Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Generated / UIElement.cs / 1 / UIElement.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.ComponentModel; using System.Diagnostics; using System.Security; using System.Security.Permissions; using System.Windows.Input; using System.Windows.Media.Animation; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings namespace System.Windows { partial class UIElement : IAnimatable { static private readonly Type _typeofThis = typeof(UIElement); #region IAnimatable ////// Applies an AnimationClock to a DepencencyProperty which will /// replace the current animations on the property using the snapshot /// and replace HandoffBehavior. /// /// /// The DependencyProperty to animate. /// /// /// The AnimationClock that will animate the property. If this is null /// then all animations will be removed from the property. /// public void ApplyAnimationClock( DependencyProperty dp, AnimationClock clock) { ApplyAnimationClock(dp, clock, HandoffBehavior.SnapshotAndReplace); } ////// Applies an AnimationClock to a DependencyProperty. The effect of /// the new AnimationClock on any current animations will be determined by /// the value of the handoffBehavior parameter. /// /// /// The DependencyProperty to animate. /// /// /// The AnimationClock that will animate the property. If parameter is null /// then animations will be removed from the property if handoffBehavior is /// SnapshotAndReplace; otherwise the method call will have no result. /// /// /// Determines how the new AnimationClock will transition from or /// affect any current animations on the property. /// public void ApplyAnimationClock( DependencyProperty dp, AnimationClock clock, HandoffBehavior handoffBehavior) { if (dp == null) { throw new ArgumentNullException("dp"); } if (!AnimationStorage.IsPropertyAnimatable(this, dp)) { #pragma warning disable 56506 // Suppress presharp warning: Parameter 'dp' to this public method must be validated: A null-dereference can occur here. throw new ArgumentException(SR.Get(SRID.Animation_DependencyPropertyIsNotAnimatable, dp.Name, this.GetType()), "dp"); #pragma warning restore 56506 } if (clock != null && !AnimationStorage.IsAnimationValid(dp, clock.Timeline)) { #pragma warning disable 56506 // Suppress presharp warning: Parameter 'dp' to this public method must be validated: A null-dereference can occur here. throw new ArgumentException(SR.Get(SRID.Animation_AnimationTimelineTypeMismatch, clock.Timeline.GetType(), dp.Name, dp.PropertyType), "clock"); #pragma warning restore 56506 } if (!HandoffBehaviorEnum.IsDefined(handoffBehavior)) { throw new ArgumentException(SR.Get(SRID.Animation_UnrecognizedHandoffBehavior)); } if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.IAnimatable_CantAnimateSealedDO, dp, this.GetType())); } AnimationStorage.ApplyAnimationClock(this, dp, clock, handoffBehavior); } ////// Starts an animation for a DependencyProperty. The animation will /// begin when the next frame is rendered. /// /// /// The DependencyProperty to animate. /// /// ///The AnimationTimeline to used to animate the property. ///If the AnimationTimeline's BeginTime is null, any current animations /// will be removed and the current value of the property will be held. ///If this value is null, all animations will be removed from the property /// and the property value will revert back to its base value. /// public void BeginAnimation(DependencyProperty dp, AnimationTimeline animation) { BeginAnimation(dp, animation, HandoffBehavior.SnapshotAndReplace); } ////// Starts an animation for a DependencyProperty. The animation will /// begin when the next frame is rendered. /// /// /// The DependencyProperty to animate. /// /// ///The AnimationTimeline to used to animate the property. ///If the AnimationTimeline's BeginTime is null, any current animations /// will be removed and the current value of the property will be held. ///If this value is null, all animations will be removed from the property /// and the property value will revert back to its base value. /// /// /// Specifies how the new animation should interact with any current /// animations already affecting the property value. /// public void BeginAnimation(DependencyProperty dp, AnimationTimeline animation, HandoffBehavior handoffBehavior) { if (dp == null) { throw new ArgumentNullException("dp"); } if (!AnimationStorage.IsPropertyAnimatable(this, dp)) { #pragma warning disable 56506 // Suppress presharp warning: Parameter 'dp' to this public method must be validated: A null-dereference can occur here. throw new ArgumentException(SR.Get(SRID.Animation_DependencyPropertyIsNotAnimatable, dp.Name, this.GetType()), "dp"); #pragma warning restore 56506 } if ( animation != null && !AnimationStorage.IsAnimationValid(dp, animation)) { throw new ArgumentException(SR.Get(SRID.Animation_AnimationTimelineTypeMismatch, animation.GetType(), dp.Name, dp.PropertyType), "animation"); } if (!HandoffBehaviorEnum.IsDefined(handoffBehavior)) { throw new ArgumentException(SR.Get(SRID.Animation_UnrecognizedHandoffBehavior)); } if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.IAnimatable_CantAnimateSealedDO, dp, this.GetType())); } AnimationStorage.BeginAnimation(this, dp, animation, handoffBehavior); } ////// Returns true if any properties on this DependencyObject have a /// persistent animation or the object has one or more clocks associated /// with any of its properties. /// public bool HasAnimatedProperties { get { VerifyAccess(); return IAnimatable_HasAnimatedProperties; } } ////// If the dependency property is animated this method will /// give you the value as if it was not animated. /// /// The DependencyProperty ////// The value that would be returned if there were no /// animations attached. If there aren't any attached, then /// the result will be the same as that returned from /// GetValue. /// public object GetAnimationBaseValue(DependencyProperty dp) { if (dp == null) { throw new ArgumentNullException("dp"); } return this.GetValueEntry( LookupEntry(dp.GlobalIndex), dp, null, RequestFlags.AnimationBaseValue).Value; } #endregion IAnimatable #region Animation ////// Allows subclasses to participate in property animated value computation /// /// /// /// EffectiveValueEntry computed by base ////// Putting an InheritanceDemand as a defense-in-depth measure, /// as this provides a hook to the property system that we don't /// want exposed under PartialTrust. /// [UIPermissionAttribute(SecurityAction.InheritanceDemand, Window=UIPermissionWindow.AllWindows)] internal sealed override void EvaluateAnimatedValueCore( DependencyProperty dp, PropertyMetadata metadata, ref EffectiveValueEntry entry) { if (IAnimatable_HasAnimatedProperties) { AnimationStorage storage = AnimationStorage.GetStorage(this, dp); if (storage != null) { object value = entry.GetFlattenedEntry(RequestFlags.FullyResolved).Value; if (entry.IsDeferredReference) { DeferredReference dr = (DeferredReference)value; value = dr.GetValue(entry.BaseValueSourceInternal); // Set the baseValue back into the entry and clear the // IsDeferredReference flag entry.SetAnimationBaseValue(value); entry.IsDeferredReference = false; } object animatedValue = AnimationStorage.GetCurrentPropertyValue(storage, this, dp, metadata, value); entry.SetAnimatedValue(animatedValue, value); } } } #endregion Animation #region Commands ////// Instance level InputBinding collection, initialized on first use. /// To have commands handled (QueryEnabled/Execute) on an element instance, /// the user of this method can add InputBinding with handlers thru this /// method. /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public InputBindingCollection InputBindings { get { VerifyAccess(); InputBindingCollection bindings = InputBindingCollectionField.GetValue(this); if (bindings == null) { bindings = new InputBindingCollection(); InputBindingCollectionField.SetValue(this, bindings); } return bindings; } } // Used by CommandManager to avoid instantiating an empty collection internal InputBindingCollection InputBindingsInternal { get { VerifyAccess(); return InputBindingCollectionField.GetValue(this); } } ////// This method is used by TypeDescriptor to determine if this property should /// be serialized. /// // for serializer to serialize only when InputBindings is not empty [EditorBrowsable(EditorBrowsableState.Never)] public bool ShouldSerializeInputBindings() { InputBindingCollection bindingCollection = InputBindingCollectionField.GetValue(this); if (bindingCollection != null && bindingCollection.Count > 0) { return true; } return false; } ////// Instance level CommandBinding collection, initialized on first use. /// To have commands handled (QueryEnabled/Execute) on an element instance, /// the user of this method can add CommandBinding with handlers thru this /// method. /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public CommandBindingCollection CommandBindings { get { VerifyAccess(); CommandBindingCollection bindings = CommandBindingCollectionField.GetValue(this); if (bindings == null) { bindings = new CommandBindingCollection(); CommandBindingCollectionField.SetValue(this, bindings); } return bindings; } } // Used by CommandManager to avoid instantiating an empty collection internal CommandBindingCollection CommandBindingsInternal { get { VerifyAccess(); return CommandBindingCollectionField.GetValue(this); } } ////// This method is used by TypeDescriptor to determine if this property should /// be serialized. /// // for serializer to serialize only when CommandBindings is not empty [EditorBrowsable(EditorBrowsableState.Never)] public bool ShouldSerializeCommandBindings() { CommandBindingCollection bindingCollection = CommandBindingCollectionField.GetValue(this); if (bindingCollection != null && bindingCollection.Count > 0) { return true; } return false; } #endregion Commands #region Events ////// Allows UIElement to augment the /// ////// /// Sub-classes of UIElement can override /// this method to custom augment the route /// /// /// Theto be /// augmented /// /// /// for the /// RoutedEvent to be raised post building /// the route /// /// /// Whether or not the route should continue past the visual tree. /// If this is true, and there are no more visual parents, the route /// building code will call the GetUIParentCore method to find the /// next non-visual parent. /// internal virtual bool BuildRouteCore(EventRoute route, RoutedEventArgs args) { return false; } ////// Builds the /// /// The/// being /// built /// /// /// for the /// RoutedEvent to be raised post building /// the route /// internal void BuildRoute(EventRoute route, RoutedEventArgs args) { UIElement.BuildRouteHelper(this, route, args); } /// /// Raise the events specified by /// ////// /// This method is a shorthand for /// /// ///and /// /// for the event to /// be raised /// /// /// By default clears the user initiated bit. /// To guard against "replay" attacks. /// public void RaiseEvent(RoutedEventArgs e) { // VerifyAccess(); if (e == null) { throw new ArgumentNullException("e"); } e.ClearUserInitiated(); UIElement.RaiseEventImpl(this, e); } ////// "Trusted" internal flavor of RaiseEvent. /// Used to set the User-initated RaiseEvent. /// ////// Critical - sets the MarkAsUserInitiated bit. /// [SecurityCritical] internal void RaiseEvent(RoutedEventArgs args, bool trusted) { if (args == null) { throw new ArgumentNullException("args"); } if ( trusted ) { args.MarkAsUserInitiated(); } else { args.ClearUserInitiated(); } // Try/finally to ensure that UserInitiated bit is cleared. try { UIElement.RaiseEventImpl(this, args); } finally { // Clear the bit - just to guarantee it's not used again args.ClearUserInitiated(); } } ////// Allows adjustment to the event source /// ////// Subclasses must override this method /// to be able to adjust the source during /// route invocation /// /// Routed Event Args /// ////// /// NOTE: Expected to return null when no /// change is made to source /// /// Returns new source /// internal virtual object AdjustEventSource(RoutedEventArgs args) { return null; } ////// See overloaded method for details /// ////// handledEventsToo defaults to false /// /// public void AddHandler(RoutedEvent routedEvent, Delegate handler) { // HandledEventToo defaults to false // Call forwarded AddHandler(routedEvent, handler, false); } ////// See overloaded method for details /// /// Adds a routed event handler for the particular /// ////// /// The handler added thus is also known as /// an instance handler /// ////// /// /// NOTE: It is not an error to add a handler twice /// (handler will simply be called twice) /// /// /// Input parameters /// and handler cannot be null /// handledEventsToo input parameter when false means /// that listener does not care about already handled events. /// Hence the handler will not be invoked on the target if /// the RoutedEvent has already been /// /// handledEventsToo input parameter when true means /// that the listener wants to hear about all events even if /// they have already been handled. Hence the handler will /// be invoked irrespective of the event being /// /// for which the handler /// is attached /// /// /// The handler that will be invoked on this object /// when the RoutedEvent is raised /// /// /// Flag indicating whether or not the listener wants to /// hear about events that have already been handled /// public void AddHandler( RoutedEvent routedEvent, Delegate handler, bool handledEventsToo) { // VerifyAccess(); if (routedEvent == null) { throw new ArgumentNullException("routedEvent"); } if (handler == null) { throw new ArgumentNullException("handler"); } if (!routedEvent.IsLegalHandler(handler)) { throw new ArgumentException(SR.Get(SRID.HandlerTypeIllegal)); } EnsureEventHandlersStore(); EventHandlersStore.AddRoutedEventHandler(routedEvent, handler, handledEventsToo); OnAddHandler (routedEvent, handler); } /// /// Notifies subclass of a new routed event handler. Note that this is /// called once for each handler added, but OnRemoveHandler is only called /// on the last removal. /// internal virtual void OnAddHandler( RoutedEvent routedEvent, Delegate handler) { } ////// Removes all instances of the specified routed /// event handler for this object instance /// ////// The handler removed thus is also known as /// an instance handler /// ////// /// /// NOTE: This method does nothing if there were /// no handlers registered with the matching /// criteria /// /// /// Input parameters /// and handler cannot be null /// This method ignores the handledEventsToo criterion /// for which the handler /// is attached /// /// /// The handler for this object instance to be removed /// public void RemoveHandler(RoutedEvent routedEvent, Delegate handler) { // VerifyAccess(); if (routedEvent == null) { throw new ArgumentNullException("routedEvent"); } if (handler == null) { throw new ArgumentNullException("handler"); } if (!routedEvent.IsLegalHandler(handler)) { throw new ArgumentException(SR.Get(SRID.HandlerTypeIllegal)); } EventHandlersStore store = EventHandlersStore; if (store != null) { store.RemoveRoutedEventHandler(routedEvent, handler); OnRemoveHandler (routedEvent, handler); if (store.Count == 0) { // last event handler was removed -- throw away underlying EventHandlersStore EventHandlersStoreField.ClearValue(this); WriteFlag(CoreFlags.ExistsEventHandlersStore, false); } } } /// /// Notifies subclass of an event for which a handler has been removed. /// internal virtual void OnRemoveHandler( RoutedEvent routedEvent, Delegate handler) { } private void EventHandlersStoreAdd(EventPrivateKey key, Delegate handler) { EnsureEventHandlersStore(); EventHandlersStore.Add(key, handler); } private void EventHandlersStoreRemove(EventPrivateKey key, Delegate handler) { EventHandlersStore store = EventHandlersStore; if (store != null) { store.Remove(key, handler); if (store.Count == 0) { // last event handler was removed -- throw away underlying EventHandlersStore EventHandlersStoreField.ClearValue(this); WriteFlag(CoreFlags.ExistsEventHandlersStore, false); } } } ////// Add the event handlers for this element to the route. /// public void AddToEventRoute(EventRoute route, RoutedEventArgs e) { if (route == null) { throw new ArgumentNullException("route"); } if (e == null) { throw new ArgumentNullException("e"); } // Get class listeners for this UIElement RoutedEventHandlerInfoList classListeners = GlobalEventManager.GetDTypedClassListeners(this.DependencyObjectType, e.RoutedEvent); // Add all class listeners for this UIElement while (classListeners != null) { for(int i = 0; i < classListeners.Handlers.Length; i++) { route.Add(this, classListeners.Handlers[i].Handler, classListeners.Handlers[i].InvokeHandledEventsToo); } classListeners = classListeners.Next; } // Get instance listeners for this UIElement FrugalObjectListinstanceListeners = null; EventHandlersStore store = EventHandlersStore; if (store != null) { instanceListeners = store[e.RoutedEvent]; // Add all instance listeners for this UIElement if (instanceListeners != null) { for (int i = 0; i < instanceListeners.Count; i++) { route.Add(this, instanceListeners[i].Handler, instanceListeners[i].InvokeHandledEventsToo); } } } // Allow Framework to add event handlers in styles AddToEventRouteCore(route, e); } /// /// This virtual method is to be overridden in Framework /// to be able to add handlers for styles /// internal virtual void AddToEventRouteCore(EventRoute route, RoutedEventArgs args) { } ////// Event Handlers Store /// ////// The idea of exposing this property is to allow /// elements in the Framework to generically use /// EventHandlersStore for Clr events as well. /// internal EventHandlersStore EventHandlersStore { [FriendAccessAllowed] // Built into Core, also used by Framework. get { if(!ReadFlag(CoreFlags.ExistsEventHandlersStore)) { return null; } return EventHandlersStoreField.GetValue(this); } } ////// Ensures that EventHandlersStore will return /// non-null when it is called. /// [FriendAccessAllowed] // Built into Core, also used by Framework. internal void EnsureEventHandlersStore() { if (EventHandlersStore == null) { EventHandlersStoreField.SetValue(this, new EventHandlersStore()); WriteFlag(CoreFlags.ExistsEventHandlersStore, true); } } #endregion Events ////// Used by UIElement, ContentElement, and UIElement3D to register common Events. /// ////// Critical: This code is used to register various thunks that are used to send input to the tree /// TreatAsSafe: This code attaches handlers that are inside the class and private. Not configurable or overridable /// [SecurityCritical,SecurityTreatAsSafe] internal static void RegisterEvents(Type type) { EventManager.RegisterClassHandler(type, Mouse.PreviewMouseDownEvent, new MouseButtonEventHandler(UIElement.OnPreviewMouseDownThunk), true); EventManager.RegisterClassHandler(type, Mouse.MouseDownEvent, new MouseButtonEventHandler(UIElement.OnMouseDownThunk), true); EventManager.RegisterClassHandler(type, Mouse.PreviewMouseUpEvent, new MouseButtonEventHandler(UIElement.OnPreviewMouseUpThunk), true); EventManager.RegisterClassHandler(type, Mouse.MouseUpEvent, new MouseButtonEventHandler(UIElement.OnMouseUpThunk), true); EventManager.RegisterClassHandler(type, UIElement.PreviewMouseLeftButtonDownEvent, new MouseButtonEventHandler(UIElement.OnPreviewMouseLeftButtonDownThunk), false); EventManager.RegisterClassHandler(type, UIElement.MouseLeftButtonDownEvent, new MouseButtonEventHandler(UIElement.OnMouseLeftButtonDownThunk), false); EventManager.RegisterClassHandler(type, UIElement.PreviewMouseLeftButtonUpEvent, new MouseButtonEventHandler(UIElement.OnPreviewMouseLeftButtonUpThunk), false); EventManager.RegisterClassHandler(type, UIElement.MouseLeftButtonUpEvent, new MouseButtonEventHandler(UIElement.OnMouseLeftButtonUpThunk), false); EventManager.RegisterClassHandler(type, UIElement.PreviewMouseRightButtonDownEvent, new MouseButtonEventHandler(UIElement.OnPreviewMouseRightButtonDownThunk), false); EventManager.RegisterClassHandler(type, UIElement.MouseRightButtonDownEvent, new MouseButtonEventHandler(UIElement.OnMouseRightButtonDownThunk), false); EventManager.RegisterClassHandler(type, UIElement.PreviewMouseRightButtonUpEvent, new MouseButtonEventHandler(UIElement.OnPreviewMouseRightButtonUpThunk), false); EventManager.RegisterClassHandler(type, UIElement.MouseRightButtonUpEvent, new MouseButtonEventHandler(UIElement.OnMouseRightButtonUpThunk), false); EventManager.RegisterClassHandler(type, Mouse.PreviewMouseMoveEvent, new MouseEventHandler(UIElement.OnPreviewMouseMoveThunk), false); EventManager.RegisterClassHandler(type, Mouse.MouseMoveEvent, new MouseEventHandler(UIElement.OnMouseMoveThunk), false); EventManager.RegisterClassHandler(type, Mouse.PreviewMouseWheelEvent, new MouseWheelEventHandler(UIElement.OnPreviewMouseWheelThunk), false); EventManager.RegisterClassHandler(type, Mouse.MouseWheelEvent, new MouseWheelEventHandler(UIElement.OnMouseWheelThunk), false); EventManager.RegisterClassHandler(type, Mouse.MouseEnterEvent, new MouseEventHandler(UIElement.OnMouseEnterThunk), false); EventManager.RegisterClassHandler(type, Mouse.MouseLeaveEvent, new MouseEventHandler(UIElement.OnMouseLeaveThunk), false); EventManager.RegisterClassHandler(type, Mouse.GotMouseCaptureEvent, new MouseEventHandler(UIElement.OnGotMouseCaptureThunk), false); EventManager.RegisterClassHandler(type, Mouse.LostMouseCaptureEvent, new MouseEventHandler(UIElement.OnLostMouseCaptureThunk), false); EventManager.RegisterClassHandler(type, Mouse.QueryCursorEvent, new QueryCursorEventHandler(UIElement.OnQueryCursorThunk), false); EventManager.RegisterClassHandler(type, Stylus.PreviewStylusDownEvent, new StylusDownEventHandler(UIElement.OnPreviewStylusDownThunk), false); EventManager.RegisterClassHandler(type, Stylus.StylusDownEvent, new StylusDownEventHandler(UIElement.OnStylusDownThunk), false); EventManager.RegisterClassHandler(type, Stylus.PreviewStylusUpEvent, new StylusEventHandler(UIElement.OnPreviewStylusUpThunk), false); EventManager.RegisterClassHandler(type, Stylus.StylusUpEvent, new StylusEventHandler(UIElement.OnStylusUpThunk), false); EventManager.RegisterClassHandler(type, Stylus.PreviewStylusMoveEvent, new StylusEventHandler(UIElement.OnPreviewStylusMoveThunk), false); EventManager.RegisterClassHandler(type, Stylus.StylusMoveEvent, new StylusEventHandler(UIElement.OnStylusMoveThunk), false); EventManager.RegisterClassHandler(type, Stylus.PreviewStylusInAirMoveEvent, new StylusEventHandler(UIElement.OnPreviewStylusInAirMoveThunk), false); EventManager.RegisterClassHandler(type, Stylus.StylusInAirMoveEvent, new StylusEventHandler(UIElement.OnStylusInAirMoveThunk), false); EventManager.RegisterClassHandler(type, Stylus.StylusEnterEvent, new StylusEventHandler(UIElement.OnStylusEnterThunk), false); EventManager.RegisterClassHandler(type, Stylus.StylusLeaveEvent, new StylusEventHandler(UIElement.OnStylusLeaveThunk), false); EventManager.RegisterClassHandler(type, Stylus.PreviewStylusInRangeEvent, new StylusEventHandler(UIElement.OnPreviewStylusInRangeThunk), false); EventManager.RegisterClassHandler(type, Stylus.StylusInRangeEvent, new StylusEventHandler(UIElement.OnStylusInRangeThunk), false); EventManager.RegisterClassHandler(type, Stylus.PreviewStylusOutOfRangeEvent, new StylusEventHandler(UIElement.OnPreviewStylusOutOfRangeThunk), false); EventManager.RegisterClassHandler(type, Stylus.StylusOutOfRangeEvent, new StylusEventHandler(UIElement.OnStylusOutOfRangeThunk), false); EventManager.RegisterClassHandler(type, Stylus.PreviewStylusSystemGestureEvent, new StylusSystemGestureEventHandler(UIElement.OnPreviewStylusSystemGestureThunk), false); EventManager.RegisterClassHandler(type, Stylus.StylusSystemGestureEvent, new StylusSystemGestureEventHandler(UIElement.OnStylusSystemGestureThunk), false); EventManager.RegisterClassHandler(type, Stylus.GotStylusCaptureEvent, new StylusEventHandler(UIElement.OnGotStylusCaptureThunk), false); EventManager.RegisterClassHandler(type, Stylus.LostStylusCaptureEvent, new StylusEventHandler(UIElement.OnLostStylusCaptureThunk), false); EventManager.RegisterClassHandler(type, Stylus.StylusButtonDownEvent, new StylusButtonEventHandler(UIElement.OnStylusButtonDownThunk), false); EventManager.RegisterClassHandler(type, Stylus.StylusButtonUpEvent, new StylusButtonEventHandler(UIElement.OnStylusButtonUpThunk), false); EventManager.RegisterClassHandler(type, Stylus.PreviewStylusButtonDownEvent, new StylusButtonEventHandler(UIElement.OnPreviewStylusButtonDownThunk), false); EventManager.RegisterClassHandler(type, Stylus.PreviewStylusButtonUpEvent, new StylusButtonEventHandler(UIElement.OnPreviewStylusButtonUpThunk), false); EventManager.RegisterClassHandler(type, Keyboard.PreviewKeyDownEvent, new KeyEventHandler(UIElement.OnPreviewKeyDownThunk), false); EventManager.RegisterClassHandler(type, Keyboard.KeyDownEvent, new KeyEventHandler(UIElement.OnKeyDownThunk), false); EventManager.RegisterClassHandler(type, Keyboard.PreviewKeyUpEvent, new