] attributep, uint size, [In, MarshalAs(UnmanagedType.U4)] OCI.ATTR attrtype, OciHandle errhp ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIBindByName ( OciHandle stmtp, out IntPtr bindpp, // can't return a SafeHandle OciHandle errhp, [In, MarshalAs(UnmanagedType.LPArray)] byte[] placeholder, int placeh_len, IntPtr valuep, // must always addref the SafeHandle int value_sz, [In, MarshalAs(UnmanagedType.U2)] OCI.DATATYPE dty, IntPtr indp, // must always addref the SafeHandle IntPtr alenp, // must always addref the SafeHandle IntPtr rcodep, // must always addref the SafeHandle uint maxarr_len, IntPtr curelap, // must always addref the SafeHandle [In, MarshalAs(UnmanagedType.U4)] OCI.MODE mode ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCICharSetToUnicode ( OciHandle hndl, IntPtr dst, // always using pinned memory; IntPtr is OK. uint dstsz, IntPtr src, // always using pinned memory; IntPtr is OK. uint srcsz, out uint size ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIDateTimeFromArray ( OciHandle hndl, OciHandle err, [In, MarshalAs(UnmanagedType.LPArray)] byte[] inarray, uint len, byte type, // OCI.DATATYPE OciHandle datetime, OciHandle reftz, byte fsprec ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIDateTimeToArray ( OciHandle hndl, OciHandle err, OciHandle datetime, OciHandle reftz, [In, MarshalAs(UnmanagedType.LPArray)] byte[] outarray, ref uint len, byte fsprec ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIDateTimeGetTimeZoneOffset ( OciHandle hndl, OciHandle err, OciHandle datetime, out sbyte hour, out sbyte min ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIDefineArrayOfStruct ( OciHandle defnp, OciHandle errhp, uint pvskip, uint indskip, uint rlskip, uint rcskip ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIDefineByPos ( OciHandle stmtp, out IntPtr hndlpp, // can't return a SafeHandle OciHandle errhp, uint position, IntPtr valuep, // must always addref the SafeHandle int value_sz, [In, MarshalAs(UnmanagedType.U2)] OCI.DATATYPE dty, IntPtr indp, // must always addref the SafeHandle IntPtr alenp, // must always addref the SafeHandle IntPtr rcodep, // must always addref the SafeHandle [In, MarshalAs(UnmanagedType.U4)] OCI.MODE mode ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIDefineDynamic ( OciHandle defnp, OciHandle errhp, IntPtr octxp, // always passed as IntPtr.Zero OCI.Callback.OCICallbackDefine ocbfp ); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIDescriptorAlloc ( OciHandle parenth, out IntPtr descp, // can't return a SafeHandle [In, MarshalAs(UnmanagedType.U4)] OCI.HTYPE type, uint xtramem_sz, IntPtr usrmempp // technically, this is an in/out, but we always pass IntPtr.Zero ); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIDescriptorFree ( IntPtr hndlp, [In, MarshalAs(UnmanagedType.U4)] OCI.HTYPE type ); // NOTE: The OciNlsEnvironmentHandle and the OciEnvironmentHandle // classes are SafeHandles, and everything will be pre-jitted becase // the constructors occur inside a CER, and all the methods that the // constructors call have a reliability contract. The upshot of all // that is that this entire method fails with a can't find entry point // OCIEnvNlsCreate if you don't have Oracle92 on the machine. This can // be problematic. If you remove the reliability contracts on the // PInvokes, the problem is solved. [....] and WeiWenLu et al claim // that once the call is made, the out value (envhpp) will be written // to reliability, so the only potential issue is that we may not have // these methods already JITted and you'll get an out of memory situation, // but since this is the first handle for each connection that really // doesn't create a problem -- nothing will be allocated that we need to // free... [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIEnvCreate ( out IntPtr envhpp, // can't return a SafeHandle [In, MarshalAs(UnmanagedType.U4)] OCI.MODE mode, IntPtr ctxp, // context to pass to user-defined function, but we always pass IntPtr.Zero IntPtr malocfp, // pointer to user-defined malloc function, but we always pass IntPtr.Zero IntPtr ralocfp, // pointer to user-defined realloc function, but we always pass IntPtr.Zero IntPtr mfreefp, // pointer to user-defined free function, but