KeyEventHandler(UIElement.OnPreviewKeyUpThunk), false); EventManager.RegisterClassHandler(type, Keyboard.KeyUpEvent, new KeyEventHandler(UIElement.OnKeyUpThunk), false); EventManager.RegisterClassHandler(type, Keyboard.PreviewGotKeyboardFocusEvent, new KeyboardFocusChangedEventHandler(UIElement.OnPreviewGotKeyboardFocusThunk), false); EventManager.RegisterClassHandler(type, Keyboard.GotKeyboardFocusEvent, new KeyboardFocusChangedEventHandler(UIElement.OnGotKeyboardFocusThunk), false); EventManager.RegisterClassHandler(type, Keyboard.PreviewLostKeyboardFocusEvent, new KeyboardFocusChangedEventHandler(UIElement.OnPreviewLostKeyboardFocusThunk), false); EventManager.RegisterClassHandler(type, Keyboard.LostKeyboardFocusEvent, new KeyboardFocusChangedEventHandler(UIElement.OnLostKeyboardFocusThunk), false); EventManager.RegisterClassHandler(type, TextCompositionManager.PreviewTextInputEvent, new TextCompositionEventHandler(UIElement.OnPreviewTextInputThunk), false); EventManager.RegisterClassHandler(type, TextCompositionManager.TextInputEvent, new TextCompositionEventHandler(UIElement.OnTextInputThunk), false); EventManager.RegisterClassHandler(type, CommandManager.PreviewExecutedEvent, new ExecutedRoutedEventHandler(UIElement.OnPreviewExecutedThunk), false); EventManager.RegisterClassHandler(type, CommandManager.ExecutedEvent, new ExecutedRoutedEventHandler(UIElement.OnExecutedThunk), false); EventManager.RegisterClassHandler(type, CommandManager.PreviewCanExecuteEvent, new CanExecuteRoutedEventHandler(UIElement.OnPreviewCanExecuteThunk), false); EventManager.RegisterClassHandler(type, CommandManager.CanExecuteEvent, new CanExecuteRoutedEventHandler(UIElement.OnCanExecuteThunk), false); EventManager.RegisterClassHandler(type, CommandDevice.CommandDeviceEvent, new CommandDeviceEventHandler(UIElement.OnCommandDeviceThunk), false); EventManager.RegisterClassHandler(type, DragDrop.PreviewQueryContinueDragEvent, new QueryContinueDragEventHandler(UIElement.OnPreviewQueryContinueDragThunk), false); EventManager.RegisterClassHandler(type, DragDrop.QueryContinueDragEvent, new QueryContinueDragEventHandler(UIElement.OnQueryContinueDragThunk), false); EventManager.RegisterClassHandler(type, DragDrop.PreviewGiveFeedbackEvent, new GiveFeedbackEventHandler(UIElement.OnPreviewGiveFeedbackThunk), false); EventManager.RegisterClassHandler(type, DragDrop.GiveFeedbackEvent, new GiveFeedbackEventHandler(UIElement.OnGiveFeedbackThunk), false); EventManager.RegisterClassHandler(type, DragDrop.PreviewDragEnterEvent, new DragEventHandler(UIElement.OnPreviewDragEnterThunk), false); EventManager.RegisterClassHandler(type, DragDrop.DragEnterEvent, new DragEventHandler(UIElement.OnDragEnterThunk), false); EventManager.RegisterClassHandler(type, DragDrop.PreviewDragOverEvent, new DragEventHandler(UIElement.OnPreviewDragOverThunk), false); EventManager.RegisterClassHandler(type, DragDrop.DragOverEvent, new DragEventHandler(UIElement.OnDragOverThunk), false); EventManager.RegisterClassHandler(type, DragDrop.PreviewDragLeaveEvent, new DragEventHandler(UIElement.OnPreviewDragLeaveThunk), false); EventManager.RegisterClassHandler(type, DragDrop.DragLeaveEvent, new DragEventHandler(UIElement.OnDragLeaveThunk), false); EventManager.RegisterClassHandler(type, DragDrop.PreviewDropEvent, new DragEventHandler(UIElement.OnPreviewDropThunk), false); EventManager.RegisterClassHandler(type, DragDrop.DropEvent, new DragEventHandler(UIElement.OnDropThunk), false); } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewMouseDownThunk(object sender, MouseButtonEventArgs e) { if(!e.Handled) { UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewMouseDown(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewMouseDown(e); } else { ((UIElement3D)sender).OnPreviewMouseDown(e); } } } // Always raise this "sub-event", but we pass along the handledness. UIElement.CrackMouseButtonEventAndReRaiseEvent((DependencyObject)sender, e); } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnMouseDownThunk(object sender, MouseButtonEventArgs e) { if(!e.Handled) { CommandManager.TranslateInput((IInputElement)sender, e); } if(!e.Handled) { UIElement uie = sender as UIElement; if (uie != null) { uie.OnMouseDown(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnMouseDown(e); } else { ((UIElement3D)sender).OnMouseDown(e); } } } // Always raise this "sub-event", but we pass along the handledness. UIElement.CrackMouseButtonEventAndReRaiseEvent((DependencyObject)sender, e); } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewMouseUpThunk(object sender, MouseButtonEventArgs e) { if(!e.Handled) { UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewMouseUp(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewMouseUp(e); } else { ((UIElement3D)sender).OnPreviewMouseUp(e); } } } // Always raise this "sub-event", but we pass along the handledness. UIElement.CrackMouseButtonEventAndReRaiseEvent((DependencyObject)sender, e); } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnMouseUpThunk(object sender, MouseButtonEventArgs e) { if(!e.Handled) { UIElement uie = sender as UIElement; if (uie != null) { uie.OnMouseUp(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnMouseUp(e); } else { ((UIElement3D)sender).OnMouseUp(e); } } } // Always raise this "sub-event", but we pass along the handledness. UIElement.CrackMouseButtonEventAndReRaiseEvent((DependencyObject)sender, e); } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewMouseLeftButtonDownThunk(object sender, MouseButtonEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewMouseLeftButtonDown(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewMouseLeftButtonDown(e); } else { ((UIElement3D)sender).OnPreviewMouseLeftButtonDown(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnMouseLeftButtonDownThunk(object sender, MouseButtonEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnMouseLeftButtonDown(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnMouseLeftButtonDown(e); } else { ((UIElement3D)sender).OnMouseLeftButtonDown(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewMouseLeftButtonUpThunk(object sender, MouseButtonEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewMouseLeftButtonUp(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewMouseLeftButtonUp(e); } else { ((UIElement3D)sender).OnPreviewMouseLeftButtonUp(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnMouseLeftButtonUpThunk(object sender, MouseButtonEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnMouseLeftButtonUp(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnMouseLeftButtonUp(e); } else { ((UIElement3D)sender).OnMouseLeftButtonUp(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewMouseRightButtonDownThunk(object sender, MouseButtonEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewMouseRightButtonDown(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewMouseRightButtonDown(e); } else { ((UIElement3D)sender).OnPreviewMouseRightButtonDown(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnMouseRightButtonDownThunk(object sender, MouseButtonEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnMouseRightButtonDown(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnMouseRightButtonDown(e); } else { ((UIElement3D)sender).OnMouseRightButtonDown(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewMouseRightButtonUpThunk(object sender, MouseButtonEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewMouseRightButtonUp(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewMouseRightButtonUp(e); } else { ((UIElement3D)sender).OnPreviewMouseRightButtonUp(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnMouseRightButtonUpThunk(object sender, MouseButtonEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnMouseRightButtonUp(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnMouseRightButtonUp(e); } else { ((UIElement3D)sender).OnMouseRightButtonUp(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewMouseMoveThunk(object sender, MouseEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewMouseMove(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewMouseMove(e); } else { ((UIElement3D)sender).OnPreviewMouseMove(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnMouseMoveThunk(object sender, MouseEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnMouseMove(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnMouseMove(e); } else { ((UIElement3D)sender).OnMouseMove(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewMouseWheelThunk(object sender, MouseWheelEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewMouseWheel(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewMouseWheel(e); } else { ((UIElement3D)sender).OnPreviewMouseWheel(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnMouseWheelThunk(object sender, MouseWheelEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); CommandManager.TranslateInput((IInputElement)sender, e); if(!e.Handled) { UIElement uie = sender as UIElement; if (uie != null) { uie.OnMouseWheel(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnMouseWheel(e); } else { ((UIElement3D)sender).OnMouseWheel(e); } } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnMouseEnterThunk(object sender, MouseEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnMouseEnter(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnMouseEnter(e); } else { ((UIElement3D)sender).OnMouseEnter(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnMouseLeaveThunk(object sender, MouseEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnMouseLeave(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnMouseLeave(e); } else { ((UIElement3D)sender).OnMouseLeave(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnGotMouseCaptureThunk(object sender, MouseEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnGotMouseCapture(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnGotMouseCapture(e); } else { ((UIElement3D)sender).OnGotMouseCapture(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnLostMouseCaptureThunk(object sender, MouseEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnLostMouseCapture(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnLostMouseCapture(e); } else { ((UIElement3D)sender).OnLostMouseCapture(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnQueryCursorThunk(object sender, QueryCursorEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnQueryCursor(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnQueryCursor(e); } else { ((UIElement3D)sender).OnQueryCursor(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewStylusDownThunk(object sender, StylusDownEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewStylusDown(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewStylusDown(e); } else { ((UIElement3D)sender).OnPreviewStylusDown(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnStylusDownThunk(object sender, StylusDownEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnStylusDown(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnStylusDown(e); } else { ((UIElement3D)sender).OnStylusDown(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewStylusUpThunk(object sender, StylusEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewStylusUp(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewStylusUp(e); } else { ((UIElement3D)sender).OnPreviewStylusUp(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnStylusUpThunk(object sender, StylusEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnStylusUp(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnStylusUp(e); } else { ((UIElement3D)sender).OnStylusUp(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewStylusMoveThunk(object sender, StylusEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewStylusMove(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewStylusMove(e); } else { ((UIElement3D)sender).OnPreviewStylusMove(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnStylusMoveThunk(object sender, StylusEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnStylusMove(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnStylusMove(e); } else { ((UIElement3D)sender).OnStylusMove(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewStylusInAirMoveThunk(object sender, StylusEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewStylusInAirMove(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewStylusInAirMove(e); } else { ((UIElement3D)sender).OnPreviewStylusInAirMove(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnStylusInAirMoveThunk(object sender, StylusEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnStylusInAirMove(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnStylusInAirMove(e); } else { ((UIElement3D)sender).OnStylusInAirMove(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnStylusEnterThunk(object sender, StylusEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnStylusEnter(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnStylusEnter(e); } else { ((UIElement3D)sender).OnStylusEnter(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnStylusLeaveThunk(object sender, StylusEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnStylusLeave(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnStylusLeave(e); } else { ((UIElement3D)sender).OnStylusLeave(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewStylusInRangeThunk(object sender, StylusEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewStylusInRange(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewStylusInRange(e); } else { ((UIElement3D)sender).OnPreviewStylusInRange(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnStylusInRangeThunk(object sender, StylusEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnStylusInRange(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnStylusInRange(e); } else { ((UIElement3D)sender).OnStylusInRange(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewStylusOutOfRangeThunk(object sender, StylusEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewStylusOutOfRange(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewStylusOutOfRange(e); } else { ((UIElement3D)sender).OnPreviewStylusOutOfRange(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnStylusOutOfRangeThunk(object sender, StylusEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnStylusOutOfRange(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnStylusOutOfRange(e); } else { ((UIElement3D)sender).OnStylusOutOfRange(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewStylusSystemGestureThunk(object sender, StylusSystemGestureEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewStylusSystemGesture(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewStylusSystemGesture(e); } else { ((UIElement3D)sender).OnPreviewStylusSystemGesture(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnStylusSystemGestureThunk(object sender, StylusSystemGestureEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnStylusSystemGesture(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnStylusSystemGesture(e); } else { ((UIElement3D)sender).OnStylusSystemGesture(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnGotStylusCaptureThunk(object sender, StylusEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnGotStylusCapture(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnGotStylusCapture(e); } else { ((UIElement3D)sender).OnGotStylusCapture(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnLostStylusCaptureThunk(object sender, StylusEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnLostStylusCapture(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnLostStylusCapture(e); } else { ((UIElement3D)sender).OnLostStylusCapture(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnStylusButtonDownThunk(object sender, StylusButtonEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnStylusButtonDown(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnStylusButtonDown(e); } else { ((UIElement3D)sender).OnStylusButtonDown(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnStylusButtonUpThunk(object sender, StylusButtonEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnStylusButtonUp(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnStylusButtonUp(e); } else { ((UIElement3D)sender).OnStylusButtonUp(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewStylusButtonDownThunk(object sender, StylusButtonEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewStylusButtonDown(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewStylusButtonDown(e); } else { ((UIElement3D)sender).OnPreviewStylusButtonDown(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewStylusButtonUpThunk(object sender, StylusButtonEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewStylusButtonUp(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewStylusButtonUp(e); } else { ((UIElement3D)sender).OnPreviewStylusButtonUp(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewKeyDownThunk(object sender, KeyEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewKeyDown(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewKeyDown(e); } else { ((UIElement3D)sender).OnPreviewKeyDown(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnKeyDownThunk(object sender, KeyEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); CommandManager.TranslateInput((IInputElement)sender, e); if(!e.Handled) { UIElement uie = sender as UIElement; if (uie != null) { uie.OnKeyDown(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnKeyDown(e); } else { ((UIElement3D)sender).OnKeyDown(e); } } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewKeyUpThunk(object sender, KeyEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewKeyUp(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewKeyUp(e); } else { ((UIElement3D)sender).OnPreviewKeyUp(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnKeyUpThunk(object sender, KeyEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnKeyUp(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnKeyUp(e); } else { ((UIElement3D)sender).OnKeyUp(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewGotKeyboardFocusThunk(object sender, KeyboardFocusChangedEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewGotKeyboardFocus(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewGotKeyboardFocus(e); } else { ((UIElement3D)sender).OnPreviewGotKeyboardFocus(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnGotKeyboardFocusThunk(object sender, KeyboardFocusChangedEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnGotKeyboardFocus(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnGotKeyboardFocus(e); } else { ((UIElement3D)sender).OnGotKeyboardFocus(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewLostKeyboardFocusThunk(object sender, KeyboardFocusChangedEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewLostKeyboardFocus(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewLostKeyboardFocus(e); } else { ((UIElement3D)sender).OnPreviewLostKeyboardFocus(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnLostKeyboardFocusThunk(object sender, KeyboardFocusChangedEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnLostKeyboardFocus(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnLostKeyboardFocus(e); } else { ((UIElement3D)sender).OnLostKeyboardFocus(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewTextInputThunk(object sender, TextCompositionEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewTextInput(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewTextInput(e); } else { ((UIElement3D)sender).OnPreviewTextInput(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnTextInputThunk(object sender, TextCompositionEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnTextInput(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnTextInput(e); } else { ((UIElement3D)sender).OnTextInput(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewExecutedThunk(object sender, ExecutedRoutedEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); // Command Manager will determine if preview or regular event. CommandManager.OnExecuted(sender, e); } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnExecutedThunk(object sender, ExecutedRoutedEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); // Command Manager will determine if preview or regular event. CommandManager.OnExecuted(sender, e); } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewCanExecuteThunk(object sender, CanExecuteRoutedEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); // Command Manager will determine if preview or regular event. CommandManager.OnCanExecute(sender, e); } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnCanExecuteThunk(object sender, CanExecuteRoutedEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); // Command Manager will determine if preview or regular event. CommandManager.OnCanExecute(sender, e); } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnCommandDeviceThunk(object sender, CommandDeviceEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); // Command Manager will determine if preview or regular event. CommandManager.OnCommandDevice(sender, e); } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewQueryContinueDragThunk(object sender, QueryContinueDragEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewQueryContinueDrag(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewQueryContinueDrag(e); } else { ((UIElement3D)sender).OnPreviewQueryContinueDrag(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnQueryContinueDragThunk(object sender, QueryContinueDragEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnQueryContinueDrag(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnQueryContinueDrag(e); } else { ((UIElement3D)sender).OnQueryContinueDrag(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewGiveFeedbackThunk(object sender, GiveFeedbackEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewGiveFeedback(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewGiveFeedback(e); } else { ((UIElement3D)sender).OnPreviewGiveFeedback(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnGiveFeedbackThunk(object sender, GiveFeedbackEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnGiveFeedback(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnGiveFeedback(e); } else { ((UIElement3D)sender).OnGiveFeedback(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewDragEnterThunk(object sender, DragEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewDragEnter(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewDragEnter(e); } else { ((UIElement3D)sender).OnPreviewDragEnter(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnDragEnterThunk(object sender, DragEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnDragEnter(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnDragEnter(e); } else { ((UIElement3D)sender).OnDragEnter(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewDragOverThunk(object sender, DragEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewDragOver(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewDragOver(e); } else { ((UIElement3D)sender).OnPreviewDragOver(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnDragOverThunk(object sender, DragEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnDragOver(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnDragOver(e); } else { ((UIElement3D)sender).OnDragOver(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewDragLeaveThunk(object sender, DragEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewDragLeave(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewDragLeave(e); } else { ((UIElement3D)sender).OnPreviewDragLeave(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnDragLeaveThunk(object sender, DragEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnDragLeave(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnDragLeave(e); } else { ((UIElement3D)sender).OnDragLeave(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewDropThunk(object sender, DragEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewDrop(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewDrop(e); } else { ((UIElement3D)sender).OnPreviewDrop(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnDropThunk(object sender, DragEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnDrop(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnDrop(e); } else { ((UIElement3D)sender).OnDrop(e); } } } ////// Alias to the Mouse.PreviewMouseDownEvent. /// public static readonly RoutedEvent PreviewMouseDownEvent = Mouse.PreviewMouseDownEvent.AddOwner(_typeofThis); ////// Event reporting the mouse button was pressed /// public event MouseButtonEventHandler PreviewMouseDown { add { AddHandler(Mouse.PreviewMouseDownEvent, value, false); } remove { RemoveHandler(Mouse.PreviewMouseDownEvent, value); } } ////// Virtual method reporting the mouse button was pressed /// protected virtual void OnPreviewMouseDown(MouseButtonEventArgs e) {} ////// Alias to the Mouse.MouseDownEvent. /// public static readonly RoutedEvent MouseDownEvent = Mouse.MouseDownEvent.AddOwner(_typeofThis); ////// Event reporting the mouse button was pressed /// public event MouseButtonEventHandler MouseDown { add { AddHandler(Mouse.MouseDownEvent, value, false); } remove { RemoveHandler(Mouse.MouseDownEvent, value); } } ////// Virtual method reporting the mouse button was pressed /// protected virtual void OnMouseDown(MouseButtonEventArgs e) {} ////// Alias to the Mouse.PreviewMouseUpEvent. /// public static readonly RoutedEvent PreviewMouseUpEvent = Mouse.PreviewMouseUpEvent.AddOwner(_typeofThis); ////// Event reporting the mouse button was released /// public event MouseButtonEventHandler PreviewMouseUp { add { AddHandler(Mouse.PreviewMouseUpEvent, value, false); } remove { RemoveHandler(Mouse.PreviewMouseUpEvent, value); } } ////// Virtual method reporting the mouse button was released /// protected virtual void OnPreviewMouseUp(MouseButtonEventArgs e) {} ////// Alias to the Mouse.MouseUpEvent. /// public static readonly RoutedEvent MouseUpEvent = Mouse.MouseUpEvent.AddOwner(_typeofThis); ////// Event reporting the mouse button was released /// public event MouseButtonEventHandler MouseUp { add { AddHandler(Mouse.MouseUpEvent, value, false); } remove { RemoveHandler(Mouse.MouseUpEvent, value); } } ////// Virtual method reporting the mouse button was released /// protected virtual void OnMouseUp(MouseButtonEventArgs e) {} ////// Declaration of the routed event reporting the left mouse button was pressed /// public static readonly RoutedEvent PreviewMouseLeftButtonDownEvent = EventManager.RegisterRoutedEvent("PreviewMouseLeftButtonDown", RoutingStrategy.Direct, typeof(MouseButtonEventHandler), _typeofThis); ////// Event reporting the left mouse button was pressed /// public event MouseButtonEventHandler PreviewMouseLeftButtonDown { add { AddHandler(UIElement.PreviewMouseLeftButtonDownEvent, value, false); } remove { RemoveHandler(UIElement.PreviewMouseLeftButtonDownEvent, value); } } ////// Virtual method reporting the left mouse button was pressed /// protected virtual void OnPreviewMouseLeftButtonDown(MouseButtonEventArgs e) {} ////// Declaration of the routed event reporting the left mouse button was pressed /// public static readonly RoutedEvent MouseLeftButtonDownEvent = EventManager.RegisterRoutedEvent("MouseLeftButtonDown", RoutingStrategy.Direct, typeof(MouseButtonEventHandler), _typeofThis); ////// Event reporting the left mouse button was pressed /// public event MouseButtonEventHandler MouseLeftButtonDown { add { AddHandler(UIElement.MouseLeftButtonDownEvent, value, false); } remove { RemoveHandler(UIElement.MouseLeftButtonDownEvent, value); } } ////// Virtual method reporting the left mouse button was pressed /// protected virtual void OnMouseLeftButtonDown(MouseButtonEventArgs e) {} ////// Declaration of the routed event reporting the left mouse button was released /// public static readonly RoutedEvent PreviewMouseLeftButtonUpEvent = EventManager.RegisterRoutedEvent("PreviewMouseLeftButtonUp", RoutingStrategy.Direct, typeof(MouseButtonEventHandler), _typeofThis); ////// Event reporting the left mouse button was released /// public event MouseButtonEventHandler PreviewMouseLeftButtonUp { add { AddHandler(UIElement.PreviewMouseLeftButtonUpEvent, value, false); } remove { RemoveHandler(UIElement.PreviewMouseLeftButtonUpEvent, value); } } ////// Virtual method reporting the left mouse button was released /// protected virtual void OnPreviewMouseLeftButtonUp(MouseButtonEventArgs e) {} ////// Declaration of the routed event reporting the left mouse button was released /// public static readonly RoutedEvent MouseLeftButtonUpEvent = EventManager.RegisterRoutedEvent("MouseLeftButtonUp", RoutingStrategy.Direct, typeof(MouseButtonEventHandler), _typeofThis); ////// Event reporting the left mouse button was released /// public event MouseButtonEventHandler MouseLeftButtonUp { add { AddHandler(UIElement.MouseLeftButtonUpEvent, value, false); } remove { RemoveHandler(UIElement.MouseLeftButtonUpEvent, value); } } ////// Virtual method reporting the left mouse button was released /// protected virtual void OnMouseLeftButtonUp(MouseButtonEventArgs e) {} ////// Declaration of the routed event reporting the right mouse button was pressed /// public static readonly RoutedEvent PreviewMouseRightButtonDownEvent = EventManager.RegisterRoutedEvent("PreviewMouseRightButtonDown", RoutingStrategy.Direct, typeof(MouseButtonEventHandler), _typeofThis); ////// Event reporting the right mouse button was pressed /// public event MouseButtonEventHandler PreviewMouseRightButtonDown { add { AddHandler(UIElement.PreviewMouseRightButtonDownEvent, value, false); } remove { RemoveHandler(UIElement.PreviewMouseRightButtonDownEvent, value); } } ////// Virtual method reporting the right mouse button was pressed /// protected virtual void OnPreviewMouseRightButtonDown(MouseButtonEventArgs e) {} ////// Declaration of the routed event reporting the right mouse button was pressed /// public static readonly RoutedEvent MouseRightButtonDownEvent = EventManager.RegisterRoutedEvent("MouseRightButtonDown", RoutingStrategy.Direct, typeof(MouseButtonEventHandler), _typeofThis); ////// Event reporting the right mouse button was pressed /// public event MouseButtonEventHandler MouseRightButtonDown { add { AddHandler(UIElement.MouseRightButtonDownEvent, value, false); } remove { RemoveHandler(UIElement.MouseRightButtonDownEvent, value); } } ////// Virtual method reporting the right mouse button was pressed /// protected virtual void OnMouseRightButtonDown(MouseButtonEventArgs e) {} ////// Declaration of the routed event reporting the right mouse button was released /// public static readonly RoutedEvent PreviewMouseRightButtonUpEvent = EventManager.RegisterRoutedEvent("PreviewMouseRightButtonUp", RoutingStrategy.Direct, typeof(MouseButtonEventHandler), _typeofThis); ////// Event reporting the right mouse button was released /// public event MouseButtonEventHandler PreviewMouseRightButtonUp { add { AddHandler(UIElement.PreviewMouseRightButtonUpEvent, value, false); } remove { RemoveHandler(UIElement.PreviewMouseRightButtonUpEvent, value); } } ////// Virtual method reporting the right mouse button was released /// protected virtual void OnPreviewMouseRightButtonUp(MouseButtonEventArgs e) {} ////// Declaration of the routed event reporting the right mouse button was released /// public static readonly RoutedEvent MouseRightButtonUpEvent = EventManager.RegisterRoutedEvent("MouseRightButtonUp", RoutingStrategy.Direct, typeof(MouseButtonEventHandler), _typeofThis); ////// Event reporting the right mouse button was released /// public event MouseButtonEventHandler MouseRightButtonUp { add { AddHandler(UIElement.MouseRightButtonUpEvent, value, false); } remove { RemoveHandler(UIElement.MouseRightButtonUpEvent, value); } } ////// Virtual method reporting the right mouse button was released /// protected virtual void OnMouseRightButtonUp(MouseButtonEventArgs e) {} ////// Alias to the Mouse.PreviewMouseMoveEvent. /// public static readonly RoutedEvent PreviewMouseMoveEvent = Mouse.PreviewMouseMoveEvent.AddOwner(_typeofThis); ////// Event reporting a mouse move /// public event MouseEventHandler PreviewMouseMove { add { AddHandler(Mouse.PreviewMouseMoveEvent, value, false); } remove { RemoveHandler(Mouse.PreviewMouseMoveEvent, value); } } ////// Virtual method reporting a mouse move /// protected virtual void OnPreviewMouseMove(MouseEventArgs e) {} ////// Alias to the Mouse.MouseMoveEvent. /// public static readonly RoutedEvent MouseMoveEvent = Mouse.MouseMoveEvent.AddOwner(_typeofThis); ////// Event reporting a mouse move /// public event MouseEventHandler MouseMove { add { AddHandler(Mouse.MouseMoveEvent, value, false); } remove { RemoveHandler(Mouse.MouseMoveEvent, value); } } ////// Virtual method reporting a mouse move /// protected virtual void OnMouseMove(MouseEventArgs e) {} ////// Alias to the Mouse.PreviewMouseWheelEvent. /// public static readonly RoutedEvent PreviewMouseWheelEvent = Mouse.PreviewMouseWheelEvent.AddOwner(_typeofThis); ////// Event reporting a mouse wheel rotation /// public event MouseWheelEventHandler PreviewMouseWheel { add { AddHandler(Mouse.PreviewMouseWheelEvent, value, false); } remove { RemoveHandler(Mouse.PreviewMouseWheelEvent, value); } } ////// Virtual method reporting a mouse wheel rotation /// protected virtual void OnPreviewMouseWheel(MouseWheelEventArgs e) {} ////// Alias to the Mouse.MouseWheelEvent. /// public static readonly RoutedEvent MouseWheelEvent = Mouse.MouseWheelEvent.AddOwner(_typeofThis); ////// Event reporting a mouse wheel rotation /// public event MouseWheelEventHandler MouseWheel { add { AddHandler(Mouse.MouseWheelEvent, value, false); } remove { RemoveHandler(Mouse.MouseWheelEvent, value); } } ////// Virtual method reporting a mouse wheel rotation /// protected virtual void OnMouseWheel(MouseWheelEventArgs e) {} ////// Alias to the Mouse.MouseEnterEvent. /// public static readonly RoutedEvent MouseEnterEvent = Mouse.MouseEnterEvent.AddOwner(_typeofThis); ////// Event reporting the mouse entered this element /// public event MouseEventHandler MouseEnter { add { AddHandler(Mouse.MouseEnterEvent, value, false); } remove { RemoveHandler(Mouse.MouseEnterEvent, value); } } ////// Virtual method reporting the mouse entered this element /// protected virtual void OnMouseEnter(MouseEventArgs e) {} ////// Alias to the Mouse.MouseLeaveEvent. /// public static readonly RoutedEvent MouseLeaveEvent = Mouse.MouseLeaveEvent.AddOwner(_typeofThis); ////// Event reporting the mouse left this element /// public event MouseEventHandler MouseLeave { add { AddHandler(Mouse.MouseLeaveEvent, value, false); } remove { RemoveHandler(Mouse.MouseLeaveEvent, value); } } ////// Virtual method reporting the mouse left this element /// protected virtual void OnMouseLeave(MouseEventArgs e) {} ////// Alias to the Mouse.GotMouseCaptureEvent. /// public static readonly RoutedEvent GotMouseCaptureEvent = Mouse.GotMouseCaptureEvent.AddOwner(_typeofThis); ////// Event reporting that this element got the mouse capture /// public event MouseEventHandler GotMouseCapture { add { AddHandler(Mouse.GotMouseCaptureEvent, value, false); } remove { RemoveHandler(Mouse.GotMouseCaptureEvent, value); } } ////// Virtual method reporting that this element got the mouse capture /// protected virtual void OnGotMouseCapture(MouseEventArgs e) {} ////// Alias to the Mouse.LostMouseCaptureEvent. /// public static readonly RoutedEvent LostMouseCaptureEvent = Mouse.LostMouseCaptureEvent.AddOwner(_typeofThis); ////// Event reporting that this element lost the mouse capture /// public event MouseEventHandler LostMouseCapture { add { AddHandler(Mouse.LostMouseCaptureEvent, value, false); } remove { RemoveHandler(Mouse.LostMouseCaptureEvent, value); } } ////// Virtual method reporting that this element lost the mouse capture /// protected virtual void OnLostMouseCapture(MouseEventArgs e) {} ////// Alias to the Mouse.QueryCursorEvent. /// public static readonly RoutedEvent QueryCursorEvent = Mouse.QueryCursorEvent.AddOwner(_typeofThis); ////// Event reporting the cursor to display was requested /// public event QueryCursorEventHandler QueryCursor { add { AddHandler(Mouse.QueryCursorEvent, value, false); } remove { RemoveHandler(Mouse.QueryCursorEvent, value); } } ////// Virtual method reporting the cursor to display was requested /// protected virtual void OnQueryCursor(QueryCursorEventArgs e) {} ////// Alias to the Stylus.PreviewStylusDownEvent. /// public static readonly RoutedEvent PreviewStylusDownEvent = Stylus.PreviewStylusDownEvent.AddOwner(_typeofThis); ////// Event reporting a stylus-down /// public event StylusDownEventHandler PreviewStylusDown { add { AddHandler(Stylus.PreviewStylusDownEvent, value, false); } remove { RemoveHandler(Stylus.PreviewStylusDownEvent, value); } } ////// Virtual method reporting a stylus-down /// protected virtual void OnPreviewStylusDown(StylusDownEventArgs e) {} ////// Alias to the Stylus.StylusDownEvent. /// public static readonly RoutedEvent StylusDownEvent = Stylus.StylusDownEvent.AddOwner(_typeofThis); ////// Event reporting a stylus-down /// public event StylusDownEventHandler StylusDown { add { AddHandler(Stylus.StylusDownEvent, value, false); } remove { RemoveHandler(Stylus.StylusDownEvent, value); } } ////// Virtual method reporting a stylus-down /// protected virtual void OnStylusDown(StylusDownEventArgs e) {} ////// Alias to the Stylus.PreviewStylusUpEvent. /// public static readonly RoutedEvent PreviewStylusUpEvent = Stylus.PreviewStylusUpEvent.AddOwner(_typeofThis); ////// Event reporting a stylus-up /// public event StylusEventHandler PreviewStylusUp { add { AddHandler(Stylus.PreviewStylusUpEvent, value, false); } remove { RemoveHandler(Stylus.PreviewStylusUpEvent, value); } } ////// Virtual method reporting a stylus-up /// protected virtual void OnPreviewStylusUp(StylusEventArgs e) {} ////// Alias to the Stylus.StylusUpEvent. /// public static readonly RoutedEvent StylusUpEvent = Stylus.StylusUpEvent.AddOwner(_typeofThis); ////// Event reporting a stylus-up /// public event StylusEventHandler StylusUp { add { AddHandler(Stylus.StylusUpEvent, value, false); } remove { RemoveHandler(Stylus.StylusUpEvent, value); } } ////// Virtual method reporting a stylus-up /// protected virtual void OnStylusUp(StylusEventArgs e) {} ////// Alias to the Stylus.PreviewStylusMoveEvent. /// public static readonly RoutedEvent PreviewStylusMoveEvent = Stylus.PreviewStylusMoveEvent.AddOwner(_typeofThis); ////// Event reporting a stylus move /// public event StylusEventHandler PreviewStylusMove { add { AddHandler(Stylus.PreviewStylusMoveEvent, value, false); } remove { RemoveHandler(Stylus.PreviewStylusMoveEvent, value); } } ////// Virtual method reporting a stylus move /// protected virtual void OnPreviewStylusMove(StylusEventArgs e) {} ////// Alias to the Stylus.StylusMoveEvent. /// public static readonly RoutedEvent StylusMoveEvent = Stylus.StylusMoveEvent.AddOwner(_typeofThis); ////// Event reporting a stylus move /// public event StylusEventHandler StylusMove { add { AddHandler(Stylus.StylusMoveEvent, value, false); } remove { RemoveHandler(Stylus.StylusMoveEvent, value); } } ////// Virtual method reporting a stylus move /// protected virtual void OnStylusMove(StylusEventArgs e) {} ////// Alias to the Stylus.PreviewStylusInAirMoveEvent. /// public static readonly RoutedEvent PreviewStylusInAirMoveEvent = Stylus.PreviewStylusInAirMoveEvent.AddOwner(_typeofThis); ////// Event reporting a stylus-in-air-move /// public event StylusEventHandler PreviewStylusInAirMove { add { AddHandler(Stylus.PreviewStylusInAirMoveEvent, value, false); } remove { RemoveHandler(Stylus.PreviewStylusInAirMoveEvent, value); } } ////// Virtual method reporting a stylus-in-air-move /// protected virtual void OnPreviewStylusInAirMove(StylusEventArgs e) {} ////// Alias to the Stylus.StylusInAirMoveEvent. /// public static readonly RoutedEvent StylusInAirMoveEvent = Stylus.StylusInAirMoveEvent.AddOwner(_typeofThis); ////// Event reporting a stylus-in-air-move /// public event StylusEventHandler StylusInAirMove { add { AddHandler(Stylus.StylusInAirMoveEvent, value, false); } remove { RemoveHandler(Stylus.StylusInAirMoveEvent, value); } } ////// Virtual method reporting a stylus-in-air-move /// protected virtual void OnStylusInAirMove(StylusEventArgs e) {} ////// Alias to the Stylus.StylusEnterEvent. /// public static readonly RoutedEvent StylusEnterEvent = Stylus.StylusEnterEvent.AddOwner(_typeofThis); ////// Event reporting the stylus entered this element /// public event StylusEventHandler StylusEnter { add { AddHandler(Stylus.StylusEnterEvent, value, false); } remove { RemoveHandler(Stylus.StylusEnterEvent, value); } } ////// Virtual method reporting the stylus entered this element /// protected virtual void OnStylusEnter(StylusEventArgs e) {} ////// Alias to the Stylus.StylusLeaveEvent. /// public static readonly RoutedEvent StylusLeaveEvent = Stylus.StylusLeaveEvent.AddOwner(_typeofThis); ////// Event reporting the stylus left this element /// public event StylusEventHandler StylusLeave { add { AddHandler(Stylus.StylusLeaveEvent, value, false); } remove { RemoveHandler(Stylus.StylusLeaveEvent, value); } } ////// Virtual method reporting the stylus left this element /// protected virtual void OnStylusLeave(StylusEventArgs e) {} ////// Alias to the Stylus.PreviewStylusInRangeEvent. /// public static readonly RoutedEvent PreviewStylusInRangeEvent = Stylus.PreviewStylusInRangeEvent.AddOwner(_typeofThis); ////// Event reporting the stylus is now in range of the digitizer /// public event StylusEventHandler PreviewStylusInRange { add { AddHandler(Stylus.PreviewStylusInRangeEvent, value, false); } remove { RemoveHandler(Stylus.PreviewStylusInRangeEvent, value); } } ////// Virtual method reporting the stylus is now in range of the digitizer /// protected virtual void OnPreviewStylusInRange(StylusEventArgs e) {} ////// Alias to the Stylus.StylusInRangeEvent. /// public static readonly RoutedEvent StylusInRangeEvent = Stylus.StylusInRangeEvent.AddOwner(_typeofThis); ////// Event reporting the stylus is now in range of the digitizer /// public event StylusEventHandler StylusInRange { add { AddHandler(Stylus.StylusInRangeEvent, value, false); } remove { RemoveHandler(Stylus.StylusInRangeEvent, value); } } ////// Virtual method reporting the stylus is now in range of the digitizer /// protected virtual void OnStylusInRange(StylusEventArgs e) {} ////// Alias to the Stylus.PreviewStylusOutOfRangeEvent. /// public static readonly RoutedEvent PreviewStylusOutOfRangeEvent = Stylus.PreviewStylusOutOfRangeEvent.AddOwner(_typeofThis); ////// Event reporting the stylus is now out of range of the digitizer /// public event StylusEventHandler PreviewStylusOutOfRange { add { AddHandler(Stylus.PreviewStylusOutOfRangeEvent, value, false); } remove { RemoveHandler(Stylus.PreviewStylusOutOfRangeEvent, value); } } ////// Virtual method reporting the stylus is now out of range of the digitizer /// protected virtual void OnPreviewStylusOutOfRange(StylusEventArgs e) {} ////// Alias to the Stylus.StylusOutOfRangeEvent. /// public static readonly RoutedEvent StylusOutOfRangeEvent = Stylus.StylusOutOfRangeEvent.AddOwner(_typeofThis); ////// Event reporting the stylus is now out of range of the digitizer /// public event StylusEventHandler StylusOutOfRange { add { AddHandler(Stylus.StylusOutOfRangeEvent, value, false); } remove { RemoveHandler(Stylus.StylusOutOfRangeEvent, value); } } ////// Virtual method reporting the stylus is now out of range of the digitizer /// protected virtual void OnStylusOutOfRange(StylusEventArgs e) {} ////// Alias to the Stylus.PreviewStylusSystemGestureEvent. /// public static readonly RoutedEvent PreviewStylusSystemGestureEvent = Stylus.PreviewStylusSystemGestureEvent.AddOwner(_typeofThis); ////// Event reporting a stylus system gesture /// public event StylusSystemGestureEventHandler PreviewStylusSystemGesture { add { AddHandler(Stylus.PreviewStylusSystemGestureEvent, value, false); } remove { RemoveHandler(Stylus.PreviewStylusSystemGestureEvent, value); } } ////// Virtual method reporting a stylus system gesture /// protected virtual void OnPreviewStylusSystemGesture(StylusSystemGestureEventArgs e) {} ////// Alias to the Stylus.StylusSystemGestureEvent. /// public static readonly RoutedEvent StylusSystemGestureEvent = Stylus.StylusSystemGestureEvent.AddOwner(_typeofThis); ////// Event reporting a stylus system gesture /// public event StylusSystemGestureEventHandler StylusSystemGesture { add { AddHandler(Stylus.StylusSystemGestureEvent, value, false); } remove { RemoveHandler(Stylus.StylusSystemGestureEvent, value); } } ////// Virtual method reporting a stylus system gesture /// protected virtual void OnStylusSystemGesture(StylusSystemGestureEventArgs e) {} ////// Alias to the Stylus.GotStylusCaptureEvent. /// public static readonly RoutedEvent GotStylusCaptureEvent = Stylus.GotStylusCaptureEvent.AddOwner(_typeofThis); ////// Event reporting that this element got the stylus capture /// public event StylusEventHandler GotStylusCapture { add { AddHandler(Stylus.GotStylusCaptureEvent, value, false); } remove { RemoveHandler(Stylus.GotStylusCaptureEvent, value); } } ////// Virtual method reporting that this element got the stylus capture /// protected virtual void OnGotStylusCapture(StylusEventArgs e) {} ////// Alias to the Stylus.LostStylusCaptureEvent. /// public static readonly RoutedEvent LostStylusCaptureEvent = Stylus.LostStylusCaptureEvent.AddOwner(_typeofThis); ////// Event reporting that this element lost the stylus capture /// public event StylusEventHandler LostStylusCapture { add { AddHandler(Stylus.LostStylusCaptureEvent, value, false); } remove { RemoveHandler(Stylus.LostStylusCaptureEvent, value); } } ////// Virtual method reporting that this element lost the stylus capture /// protected virtual void OnLostStylusCapture(StylusEventArgs e) {} ////// Alias to the Stylus.StylusButtonDownEvent. /// public static readonly RoutedEvent StylusButtonDownEvent = Stylus.StylusButtonDownEvent.AddOwner(_typeofThis); ////// Event reporting the stylus button is down /// public event StylusButtonEventHandler StylusButtonDown { add { AddHandler(Stylus.StylusButtonDownEvent, value, false); } remove { RemoveHandler(Stylus.StylusButtonDownEvent, value); } } ////// Virtual method reporting the stylus button is down /// protected virtual void OnStylusButtonDown(StylusButtonEventArgs e) {} ////// Alias to the Stylus.StylusButtonUpEvent. /// public static readonly RoutedEvent StylusButtonUpEvent = Stylus.StylusButtonUpEvent.AddOwner(_typeofThis); ////// Event reporting the stylus button is up /// public event StylusButtonEventHandler StylusButtonUp { add { AddHandler(Stylus.StylusButtonUpEvent, value, false); } remove { RemoveHandler(Stylus.StylusButtonUpEvent, value); } } ////// Virtual method reporting the stylus button is up /// protected virtual void OnStylusButtonUp(StylusButtonEventArgs e) {} ////// Alias to the Stylus.PreviewStylusButtonDownEvent. /// public static readonly RoutedEvent PreviewStylusButtonDownEvent = Stylus.PreviewStylusButtonDownEvent.AddOwner(_typeofThis); ////// Event reporting the stylus button is down /// public event StylusButtonEventHandler PreviewStylusButtonDown { add { AddHandler(Stylus.PreviewStylusButtonDownEvent, value, false); } remove { RemoveHandler(Stylus.PreviewStylusButtonDownEvent, value); } } ////// Virtual method reporting the stylus button is down /// protected virtual void OnPreviewStylusButtonDown(StylusButtonEventArgs e) {} ////// Alias to the Stylus.PreviewStylusButtonUpEvent. /// public static readonly RoutedEvent PreviewStylusButtonUpEvent = Stylus.PreviewStylusButtonUpEvent.AddOwner(_typeofThis); ////// Event reporting the stylus button is up /// public event StylusButtonEventHandler PreviewStylusButtonUp { add { AddHandler(Stylus.PreviewStylusButtonUpEvent, value, false); } remove { RemoveHandler(Stylus.PreviewStylusButtonUpEvent, value); } } ////// Virtual method reporting the stylus button is up /// protected virtual void OnPreviewStylusButtonUp(StylusButtonEventArgs e) {} ////// Alias to the Keyboard.PreviewKeyDownEvent. /// public static readonly RoutedEvent PreviewKeyDownEvent = Keyboard.PreviewKeyDownEvent.AddOwner(_typeofThis); ////// Event reporting a key was pressed /// public event KeyEventHandler PreviewKeyDown { add { AddHandler(Keyboard.PreviewKeyDownEvent, value, false); } remove { RemoveHandler(Keyboard.PreviewKeyDownEvent, value); } } ////// Virtual method reporting a key was pressed /// protected virtual void OnPreviewKeyDown(KeyEventArgs e) {} ////// Alias to the Keyboard.KeyDownEvent. /// public static readonly RoutedEvent KeyDownEvent = Keyboard.KeyDownEvent.AddOwner(_typeofThis); ////// Event reporting a key was pressed /// public event KeyEventHandler KeyDown { add { AddHandler(Keyboard.KeyDownEvent, value, false); } remove { RemoveHandler(Keyboard.KeyDownEvent, value); } } ////// Virtual method reporting a key was pressed /// protected virtual void OnKeyDown(KeyEventArgs e) {} ////// Alias to the Keyboard.PreviewKeyUpEvent. /// public static readonly RoutedEvent PreviewKeyUpEvent = Keyboard.PreviewKeyUpEvent.AddOwner(_typeofThis); ////// Event reporting a key was released /// public event KeyEventHandler PreviewKeyUp { add { AddHandler(Keyboard.PreviewKeyUpEvent, value, false); } remove { RemoveHandler(Keyboard.PreviewKeyUpEvent, value); } } ////// Virtual method reporting a key was released /// protected virtual void OnPreviewKeyUp(KeyEventArgs e) {} ////// Alias to the Keyboard.KeyUpEvent. /// public static readonly RoutedEvent KeyUpEvent = Keyboard.KeyUpEvent.AddOwner(_typeofThis); ////// Event reporting a key was released /// public