we always pass IntPtr.Zero uint xtramemsz, IntPtr usrmempp // technically, this is an in/out, but we always pass IntPtr.Zero ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIEnvNlsCreate ( out IntPtr envhpp, // can't return a SafeHandle [In, MarshalAs(UnmanagedType.U4)] OCI.MODE mode, IntPtr ctxp, // context to pass to user-defined function, but we always pass IntPtr.Zero IntPtr malocfp, // pointer to user-defined malloc function, but we always pass IntPtr.Zero IntPtr ralocfp, // pointer to user-defined realloc function, but we always pass IntPtr.Zero IntPtr mfreefp, // pointer to user-defined free function, but we always pass IntPtr.Zero uint xtramemsz, IntPtr usrmempp, // technically, this is an in/out, but we always pass IntPtr.Zero ushort charset, ushort ncharset ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIErrorGet ( OciHandle hndlp, // The error handle, in most cases, or the environment handle uint recordno, IntPtr sqlstate, // always passed as IntPtr.Zero out int errcodep, NativeBuffer bufp, uint bufsiz, [In, MarshalAs(UnmanagedType.U4)] OCI.HTYPE type ); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIHandleAlloc ( OciHandle parenth, // environment handle out IntPtr hndlpp, // can't return a SafeHandle [In, MarshalAs(UnmanagedType.U4)] OCI.HTYPE type, uint xtramemsz, IntPtr usrmempp // technically, this is an in/out, but we always pass IntPtr.Zero ); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIHandleFree ( IntPtr hndlp, [In, MarshalAs(UnmanagedType.U4)] OCI.HTYPE type ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobAppend ( OciHandle svchp, // service context handle OciHandle errhp, // error handle OciHandle dst_locp, OciHandle src_locp ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobClose ( OciHandle svchp, // service context handle OciHandle errhp, // error handle OciHandle locp ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobCopy ( OciHandle svchp, // service context handle OciHandle errhp, // error handle OciHandle dst_locp, OciHandle src_locp, uint amount, uint dst_offset, uint src_offset ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobCopy2 ( IntPtr svchp, // service context handle IntPtr errhp, // error handle IntPtr dst_locp, IntPtr src_locp, ulong amount, ulong dst_offset, ulong src_offset ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobCreateTemporary ( OciHandle svchp, OciHandle errhp, OciHandle locp, ushort csid, [In, MarshalAs(UnmanagedType.U1)] OCI.CHARSETFORM csfrm, [In, MarshalAs(UnmanagedType.U1)] OCI.LOB_TYPE lobtype, int cache, [In, MarshalAs(UnmanagedType.U2)] OCI.DURATION duration ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobErase ( OciHandle svchp, OciHandle errhp, OciHandle locp, ref uint amount, uint offset ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobFileExists ( OciHandle svchp, OciHandle errhp, OciHandle locp, out int flag ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobFileGetName ( OciHandle envhp, // environment handle. OciHandle errhp, // error handle OciHandle filep, IntPtr dir_alias, // NOTE: must addref the safehandle ref ushort d_length, IntPtr filename, // NOTE: must addref the safehandle ref ushort f_length ); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobFileSetName ( OciHandle envhp, OciHandle errhp, ref IntPtr filep, // can't return a SafeHandle [In, MarshalAs(UnmanagedType.LPArray)] byte[] dir_alias, ushort d_length, [In, MarshalAs(UnmanagedType.LPArray)] byte[] filename, ushort f_length ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobFreeTemporary ( OciHandle svchp, OciHandle errhp, OciHandle locp ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobGetChunkSize ( OciHandle svchp, OciHandle errhp, OciHandle locp, out uint lenp ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobGetLength ( OciHandle svchp, OciHandle errhp, OciHandle locp, out uint lenp ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobIsOpen ( OciHandle svchp, OciHandle errhp, OciHandle locp, out int flag ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobIsTemporary ( OciHandle envhp, OciHandle errhp, OciHandle locp, out int flag ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobLoadFromFile ( OciHandle svchp, OciHandle errhp, OciHandle