event KeyEventHandler KeyUp { add { AddHandler(Keyboard.KeyUpEvent, value, false); } remove { RemoveHandler(Keyboard.KeyUpEvent, value); } } ////// Virtual method reporting a key was released /// protected virtual void OnKeyUp(KeyEventArgs e) {} ////// Alias to the Keyboard.PreviewGotKeyboardFocusEvent. /// public static readonly RoutedEvent PreviewGotKeyboardFocusEvent = Keyboard.PreviewGotKeyboardFocusEvent.AddOwner(_typeofThis); ////// Event reporting that the keyboard is focused on this element /// public event KeyboardFocusChangedEventHandler PreviewGotKeyboardFocus { add { AddHandler(Keyboard.PreviewGotKeyboardFocusEvent, value, false); } remove { RemoveHandler(Keyboard.PreviewGotKeyboardFocusEvent, value); } } ////// Virtual method reporting that the keyboard is focused on this element /// protected virtual void OnPreviewGotKeyboardFocus(KeyboardFocusChangedEventArgs e) {} ////// Alias to the Keyboard.GotKeyboardFocusEvent. /// public static readonly RoutedEvent GotKeyboardFocusEvent = Keyboard.GotKeyboardFocusEvent.AddOwner(_typeofThis); ////// Event reporting that the keyboard is focused on this element /// public event KeyboardFocusChangedEventHandler GotKeyboardFocus { add { AddHandler(Keyboard.GotKeyboardFocusEvent, value, false); } remove { RemoveHandler(Keyboard.GotKeyboardFocusEvent, value); } } ////// Virtual method reporting that the keyboard is focused on this element /// protected virtual void OnGotKeyboardFocus(KeyboardFocusChangedEventArgs e) {} ////// Alias to the Keyboard.PreviewLostKeyboardFocusEvent. /// public static readonly RoutedEvent PreviewLostKeyboardFocusEvent = Keyboard.PreviewLostKeyboardFocusEvent.AddOwner(_typeofThis); ////// Event reporting that the keyboard is no longer focusekeyboard is no longer focuseed /// public event KeyboardFocusChangedEventHandler PreviewLostKeyboardFocus { add { AddHandler(Keyboard.PreviewLostKeyboardFocusEvent, value, false); } remove { RemoveHandler(Keyboard.PreviewLostKeyboardFocusEvent, value); } } ////// Virtual method reporting that the keyboard is no longer focusekeyboard is no longer focuseed /// protected virtual void OnPreviewLostKeyboardFocus(KeyboardFocusChangedEventArgs e) {} ////// Alias to the Keyboard.LostKeyboardFocusEvent. /// public static readonly RoutedEvent LostKeyboardFocusEvent = Keyboard.LostKeyboardFocusEvent.AddOwner(_typeofThis); ////// Event reporting that the keyboard is no longer focusekeyboard is no longer focuseed /// public event KeyboardFocusChangedEventHandler LostKeyboardFocus { add { AddHandler(Keyboard.LostKeyboardFocusEvent, value, false); } remove { RemoveHandler(Keyboard.LostKeyboardFocusEvent, value); } } ////// Virtual method reporting that the keyboard is no longer focusekeyboard is no longer focuseed /// protected virtual void OnLostKeyboardFocus(KeyboardFocusChangedEventArgs e) {} ////// Alias to the TextCompositionManager.PreviewTextInputEvent. /// public static readonly RoutedEvent PreviewTextInputEvent = TextCompositionManager.PreviewTextInputEvent.AddOwner(_typeofThis); ////// Event reporting text composition /// public event TextCompositionEventHandler PreviewTextInput { add { AddHandler(TextCompositionManager.PreviewTextInputEvent, value, false); } remove { RemoveHandler(TextCompositionManager.PreviewTextInputEvent, value); } } ////// Virtual method reporting text composition /// protected virtual void OnPreviewTextInput(TextCompositionEventArgs e) {} ////// Alias to the TextCompositionManager.TextInputEvent. /// public static readonly RoutedEvent TextInputEvent = TextCompositionManager.TextInputEvent.AddOwner(_typeofThis); ////// Event reporting text composition /// public event TextCompositionEventHandler TextInput { add { AddHandler(TextCompositionManager.TextInputEvent, value, false); } remove { RemoveHandler(TextCompositionManager.TextInputEvent, value); } } ////// Virtual method reporting text composition /// protected virtual void OnTextInput(TextCompositionEventArgs e) {} ////// Alias to the DragDrop.PreviewQueryContinueDragEvent. /// public static readonly RoutedEvent PreviewQueryContinueDragEvent = DragDrop.PreviewQueryContinueDragEvent.AddOwner(_typeofThis); ////// Event reporting the preview query continue drag is going to happen /// public event QueryContinueDragEventHandler PreviewQueryContinueDrag { add { AddHandler(DragDrop.PreviewQueryContinueDragEvent, value, false); } remove { RemoveHandler(DragDrop.PreviewQueryContinueDragEvent, value); } } ////// Virtual method reporting the preview query continue drag is going to happen /// protected virtual void OnPreviewQueryContinueDrag(QueryContinueDragEventArgs e) {} ////// Alias to the DragDrop.QueryContinueDragEvent. /// public static readonly RoutedEvent QueryContinueDragEvent = DragDrop.QueryContinueDragEvent.AddOwner(_typeofThis); ////// Event reporting the query continue drag is going to happen /// public event QueryContinueDragEventHandler QueryContinueDrag { add { AddHandler(DragDrop.QueryContinueDragEvent, value, false); } remove { RemoveHandler(DragDrop.QueryContinueDragEvent, value); } } ////// Virtual method reporting the query continue drag is going to happen /// protected virtual void OnQueryContinueDrag(QueryContinueDragEventArgs e) {} ////// Alias to the DragDrop.PreviewGiveFeedbackEvent. /// public static readonly RoutedEvent PreviewGiveFeedbackEvent = DragDrop.PreviewGiveFeedbackEvent.AddOwner(_typeofThis); ////// Event reporting the preview give feedback is going to happen /// public event GiveFeedbackEventHandler PreviewGiveFeedback { add { AddHandler(DragDrop.PreviewGiveFeedbackEvent, value, false); } remove { RemoveHandler(DragDrop.PreviewGiveFeedbackEvent, value); } } ////// Virtual method reporting the preview give feedback is going to happen /// protected virtual void OnPreviewGiveFeedback(GiveFeedbackEventArgs e) {} ////// Alias to the DragDrop.GiveFeedbackEvent. /// public static readonly RoutedEvent GiveFeedbackEvent = DragDrop.GiveFeedbackEvent.AddOwner(_typeofThis); ////// Event reporting the give feedback is going to happen /// public event GiveFeedbackEventHandler GiveFeedback { add { AddHandler(DragDrop.GiveFeedbackEvent, value, false); } remove { RemoveHandler(DragDrop.GiveFeedbackEvent, value); } } ////// Virtual method reporting the give feedback is going to happen /// protected virtual void OnGiveFeedback(GiveFeedbackEventArgs e) {} ////// Alias to the DragDrop.PreviewDragEnterEvent. /// public static readonly RoutedEvent PreviewDragEnterEvent = DragDrop.PreviewDragEnterEvent.AddOwner(_typeofThis); ////// Event reporting the preview drag enter is going to happen /// public event DragEventHandler PreviewDragEnter { add { AddHandler(DragDrop.PreviewDragEnterEvent, value, false); } remove { RemoveHandler(DragDrop.PreviewDragEnterEvent, value); } } ////// Virtual method reporting the preview drag enter is going to happen /// protected virtual void OnPreviewDragEnter(DragEventArgs e) {} ////// Alias to the DragDrop.DragEnterEvent. /// public static readonly RoutedEvent DragEnterEvent = DragDrop.DragEnterEvent.AddOwner(_typeofThis); ////// Event reporting the drag enter is going to happen /// public event DragEventHandler DragEnter { add { AddHandler(DragDrop.DragEnterEvent, value, false); } remove { RemoveHandler(DragDrop.DragEnterEvent, value); } } ////// Virtual method reporting the drag enter is going to happen /// protected virtual void OnDragEnter(DragEventArgs e) {} ////// Alias to the DragDrop.PreviewDragOverEvent. /// public static readonly RoutedEvent PreviewDragOverEvent = DragDrop.PreviewDragOverEvent.AddOwner(_typeofThis); ////// Event reporting the preview drag over is going to happen /// public event DragEventHandler PreviewDragOver { add { AddHandler(DragDrop.PreviewDragOverEvent, value, false); } remove { RemoveHandler(DragDrop.PreviewDragOverEvent, value); } } ////// Virtual method reporting the preview drag over is going to happen /// protected virtual void OnPreviewDragOver(DragEventArgs e) {} ////// Alias to the DragDrop.DragOverEvent. /// public static readonly RoutedEvent DragOverEvent = DragDrop.DragOverEvent.AddOwner(_typeofThis); ////// Event reporting the drag over is going to happen /// public event DragEventHandler DragOver { add { AddHandler(DragDrop.DragOverEvent, value, false); } remove { RemoveHandler(DragDrop.DragOverEvent, value); } } ////// Virtual method reporting the drag over is going to happen /// protected virtual void OnDragOver(DragEventArgs e) {} ////// Alias to the DragDrop.PreviewDragLeaveEvent. /// public static readonly RoutedEvent PreviewDragLeaveEvent = DragDrop.PreviewDragLeaveEvent.AddOwner(_typeofThis); ////// Event reporting the preview drag leave is going to happen /// public event DragEventHandler PreviewDragLeave { add { AddHandler(DragDrop.PreviewDragLeaveEvent, value, false); } remove { RemoveHandler(DragDrop.PreviewDragLeaveEvent, value); } } ////// Virtual method reporting the preview drag leave is going to happen /// protected virtual void OnPreviewDragLeave(DragEventArgs e) {} ////// Alias to the DragDrop.DragLeaveEvent. /// public static readonly RoutedEvent DragLeaveEvent = DragDrop.DragLeaveEvent.AddOwner(_typeofThis); ////// Event reporting the drag leave is going to happen /// public event DragEventHandler DragLeave { add { AddHandler(DragDrop.DragLeaveEvent, value, false); } remove { RemoveHandler(DragDrop.DragLeaveEvent, value); } } ////// Virtual method reporting the drag leave is going to happen /// protected virtual void OnDragLeave(DragEventArgs e) {} ////// Alias to the DragDrop.PreviewDropEvent. /// public static readonly RoutedEvent PreviewDropEvent = DragDrop.PreviewDropEvent.AddOwner(_typeofThis); ////// Event reporting the preview drop is going to happen /// public event DragEventHandler PreviewDrop { add { AddHandler(DragDrop.PreviewDropEvent, value, false); } remove { RemoveHandler(DragDrop.PreviewDropEvent, value); } } ////// Virtual method reporting the preview drop is going to happen /// protected virtual void OnPreviewDrop(DragEventArgs e) {} ////// Alias to the DragDrop.DropEvent. /// public static readonly RoutedEvent DropEvent = DragDrop.DropEvent.AddOwner(_typeofThis); ////// Event reporting the drag enter is going to happen /// public event DragEventHandler Drop { add { AddHandler(DragDrop.DropEvent, value, false); } remove { RemoveHandler(DragDrop.DropEvent, value); } } ////// Virtual method reporting the drag enter is going to happen /// protected virtual void OnDrop(DragEventArgs e) {} ////// The key needed set a read-only property. /// internal static readonly DependencyPropertyKey IsMouseDirectlyOverPropertyKey = DependencyProperty.RegisterReadOnly( "IsMouseDirectlyOver", typeof(bool), _typeofThis, new PropertyMetadata( BooleanBoxes.FalseBox, // default value new PropertyChangedCallback(IsMouseDirectlyOver_Changed))); ////// The dependency property for the IsMouseDirectlyOver property. /// public static readonly DependencyProperty IsMouseDirectlyOverProperty = IsMouseDirectlyOverPropertyKey.DependencyProperty; private static void IsMouseDirectlyOver_Changed(DependencyObject d, DependencyPropertyChangedEventArgs e) { ((UIElement) d).RaiseIsMouseDirectlyOverChanged(e); } ////// IsMouseDirectlyOverChanged private key /// internal static readonly EventPrivateKey IsMouseDirectlyOverChangedKey = new EventPrivateKey(); ////// An event reporting that the IsMouseDirectlyOver property changed. /// public event DependencyPropertyChangedEventHandler IsMouseDirectlyOverChanged { add { EventHandlersStoreAdd(UIElement.IsMouseDirectlyOverChangedKey, value); } remove { EventHandlersStoreRemove(UIElement.IsMouseDirectlyOverChangedKey, value); } } ////// An event reporting that the IsMouseDirectlyOver property changed. /// protected virtual void OnIsMouseDirectlyOverChanged(DependencyPropertyChangedEventArgs e) { } private void RaiseIsMouseDirectlyOverChanged(DependencyPropertyChangedEventArgs args) { // Call the virtual method first. OnIsMouseDirectlyOverChanged(args); // Raise the public event second. RaiseDependencyPropertyChanged(UIElement.IsMouseDirectlyOverChangedKey, args); } ////// The key needed set a read-only property. /// internal static readonly DependencyPropertyKey IsMouseOverPropertyKey = DependencyProperty.RegisterReadOnly( "IsMouseOver", typeof(bool), _typeofThis, new PropertyMetadata( BooleanBoxes.FalseBox)); ////// The dependency property for the IsMouseOver property. /// public static readonly DependencyProperty IsMouseOverProperty = IsMouseOverPropertyKey.DependencyProperty; ////// The key needed set a read-only property. /// internal static readonly DependencyPropertyKey IsStylusOverPropertyKey = DependencyProperty.RegisterReadOnly( "IsStylusOver", typeof(bool), _typeofThis, new PropertyMetadata( BooleanBoxes.FalseBox)); ////// The dependency property for the IsStylusOver property. /// public static readonly DependencyProperty IsStylusOverProperty = IsStylusOverPropertyKey.DependencyProperty; ////// The key needed set a read-only property. /// internal static readonly DependencyPropertyKey IsKeyboardFocusWithinPropertyKey = DependencyProperty.RegisterReadOnly( "IsKeyboardFocusWithin", typeof(bool), _typeofThis, new PropertyMetadata( BooleanBoxes.FalseBox)); ////// The dependency property for the IsKeyboardFocusWithin property. /// public static readonly DependencyProperty IsKeyboardFocusWithinProperty = IsKeyboardFocusWithinPropertyKey.DependencyProperty; ////// IsKeyboardFocusWithinChanged private key /// internal static readonly EventPrivateKey IsKeyboardFocusWithinChangedKey = new EventPrivateKey(); ////// An event reporting that the IsKeyboardFocusWithin property changed. /// public event DependencyPropertyChangedEventHandler IsKeyboardFocusWithinChanged { add { EventHandlersStoreAdd(UIElement.IsKeyboardFocusWithinChangedKey, value); } remove { EventHandlersStoreRemove(UIElement.IsKeyboardFocusWithinChangedKey, value); } } ////// An event reporting that the IsKeyboardFocusWithin property changed. /// protected virtual void OnIsKeyboardFocusWithinChanged(DependencyPropertyChangedEventArgs e) { } internal void RaiseIsKeyboardFocusWithinChanged(DependencyPropertyChangedEventArgs args) { // Call the virtual method first. OnIsKeyboardFocusWithinChanged(args); // Raise the public event second. RaiseDependencyPropertyChanged(UIElement.IsKeyboardFocusWithinChangedKey, args); } ////// The key needed set a read-only property. /// internal static readonly DependencyPropertyKey IsMouseCapturedPropertyKey = DependencyProperty.RegisterReadOnly( "IsMouseCaptured", typeof(bool), _typeofThis, new PropertyMetadata( BooleanBoxes.FalseBox, // default value new PropertyChangedCallback(IsMouseCaptured_Changed))); ////// The dependency property for the IsMouseCaptured property. /// public static readonly DependencyProperty IsMouseCapturedProperty = IsMouseCapturedPropertyKey.DependencyProperty; private static void IsMouseCaptured_Changed(DependencyObject d, DependencyPropertyChangedEventArgs e) { ((UIElement) d).RaiseIsMouseCapturedChanged(e); } ////// IsMouseCapturedChanged private key /// internal static readonly EventPrivateKey IsMouseCapturedChangedKey = new EventPrivateKey(); ////// An event reporting that the IsMouseCaptured property changed. /// public event DependencyPropertyChangedEventHandler IsMouseCapturedChanged { add { EventHandlersStoreAdd(UIElement.IsMouseCapturedChangedKey, value); } remove { EventHandlersStoreRemove(UIElement.IsMouseCapturedChangedKey, value); } } ////// An event reporting that the IsMouseCaptured property changed. /// protected virtual void OnIsMouseCapturedChanged(DependencyPropertyChangedEventArgs e) { } private void RaiseIsMouseCapturedChanged(DependencyPropertyChangedEventArgs args) { // Call the virtual method first. OnIsMouseCapturedChanged(args); // Raise the public event second. RaiseDependencyPropertyChanged(UIElement.IsMouseCapturedChangedKey, args); } ////// The key needed set a read-only property. /// internal static readonly DependencyPropertyKey IsMouseCaptureWithinPropertyKey = DependencyProperty.RegisterReadOnly( "IsMouseCaptureWithin", typeof(bool), _typeofThis, new PropertyMetadata( BooleanBoxes.FalseBox)); ////// The dependency property for the IsMouseCaptureWithin property. /// public static readonly DependencyProperty IsMouseCaptureWithinProperty = IsMouseCaptureWithinPropertyKey.DependencyProperty; ////// IsMouseCaptureWithinChanged private key /// internal static readonly EventPrivateKey IsMouseCaptureWithinChangedKey = new EventPrivateKey(); ////// An event reporting that the IsMouseCaptureWithin property changed. /// public event DependencyPropertyChangedEventHandler IsMouseCaptureWithinChanged { add { EventHandlersStoreAdd(UIElement.IsMouseCaptureWithinChangedKey, value); } remove { EventHandlersStoreRemove(UIElement.IsMouseCaptureWithinChangedKey, value); } } ////// An event reporting that the IsMouseCaptureWithin property changed. /// protected virtual void OnIsMouseCaptureWithinChanged(DependencyPropertyChangedEventArgs e) { } internal void RaiseIsMouseCaptureWithinChanged(DependencyPropertyChangedEventArgs args) { // Call the virtual method first. OnIsMouseCaptureWithinChanged(args); // Raise the public event second. RaiseDependencyPropertyChanged(UIElement.IsMouseCaptureWithinChangedKey, args); } ////// The key needed set a read-only property. /// internal static readonly DependencyPropertyKey IsStylusDirectlyOverPropertyKey = DependencyProperty.RegisterReadOnly( "IsStylusDirectlyOver", typeof(bool), _typeofThis, new PropertyMetadata( BooleanBoxes.FalseBox, // default value new PropertyChangedCallback(IsStylusDirectlyOver_Changed))); ////// The dependency property for the IsStylusDirectlyOver property. /// public static readonly DependencyProperty IsStylusDirectlyOverProperty = IsStylusDirectlyOverPropertyKey.DependencyProperty; private static void IsStylusDirectlyOver_Changed(DependencyObject d, DependencyPropertyChangedEventArgs e) { ((UIElement) d).RaiseIsStylusDirectlyOverChanged(e); } ////// IsStylusDirectlyOverChanged private key /// internal static readonly EventPrivateKey IsStylusDirectlyOverChangedKey = new EventPrivateKey(); ////// An event reporting that the IsStylusDirectlyOver property changed. /// public event DependencyPropertyChangedEventHandler IsStylusDirectlyOverChanged { add { EventHandlersStoreAdd(UIElement.IsStylusDirectlyOverChangedKey, value); } remove { EventHandlersStoreRemove(UIElement.IsStylusDirectlyOverChangedKey, value); } } ////// An event reporting that the IsStylusDirectlyOver property changed. /// protected virtual void OnIsStylusDirectlyOverChanged(DependencyPropertyChangedEventArgs e) { } private void RaiseIsStylusDirectlyOverChanged(DependencyPropertyChangedEventArgs args) { // Call the virtual method first. OnIsStylusDirectlyOverChanged(args); // Raise the public event second. RaiseDependencyPropertyChanged(UIElement.IsStylusDirectlyOverChangedKey, args); } ////// The key needed set a read-only property. /// internal static readonly DependencyPropertyKey IsStylusCapturedPropertyKey = DependencyProperty.RegisterReadOnly( "IsStylusCaptured", typeof(bool), _typeofThis, new PropertyMetadata( BooleanBoxes.FalseBox, // default value new PropertyChangedCallback(IsStylusCaptured_Changed))); ////// The dependency property for the IsStylusCaptured property. /// public static readonly DependencyProperty IsStylusCapturedProperty = IsStylusCapturedPropertyKey.DependencyProperty; private static void IsStylusCaptured_Changed(DependencyObject d, DependencyPropertyChangedEventArgs e) { ((UIElement) d).RaiseIsStylusCapturedChanged(e); } ////// IsStylusCapturedChanged private key /// internal static readonly EventPrivateKey IsStylusCapturedChangedKey = new EventPrivateKey(); ////// An event reporting that the IsStylusCaptured property changed. /// public event DependencyPropertyChangedEventHandler IsStylusCapturedChanged { add { EventHandlersStoreAdd(UIElement.IsStylusCapturedChangedKey, value); } remove { EventHandlersStoreRemove(UIElement.IsStylusCapturedChangedKey, value); } } ////// An event reporting that the IsStylusCaptured property changed. /// protected virtual void OnIsStylusCapturedChanged(DependencyPropertyChangedEventArgs e) { } private void RaiseIsStylusCapturedChanged(DependencyPropertyChangedEventArgs args) { // Call the virtual method first. OnIsStylusCapturedChanged(args); // Raise the public event second. RaiseDependencyPropertyChanged(UIElement.IsStylusCapturedChangedKey, args); } ////// The key needed set a read-only property. /// internal static readonly DependencyPropertyKey IsStylusCaptureWithinPropertyKey = DependencyProperty.RegisterReadOnly( "IsStylusCaptureWithin", typeof(bool), _typeofThis, new PropertyMetadata( BooleanBoxes.FalseBox)); ////// The dependency property for the IsStylusCaptureWithin property. /// public static readonly DependencyProperty IsStylusCaptureWithinProperty = IsStylusCaptureWithinPropertyKey.DependencyProperty; ////// IsStylusCaptureWithinChanged private key /// internal static readonly EventPrivateKey IsStylusCaptureWithinChangedKey = new EventPrivateKey(); ////// An event reporting that the IsStylusCaptureWithin property changed. /// public event DependencyPropertyChangedEventHandler IsStylusCaptureWithinChanged { add { EventHandlersStoreAdd(UIElement.IsStylusCaptureWithinChangedKey, value); } remove { EventHandlersStoreRemove(UIElement.IsStylusCaptureWithinChangedKey, value); } } ////// An event reporting that the IsStylusCaptureWithin property changed. /// protected virtual void OnIsStylusCaptureWithinChanged(DependencyPropertyChangedEventArgs e) { } internal void RaiseIsStylusCaptureWithinChanged(DependencyPropertyChangedEventArgs args) { // Call the virtual method first. OnIsStylusCaptureWithinChanged(args); // Raise the public event second. RaiseDependencyPropertyChanged(UIElement.IsStylusCaptureWithinChangedKey, args); } ////// The key needed set a read-only property. /// internal static readonly DependencyPropertyKey IsKeyboardFocusedPropertyKey = DependencyProperty.RegisterReadOnly( "IsKeyboardFocused", typeof(bool), _typeofThis, new PropertyMetadata( BooleanBoxes.FalseBox, // default value new PropertyChangedCallback(IsKeyboardFocused_Changed))); ////// The dependency property for the IsKeyboardFocused property. /// public static readonly DependencyProperty IsKeyboardFocusedProperty = IsKeyboardFocusedPropertyKey.DependencyProperty; private static void IsKeyboardFocused_Changed(DependencyObject d, DependencyPropertyChangedEventArgs e) { ((UIElement) d).RaiseIsKeyboardFocusedChanged(e); } ////// IsKeyboardFocusedChanged private key /// internal static readonly EventPrivateKey IsKeyboardFocusedChangedKey = new EventPrivateKey(); ////// An event reporting that the IsKeyboardFocused property changed. /// public event DependencyPropertyChangedEventHandler IsKeyboardFocusedChanged { add { EventHandlersStoreAdd(UIElement.IsKeyboardFocusedChangedKey, value); } remove { EventHandlersStoreRemove(UIElement.IsKeyboardFocusedChangedKey, value); } } ////// An event reporting that the IsKeyboardFocused property changed. /// protected virtual void OnIsKeyboardFocusedChanged(DependencyPropertyChangedEventArgs e) { } private void RaiseIsKeyboardFocusedChanged(DependencyPropertyChangedEventArgs args) { // Call the virtual method first. OnIsKeyboardFocusedChanged(args); // Raise the public event second. RaiseDependencyPropertyChanged(UIElement.IsKeyboardFocusedChangedKey, args); } internal bool ReadFlag(CoreFlags field) { return (_flags & field) != 0; } internal void WriteFlag(CoreFlags field,bool value) { if (value) { _flags |= field; } else { _flags &= (~field); } } private CoreFlags _flags; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.ComponentModel; using System.Diagnostics; using System.Security; using System.Security.Permissions; using System.Windows.Input; using System.Windows.Media.Animation; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings namespace System.Windows { partial class UIElement : IAnimatable { static private readonly Type _typeofThis = typeof(UIElement); #region IAnimatable ////// Applies an AnimationClock to a DepencencyProperty which will /// replace the current animations on the property using the snapshot /// and replace HandoffBehavior. /// /// /// The DependencyProperty to animate. /// /// /// The AnimationClock that will animate the property. If this is null /// then all animations will be removed from the property. /// public void ApplyAnimationClock( DependencyProperty dp, AnimationClock clock) { ApplyAnimationClock(dp, clock, HandoffBehavior.SnapshotAndReplace); } ////// Applies an AnimationClock to a DependencyProperty. The effect of /// the new AnimationClock on any current animations will be determined by /// the value of the handoffBehavior parameter. /// /// /// The DependencyProperty to animate. /// /// /// The AnimationClock that will animate the property. If parameter is null /// then animations will be removed from the property if handoffBehavior is /// SnapshotAndReplace; otherwise the method call will have no result. /// /// /// Determines how the new AnimationClock will transition from or /// affect any current animations on the property. /// public void ApplyAnimationClock( DependencyProperty dp, AnimationClock clock, HandoffBehavior handoffBehavior) { if (dp == null) { throw new ArgumentNullException("dp"); } if (!AnimationStorage.IsPropertyAnimatable(this, dp)) { #pragma warning disable 56506 // Suppress presharp warning: Parameter 'dp' to this public method must be validated: A null-dereference can occur here. throw new ArgumentException(SR.Get(SRID.Animation_DependencyPropertyIsNotAnimatable, dp.Name, this.GetType()), "dp"); #pragma warning restore 56506 } if (clock != null && !AnimationStorage.IsAnimationValid(dp, clock.Timeline)) { #pragma warning disable 56506 // Suppress presharp warning: Parameter 'dp' to this public method must be validated: A null-dereference can occur here. throw new ArgumentException(SR.Get(SRID.Animation_AnimationTimelineTypeMismatch, clock.Timeline.GetType(), dp.Name, dp.PropertyType), "clock"); #pragma warning restore 56506 } if (!HandoffBehaviorEnum.IsDefined(handoffBehavior)) { throw new ArgumentException(SR.Get(SRID.Animation_UnrecognizedHandoffBehavior)); } if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.IAnimatable_CantAnimateSealedDO, dp, this.GetType())); } AnimationStorage.ApplyAnimationClock(this, dp, clock, handoffBehavior); } ////// Starts an animation for a DependencyProperty. The animation will /// begin when the next frame is rendered. /// /// /// The DependencyProperty to animate. /// /// ///The AnimationTimeline to used to animate the property. ///If the AnimationTimeline's BeginTime is null, any current animations /// will be removed and the current value of the property will be held. ///If this value is null, all animations will be removed from the property /// and the property value will revert back to its base value. /// public void BeginAnimation(DependencyProperty dp, AnimationTimeline animation) { BeginAnimation(dp, animation, HandoffBehavior.SnapshotAndReplace); } ////// Starts an animation for a DependencyProperty. The animation will /// begin when the next frame is rendered. /// /// /// The DependencyProperty to animate. /// /// ///The AnimationTimeline to used to animate the property. ///If the AnimationTimeline's BeginTime is null, any current animations /// will be removed and the current value of the property will be held. ///If this value is null, all animations will be removed from the property /// and the property value will revert back to its base value. /// /// /// Specifies how the new animation should interact with any current /// animations already affecting the property value. /// public void BeginAnimation(DependencyProperty dp, AnimationTimeline animation, HandoffBehavior handoffBehavior) { if (dp == null) { throw new ArgumentNullException("dp"); } if (!AnimationStorage.IsPropertyAnimatable(this, dp)) { #pragma warning disable 56506 // Suppress presharp warning: Parameter 'dp' to this public method must be validated: A null-dereference can occur here. throw new ArgumentException(SR.Get(SRID.Animation_DependencyPropertyIsNotAnimatable, dp.Name, this.GetType()), "dp"); #pragma warning restore 56506 } if ( animation != null && !AnimationStorage.IsAnimationValid(dp, animation)) { throw new ArgumentException(SR.Get(SRID.Animation_AnimationTimelineTypeMismatch, animation.GetType(), dp.Name, dp.PropertyType), "animation"); } if (!HandoffBehaviorEnum.IsDefined(handoffBehavior)) { throw new ArgumentException(SR.Get(SRID.Animation_UnrecognizedHandoffBehavior)); } if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.IAnimatable_CantAnimateSealedDO, dp, this.GetType())); } AnimationStorage.BeginAnimation(this, dp, animation, handoffBehavior); } ////// Returns true if any properties on this DependencyObject have a /// persistent animation or the object has one or more clocks associated /// with any of its properties. /// public bool HasAnimatedProperties { get { VerifyAccess(); return IAnimatable_HasAnimatedProperties; } } ////// If the dependency property is animated this method will /// give you the value as if it was not animated. /// /// The DependencyProperty ////// The value that would be returned if there were no /// animations attached. If there aren't any attached, then /// the result will be the same as that returned from /// GetValue. /// public object GetAnimationBaseValue(DependencyProperty dp) { if (dp == null) { throw new ArgumentNullException("dp"); } return this.GetValueEntry( LookupEntry(dp.GlobalIndex), dp, null, RequestFlags.AnimationBaseValue).Value; } #endregion IAnimatable #region Animation ////// Allows subclasses to participate in property animated value computation /// /// /// /// EffectiveValueEntry computed by base ////// Putting an InheritanceDemand as a defense-in-depth measure, /// as this provides a hook to the property system that we don't /// want exposed under PartialTrust. /// [UIPermissionAttribute(SecurityAction.InheritanceDemand, Window=UIPermissionWindow.AllWindows)] internal sealed override void EvaluateAnimatedValueCore( DependencyProperty dp, PropertyMetadata metadata, ref EffectiveValueEntry entry) { if (IAnimatable_HasAnimatedProperties) { AnimationStorage storage = AnimationStorage.GetStorage(this, dp); if (storage != null) { object value = entry.GetFlattenedEntry(RequestFlags.FullyResolved).Value; if (entry.IsDeferredReference) { DeferredReference dr = (DeferredReference)value; value = dr.GetValue(entry.BaseValueSourceInternal); // Set the baseValue back into the entry and clear the // IsDeferredReference flag entry.SetAnimationBaseValue(value); entry.IsDeferredReference = false; } object animatedValue = AnimationStorage.GetCurrentPropertyValue(storage, this, dp, metadata, value); entry.SetAnimatedValue(animatedValue, value); } } } #endregion Animation #region Commands ////// Instance level InputBinding collection, initialized on first use. /// To have commands handled (QueryEnabled/Execute) on an element instance, /// the user of this method can add InputBinding with handlers thru this /// method. /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public InputBindingCollection InputBindings { get { VerifyAccess(); InputBindingCollection bindings = InputBindingCollectionField.GetValue(this); if (bindings == null) { bindings = new InputBindingCollection(); InputBindingCollectionField.SetValue(this, bindings); } return bindings; } } // Used by CommandManager to avoid instantiating an empty collection internal InputBindingCollection InputBindingsInternal { get { VerifyAccess(); return InputBindingCollectionField.GetValue(this); } } ////// This method is used by TypeDescriptor to determine if this property should /// be serialized. /// // for serializer to serialize only when InputBindings is not empty [EditorBrowsable(EditorBrowsableState.Never)] public bool ShouldSerializeInputBindings() { InputBindingCollection bindingCollection = InputBindingCollectionField.GetValue(this); if (bindingCollection != null && bindingCollection.Count > 0) { return true; } return false; } ////// Instance level CommandBinding collection, initialized on first use. /// To have commands handled (QueryEnabled/Execute) on an element instance, /// the user of this method can add CommandBinding with handlers thru this /// method. /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public CommandBindingCollection CommandBindings { get { VerifyAccess(); CommandBindingCollection bindings = CommandBindingCollectionField.GetValue(this); if (bindings == null) { bindings = new CommandBindingCollection(); CommandBindingCollectionField.SetValue(this, bindings); } return bindings; } } // Used by CommandManager to avoid instantiating an empty collection internal CommandBindingCollection CommandBindingsInternal { get { VerifyAccess(); return CommandBindingCollectionField.GetValue(this); } } ////// This method is used by TypeDescriptor to determine if this property should /// be serialized. /// // for serializer to serialize only when CommandBindings is not empty [EditorBrowsable(EditorBrowsableState.Never)] public bool ShouldSerializeCommandBindings() { CommandBindingCollection bindingCollection = CommandBindingCollectionField.GetValue(this); if (bindingCollection != null && bindingCollection.Count > 0) { return true; } return false; } #endregion Commands #region Events ////// Allows UIElement to augment the /// ////// /// Sub-classes of UIElement can override /// this method to custom augment the route /// /// /// Theto be /// augmented /// /// /// for the /// RoutedEvent to be raised post building /// the route /// /// /// Whether or not the route should continue past the visual tree. /// If this is true, and there are no more visual parents, the route /// building code will call the GetUIParentCore method to find the /// next non-visual parent. /// internal virtual bool BuildRouteCore(EventRoute route, RoutedEventArgs args) { return false; } ////// Builds the /// /// The/// being /// built /// /// /// for the /// RoutedEvent to be raised post building /// the route /// internal void BuildRoute(EventRoute route, RoutedEventArgs args) { UIElement.BuildRouteHelper(this, route, args); } /// /// Raise the events specified by /// ////// /// This method is a shorthand for /// /// ///and /// /// for the event to /// be raised /// /// /// By default clears the user initiated bit. /// To guard against "replay" attacks. /// public void RaiseEvent(RoutedEventArgs e) { // VerifyAccess(); if (e == null) { throw new ArgumentNullException("e"); } e.ClearUserInitiated(); UIElement.RaiseEventImpl(this, e); } ////// "Trusted" internal flavor of RaiseEvent. /// Used to set the User-initated RaiseEvent. /// ////// Critical - sets the MarkAsUserInitiated bit. /// [SecurityCritical] internal void RaiseEvent(RoutedEventArgs args, bool trusted) { if (args == null) { throw new ArgumentNullException("args"); } if ( trusted ) { args.MarkAsUserInitiated(); } else { args.ClearUserInitiated(); } // Try/finally to ensure that UserInitiated bit is cleared. try { UIElement.RaiseEventImpl(this, args); } finally { // Clear the bit - just to guarantee it's not used again args.ClearUserInitiated(); } } ////// Allows adjustment to the event source /// ////// Subclasses must override this method /// to be able to adjust the source during /// route invocation /// /// Routed Event Args /// ////// /// NOTE: Expected to return null when no /// change is made to source /// /// Returns new source /// internal virtual object AdjustEventSource(RoutedEventArgs args) { return null; } ////// See overloaded method for details /// ////// handledEventsToo defaults to false /// /// public void AddHandler(RoutedEvent routedEvent, Delegate handler) { // HandledEventToo defaults to false // Call forwarded AddHandler(routedEvent, handler, false); } ////// See overloaded method for details /// /// Adds a routed event handler for the particular /// ////// /// The handler added thus is also known as /// an instance handler /// ////// /// /// NOTE: It is not an error to add a handler twice /// (handler will simply be called twice) /// /// /// Input parameters /// and handler cannot be null /// handledEventsToo input parameter when false means /// that listener does not care about already handled events. /// Hence the handler will not be invoked on the target if /// the RoutedEvent has already been /// /// handledEventsToo input parameter when true means /// that the listener wants to hear about all events even if /// they have already been handled. Hence the handler will /// be invoked irrespective of the event being /// /// for which the handler /// is attached /// /// /// The handler that will be invoked on this object /// when the RoutedEvent is raised /// /// /// Flag indicating whether or not the listener wants to /// hear about events that have already been handled /// public void AddHandler( RoutedEvent routedEvent, Delegate handler, bool handledEventsToo) { // VerifyAccess(); if (routedEvent == null) { throw new ArgumentNullException("routedEvent"); } if (handler == null) { throw new ArgumentNullException("handler"); } if (!routedEvent.IsLegalHandler(handler)) { throw new ArgumentException(SR.Get(SRID.HandlerTypeIllegal)); } EnsureEventHandlersStore(); EventHandlersStore.AddRoutedEventHandler(routedEvent, handler, handledEventsToo); OnAddHandler (routedEvent, handler); } /// /// Notifies subclass of a new routed event handler. Note that this is /// called once for each handler added, but OnRemoveHandler is only called /// on the last removal. /// internal virtual void OnAddHandler( RoutedEvent routedEvent, Delegate handler) { } ////// Removes all instances of the specified routed /// event handler for this object instance /// ////// The handler removed thus is also known as /// an instance handler /// ////// /// /// NOTE: This method does nothing if there were /// no handlers registered with the matching /// criteria /// /// /// Input parameters /// and handler cannot be null /// This method ignores the handledEventsToo criterion /// for which the handler /// is attached /// /// /// The handler for this object instance to be removed /// public void RemoveHandler(RoutedEvent routedEvent, Delegate handler) { // VerifyAccess(); if (routedEvent == null) { throw new ArgumentNullException("routedEvent"); } if (handler == null) { throw new ArgumentNullException("handler"); } if (!routedEvent.IsLegalHandler(handler)) { throw new ArgumentException(SR.Get(SRID.HandlerTypeIllegal)); } EventHandlersStore store = EventHandlersStore; if (store != null) { store.RemoveRoutedEventHandler(routedEvent, handler); OnRemoveHandler (routedEvent, handler); if (store.Count == 0) { // last event handler was removed -- throw away underlying EventHandlersStore EventHandlersStoreField.ClearValue(this); WriteFlag(CoreFlags.ExistsEventHandlersStore, false); } } } /// /// Notifies subclass of an event for which a handler has been removed. /// internal virtual void OnRemoveHandler( RoutedEvent routedEvent, Delegate handler) { } private void EventHandlersStoreAdd(EventPrivateKey key, Delegate handler) { EnsureEventHandlersStore(); EventHandlersStore.Add(key, handler); } private void EventHandlersStoreRemove(EventPrivateKey key, Delegate handler) { EventHandlersStore store = EventHandlersStore; if (store != null) { store.Remove(key, handler); if (store.Count == 0) { // last event handler was removed -- throw away underlying EventHandlersStore EventHandlersStoreField.ClearValue(this); WriteFlag(CoreFlags.ExistsEventHandlersStore, false); } } } ////// Add the event handlers for this element to the route. /// public void AddToEventRoute(EventRoute route, RoutedEventArgs e) { if (route == null) { throw new ArgumentNullException("route"); } if (e == null) { throw new ArgumentNullException("e"); } // Get class listeners for this UIElement RoutedEventHandlerInfoList classListeners = GlobalEventManager.GetDTypedClassListeners(this.DependencyObjectType, e.RoutedEvent); // Add all class listeners for this UIElement while (classListeners != null) { for(int i = 0; i < classListeners.Handlers.Length; i++) { route.Add(this, classListeners.Handlers[i].Handler, classListeners.Handlers[i].InvokeHandledEventsToo); } classListeners = classListeners.Next; } // Get instance listeners for this UIElement FrugalObjectListinstanceListeners = null; EventHandlersStore store = EventHandlersStore; if (store != null) { instanceListeners = store[e.RoutedEvent]; // Add all instance listeners for this UIElement if (instanceListeners != null) { for (int i = 0; i < instanceListeners.Count; i++) { route.Add(this, instanceListeners[i].Handler, instanceListeners[i].InvokeHandledEventsToo); } } } // Allow Framework to add event handlers in styles AddToEventRouteCore(route, e); } /// /// This virtual method is to be overridden in Framework /// to be able to add handlers for styles /// internal virtual void AddToEventRouteCore(EventRoute route, RoutedEventArgs args) { } ////// Event Handlers Store /// ////// The idea of exposing this property is to allow /// elements in the Framework to generically use /// EventHandlersStore for Clr events as well. /// internal EventHandlersStore EventHandlersStore { [FriendAccessAllowed] // Built into Core, also used by Framework. get { if(!ReadFlag(CoreFlags.ExistsEventHandlersStore)) { return null; } return EventHandlersStoreField.GetValue(this); } } ////// Ensures that EventHandlersStore will return /// non-null when it is called. /// [FriendAccessAllowed] // Built into Core, also used by Framework. internal void EnsureEventHandlersStore() { if (EventHandlersStore == null) { EventHandlersStoreField.SetValue(this, new EventHandlersStore()); WriteFlag(CoreFlags.ExistsEventHandlersStore, true); } } #endregion Events ////// Used by UIElement, ContentElement, and UIElement3D to register common Events. /// ////// Critical: This code is used to register various thunks that are used to send input to the tree /// TreatAsSafe: This code attaches handlers that are inside the class and private. Not configurable or overridable /// [SecurityCritical,SecurityTreatAsSafe] internal static void RegisterEvents(Type type) { EventManager.RegisterClassHandler(type, Mouse.PreviewMouseDownEvent, new MouseButtonEventHandler(UIElement.OnPreviewMouseDownThunk), true); EventManager.RegisterClassHandler(type, Mouse.MouseDownEvent, new MouseButtonEventHandler(UIElement.OnMouseDownThunk), true); EventManager.RegisterClassHandler(type, Mouse.PreviewMouseUpEvent, new MouseButtonEventHandler(UIElement.OnPreviewMouseUpThunk), true); EventManager.RegisterClassHandler(type, Mouse.MouseUpEvent, new MouseButtonEventHandler(UIElement.OnMouseUpThunk), true); EventManager.RegisterClassHandler(type, UIElement.PreviewMouseLeftButtonDownEvent, new MouseButtonEventHandler(UIElement.OnPreviewMouseLeftButtonDownThunk), false); EventManager.RegisterClassHandler(type, UIElement.MouseLeftButtonDownEvent, new MouseButtonEventHandler(UIElement.OnMouseLeftButtonDownThunk), false); EventManager.RegisterClassHandler(type, UIElement.PreviewMouseLeftButtonUpEvent, new MouseButtonEventHandler(UIElement.OnPreviewMouseLeftButtonUpThunk), false); EventManager.RegisterClassHandler(type, UIElement.MouseLeftButtonUpEvent, new MouseButtonEventHandler(UIElement.OnMouseLeftButtonUpThunk), false); EventManager.RegisterClassHandler(type, UIElement.PreviewMouseRightButtonDownEvent, new MouseButtonEventHandler(UIElement.OnPreviewMouseRightButtonDownThunk), false); EventManager.RegisterClassHandler(type, UIElement.MouseRightButtonDownEvent, new MouseButtonEventHandler(UIElement.OnMouseRightButtonDownThunk), false); EventManager.RegisterClassHandler(type, UIElement.PreviewMouseRightButtonUpEvent, new MouseButtonEventHandler(UIElement.OnPreviewMouseRightButtonUpThunk), false); EventManager.RegisterClassHandler(type, UIElement.MouseRightButtonUpEvent, new MouseButtonEventHandler(UIElement.OnMouseRightButtonUpThunk), false); EventManager.RegisterClassHandler(type, Mouse.PreviewMouseMoveEvent, new MouseEventHandler(UIElement.OnPreviewMouseMoveThunk), false); EventManager.RegisterClassHandler(type, Mouse.MouseMoveEvent, new MouseEventHandler(UIElement.OnMouseMoveThunk), false); EventManager.RegisterClassHandler(type, Mouse.PreviewMouseWheelEvent, new MouseWheelEventHandler(UIElement.OnPreviewMouseWheelThunk), false); EventManager.RegisterClassHandler(type, Mouse.MouseWheelEvent, new MouseWheelEventHandler(UIElement.OnMouseWheelThunk), false); EventManager.RegisterClassHandler(type, Mouse.MouseEnterEvent, new MouseEventHandler(UIElement.OnMouseEnterThunk), false); EventManager.RegisterClassHandler(type, Mouse.MouseLeaveEvent, new MouseEventHandler(UIElement.OnMouseLeaveThunk), false); EventManager.RegisterClassHandler(type, Mouse.GotMouseCaptureEvent, new MouseEventHandler(UIElement.OnGotMouseCaptureThunk), false); EventManager.RegisterClassHandler(type, Mouse.LostMouseCaptureEvent, new MouseEventHandler(UIElement.OnLostMouseCaptureThunk), false); EventManager.RegisterClassHandler(type, Mouse.QueryCursorEvent, new QueryCursorEventHandler(UIElement.OnQueryCursorThunk), false); EventManager.RegisterClassHandler(type, Stylus.PreviewStylusDownEvent, new StylusDownEventHandler(UIElement.OnPreviewStylusDownThunk), false); EventManager.RegisterClassHandler(type, Stylus.StylusDownEvent, new StylusDownEventHandler(UIElement.OnStylusDownThunk), false); EventManager.RegisterClassHandler(type, Stylus.PreviewStylusUpEvent, new StylusEventHandler(UIElement.OnPreviewStylusUpThunk), false); EventManager.RegisterClassHandler(type, Stylus.StylusUpEvent, new StylusEventHandler(UIElement.OnStylusUpThunk), false); EventManager.RegisterClassHandler(type, Stylus.PreviewStylusMoveEvent, new StylusEventHandler(UIElement.OnPreviewStylusMoveThunk), false); EventManager.RegisterClassHandler(type, Stylus.StylusMoveEvent, new StylusEventHandler(UIElement.OnStylusMoveThunk), false); EventManager.RegisterClassHandler(type, Stylus.PreviewStylusInAirMoveEvent, new StylusEventHandler(UIElement.OnPreviewStylusInAirMoveThunk), false); EventManager.RegisterClassHandler(type, Stylus.StylusInAirMoveEvent, new StylusEventHandler(UIElement.OnStylusInAirMoveThunk), false); EventManager.RegisterClassHandler(type, Stylus.StylusEnterEvent, new StylusEventHandler(UIElement.OnStylusEnterThunk), false); EventManager.RegisterClassHandler(type, Stylus.StylusLeaveEvent, new StylusEventHandler(UIElement.OnStylusLeaveThunk), false); EventManager.RegisterClassHandler(type, Stylus.PreviewStylusInRangeEvent, new StylusEventHandler(UIElement.OnPreviewStylusInRangeThunk), false); EventManager.RegisterClassHandler(type, Stylus.StylusInRangeEvent, new StylusEventHandler(UIElement.OnStylusInRangeThunk), false); EventManager.RegisterClassHandler(type, Stylus.PreviewStylusOutOfRangeEvent, new StylusEventHandler(UIElement.OnPreviewStylusOutOfRangeThunk), false); EventManager.RegisterClassHandler(type, Stylus.StylusOutOfRangeEvent, new StylusEventHandler(UIElement.OnStylusOutOfRangeThunk), false); EventManager.RegisterClassHandler(type, Stylus.PreviewStylusSystemGestureEvent, new StylusSystemGestureEventHandler(UIElement.OnPreviewStylusSystemGestureThunk), false); EventManager.RegisterClassHandler(type, Stylus.StylusSystemGestureEvent, new StylusSystemGestureEventHandler(UIElement.OnStylusSystemGestureThunk), false); EventManager.RegisterClassHandler(type, Stylus.GotStylusCaptureEvent, new StylusEventHandler(UIElement.OnGotStylusCaptureThunk), false); EventManager.RegisterClassHandler(type, Stylus.LostStylusCaptureEvent, new StylusEventHandler(UIElement.OnLostStylusCaptureThunk), false); EventManager.RegisterClassHandler(type, Stylus.StylusButtonDownEvent, new StylusButtonEventHandler(UIElement.OnStylusButtonDownThunk), false); EventManager.RegisterClassHandler(type, Stylus.StylusButtonUpEvent, new StylusButtonEventHandler(UIElement.OnStylusButtonUpThunk), false); EventManager.RegisterClassHandler(type, Stylus.PreviewStylusButtonDownEvent, new StylusButtonEventHandler(UIElement.OnPreviewStylusButtonDownThunk), false); EventManager.RegisterClassHandler(type, Stylus.PreviewStylusButtonUpEvent, new StylusButtonEventHandler(UIElement.OnPreviewStylusButtonUpThunk), false); EventManager.RegisterClassHandler(type, Keyboard.PreviewKeyDownEvent, new KeyEventHandler(UIElement.OnPreviewKeyDownThunk), false); EventManager.RegisterClassHandler(type, Keyboard.KeyDownEvent, new KeyEventHandler(UIElement.OnKeyDownThunk), false); EventManager.RegisterClassHandler(type, Keyboard.PreviewKeyUpEvent, new KeyEventHandler(UIElement.OnPreviewKeyUpThunk), false); EventManager.RegisterClassHandler(type, Keyboard.KeyUpEvent, new KeyEventHandler(UIElement.OnKeyUpThunk), false); EventManager.RegisterClassHandler(type, Keyboard.PreviewGotKeyboardFocusEvent, new KeyboardFocusChangedEventHandler(UIElement.OnPreviewGotKeyboardFocusThunk), false); EventManager.RegisterClassHandler(type, Keyboard.GotKeyboardFocusEvent, new KeyboardFocusChangedEventHandler(UIElement.OnGotKeyboardFocusThunk), false); EventManager.RegisterClassHandler(type, Keyboard.PreviewLostKeyboardFocusEvent, new KeyboardFocusChangedEventHandler(UIElement.OnPreviewLostKeyboardFocusThunk), false); EventManager.RegisterClassHandler(type, Keyboard.LostKeyboardFocusEvent, new KeyboardFocusChangedEventHandler(UIElement.OnLostKeyboardFocusThunk), false); EventManager.RegisterClassHandler(type, TextCompositionManager.PreviewTextInputEvent, new TextCompositionEventHandler(UIElement.OnPreviewTextInputThunk), false); EventManager.RegisterClassHandler(type, TextCompositionManager.TextInputEvent, new TextCompositionEventHandler(UIElement.OnTextInputThunk), false); EventManager.RegisterClassHandler(type, CommandManager.PreviewExecutedEvent, new ExecutedRoutedEventHandler(UIElement.OnPreviewExecutedThunk), false); EventManager.RegisterClassHandler(type, CommandManager.ExecutedEvent, new ExecutedRoutedEventHandler(UIElement.OnExecutedThunk), false); EventManager.RegisterClassHandler(type, CommandManager.PreviewCanExecuteEvent, new