dst_locp, OciHandle src_locp, uint amount, uint dst_offset, uint src_offset ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobOpen ( OciHandle svchp, OciHandle errhp, OciHandle locp, byte mode ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobRead ( OciHandle svchp, OciHandle errhp, OciHandle locp, ref uint amtp, uint offset, IntPtr bufp, // always using pinned memory, IntPtr is OK uint bufl, IntPtr ctxp, // context passed to callback function, but we always pass IntPtr.Zero IntPtr cbfp, // pointer to callback function to allow piecewise reading, but we always pass IntPtr.Zero ushort csid, [In, MarshalAs(UnmanagedType.U1)] OCI.CHARSETFORM csfrm ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobTrim ( OciHandle svchp, OciHandle errhp, OciHandle locp, uint newlen ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCILobWrite ( OciHandle svchp, OciHandle errhp, OciHandle locp, ref uint amtp, uint offset, IntPtr bufp, // always using pinned memory, IntPtr is OK uint buflen, byte piece, IntPtr ctxp, // context passed to callback function, but we always pass IntPtr.Zero IntPtr cbfp, // pointer to callback function to allow piecewise writing, but we always pass IntPtr.Zero ushort csid, [In, MarshalAs(UnmanagedType.U1)] OCI.CHARSETFORM csfrm ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberAbs ( OciHandle err, // error handle byte[] number, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberAdd ( OciHandle err, byte[] number1, byte[] number2, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberArcCos ( OciHandle err, byte[] number, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberArcSin ( OciHandle err, byte[] number, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberArcTan ( OciHandle err, byte[] number, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberArcTan2 ( OciHandle err, byte[] number1, byte[] number2, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberCeil ( OciHandle err, byte[] number, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberCmp ( OciHandle err, byte[] number1, byte[] number2, out int result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberCos ( OciHandle err, byte[] number, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberDiv ( OciHandle err, byte[] number1, byte[] number2, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberExp ( OciHandle err, byte[] p, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberFloor ( OciHandle err, byte[] number, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberFromInt ( OciHandle err, ref int inum, uint inum_length, OCI.SIGN inum_s_flag, byte[] number ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberFromInt ( OciHandle err, ref uint inum, uint inum_length, [In, MarshalAs(UnmanagedType.U4)] OCI.SIGN inum_s_flag, byte[] number ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberFromInt ( OciHandle err, ref long inum, uint inum_length, [In, MarshalAs(UnmanagedType.U4)] OCI.SIGN inum_s_flag, byte[] number ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberFromInt ( OciHandle err, ref ulong inum, uint inum_length, [In, MarshalAs(UnmanagedType.U4)] OCI.SIGN inum_s_flag, byte[] number ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberFromReal ( OciHandle err, ref double rnum, uint rnum_length, byte[] number ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl, CharSet=CharSet.Ansi, BestFitMapping=false, ThrowOnUnmappableChar=true)] static internal extern int OCINumberFromText ( OciHandle err, [In, MarshalAs(UnmanagedType.LPStr)] // NOTE: Should be MultiByte, but UnmanagedType doesn't have it, and we don't pass user-data here. string str, uint str_length, [In, MarshalAs(UnmanagedType.LPStr)] // NOTE: Should be MultiByte, but UnmanagedType doesn't have it, and we don't pass user-data here. string fmt, uint fmt_length, IntPtr nls_params, // always passing IntPtr.Zero uint nls_p_length, byte[] number ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberHypCos ( OciHandle err, byte[] number, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberHypSin ( OciHandle err, byte[] number, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberHypTan ( OciHandle err, byte[] number, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberIntPower ( OciHandle