CanExecuteRoutedEventHandler(UIElement.OnPreviewCanExecuteThunk), false); EventManager.RegisterClassHandler(type, CommandManager.CanExecuteEvent, new CanExecuteRoutedEventHandler(UIElement.OnCanExecuteThunk), false); EventManager.RegisterClassHandler(type, CommandDevice.CommandDeviceEvent, new CommandDeviceEventHandler(UIElement.OnCommandDeviceThunk), false); EventManager.RegisterClassHandler(type, DragDrop.PreviewQueryContinueDragEvent, new QueryContinueDragEventHandler(UIElement.OnPreviewQueryContinueDragThunk), false); EventManager.RegisterClassHandler(type, DragDrop.QueryContinueDragEvent, new QueryContinueDragEventHandler(UIElement.OnQueryContinueDragThunk), false); EventManager.RegisterClassHandler(type, DragDrop.PreviewGiveFeedbackEvent, new GiveFeedbackEventHandler(UIElement.OnPreviewGiveFeedbackThunk), false); EventManager.RegisterClassHandler(type, DragDrop.GiveFeedbackEvent, new GiveFeedbackEventHandler(UIElement.OnGiveFeedbackThunk), false); EventManager.RegisterClassHandler(type, DragDrop.PreviewDragEnterEvent, new DragEventHandler(UIElement.OnPreviewDragEnterThunk), false); EventManager.RegisterClassHandler(type, DragDrop.DragEnterEvent, new DragEventHandler(UIElement.OnDragEnterThunk), false); EventManager.RegisterClassHandler(type, DragDrop.PreviewDragOverEvent, new DragEventHandler(UIElement.OnPreviewDragOverThunk), false); EventManager.RegisterClassHandler(type, DragDrop.DragOverEvent, new DragEventHandler(UIElement.OnDragOverThunk), false); EventManager.RegisterClassHandler(type, DragDrop.PreviewDragLeaveEvent, new DragEventHandler(UIElement.OnPreviewDragLeaveThunk), false); EventManager.RegisterClassHandler(type, DragDrop.DragLeaveEvent, new DragEventHandler(UIElement.OnDragLeaveThunk), false); EventManager.RegisterClassHandler(type, DragDrop.PreviewDropEvent, new DragEventHandler(UIElement.OnPreviewDropThunk), false); EventManager.RegisterClassHandler(type, DragDrop.DropEvent, new DragEventHandler(UIElement.OnDropThunk), false); } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewMouseDownThunk(object sender, MouseButtonEventArgs e) { if(!e.Handled) { UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewMouseDown(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewMouseDown(e); } else { ((UIElement3D)sender).OnPreviewMouseDown(e); } } } // Always raise this "sub-event", but we pass along the handledness. UIElement.CrackMouseButtonEventAndReRaiseEvent((DependencyObject)sender, e); } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnMouseDownThunk(object sender, MouseButtonEventArgs e) { if(!e.Handled) { CommandManager.TranslateInput((IInputElement)sender, e); } if(!e.Handled) { UIElement uie = sender as UIElement; if (uie != null) { uie.OnMouseDown(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnMouseDown(e); } else { ((UIElement3D)sender).OnMouseDown(e); } } } // Always raise this "sub-event", but we pass along the handledness. UIElement.CrackMouseButtonEventAndReRaiseEvent((DependencyObject)sender, e); } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewMouseUpThunk(object sender, MouseButtonEventArgs e) { if(!e.Handled) { UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewMouseUp(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewMouseUp(e); } else { ((UIElement3D)sender).OnPreviewMouseUp(e); } } } // Always raise this "sub-event", but we pass along the handledness. UIElement.CrackMouseButtonEventAndReRaiseEvent((DependencyObject)sender, e); } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnMouseUpThunk(object sender, MouseButtonEventArgs e) { if(!e.Handled) { UIElement uie = sender as UIElement; if (uie != null) { uie.OnMouseUp(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnMouseUp(e); } else { ((UIElement3D)sender).OnMouseUp(e); } } } // Always raise this "sub-event", but we pass along the handledness. UIElement.CrackMouseButtonEventAndReRaiseEvent((DependencyObject)sender, e); } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewMouseLeftButtonDownThunk(object sender, MouseButtonEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewMouseLeftButtonDown(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewMouseLeftButtonDown(e); } else { ((UIElement3D)sender).OnPreviewMouseLeftButtonDown(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnMouseLeftButtonDownThunk(object sender, MouseButtonEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnMouseLeftButtonDown(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnMouseLeftButtonDown(e); } else { ((UIElement3D)sender).OnMouseLeftButtonDown(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewMouseLeftButtonUpThunk(object sender, MouseButtonEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewMouseLeftButtonUp(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewMouseLeftButtonUp(e); } else { ((UIElement3D)sender).OnPreviewMouseLeftButtonUp(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnMouseLeftButtonUpThunk(object sender, MouseButtonEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnMouseLeftButtonUp(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnMouseLeftButtonUp(e); } else { ((UIElement3D)sender).OnMouseLeftButtonUp(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewMouseRightButtonDownThunk(object sender, MouseButtonEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewMouseRightButtonDown(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewMouseRightButtonDown(e); } else { ((UIElement3D)sender).OnPreviewMouseRightButtonDown(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnMouseRightButtonDownThunk(object sender, MouseButtonEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnMouseRightButtonDown(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnMouseRightButtonDown(e); } else { ((UIElement3D)sender).OnMouseRightButtonDown(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewMouseRightButtonUpThunk(object sender, MouseButtonEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewMouseRightButtonUp(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewMouseRightButtonUp(e); } else { ((UIElement3D)sender).OnPreviewMouseRightButtonUp(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnMouseRightButtonUpThunk(object sender, MouseButtonEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnMouseRightButtonUp(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnMouseRightButtonUp(e); } else { ((UIElement3D)sender).OnMouseRightButtonUp(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewMouseMoveThunk(object sender, MouseEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewMouseMove(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewMouseMove(e); } else { ((UIElement3D)sender).OnPreviewMouseMove(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnMouseMoveThunk(object sender, MouseEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnMouseMove(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnMouseMove(e); } else { ((UIElement3D)sender).OnMouseMove(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewMouseWheelThunk(object sender, MouseWheelEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewMouseWheel(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewMouseWheel(e); } else { ((UIElement3D)sender).OnPreviewMouseWheel(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnMouseWheelThunk(object sender, MouseWheelEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); CommandManager.TranslateInput((IInputElement)sender, e); if(!e.Handled) { UIElement uie = sender as UIElement; if (uie != null) { uie.OnMouseWheel(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnMouseWheel(e); } else { ((UIElement3D)sender).OnMouseWheel(e); } } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnMouseEnterThunk(object sender, MouseEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnMouseEnter(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnMouseEnter(e); } else { ((UIElement3D)sender).OnMouseEnter(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnMouseLeaveThunk(object sender, MouseEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnMouseLeave(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnMouseLeave(e); } else { ((UIElement3D)sender).OnMouseLeave(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnGotMouseCaptureThunk(object sender, MouseEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnGotMouseCapture(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnGotMouseCapture(e); } else { ((UIElement3D)sender).OnGotMouseCapture(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnLostMouseCaptureThunk(object sender, MouseEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnLostMouseCapture(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnLostMouseCapture(e); } else { ((UIElement3D)sender).OnLostMouseCapture(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnQueryCursorThunk(object sender, QueryCursorEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnQueryCursor(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnQueryCursor(e); } else { ((UIElement3D)sender).OnQueryCursor(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewStylusDownThunk(object sender, StylusDownEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewStylusDown(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewStylusDown(e); } else { ((UIElement3D)sender).OnPreviewStylusDown(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnStylusDownThunk(object sender, StylusDownEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnStylusDown(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnStylusDown(e); } else { ((UIElement3D)sender).OnStylusDown(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewStylusUpThunk(object sender, StylusEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewStylusUp(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewStylusUp(e); } else { ((UIElement3D)sender).OnPreviewStylusUp(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnStylusUpThunk(object sender, StylusEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnStylusUp(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnStylusUp(e); } else { ((UIElement3D)sender).OnStylusUp(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewStylusMoveThunk(object sender, StylusEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewStylusMove(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewStylusMove(e); } else { ((UIElement3D)sender).OnPreviewStylusMove(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnStylusMoveThunk(object sender, StylusEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnStylusMove(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnStylusMove(e); } else { ((UIElement3D)sender).OnStylusMove(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewStylusInAirMoveThunk(object sender, StylusEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewStylusInAirMove(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewStylusInAirMove(e); } else { ((UIElement3D)sender).OnPreviewStylusInAirMove(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnStylusInAirMoveThunk(object sender, StylusEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnStylusInAirMove(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnStylusInAirMove(e); } else { ((UIElement3D)sender).OnStylusInAirMove(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnStylusEnterThunk(object sender, StylusEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnStylusEnter(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnStylusEnter(e); } else { ((UIElement3D)sender).OnStylusEnter(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnStylusLeaveThunk(object sender, StylusEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnStylusLeave(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnStylusLeave(e); } else { ((UIElement3D)sender).OnStylusLeave(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewStylusInRangeThunk(object sender, StylusEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewStylusInRange(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewStylusInRange(e); } else { ((UIElement3D)sender).OnPreviewStylusInRange(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnStylusInRangeThunk(object sender, StylusEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnStylusInRange(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnStylusInRange(e); } else { ((UIElement3D)sender).OnStylusInRange(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewStylusOutOfRangeThunk(object sender, StylusEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewStylusOutOfRange(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewStylusOutOfRange(e); } else { ((UIElement3D)sender).OnPreviewStylusOutOfRange(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnStylusOutOfRangeThunk(object sender, StylusEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnStylusOutOfRange(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnStylusOutOfRange(e); } else { ((UIElement3D)sender).OnStylusOutOfRange(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewStylusSystemGestureThunk(object sender, StylusSystemGestureEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewStylusSystemGesture(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewStylusSystemGesture(e); } else { ((UIElement3D)sender).OnPreviewStylusSystemGesture(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnStylusSystemGestureThunk(object sender, StylusSystemGestureEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnStylusSystemGesture(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnStylusSystemGesture(e); } else { ((UIElement3D)sender).OnStylusSystemGesture(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnGotStylusCaptureThunk(object sender, StylusEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnGotStylusCapture(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnGotStylusCapture(e); } else { ((UIElement3D)sender).OnGotStylusCapture(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnLostStylusCaptureThunk(object sender, StylusEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnLostStylusCapture(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnLostStylusCapture(e); } else { ((UIElement3D)sender).OnLostStylusCapture(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnStylusButtonDownThunk(object sender, StylusButtonEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnStylusButtonDown(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnStylusButtonDown(e); } else { ((UIElement3D)sender).OnStylusButtonDown(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnStylusButtonUpThunk(object sender, StylusButtonEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnStylusButtonUp(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnStylusButtonUp(e); } else { ((UIElement3D)sender).OnStylusButtonUp(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewStylusButtonDownThunk(object sender, StylusButtonEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewStylusButtonDown(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewStylusButtonDown(e); } else { ((UIElement3D)sender).OnPreviewStylusButtonDown(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewStylusButtonUpThunk(object sender, StylusButtonEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewStylusButtonUp(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewStylusButtonUp(e); } else { ((UIElement3D)sender).OnPreviewStylusButtonUp(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewKeyDownThunk(object sender, KeyEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewKeyDown(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewKeyDown(e); } else { ((UIElement3D)sender).OnPreviewKeyDown(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnKeyDownThunk(object sender, KeyEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); CommandManager.TranslateInput((IInputElement)sender, e); if(!e.Handled) { UIElement uie = sender as UIElement; if (uie != null) { uie.OnKeyDown(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnKeyDown(e); } else { ((UIElement3D)sender).OnKeyDown(e); } } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewKeyUpThunk(object sender, KeyEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewKeyUp(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewKeyUp(e); } else { ((UIElement3D)sender).OnPreviewKeyUp(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnKeyUpThunk(object sender, KeyEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnKeyUp(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnKeyUp(e); } else { ((UIElement3D)sender).OnKeyUp(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewGotKeyboardFocusThunk(object sender, KeyboardFocusChangedEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewGotKeyboardFocus(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewGotKeyboardFocus(e); } else { ((UIElement3D)sender).OnPreviewGotKeyboardFocus(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnGotKeyboardFocusThunk(object sender, KeyboardFocusChangedEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnGotKeyboardFocus(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnGotKeyboardFocus(e); } else { ((UIElement3D)sender).OnGotKeyboardFocus(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewLostKeyboardFocusThunk(object sender, KeyboardFocusChangedEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewLostKeyboardFocus(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewLostKeyboardFocus(e); } else { ((UIElement3D)sender).OnPreviewLostKeyboardFocus(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnLostKeyboardFocusThunk(object sender, KeyboardFocusChangedEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnLostKeyboardFocus(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnLostKeyboardFocus(e); } else { ((UIElement3D)sender).OnLostKeyboardFocus(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewTextInputThunk(object sender, TextCompositionEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewTextInput(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewTextInput(e); } else { ((UIElement3D)sender).OnPreviewTextInput(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnTextInputThunk(object sender, TextCompositionEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnTextInput(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnTextInput(e); } else { ((UIElement3D)sender).OnTextInput(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewExecutedThunk(object sender, ExecutedRoutedEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); // Command Manager will determine if preview or regular event. CommandManager.OnExecuted(sender, e); } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnExecutedThunk(object sender, ExecutedRoutedEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); // Command Manager will determine if preview or regular event. CommandManager.OnExecuted(sender, e); } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewCanExecuteThunk(object sender, CanExecuteRoutedEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); // Command Manager will determine if preview or regular event. CommandManager.OnCanExecute(sender, e); } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnCanExecuteThunk(object sender, CanExecuteRoutedEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); // Command Manager will determine if preview or regular event. CommandManager.OnCanExecute(sender, e); } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnCommandDeviceThunk(object sender, CommandDeviceEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); // Command Manager will determine if preview or regular event. CommandManager.OnCommandDevice(sender, e); } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewQueryContinueDragThunk(object sender, QueryContinueDragEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewQueryContinueDrag(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewQueryContinueDrag(e); } else { ((UIElement3D)sender).OnPreviewQueryContinueDrag(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnQueryContinueDragThunk(object sender, QueryContinueDragEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnQueryContinueDrag(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnQueryContinueDrag(e); } else { ((UIElement3D)sender).OnQueryContinueDrag(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewGiveFeedbackThunk(object sender, GiveFeedbackEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewGiveFeedback(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewGiveFeedback(e); } else { ((UIElement3D)sender).OnPreviewGiveFeedback(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnGiveFeedbackThunk(object sender, GiveFeedbackEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnGiveFeedback(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnGiveFeedback(e); } else { ((UIElement3D)sender).OnGiveFeedback(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewDragEnterThunk(object sender, DragEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewDragEnter(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewDragEnter(e); } else { ((UIElement3D)sender).OnPreviewDragEnter(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnDragEnterThunk(object sender, DragEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnDragEnter(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnDragEnter(e); } else { ((UIElement3D)sender).OnDragEnter(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewDragOverThunk(object sender, DragEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewDragOver(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewDragOver(e); } else { ((UIElement3D)sender).OnPreviewDragOver(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnDragOverThunk(object sender, DragEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnDragOver(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnDragOver(e); } else { ((UIElement3D)sender).OnDragOver(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewDragLeaveThunk(object sender, DragEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewDragLeave(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewDragLeave(e); } else { ((UIElement3D)sender).OnPreviewDragLeave(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnDragLeaveThunk(object sender, DragEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnDragLeave(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnDragLeave(e); } else { ((UIElement3D)sender).OnDragLeave(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnPreviewDropThunk(object sender, DragEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnPreviewDrop(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnPreviewDrop(e); } else { ((UIElement3D)sender).OnPreviewDrop(e); } } } ////// Critical: This code can be used to spoof input /// [SecurityCritical] private static void OnDropThunk(object sender, DragEventArgs e) { Invariant.Assert(!e.Handled, "Unexpected: Event has already been handled."); UIElement uie = sender as UIElement; if (uie != null) { uie.OnDrop(e); } else { ContentElement ce = sender as ContentElement; if (ce != null) { ce.OnDrop(e); } else { ((UIElement3D)sender).OnDrop(e); } } } ////// Alias to the Mouse.PreviewMouseDownEvent. /// public static readonly RoutedEvent PreviewMouseDownEvent = Mouse.PreviewMouseDownEvent.AddOwner(_typeofThis); ////// Event reporting the mouse button was pressed /// public event MouseButtonEventHandler PreviewMouseDown { add { AddHandler(Mouse.PreviewMouseDownEvent, value, false); } remove { RemoveHandler(Mouse.PreviewMouseDownEvent, value); } } ////// Virtual method reporting the mouse button was pressed /// protected virtual void OnPreviewMouseDown(MouseButtonEventArgs e) {} ////// Alias to the Mouse.MouseDownEvent. /// public static readonly RoutedEvent MouseDownEvent = Mouse.MouseDownEvent.AddOwner(_typeofThis); ////// Event reporting the mouse button was pressed /// public event MouseButtonEventHandler MouseDown { add { AddHandler(Mouse.MouseDownEvent, value, false); } remove { RemoveHandler(Mouse.MouseDownEvent, value); } } ////// Virtual method reporting the mouse button was pressed /// protected virtual void OnMouseDown(MouseButtonEventArgs e) {} ////// Alias to the Mouse.PreviewMouseUpEvent. /// public static readonly RoutedEvent PreviewMouseUpEvent = Mouse.PreviewMouseUpEvent.AddOwner(_typeofThis); ////// Event reporting the mouse button was released /// public event MouseButtonEventHandler PreviewMouseUp { add { AddHandler(Mouse.PreviewMouseUpEvent, value, false); } remove { RemoveHandler(Mouse.PreviewMouseUpEvent, value); } } ////// Virtual method reporting the mouse button was released /// protected virtual void OnPreviewMouseUp(MouseButtonEventArgs e) {} ////// Alias to the Mouse.MouseUpEvent. /// public static readonly RoutedEvent MouseUpEvent = Mouse.MouseUpEvent.AddOwner(_typeofThis); ////// Event reporting the mouse button