err, byte[] baseNumber, int exponent, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberIsInt ( OciHandle err, byte[] number, out int result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberLn ( OciHandle err, byte[] number, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberLog ( OciHandle err, byte[] b, byte[] number, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberMod ( OciHandle err, byte[] number1, byte[] number2, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberMul ( OciHandle err, byte[] number1, byte[] number2, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberNeg ( OciHandle err, byte[] number, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberPower ( OciHandle err, byte[] baseNumber, byte[] exponent, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberRound ( OciHandle err, byte[] number, int decplace, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberShift ( OciHandle err, byte[] baseNumber, int nDig, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberSign ( OciHandle err, byte[] number, out int result ); #if GENERATENUMBERCONSTANTS [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern void OCINumberSetPi ( OciHandle err, byte[] number ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern void OCINumberSetZero ( OciHandle err, byte[] number ); #endif //GENERATENUMBERCONSTANTS [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberSin ( OciHandle err, byte[] number, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberSqrt ( OciHandle err, byte[] number, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberSub ( OciHandle err, byte[] number1, byte[] number2, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberTan ( OciHandle err, byte[] number, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberToInt ( OciHandle err, byte[] number, uint rsl_length, [In, MarshalAs(UnmanagedType.U4)] OCI.SIGN rsl_flag, out int rsl ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberToInt ( OciHandle err, byte[] number, uint rsl_length, [In, MarshalAs(UnmanagedType.U4)] OCI.SIGN rsl_flag, out uint rsl ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberToInt ( OciHandle err, byte[] number, uint rsl_length, [In, MarshalAs(UnmanagedType.U4)] OCI.SIGN rsl_flag, out long rsl ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberToInt ( OciHandle err, byte[] number, uint rsl_length, [In, MarshalAs(UnmanagedType.U4)] OCI.SIGN rsl_flag, out ulong rsl ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberToReal ( OciHandle err, byte[] number, uint rsl_length, out double rsl ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl, CharSet=CharSet.Ansi, BestFitMapping=false, ThrowOnUnmappableChar=true)] static internal extern int OCINumberToText ( OciHandle err, byte[] number, [In, MarshalAs(UnmanagedType.LPStr)] // NOTE: Should be MultiByte, but UnmanagedType doesn't have it, and we don't pass user-data here. string fmt, int fmt_length, IntPtr nls_params, // always passing IntPtr.Zero uint nls_p_length, ref uint buf_size, [In, Out, MarshalAs(UnmanagedType.LPArray)] byte[] buffer ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCINumberTrunc ( OciHandle err, byte[] number, int decplace, byte[] result ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIParamGet ( OciHandle hndlp, [In, MarshalAs(UnmanagedType.U4)] OCI.HTYPE htype, OciHandle errhp, out IntPtr paramdpp, // can't return a SafeHandle uint pos ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIRowidToChar ( OciHandle rowidDesc, NativeBuffer outbfp, ref ushort outbflp, OciHandle errhp ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIServerAttach ( OciHandle srvhp, // server handle OciHandle errhp, [In, MarshalAs(UnmanagedType.LPArray)] byte[] dblink, int dblink_len, [In, MarshalAs(UnmanagedType.U4)] OCI.MODE mode // Must always be OCI_DEFAULT ); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIServerDetach ( IntPtr srvhp, IntPtr errhp, [In, MarshalAs(UnmanagedType.U4)] OCI.MODE mode ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIServerVersion ( OciHandle hndlp, OciHandle errhp, NativeBuffer bufp, uint bufsz, [In, MarshalAs(UnmanagedType.U1)] byte hndltype ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCISessionBegin ( OciHandle svchp, OciHandle errhp, OciHandle usrhp, [In, MarshalAs(UnmanagedType.U4)] OCI.CRED credt, [In, MarshalAs(UnmanagedType.U4)] OCI.MODE mode ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCISessionEnd ( IntPtr svchp, IntPtr errhp, IntPtr usrhp, [In, MarshalAs(UnmanagedType.U4)] OCI.MODE mode ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIStmtExecute ( OciHandle svchp, // service context handle OciHandle stmtp, // statement handle OciHandle errhp, // error handle uint iters, uint rowoff, IntPtr snap_in, // always passing IntPtr.Zero IntPtr snap_out, // always passing IntPtr.Zero [In, MarshalAs(UnmanagedType.U4)] OCI.MODE mode ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIStmtFetch ( OciHandle stmtp, OciHandle errhp, uint nrows, [In, MarshalAs(UnmanagedType.U2)] OCI.FETCH orientation, [In, MarshalAs(UnmanagedType.U4)] OCI.MODE mode ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIStmtPrepare ( OciHandle stmtp, OciHandle errhp, [In, MarshalAs(UnmanagedType.LPArray)] byte[] stmt, uint stmt_len, [In, MarshalAs(UnmanagedType.U4)] OCI.SYNTAX language, [In, MarshalAs(UnmanagedType.U4)] OCI.MODE mode ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCITransCommit ( OciHandle svchp, OciHandle errhp, [In, MarshalAs(UnmanagedType.U4)] OCI.MODE flags ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCITransRollback ( OciHandle svchp, OciHandle errhp, [In, MarshalAs(UnmanagedType.U4)] OCI.MODE flags ); [DllImport(ExternDll.OciDll, CallingConvention=CallingConvention.Cdecl)] static internal extern int OCIUnicodeToCharSet ( OciHandle hndl, IntPtr dst, // Using pinned memory; IntPtr is OK. uint dstsz, IntPtr src, // Using pinned memory; IntPtr is OK. uint srcsz, out uint size ); }; } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
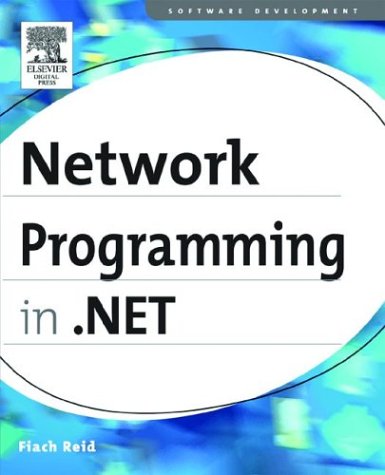
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WorkflowService.cs
- ClientSettingsProvider.cs
- TypeDelegator.cs
- SystemIPv4InterfaceProperties.cs
- Type.cs
- OleDbPermission.cs
- CodeAttachEventStatement.cs
- KeyPullup.cs
- GridViewRow.cs
- ProxyWebPartConnectionCollection.cs
- SafeNativeMethods.cs
- RelatedEnd.cs
- TypeSource.cs
- ConfigurationManagerInternal.cs
- FlowDocumentPaginator.cs
- WebCategoryAttribute.cs
- OleDbParameter.cs
- WmfPlaceableFileHeader.cs
- ScopelessEnumAttribute.cs
- DataGridItemCollection.cs
- DefaultHttpHandler.cs
- TablePatternIdentifiers.cs
- DiagnosticTrace.cs
- RbTree.cs
- XmlSchema.cs
- WebPartZoneCollection.cs
- LockedAssemblyCache.cs
- ComboBoxAutomationPeer.cs
- CompilerResults.cs
- SafeRightsManagementSessionHandle.cs
- GradientSpreadMethodValidation.cs
- TextTreeTextBlock.cs
- OleDbDataReader.cs
- ColumnMapTranslator.cs
- PreloadedPackages.cs
- Cursor.cs
- Point3DValueSerializer.cs
- GenericUriParser.cs
- SafeNativeMethods.cs
- ComponentDispatcher.cs
- CustomAttributeFormatException.cs
- UrlMappingsSection.cs
- LocationSectionRecord.cs
- ProxyHwnd.cs
- Cursors.cs
- CommandBindingCollection.cs
- DetailsViewPagerRow.cs
- QuotaThrottle.cs
- ComponentSerializationService.cs
- AssociationSetEnd.cs
- XmlIlGenerator.cs
- CacheDependency.cs
- ListItemViewControl.cs
- AppDomainShutdownMonitor.cs
- ButtonBase.cs
- PhonemeConverter.cs
- RangeValidator.cs
- SelectionProcessor.cs
- TypeSchema.cs
- GraphicsContext.cs
- ProtocolsSection.cs
- HelloMessageApril2005.cs
- StringReader.cs
- GlobalItem.cs
- VariableQuery.cs
- ExecutionProperties.cs
- BinaryWriter.cs
- SelfIssuedAuthAsymmetricKey.cs
- OrderablePartitioner.cs
- AddInPipelineAttributes.cs
- DragDeltaEventArgs.cs
- XmlDataSource.cs
- NonBatchDirectoryCompiler.cs
- _DomainName.cs
- XmlSubtreeReader.cs
- ConfigurationSectionGroup.cs
- AudioFormatConverter.cs
- Comparer.cs
- EventSourceCreationData.cs
- StyleCollection.cs
- SuppressMessageAttribute.cs
- sortedlist.cs
- DataSourceComponent.cs
- XmlAttributes.cs
- RotateTransform3D.cs
- CacheModeConverter.cs
- BounceEase.cs
- VariableModifiersHelper.cs
- XmlReflectionImporter.cs
- TraceSection.cs
- EntityObject.cs
- ZoneLinkButton.cs
- RequestCachePolicyConverter.cs
- DetailsViewUpdateEventArgs.cs
- ToolStripPanelSelectionGlyph.cs
- MediaTimeline.cs
- ServerProtocol.cs
- NotifyCollectionChangedEventArgs.cs
- ListViewDataItem.cs
- HttpStaticObjectsCollectionBase.cs