was released /// public event MouseButtonEventHandler MouseUp { add { AddHandler(Mouse.MouseUpEvent, value, false); } remove { RemoveHandler(Mouse.MouseUpEvent, value); } } ////// Virtual method reporting the mouse button was released /// protected virtual void OnMouseUp(MouseButtonEventArgs e) {} ////// Declaration of the routed event reporting the left mouse button was pressed /// public static readonly RoutedEvent PreviewMouseLeftButtonDownEvent = EventManager.RegisterRoutedEvent("PreviewMouseLeftButtonDown", RoutingStrategy.Direct, typeof(MouseButtonEventHandler), _typeofThis); ////// Event reporting the left mouse button was pressed /// public event MouseButtonEventHandler PreviewMouseLeftButtonDown { add { AddHandler(UIElement.PreviewMouseLeftButtonDownEvent, value, false); } remove { RemoveHandler(UIElement.PreviewMouseLeftButtonDownEvent, value); } } ////// Virtual method reporting the left mouse button was pressed /// protected virtual void OnPreviewMouseLeftButtonDown(MouseButtonEventArgs e) {} ////// Declaration of the routed event reporting the left mouse button was pressed /// public static readonly RoutedEvent MouseLeftButtonDownEvent = EventManager.RegisterRoutedEvent("MouseLeftButtonDown", RoutingStrategy.Direct, typeof(MouseButtonEventHandler), _typeofThis); ////// Event reporting the left mouse button was pressed /// public event MouseButtonEventHandler MouseLeftButtonDown { add { AddHandler(UIElement.MouseLeftButtonDownEvent, value, false); } remove { RemoveHandler(UIElement.MouseLeftButtonDownEvent, value); } } ////// Virtual method reporting the left mouse button was pressed /// protected virtual void OnMouseLeftButtonDown(MouseButtonEventArgs e) {} ////// Declaration of the routed event reporting the left mouse button was released /// public static readonly RoutedEvent PreviewMouseLeftButtonUpEvent = EventManager.RegisterRoutedEvent("PreviewMouseLeftButtonUp", RoutingStrategy.Direct, typeof(MouseButtonEventHandler), _typeofThis); ////// Event reporting the left mouse button was released /// public event MouseButtonEventHandler PreviewMouseLeftButtonUp { add { AddHandler(UIElement.PreviewMouseLeftButtonUpEvent, value, false); } remove { RemoveHandler(UIElement.PreviewMouseLeftButtonUpEvent, value); } } ////// Virtual method reporting the left mouse button was released /// protected virtual void OnPreviewMouseLeftButtonUp(MouseButtonEventArgs e) {} ////// Declaration of the routed event reporting the left mouse button was released /// public static readonly RoutedEvent MouseLeftButtonUpEvent = EventManager.RegisterRoutedEvent("MouseLeftButtonUp", RoutingStrategy.Direct, typeof(MouseButtonEventHandler), _typeofThis); ////// Event reporting the left mouse button was released /// public event MouseButtonEventHandler MouseLeftButtonUp { add { AddHandler(UIElement.MouseLeftButtonUpEvent, value, false); } remove { RemoveHandler(UIElement.MouseLeftButtonUpEvent, value); } } ////// Virtual method reporting the left mouse button was released /// protected virtual void OnMouseLeftButtonUp(MouseButtonEventArgs e) {} ////// Declaration of the routed event reporting the right mouse button was pressed /// public static readonly RoutedEvent PreviewMouseRightButtonDownEvent = EventManager.RegisterRoutedEvent("PreviewMouseRightButtonDown", RoutingStrategy.Direct, typeof(MouseButtonEventHandler), _typeofThis); ////// Event reporting the right mouse button was pressed /// public event MouseButtonEventHandler PreviewMouseRightButtonDown { add { AddHandler(UIElement.PreviewMouseRightButtonDownEvent, value, false); } remove { RemoveHandler(UIElement.PreviewMouseRightButtonDownEvent, value); } } ////// Virtual method reporting the right mouse button was pressed /// protected virtual void OnPreviewMouseRightButtonDown(MouseButtonEventArgs e) {} ////// Declaration of the routed event reporting the right mouse button was pressed /// public static readonly RoutedEvent MouseRightButtonDownEvent = EventManager.RegisterRoutedEvent("MouseRightButtonDown", RoutingStrategy.Direct, typeof(MouseButtonEventHandler), _typeofThis); ////// Event reporting the right mouse button was pressed /// public event MouseButtonEventHandler MouseRightButtonDown { add { AddHandler(UIElement.MouseRightButtonDownEvent, value, false); } remove { RemoveHandler(UIElement.MouseRightButtonDownEvent, value); } } ////// Virtual method reporting the right mouse button was pressed /// protected virtual void OnMouseRightButtonDown(MouseButtonEventArgs e) {} ////// Declaration of the routed event reporting the right mouse button was released /// public static readonly RoutedEvent PreviewMouseRightButtonUpEvent = EventManager.RegisterRoutedEvent("PreviewMouseRightButtonUp", RoutingStrategy.Direct, typeof(MouseButtonEventHandler), _typeofThis); ////// Event reporting the right mouse button was released /// public event MouseButtonEventHandler PreviewMouseRightButtonUp { add { AddHandler(UIElement.PreviewMouseRightButtonUpEvent, value, false); } remove { RemoveHandler(UIElement.PreviewMouseRightButtonUpEvent, value); } } ////// Virtual method reporting the right mouse button was released /// protected virtual void OnPreviewMouseRightButtonUp(MouseButtonEventArgs e) {} ////// Declaration of the routed event reporting the right mouse button was released /// public static readonly RoutedEvent MouseRightButtonUpEvent = EventManager.RegisterRoutedEvent("MouseRightButtonUp", RoutingStrategy.Direct, typeof(MouseButtonEventHandler), _typeofThis); ////// Event reporting the right mouse button was released /// public event MouseButtonEventHandler MouseRightButtonUp { add { AddHandler(UIElement.MouseRightButtonUpEvent, value, false); } remove { RemoveHandler(UIElement.MouseRightButtonUpEvent, value); } } ////// Virtual method reporting the right mouse button was released /// protected virtual void OnMouseRightButtonUp(MouseButtonEventArgs e) {} ////// Alias to the Mouse.PreviewMouseMoveEvent. /// public static readonly RoutedEvent PreviewMouseMoveEvent = Mouse.PreviewMouseMoveEvent.AddOwner(_typeofThis); ////// Event reporting a mouse move /// public event MouseEventHandler PreviewMouseMove { add { AddHandler(Mouse.PreviewMouseMoveEvent, value, false); } remove { RemoveHandler(Mouse.PreviewMouseMoveEvent, value); } } ////// Virtual method reporting a mouse move /// protected virtual void OnPreviewMouseMove(MouseEventArgs e) {} ////// Alias to the Mouse.MouseMoveEvent. /// public static readonly RoutedEvent MouseMoveEvent = Mouse.MouseMoveEvent.AddOwner(_typeofThis); ////// Event reporting a mouse move /// public event MouseEventHandler MouseMove { add { AddHandler(Mouse.MouseMoveEvent, value, false); } remove { RemoveHandler(Mouse.MouseMoveEvent, value); } } ////// Virtual method reporting a mouse move /// protected virtual void OnMouseMove(MouseEventArgs e) {} ////// Alias to the Mouse.PreviewMouseWheelEvent. /// public static readonly RoutedEvent PreviewMouseWheelEvent = Mouse.PreviewMouseWheelEvent.AddOwner(_typeofThis); ////// Event reporting a mouse wheel rotation /// public event MouseWheelEventHandler PreviewMouseWheel { add { AddHandler(Mouse.PreviewMouseWheelEvent, value, false); } remove { RemoveHandler(Mouse.PreviewMouseWheelEvent, value); } } ////// Virtual method reporting a mouse wheel rotation /// protected virtual void OnPreviewMouseWheel(MouseWheelEventArgs e) {} ////// Alias to the Mouse.MouseWheelEvent. /// public static readonly RoutedEvent MouseWheelEvent = Mouse.MouseWheelEvent.AddOwner(_typeofThis); ////// Event reporting a mouse wheel rotation /// public event MouseWheelEventHandler MouseWheel { add { AddHandler(Mouse.MouseWheelEvent, value, false); } remove { RemoveHandler(Mouse.MouseWheelEvent, value); } } ////// Virtual method reporting a mouse wheel rotation /// protected virtual void OnMouseWheel(MouseWheelEventArgs e) {} ////// Alias to the Mouse.MouseEnterEvent. /// public static readonly RoutedEvent MouseEnterEvent = Mouse.MouseEnterEvent.AddOwner(_typeofThis); ////// Event reporting the mouse entered this element /// public event MouseEventHandler MouseEnter { add { AddHandler(Mouse.MouseEnterEvent, value, false); } remove { RemoveHandler(Mouse.MouseEnterEvent, value); } } ////// Virtual method reporting the mouse entered this element /// protected virtual void OnMouseEnter(MouseEventArgs e) {} ////// Alias to the Mouse.MouseLeaveEvent. /// public static readonly RoutedEvent MouseLeaveEvent = Mouse.MouseLeaveEvent.AddOwner(_typeofThis); ////// Event reporting the mouse left this element /// public event MouseEventHandler MouseLeave { add { AddHandler(Mouse.MouseLeaveEvent, value, false); } remove { RemoveHandler(Mouse.MouseLeaveEvent, value); } } ////// Virtual method reporting the mouse left this element /// protected virtual void OnMouseLeave(MouseEventArgs e) {} ////// Alias to the Mouse.GotMouseCaptureEvent. /// public static readonly RoutedEvent GotMouseCaptureEvent = Mouse.GotMouseCaptureEvent.AddOwner(_typeofThis); ////// Event reporting that this element got the mouse capture /// public event MouseEventHandler GotMouseCapture { add { AddHandler(Mouse.GotMouseCaptureEvent, value, false); } remove { RemoveHandler(Mouse.GotMouseCaptureEvent, value); } } ////// Virtual method reporting that this element got the mouse capture /// protected virtual void OnGotMouseCapture(MouseEventArgs e) {} ////// Alias to the Mouse.LostMouseCaptureEvent. /// public static readonly RoutedEvent LostMouseCaptureEvent = Mouse.LostMouseCaptureEvent.AddOwner(_typeofThis); ////// Event reporting that this element lost the mouse capture /// public event MouseEventHandler LostMouseCapture { add { AddHandler(Mouse.LostMouseCaptureEvent, value, false); } remove { RemoveHandler(Mouse.LostMouseCaptureEvent, value); } } ////// Virtual method reporting that this element lost the mouse capture /// protected virtual void OnLostMouseCapture(MouseEventArgs e) {} ////// Alias to the Mouse.QueryCursorEvent. /// public static readonly RoutedEvent QueryCursorEvent = Mouse.QueryCursorEvent.AddOwner(_typeofThis); ////// Event reporting the cursor to display was requested /// public event QueryCursorEventHandler QueryCursor { add { AddHandler(Mouse.QueryCursorEvent, value, false); } remove { RemoveHandler(Mouse.QueryCursorEvent, value); } } ////// Virtual method reporting the cursor to display was requested /// protected virtual void OnQueryCursor(QueryCursorEventArgs e) {} ////// Alias to the Stylus.PreviewStylusDownEvent. /// public static readonly RoutedEvent PreviewStylusDownEvent = Stylus.PreviewStylusDownEvent.AddOwner(_typeofThis); ////// Event reporting a stylus-down /// public event StylusDownEventHandler PreviewStylusDown { add { AddHandler(Stylus.PreviewStylusDownEvent, value, false); } remove { RemoveHandler(Stylus.PreviewStylusDownEvent, value); } } ////// Virtual method reporting a stylus-down /// protected virtual void OnPreviewStylusDown(StylusDownEventArgs e) {} ////// Alias to the Stylus.StylusDownEvent. /// public static readonly RoutedEvent StylusDownEvent = Stylus.StylusDownEvent.AddOwner(_typeofThis); ////// Event reporting a stylus-down /// public event StylusDownEventHandler StylusDown { add { AddHandler(Stylus.StylusDownEvent, value, false); } remove { RemoveHandler(Stylus.StylusDownEvent, value); } } ////// Virtual method reporting a stylus-down /// protected virtual void OnStylusDown(StylusDownEventArgs e) {} ////// Alias to the Stylus.PreviewStylusUpEvent. /// public static readonly RoutedEvent PreviewStylusUpEvent = Stylus.PreviewStylusUpEvent.AddOwner(_typeofThis); ////// Event reporting a stylus-up /// public event StylusEventHandler PreviewStylusUp { add { AddHandler(Stylus.PreviewStylusUpEvent, value, false); } remove { RemoveHandler(Stylus.PreviewStylusUpEvent, value); } } ////// Virtual method reporting a stylus-up /// protected virtual void OnPreviewStylusUp(StylusEventArgs e) {} ////// Alias to the Stylus.StylusUpEvent. /// public static readonly RoutedEvent StylusUpEvent = Stylus.StylusUpEvent.AddOwner(_typeofThis); ////// Event reporting a stylus-up /// public event StylusEventHandler StylusUp { add { AddHandler(Stylus.StylusUpEvent, value, false); } remove { RemoveHandler(Stylus.StylusUpEvent, value); } } ////// Virtual method reporting a stylus-up /// protected virtual void OnStylusUp(StylusEventArgs e) {} ////// Alias to the Stylus.PreviewStylusMoveEvent. /// public static readonly RoutedEvent PreviewStylusMoveEvent = Stylus.PreviewStylusMoveEvent.AddOwner(_typeofThis); ////// Event reporting a stylus move /// public event StylusEventHandler PreviewStylusMove { add { AddHandler(Stylus.PreviewStylusMoveEvent, value, false); } remove { RemoveHandler(Stylus.PreviewStylusMoveEvent, value); } } ////// Virtual method reporting a stylus move /// protected virtual void OnPreviewStylusMove(StylusEventArgs e) {} ////// Alias to the Stylus.StylusMoveEvent. /// public static readonly RoutedEvent StylusMoveEvent = Stylus.StylusMoveEvent.AddOwner(_typeofThis); ////// Event reporting a stylus move /// public event StylusEventHandler StylusMove { add { AddHandler(Stylus.StylusMoveEvent, value, false); } remove { RemoveHandler(Stylus.StylusMoveEvent, value); } } ////// Virtual method reporting a stylus move /// protected virtual void OnStylusMove(StylusEventArgs e) {} ////// Alias to the Stylus.PreviewStylusInAirMoveEvent. /// public static readonly RoutedEvent PreviewStylusInAirMoveEvent = Stylus.PreviewStylusInAirMoveEvent.AddOwner(_typeofThis); ////// Event reporting a stylus-in-air-move /// public event StylusEventHandler PreviewStylusInAirMove { add { AddHandler(Stylus.PreviewStylusInAirMoveEvent, value, false); } remove { RemoveHandler(Stylus.PreviewStylusInAirMoveEvent, value); } } ////// Virtual method reporting a stylus-in-air-move /// protected virtual void OnPreviewStylusInAirMove(StylusEventArgs e) {} ////// Alias to the Stylus.StylusInAirMoveEvent. /// public static readonly RoutedEvent StylusInAirMoveEvent = Stylus.StylusInAirMoveEvent.AddOwner(_typeofThis); ////// Event reporting a stylus-in-air-move /// public event StylusEventHandler StylusInAirMove { add { AddHandler(Stylus.StylusInAirMoveEvent, value, false); } remove { RemoveHandler(Stylus.StylusInAirMoveEvent, value); } } ////// Virtual method reporting a stylus-in-air-move /// protected virtual void OnStylusInAirMove(StylusEventArgs e) {} ////// Alias to the Stylus.StylusEnterEvent. /// public static readonly RoutedEvent StylusEnterEvent = Stylus.StylusEnterEvent.AddOwner(_typeofThis); ////// Event reporting the stylus entered this element /// public event StylusEventHandler StylusEnter { add { AddHandler(Stylus.StylusEnterEvent, value, false); } remove { RemoveHandler(Stylus.StylusEnterEvent, value); } } ////// Virtual method reporting the stylus entered this element /// protected virtual void OnStylusEnter(StylusEventArgs e) {} ////// Alias to the Stylus.StylusLeaveEvent. /// public static readonly RoutedEvent StylusLeaveEvent = Stylus.StylusLeaveEvent.AddOwner(_typeofThis); ////// Event reporting the stylus left this element /// public event StylusEventHandler StylusLeave { add { AddHandler(Stylus.StylusLeaveEvent, value, false); } remove { RemoveHandler(Stylus.StylusLeaveEvent, value); } } ////// Virtual method reporting the stylus left this element /// protected virtual void OnStylusLeave(StylusEventArgs e) {} ////// Alias to the Stylus.PreviewStylusInRangeEvent. /// public static readonly RoutedEvent PreviewStylusInRangeEvent = Stylus.PreviewStylusInRangeEvent.AddOwner(_typeofThis); ////// Event reporting the stylus is now in range of the digitizer /// public event StylusEventHandler PreviewStylusInRange { add { AddHandler(Stylus.PreviewStylusInRangeEvent, value, false); } remove { RemoveHandler(Stylus.PreviewStylusInRangeEvent, value); } } ////// Virtual method reporting the stylus is now in range of the digitizer /// protected virtual void OnPreviewStylusInRange(StylusEventArgs e) {} ////// Alias to the Stylus.StylusInRangeEvent. /// public static readonly RoutedEvent StylusInRangeEvent = Stylus.StylusInRangeEvent.AddOwner(_typeofThis); ////// Event reporting the stylus is now in range of the digitizer /// public event StylusEventHandler StylusInRange { add { AddHandler(Stylus.StylusInRangeEvent, value, false); } remove { RemoveHandler(Stylus.StylusInRangeEvent, value); } } ////// Virtual method reporting the stylus is now in range of the digitizer /// protected virtual void OnStylusInRange(StylusEventArgs e) {} ////// Alias to the Stylus.PreviewStylusOutOfRangeEvent. /// public static readonly RoutedEvent PreviewStylusOutOfRangeEvent = Stylus.PreviewStylusOutOfRangeEvent.AddOwner(_typeofThis); ////// Event reporting the stylus is now out of range of the digitizer /// public event StylusEventHandler PreviewStylusOutOfRange { add { AddHandler(Stylus.PreviewStylusOutOfRangeEvent, value, false); } remove { RemoveHandler(Stylus.PreviewStylusOutOfRangeEvent, value); } } ////// Virtual method reporting the stylus is now out of range of the digitizer /// protected virtual void OnPreviewStylusOutOfRange(StylusEventArgs e) {} ////// Alias to the Stylus.StylusOutOfRangeEvent. /// public static readonly RoutedEvent StylusOutOfRangeEvent = Stylus.StylusOutOfRangeEvent.AddOwner(_typeofThis); ////// Event reporting the stylus is now out of range of the digitizer /// public event StylusEventHandler StylusOutOfRange { add { AddHandler(Stylus.StylusOutOfRangeEvent, value, false); } remove { RemoveHandler(Stylus.StylusOutOfRangeEvent, value); } } ////// Virtual method reporting the stylus is now out of range of the digitizer /// protected virtual void OnStylusOutOfRange(StylusEventArgs e) {} ////// Alias to the Stylus.PreviewStylusSystemGestureEvent. /// public static readonly RoutedEvent PreviewStylusSystemGestureEvent = Stylus.PreviewStylusSystemGestureEvent.AddOwner(_typeofThis); ////// Event reporting a stylus system gesture /// public event StylusSystemGestureEventHandler PreviewStylusSystemGesture { add { AddHandler(Stylus.PreviewStylusSystemGestureEvent, value, false); } remove { RemoveHandler(Stylus.PreviewStylusSystemGestureEvent, value); } } ////// Virtual method reporting a stylus system gesture /// protected virtual void OnPreviewStylusSystemGesture(StylusSystemGestureEventArgs e) {} ////// Alias to the Stylus.StylusSystemGestureEvent. /// public static readonly RoutedEvent StylusSystemGestureEvent = Stylus.StylusSystemGestureEvent.AddOwner(_typeofThis); ////// Event reporting a stylus system gesture /// public event StylusSystemGestureEventHandler StylusSystemGesture { add { AddHandler(Stylus.StylusSystemGestureEvent, value, false); } remove { RemoveHandler(Stylus.StylusSystemGestureEvent, value); } } ////// Virtual method reporting a stylus system gesture /// protected virtual void OnStylusSystemGesture(StylusSystemGestureEventArgs e) {} ////// Alias to the Stylus.GotStylusCaptureEvent. /// public static readonly RoutedEvent GotStylusCaptureEvent = Stylus.GotStylusCaptureEvent.AddOwner(_typeofThis); ////// Event reporting that this element got the stylus capture /// public event StylusEventHandler GotStylusCapture { add { AddHandler(Stylus.GotStylusCaptureEvent, value, false); } remove { RemoveHandler(Stylus.GotStylusCaptureEvent, value); } } ////// Virtual method reporting that this element got the stylus capture /// protected virtual void OnGotStylusCapture(StylusEventArgs e) {} ////// Alias to the Stylus.LostStylusCaptureEvent. /// public static readonly RoutedEvent LostStylusCaptureEvent = Stylus.LostStylusCaptureEvent.AddOwner(_typeofThis); ////// Event reporting that this element lost the stylus capture /// public event StylusEventHandler LostStylusCapture { add { AddHandler(Stylus.LostStylusCaptureEvent, value, false); } remove { RemoveHandler(Stylus.LostStylusCaptureEvent, value); } } ////// Virtual method reporting that this element lost the stylus capture /// protected virtual void OnLostStylusCapture(StylusEventArgs e) {} ////// Alias to the Stylus.StylusButtonDownEvent. /// public static readonly RoutedEvent StylusButtonDownEvent = Stylus.StylusButtonDownEvent.AddOwner(_typeofThis); ////// Event reporting the stylus button is down /// public event StylusButtonEventHandler StylusButtonDown { add { AddHandler(Stylus.StylusButtonDownEvent, value, false); } remove { RemoveHandler(Stylus.StylusButtonDownEvent, value); } } ////// Virtual method reporting the stylus button is down /// protected virtual void OnStylusButtonDown(StylusButtonEventArgs e) {} ////// Alias to the Stylus.StylusButtonUpEvent. /// public static readonly RoutedEvent StylusButtonUpEvent = Stylus.StylusButtonUpEvent.AddOwner(_typeofThis); ////// Event reporting the stylus button is up /// public event StylusButtonEventHandler StylusButtonUp { add { AddHandler(Stylus.StylusButtonUpEvent, value, false); } remove { RemoveHandler(Stylus.StylusButtonUpEvent, value); } } ////// Virtual method reporting the stylus button is up /// protected virtual void OnStylusButtonUp(StylusButtonEventArgs e) {} ////// Alias to the Stylus.PreviewStylusButtonDownEvent. /// public static readonly RoutedEvent PreviewStylusButtonDownEvent = Stylus.PreviewStylusButtonDownEvent.AddOwner(_typeofThis); ////// Event reporting the stylus button is down /// public event StylusButtonEventHandler PreviewStylusButtonDown { add { AddHandler(Stylus.PreviewStylusButtonDownEvent, value, false); } remove { RemoveHandler(Stylus.PreviewStylusButtonDownEvent, value); } } ////// Virtual method reporting the stylus button is down /// protected virtual void OnPreviewStylusButtonDown(StylusButtonEventArgs e) {} ////// Alias to the Stylus.PreviewStylusButtonUpEvent. /// public static readonly RoutedEvent PreviewStylusButtonUpEvent = Stylus.PreviewStylusButtonUpEvent.AddOwner(_typeofThis); ////// Event reporting the stylus button is up /// public event StylusButtonEventHandler PreviewStylusButtonUp { add { AddHandler(Stylus.PreviewStylusButtonUpEvent, value, false); } remove { RemoveHandler(Stylus.PreviewStylusButtonUpEvent, value); } } ////// Virtual method reporting the stylus button is up /// protected virtual void OnPreviewStylusButtonUp(StylusButtonEventArgs e) {} ////// Alias to the Keyboard.PreviewKeyDownEvent. /// public static readonly RoutedEvent PreviewKeyDownEvent = Keyboard.PreviewKeyDownEvent.AddOwner(_typeofThis); ////// Event reporting a key was pressed /// public event KeyEventHandler PreviewKeyDown { add { AddHandler(Keyboard.PreviewKeyDownEvent, value, false); } remove { RemoveHandler(Keyboard.PreviewKeyDownEvent, value); } } ////// Virtual method reporting a key was pressed /// protected virtual void OnPreviewKeyDown(KeyEventArgs e) {} ////// Alias to the Keyboard.KeyDownEvent. /// public static readonly RoutedEvent KeyDownEvent = Keyboard.KeyDownEvent.AddOwner(_typeofThis); ////// Event reporting a key was pressed /// public event KeyEventHandler KeyDown { add { AddHandler(Keyboard.KeyDownEvent, value, false); } remove { RemoveHandler(Keyboard.KeyDownEvent, value); } } ////// Virtual method reporting a key was pressed /// protected virtual void OnKeyDown(KeyEventArgs e) {} ////// Alias to the Keyboard.PreviewKeyUpEvent. /// public static readonly RoutedEvent PreviewKeyUpEvent = Keyboard.PreviewKeyUpEvent.AddOwner(_typeofThis); ////// Event reporting a key was released /// public event KeyEventHandler PreviewKeyUp { add { AddHandler(Keyboard.PreviewKeyUpEvent, value, false); } remove { RemoveHandler(Keyboard.PreviewKeyUpEvent, value); } } ////// Virtual method reporting a key was released /// protected virtual void OnPreviewKeyUp(KeyEventArgs e) {} ////// Alias to the Keyboard.KeyUpEvent. /// public static readonly RoutedEvent KeyUpEvent = Keyboard.KeyUpEvent.AddOwner(_typeofThis); ////// Event reporting a key was released /// public event KeyEventHandler KeyUp { add { AddHandler(Keyboard.KeyUpEvent, value, false); } remove { RemoveHandler(Keyboard.KeyUpEvent, value); } } ////// Virtual method reporting a key was released /// protected virtual void OnKeyUp(KeyEventArgs e) {} ////// Alias to the Keyboard.PreviewGotKeyboardFocusEvent. /// public static readonly RoutedEvent PreviewGotKeyboardFocusEvent = Keyboard.PreviewGotKeyboardFocusEvent.AddOwner(_typeofThis); ////// Event reporting that the keyboard is focused on this element /// public event KeyboardFocusChangedEventHandler PreviewGotKeyboardFocus { add { AddHandler(Keyboard.PreviewGotKeyboardFocusEvent, value, false); } remove { RemoveHandler(Keyboard.PreviewGotKeyboardFocusEvent, value); } } ////// Virtual method reporting that the keyboard is focused on this element /// protected virtual void OnPreviewGotKeyboardFocus(KeyboardFocusChangedEventArgs e) {} ////// Alias to the Keyboard.GotKeyboardFocusEvent. /// public static readonly RoutedEvent GotKeyboardFocusEvent = Keyboard.GotKeyboardFocusEvent.AddOwner(_typeofThis); ////// Event reporting that the keyboard is focused on this element /// public event KeyboardFocusChangedEventHandler GotKeyboardFocus { add { AddHandler(Keyboard.GotKeyboardFocusEvent, value, false); } remove { RemoveHandler(Keyboard.GotKeyboardFocusEvent, value); } } ////// Virtual method reporting that the keyboard is focused on this element /// protected virtual void OnGotKeyboardFocus(KeyboardFocusChangedEventArgs e) {} ////// Alias to the Keyboard.PreviewLostKeyboardFocusEvent. /// public static readonly RoutedEvent PreviewLostKeyboardFocusEvent = Keyboard.PreviewLostKeyboardFocusEvent.AddOwner(_typeofThis); ////// Event reporting that the keyboard is no longer focusekeyboard is no longer focuseed /// public event KeyboardFocusChangedEventHandler PreviewLostKeyboardFocus { add { AddHandler(Keyboard.PreviewLostKeyboardFocusEvent, value, false); } remove { RemoveHandler(Keyboard.PreviewLostKeyboardFocusEvent, value); } } ////// Virtual method reporting that the keyboard is no longer focusekeyboard is no longer focuseed /// protected virtual void OnPreviewLostKeyboardFocus(KeyboardFocusChangedEventArgs e) {} ////// Alias to the Keyboard.LostKeyboardFocusEvent. /// public static readonly RoutedEvent LostKeyboardFocusEvent = Keyboard.LostKeyboardFocusEvent.AddOwner(_typeofThis); ////// Event reporting that the keyboard is no longer focusekeyboard is no longer focuseed /// public event KeyboardFocusChangedEventHandler LostKeyboardFocus { add { AddHandler(Keyboard.LostKeyboardFocusEvent, value, false); } remove { RemoveHandler(Keyboard.LostKeyboardFocusEvent, value); } } ////// Virtual method reporting that the keyboard is no longer focusekeyboard is no longer focuseed /// protected virtual void OnLostKeyboardFocus(KeyboardFocusChangedEventArgs e) {} ////// Alias to the TextCompositionManager.PreviewTextInputEvent. /// public static readonly RoutedEvent PreviewTextInputEvent = TextCompositionManager.PreviewTextInputEvent.AddOwner(_typeofThis); ////// Event reporting text composition /// public event TextCompositionEventHandler PreviewTextInput { add { AddHandler(TextCompositionManager.PreviewTextInputEvent, value, false); } remove { RemoveHandler(TextCompositionManager.PreviewTextInputEvent, value); } } ////// Virtual method reporting text composition /// protected virtual void OnPreviewTextInput(TextCompositionEventArgs e) {} ////// Alias to the TextCompositionManager.TextInputEvent. /// public static readonly RoutedEvent TextInputEvent = TextCompositionManager.TextInputEvent.AddOwner(_typeofThis); ////// Event reporting text composition /// public event TextCompositionEventHandler TextInput { add { AddHandler(TextCompositionManager.TextInputEvent, value, false); } remove { RemoveHandler(TextCompositionManager.TextInputEvent, value); } } ////// Virtual method reporting text composition /// protected virtual void OnTextInput(TextCompositionEventArgs e) {} ////// Alias to the DragDrop.PreviewQueryContinueDragEvent. /// public static readonly RoutedEvent PreviewQueryContinueDragEvent = DragDrop.PreviewQueryContinueDragEvent.AddOwner(_typeofThis); ////// Event reporting the preview query continue drag is going to happen /// public event QueryContinueDragEventHandler PreviewQueryContinueDrag { add { AddHandler(DragDrop.PreviewQueryContinueDragEvent, value, false); } remove { RemoveHandler(DragDrop.PreviewQueryContinueDragEvent, value); } } ////// Virtual method reporting the preview query continue drag is going to happen /// protected virtual void OnPreviewQueryContinueDrag(QueryContinueDragEventArgs e) {} ////// Alias to the DragDrop.QueryContinueDragEvent. /// public static readonly RoutedEvent QueryContinueDragEvent = DragDrop.QueryContinueDragEvent.AddOwner(_typeofThis); ////// Event reporting the query continue drag is going to happen /// public event QueryContinueDragEventHandler QueryContinueDrag { add { AddHandler(DragDrop.QueryContinueDragEvent, value, false); } remove { RemoveHandler(DragDrop.QueryContinueDragEvent, value); } } ////// Virtual method reporting the query continue drag is going to happen /// protected virtual void OnQueryContinueDrag(QueryContinueDragEventArgs e) {} ////// Alias to the DragDrop.PreviewGiveFeedbackEvent. /// public static readonly RoutedEvent PreviewGiveFeedbackEvent = DragDrop.PreviewGiveFeedbackEvent.AddOwner(_typeofThis); ////// Event reporting the preview give feedback is going to happen /// public event GiveFeedbackEventHandler PreviewGiveFeedback { add { AddHandler(DragDrop.PreviewGiveFeedbackEvent, value, false); } remove { RemoveHandler(DragDrop.PreviewGiveFeedbackEvent, value); } } ////// Virtual method reporting the preview give feedback is going to happen /// protected virtual void OnPreviewGiveFeedback(GiveFeedbackEventArgs e) {} ////// Alias to the DragDrop.GiveFeedbackEvent. /// public static readonly RoutedEvent GiveFeedbackEvent = DragDrop.GiveFeedbackEvent.AddOwner(_typeofThis); ////// Event reporting the give feedback is going to happen /// public event GiveFeedbackEventHandler GiveFeedback { add { AddHandler(DragDrop.GiveFeedbackEvent, value, false); } remove { RemoveHandler(DragDrop.GiveFeedbackEvent, value); } } ////// Virtual method reporting the give feedback is going to happen /// protected virtual void OnGiveFeedback(GiveFeedbackEventArgs e) {} ////// Alias to the DragDrop.PreviewDragEnterEvent. /// public static readonly RoutedEvent PreviewDragEnterEvent = DragDrop.PreviewDragEnterEvent.AddOwner(_typeofThis); ////// Event reporting the preview drag enter is going to happen /// public event DragEventHandler PreviewDragEnter { add { AddHandler(DragDrop.PreviewDragEnterEvent, value, false); } remove { RemoveHandler(DragDrop.PreviewDragEnterEvent, value); } } ////// Virtual method reporting the preview drag enter is going to happen /// protected virtual void OnPreviewDragEnter(DragEventArgs e) {} ////// Alias to the DragDrop.DragEnterEvent. /// public static readonly RoutedEvent DragEnterEvent = DragDrop.DragEnterEvent.AddOwner(_typeofThis); ////// Event reporting the drag enter is going to happen /// public event DragEventHandler DragEnter { add { AddHandler(DragDrop.DragEnterEvent, value, false); } remove { RemoveHandler(DragDrop.DragEnterEvent, value); } } ////// Virtual method reporting the drag enter is going to happen /// protected virtual void OnDragEnter(DragEventArgs e) {} ////// Alias to the DragDrop.PreviewDragOverEvent. /// public static readonly RoutedEvent PreviewDragOverEvent = DragDrop.PreviewDragOverEvent.AddOwner(_typeofThis); ////// Event reporting the preview drag over is going to happen /// public event DragEventHandler PreviewDragOver { add { AddHandler(DragDrop.PreviewDragOverEvent, value, false); } remove { RemoveHandler(DragDrop.PreviewDragOverEvent, value); } } ////// Virtual method reporting the preview drag over is going to happen /// protected virtual void OnPreviewDragOver(DragEventArgs e) {} ////// Alias to the DragDrop.DragOverEvent. /// public static readonly RoutedEvent DragOverEvent = DragDrop.DragOverEvent.AddOwner(_typeofThis); ////// Event reporting the drag over is going to happen /// public event DragEventHandler DragOver { add { AddHandler(DragDrop.DragOverEvent, value, false); } remove { RemoveHandler(DragDrop.DragOverEvent, value); } } ////// Virtual method reporting the drag over is going to happen /// protected virtual void OnDragOver(DragEventArgs e) {} ////// Alias to the DragDrop.PreviewDragLeaveEvent. /// public static readonly RoutedEvent PreviewDragLeaveEvent = DragDrop.PreviewDragLeaveEvent.AddOwner(_typeofThis); ////// Event reporting the preview drag leave is going to happen /// public event DragEventHandler PreviewDragLeave { add { AddHandler(DragDrop.PreviewDragLeaveEvent, value, false); } remove { RemoveHandler(DragDrop.PreviewDragLeaveEvent, value); } } ////// Virtual method reporting the preview drag leave is going to happen /// protected virtual void OnPreviewDragLeave(DragEventArgs e) {} ////// Alias to the DragDrop.DragLeaveEvent. /// public static readonly RoutedEvent DragLeaveEvent = DragDrop.DragLeaveEvent.AddOwner(_typeofThis); ////// Event reporting the drag leave is going to happen /// public event DragEventHandler DragLeave { add { AddHandler(DragDrop.DragLeaveEvent, value, false); } remove { RemoveHandler(DragDrop.DragLeaveEvent, value); } } ////// Virtual method reporting the drag leave is going to happen /// protected virtual void OnDragLeave(DragEventArgs e) {} ////// Alias to the DragDrop.PreviewDropEvent. /// public static readonly RoutedEvent PreviewDropEvent = DragDrop.PreviewDropEvent.AddOwner(_typeofThis); ////// Event reporting the preview drop is going to happen /// public event DragEventHandler PreviewDrop { add { AddHandler(DragDrop.PreviewDropEvent, value, false); } remove { RemoveHandler(DragDrop.PreviewDropEvent, value); } } ////// Virtual method reporting the preview drop is going to happen /// protected virtual void OnPreviewDrop(DragEventArgs e) {} ////// Alias to the DragDrop.DropEvent. /// public static readonly RoutedEvent DropEvent = DragDrop.DropEvent.AddOwner(_typeofThis); ////// Event reporting the drag enter is going to happen /// public event DragEventHandler Drop { add { AddHandler(DragDrop.DropEvent, value, false); } remove { RemoveHandler(DragDrop.DropEvent, value); } } ////// Virtual method reporting the drag enter is going to happen /// protected virtual void OnDrop(DragEventArgs e) {} ////// The key needed set a read-only property. /// internal static readonly DependencyPropertyKey IsMouseDirectlyOverPropertyKey = DependencyProperty.RegisterReadOnly( "IsMouseDirectlyOver", typeof(bool), _typeofThis, new PropertyMetadata( BooleanBoxes.FalseBox, // default value new PropertyChangedCallback(IsMouseDirectlyOver_Changed))); ////// The dependency property for the IsMouseDirectlyOver property. /// public static readonly DependencyProperty IsMouseDirectlyOverProperty = IsMouseDirectlyOverPropertyKey.DependencyProperty; private static void IsMouseDirectlyOver_Changed(DependencyObject d, DependencyPropertyChangedEventArgs e) { ((UIElement) d).RaiseIsMouseDirectlyOverChanged(e); } ////// IsMouseDirectlyOverChanged private key /// internal static readonly EventPrivateKey IsMouseDirectlyOverChangedKey = new EventPrivateKey(); ////// An event reporting that the IsMouseDirectlyOver property changed. /// public event DependencyPropertyChangedEventHandler IsMouseDirectlyOverChanged { add { EventHandlersStoreAdd(UIElement.IsMouseDirectlyOverChangedKey, value); } remove { EventHandlersStoreRemove(UIElement.IsMouseDirectlyOverChangedKey, value); } } ////// An event reporting that the IsMouseDirectlyOver property changed. /// protected virtual void OnIsMouseDirectlyOverChanged(DependencyPropertyChangedEventArgs e) { } private void RaiseIsMouseDirectlyOverChanged(DependencyPropertyChangedEventArgs args) { // Call the virtual method first. OnIsMouseDirectlyOverChanged(args); // Raise the public event second. RaiseDependencyPropertyChanged(UIElement.IsMouseDirectlyOverChangedKey, args); } ////// The key needed set a read-only property. /// internal static readonly DependencyPropertyKey IsMouseOverPropertyKey = DependencyProperty.RegisterReadOnly( "IsMouseOver", typeof(bool), _typeofThis, new PropertyMetadata( BooleanBoxes.FalseBox)); ////// The dependency property for the IsMouseOver property. /// public static readonly DependencyProperty IsMouseOverProperty = IsMouseOverPropertyKey.DependencyProperty; ////// The key needed set a read-only property. /// internal static readonly DependencyPropertyKey IsStylusOverPropertyKey = DependencyProperty.RegisterReadOnly( "IsStylusOver", typeof(bool), _typeofThis, new PropertyMetadata( BooleanBoxes.FalseBox)); ////// The dependency property for the IsStylusOver property. /// public static readonly DependencyProperty IsStylusOverProperty = IsStylusOverPropertyKey.DependencyProperty; ////// The key needed set a read-only property. /// internal static readonly DependencyPropertyKey IsKeyboardFocusWithinPropertyKey = DependencyProperty.RegisterReadOnly( "IsKeyboardFocusWithin", typeof(bool), _typeofThis, new PropertyMetadata( BooleanBoxes.FalseBox)); ////// The dependency property for the IsKeyboardFocusWithin property. /// public static readonly DependencyProperty IsKeyboardFocusWithinProperty = IsKeyboardFocusWithinPropertyKey.DependencyProperty; ////// IsKeyboardFocusWithinChanged private key /// internal static readonly EventPrivateKey IsKeyboardFocusWithinChangedKey = new EventPrivateKey(); ////// An event reporting that the IsKeyboardFocusWithin property changed. /// public event DependencyPropertyChangedEventHandler IsKeyboardFocusWithinChanged { add { EventHandlersStoreAdd(UIElement.IsKeyboardFocusWithinChangedKey, value); } remove { EventHandlersStoreRemove(UIElement.IsKeyboardFocusWithinChangedKey, value); } } ////// An event reporting that the IsKeyboardFocusWithin property changed. /// protected virtual void OnIsKeyboardFocusWithinChanged(DependencyPropertyChangedEventArgs e) { } internal void RaiseIsKeyboardFocusWithinChanged(DependencyPropertyChangedEventArgs args) { // Call the virtual method first. OnIsKeyboardFocusWithinChanged(args); // Raise the public event second. RaiseDependencyPropertyChanged(UIElement.IsKeyboardFocusWithinChangedKey, args); } ////// The key needed set a read-only property. /// internal static readonly DependencyPropertyKey IsMouseCapturedPropertyKey = DependencyProperty.RegisterReadOnly( "IsMouseCaptured", typeof(bool), _typeofThis, new PropertyMetadata( BooleanBoxes.FalseBox, // default value new PropertyChangedCallback(IsMouseCaptured_Changed))); ////// The dependency property for the IsMouseCaptured property. /// public static readonly DependencyProperty IsMouseCapturedProperty = IsMouseCapturedPropertyKey.DependencyProperty; private static void IsMouseCaptured_Changed(DependencyObject d, DependencyPropertyChangedEventArgs e) { ((UIElement) d).RaiseIsMouseCapturedChanged(e); } ////// IsMouseCapturedChanged private key /// internal static readonly EventPrivateKey IsMouseCapturedChangedKey = new EventPrivateKey(); ////// An event reporting that the IsMouseCaptured property changed. /// public event DependencyPropertyChangedEventHandler IsMouseCapturedChanged { add { EventHandlersStoreAdd(UIElement.IsMouseCapturedChangedKey, value); } remove { EventHandlersStoreRemove(UIElement.IsMouseCapturedChangedKey, value); } } ////// An event reporting that the IsMouseCaptured property changed. /// protected virtual void OnIsMouseCapturedChanged(DependencyPropertyChangedEventArgs e) { } private void RaiseIsMouseCapturedChanged(DependencyPropertyChangedEventArgs args) { // Call the virtual method first. OnIsMouseCapturedChanged(args); // Raise the public event second. RaiseDependencyPropertyChanged(UIElement.IsMouseCapturedChangedKey, args); } ////// The key needed set a read-only property. /// internal static readonly DependencyPropertyKey IsMouseCaptureWithinPropertyKey = DependencyProperty.RegisterReadOnly( "IsMouseCaptureWithin", typeof(bool), _typeofThis, new PropertyMetadata( BooleanBoxes.FalseBox)); ////// The dependency property for the IsMouseCaptureWithin property. /// public static readonly DependencyProperty IsMouseCaptureWithinProperty = IsMouseCaptureWithinPropertyKey.DependencyProperty; ////// IsMouseCaptureWithinChanged private key /// internal static readonly EventPrivateKey IsMouseCaptureWithinChangedKey = new EventPrivateKey(); ////// An event reporting that the IsMouseCaptureWithin property changed. /// public event DependencyPropertyChangedEventHandler IsMouseCaptureWithinChanged { add { EventHandlersStoreAdd(UIElement.IsMouseCaptureWithinChangedKey, value); } remove { EventHandlersStoreRemove(UIElement.IsMouseCaptureWithinChangedKey, value); } } ////// An event reporting that the IsMouseCaptureWithin property changed. /// protected virtual void OnIsMouseCaptureWithinChanged(DependencyPropertyChangedEventArgs e) { } internal void RaiseIsMouseCaptureWithinChanged(DependencyPropertyChangedEventArgs args) { // Call the virtual method first. OnIsMouseCaptureWithinChanged(args); // Raise the public event second. RaiseDependencyPropertyChanged(UIElement.IsMouseCaptureWithinChangedKey, args); } ////// The key needed set a read-only property. /// internal static readonly DependencyPropertyKey IsStylusDirectlyOverPropertyKey = DependencyProperty.RegisterReadOnly( "IsStylusDirectlyOver", typeof(bool), _typeofThis, new PropertyMetadata( BooleanBoxes.FalseBox, // default value new PropertyChangedCallback(IsStylusDirectlyOver_Changed))); ////// The dependency property for the IsStylusDirectlyOver property. /// public static readonly DependencyProperty IsStylusDirectlyOverProperty = IsStylusDirectlyOverPropertyKey.DependencyProperty; private static void IsStylusDirectlyOver_Changed(DependencyObject d, DependencyPropertyChangedEventArgs e) { ((UIElement) d).RaiseIsStylusDirectlyOverChanged(e); } ////// IsStylusDirectlyOverChanged private key /// internal static readonly EventPrivateKey IsStylusDirectlyOverChangedKey = new EventPrivateKey(); ////// An event reporting that the IsStylusDirectlyOver property changed. /// public event DependencyPropertyChangedEventHandler IsStylusDirectlyOverChanged { add { EventHandlersStoreAdd(UIElement.IsStylusDirectlyOverChangedKey, value); } remove { EventHandlersStoreRemove(UIElement.IsStylusDirectlyOverChangedKey, value); } } ////// An event reporting that the IsStylusDirectlyOver property changed. /// protected virtual void OnIsStylusDirectlyOverChanged(DependencyPropertyChangedEventArgs e) { } private void RaiseIsStylusDirectlyOverChanged(DependencyPropertyChangedEventArgs args) { // Call the virtual method first. OnIsStylusDirectlyOverChanged(args); // Raise the public event second. RaiseDependencyPropertyChanged(UIElement.IsStylusDirectlyOverChangedKey, args); } ////// The key needed set a read-only property. /// internal static readonly DependencyPropertyKey IsStylusCapturedPropertyKey = DependencyProperty.RegisterReadOnly( "IsStylusCaptured", typeof(bool), _typeofThis, new PropertyMetadata( BooleanBoxes.FalseBox, // default value new PropertyChangedCallback(IsStylusCaptured_Changed))); ////// The dependency property for the IsStylusCaptured property. /// public static readonly DependencyProperty IsStylusCapturedProperty = IsStylusCapturedPropertyKey.DependencyProperty; private static void IsStylusCaptured_Changed(DependencyObject d, DependencyPropertyChangedEventArgs e) { ((UIElement) d).RaiseIsStylusCapturedChanged(e); } ////// IsStylusCapturedChanged private key /// internal static readonly EventPrivateKey IsStylusCapturedChangedKey = new EventPrivateKey(); ////// An event reporting that the IsStylusCaptured property changed. /// public event DependencyPropertyChangedEventHandler IsStylusCapturedChanged { add { EventHandlersStoreAdd(UIElement.IsStylusCapturedChangedKey, value); } remove { EventHandlersStoreRemove(UIElement.IsStylusCapturedChangedKey, value); } } ////// An event reporting that the IsStylusCaptured property changed. /// protected virtual void OnIsStylusCapturedChanged(DependencyPropertyChangedEventArgs e) { } private void RaiseIsStylusCapturedChanged(DependencyPropertyChangedEventArgs args) { // Call the virtual method first. OnIsStylusCapturedChanged(args); // Raise the public event second. RaiseDependencyPropertyChanged(UIElement.IsStylusCapturedChangedKey, args); } ////// The key needed set a read-only property. /// internal static readonly DependencyPropertyKey IsStylusCaptureWithinPropertyKey = DependencyProperty.RegisterReadOnly( "IsStylusCaptureWithin", typeof(bool), _typeofThis, new PropertyMetadata( BooleanBoxes.FalseBox)); ////// The dependency property for the IsStylusCaptureWithin property. /// public static readonly DependencyProperty IsStylusCaptureWithinProperty = IsStylusCaptureWithinPropertyKey.DependencyProperty; ////// IsStylusCaptureWithinChanged private key /// internal static readonly EventPrivateKey IsStylusCaptureWithinChangedKey = new EventPrivateKey(); ////// An event reporting that the IsStylusCaptureWithin property changed. /// public event DependencyPropertyChangedEventHandler IsStylusCaptureWithinChanged { add { EventHandlersStoreAdd(UIElement.IsStylusCaptureWithinChangedKey, value); } remove { EventHandlersStoreRemove(UIElement.IsStylusCaptureWithinChangedKey, value); } } ////// An event reporting that the IsStylusCaptureWithin property changed. /// protected virtual void OnIsStylusCaptureWithinChanged(DependencyPropertyChangedEventArgs e) { } internal void RaiseIsStylusCaptureWithinChanged(DependencyPropertyChangedEventArgs args) { // Call the virtual method first. OnIsStylusCaptureWithinChanged(args); // Raise the public event second. RaiseDependencyPropertyChanged(UIElement.IsStylusCaptureWithinChangedKey, args); } ////// The key needed set a read-only property. /// internal static readonly DependencyPropertyKey IsKeyboardFocusedPropertyKey = DependencyProperty.RegisterReadOnly( "IsKeyboardFocused", typeof(bool), _typeofThis, new PropertyMetadata( BooleanBoxes.FalseBox, // default value new PropertyChangedCallback(IsKeyboardFocused_Changed))); ////// The dependency property for the IsKeyboardFocused property. /// public static readonly DependencyProperty IsKeyboardFocusedProperty = IsKeyboardFocusedPropertyKey.DependencyProperty; private static void IsKeyboardFocused_Changed(DependencyObject d, DependencyPropertyChangedEventArgs e) { ((UIElement) d).RaiseIsKeyboardFocusedChanged(e); } ////// IsKeyboardFocusedChanged private key /// internal static readonly EventPrivateKey IsKeyboardFocusedChangedKey = new EventPrivateKey(); ////// An event reporting that the IsKeyboardFocused property changed. /// public event DependencyPropertyChangedEventHandler IsKeyboardFocusedChanged { add { EventHandlersStoreAdd(UIElement.IsKeyboardFocusedChangedKey, value); } remove { EventHandlersStoreRemove(UIElement.IsKeyboardFocusedChangedKey, value); } } ////// An event reporting that the IsKeyboardFocused property changed. /// protected virtual void OnIsKeyboardFocusedChanged(DependencyPropertyChangedEventArgs e) { } private void RaiseIsKeyboardFocusedChanged(DependencyPropertyChangedEventArgs args) { // Call the virtual method first. OnIsKeyboardFocusedChanged(args); // Raise the public event second. RaiseDependencyPropertyChanged(UIElement.IsKeyboardFocusedChangedKey, args); } internal bool ReadFlag(CoreFlags field) { return (_flags & field) != 0; } internal void WriteFlag(CoreFlags field,bool value) { if (value) { _flags |= field; } else { _flags &= (~field); } } private CoreFlags _flags; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
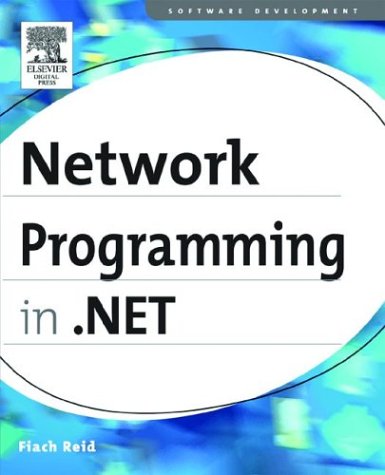
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DbConnectionPoolGroupProviderInfo.cs
- UpdatePanel.cs
- ProxyWebPartConnectionCollection.cs
- AsyncResult.cs
- ProjectionPlan.cs
- FormViewInsertedEventArgs.cs
- DataGridViewCellStyleChangedEventArgs.cs
- FileNotFoundException.cs
- DiscoveryClientElement.cs
- SQLGuid.cs
- VerificationAttribute.cs
- System.Data_BID.cs
- DBConnectionString.cs
- IndexOutOfRangeException.cs
- MainMenu.cs
- FaultPropagationQuery.cs
- WindowsUserNameSecurityTokenAuthenticator.cs
- ConstraintEnumerator.cs
- DocumentEventArgs.cs
- OuterProxyWrapper.cs
- PersonalizationStateInfoCollection.cs
- TreeNodeCollectionEditor.cs
- DictionaryBase.cs
- ItemCollection.cs
- CookielessHelper.cs
- ConfigXmlAttribute.cs
- BuildTopDownAttribute.cs
- COM2Properties.cs
- HideDisabledControlAdapter.cs
- ContextMenu.cs
- CustomErrorsSection.cs
- AlphabeticalEnumConverter.cs
- MutableAssemblyCacheEntry.cs
- AccessViolationException.cs
- RegisterInfo.cs
- IteratorDescriptor.cs
- CreateUserErrorEventArgs.cs
- EdmFunctions.cs
- StatusBar.cs
- ContextActivityUtils.cs
- ReversePositionQuery.cs
- FlowLayoutSettings.cs
- RunClient.cs
- FileSystemInfo.cs
- ContainerParaClient.cs
- InfiniteTimeSpanConverter.cs
- ReaderWriterLockWrapper.cs
- UnicastIPAddressInformationCollection.cs
- ThreadStateException.cs
- InternalConfigSettingsFactory.cs
- CodeBinaryOperatorExpression.cs
- GlobalProxySelection.cs
- ModelFunction.cs
- ActivityBuilderHelper.cs
- ExpandCollapsePattern.cs
- SerialErrors.cs
- UrlMappingsModule.cs
- InputProcessorProfiles.cs
- XamlPathDataSerializer.cs
- MessageParameterAttribute.cs
- InteropAutomationProvider.cs
- IIS7WorkerRequest.cs
- DesignerLoader.cs
- MediaContextNotificationWindow.cs
- NotFiniteNumberException.cs
- DbModificationCommandTree.cs
- XmlSchemaInfo.cs
- DefinitionUpdate.cs
- WriteableBitmap.cs
- FormParameter.cs
- Permission.cs
- BindingExpressionUncommonField.cs
- CodeDomSerializationProvider.cs
- InkSerializer.cs
- DebugView.cs
- Ray3DHitTestResult.cs
- ValidationUtility.cs
- WebPartZoneBase.cs
- SqlDataSourceSelectingEventArgs.cs
- HandleValueEditor.cs
- FacetEnabledSchemaElement.cs
- Symbol.cs
- Trustee.cs
- TreeViewCancelEvent.cs
- BitmapFrameEncode.cs
- _FtpDataStream.cs
- DateTimeOffset.cs
- ResourceSet.cs
- WsatAdminException.cs
- ListViewHitTestInfo.cs
- TrustManagerPromptUI.cs
- SystemColorTracker.cs
- PartManifestEntry.cs
- Freezable.cs
- InfiniteIntConverter.cs
- AuthorizationSection.cs
- WaitHandle.cs
- Configuration.cs
- InvalidWMPVersionException.cs
- GenericNameHandler.cs