Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / CompMod / System / ComponentModel / DesignerCategoryAttribute.cs / 1 / DesignerCategoryAttribute.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel { using System; using System.ComponentModel; using System.Security.Permissions; ////// [AttributeUsage(AttributeTargets.Class, AllowMultiple = false, Inherited = true)] public sealed class DesignerCategoryAttribute : Attribute { private string category; private string typeId; ///Specifies that the designer for a class belongs to a certain /// category. ////// public static readonly DesignerCategoryAttribute Component = new DesignerCategoryAttribute("Component"); ////// Specifies that a component marked with this category uses a /// component designer. This ///field is read-only. /// /// public static readonly DesignerCategoryAttribute Default = new DesignerCategoryAttribute(); ////// Specifies that a component marked with this category cannot use a visual /// designer. This ///field is read-only. /// /// public static readonly DesignerCategoryAttribute Form = new DesignerCategoryAttribute("Form"); ////// Specifies that a component marked with this category uses a form designer. /// This ///field is read-only. /// /// public static readonly DesignerCategoryAttribute Generic = new DesignerCategoryAttribute("Designer"); ////// Specifies that a component marked with this category uses a generic designer. /// This ///field is read-only. /// /// public DesignerCategoryAttribute() { category = string.Empty; } ////// Initializes a new instance of the ///class with the /// default category. /// /// public DesignerCategoryAttribute(string category) { this.category = category; } ////// Initializes a new instance of the ///class with /// the given category name. /// /// public string Category { get { return category; } } ////// Gets the name of the category. /// ////// /// public override object TypeId { get { if (typeId == null) { typeId = GetType().FullName + Category; } return typeId; } } ////// This defines a unique ID for this attribute type. It is used /// by filtering algorithms to identify two attributes that are /// the same type. For most attributes, this just returns the /// Type instance for the attribute. DesignerAttribute overrides /// this to include the name of the category /// ////// ////// ////// public override bool Equals(object obj){ if (obj == this) { return true; } DesignerCategoryAttribute other = obj as DesignerCategoryAttribute; return (other != null) && other.category == category; } /// /// public override int GetHashCode() { return category.GetHashCode(); } ///[To be supplied.] ////// ////// ////// public override bool IsDefaultAttribute() { return category.Equals(Default.Category); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel { using System; using System.ComponentModel; using System.Security.Permissions; ////// [AttributeUsage(AttributeTargets.Class, AllowMultiple = false, Inherited = true)] public sealed class DesignerCategoryAttribute : Attribute { private string category; private string typeId; ///Specifies that the designer for a class belongs to a certain /// category. ////// public static readonly DesignerCategoryAttribute Component = new DesignerCategoryAttribute("Component"); ////// Specifies that a component marked with this category uses a /// component designer. This ///field is read-only. /// /// public static readonly DesignerCategoryAttribute Default = new DesignerCategoryAttribute(); ////// Specifies that a component marked with this category cannot use a visual /// designer. This ///field is read-only. /// /// public static readonly DesignerCategoryAttribute Form = new DesignerCategoryAttribute("Form"); ////// Specifies that a component marked with this category uses a form designer. /// This ///field is read-only. /// /// public static readonly DesignerCategoryAttribute Generic = new DesignerCategoryAttribute("Designer"); ////// Specifies that a component marked with this category uses a generic designer. /// This ///field is read-only. /// /// public DesignerCategoryAttribute() { category = string.Empty; } ////// Initializes a new instance of the ///class with the /// default category. /// /// public DesignerCategoryAttribute(string category) { this.category = category; } ////// Initializes a new instance of the ///class with /// the given category name. /// /// public string Category { get { return category; } } ////// Gets the name of the category. /// ////// /// public override object TypeId { get { if (typeId == null) { typeId = GetType().FullName + Category; } return typeId; } } ////// This defines a unique ID for this attribute type. It is used /// by filtering algorithms to identify two attributes that are /// the same type. For most attributes, this just returns the /// Type instance for the attribute. DesignerAttribute overrides /// this to include the name of the category /// ////// ////// ////// public override bool Equals(object obj){ if (obj == this) { return true; } DesignerCategoryAttribute other = obj as DesignerCategoryAttribute; return (other != null) && other.category == category; } /// /// public override int GetHashCode() { return category.GetHashCode(); } ///[To be supplied.] ////// ////// ////// public override bool IsDefaultAttribute() { return category.Equals(Default.Category); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
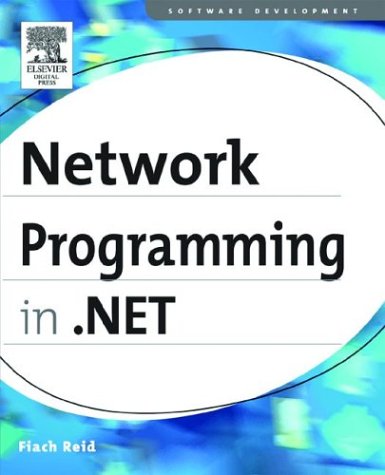
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SHA1Managed.cs
- ErrorTableItemStyle.cs
- WinInetCache.cs
- XmlSchemaExternal.cs
- DesignerVerbCollection.cs
- ReferencedAssembly.cs
- HttpCookie.cs
- ExpressionConverter.cs
- Compress.cs
- COM2TypeInfoProcessor.cs
- EntryWrittenEventArgs.cs
- IgnoreDataMemberAttribute.cs
- SmtpNetworkElement.cs
- XNameConverter.cs
- SimpleBitVector32.cs
- EncoderParameter.cs
- DBDataPermissionAttribute.cs
- StringExpressionSet.cs
- AnnotationAuthorChangedEventArgs.cs
- NumberSubstitution.cs
- TreeNodeCollectionEditor.cs
- TableNameAttribute.cs
- webeventbuffer.cs
- DependencyPropertyKind.cs
- SQLSingle.cs
- StrongTypingException.cs
- SoapReflectionImporter.cs
- AuthenticationConfig.cs
- Model3D.cs
- CacheMemory.cs
- RuntimeArgumentHandle.cs
- Condition.cs
- ProcessHostConfigUtils.cs
- ConnectionProviderAttribute.cs
- ContentWrapperAttribute.cs
- ColorTranslator.cs
- SystemKeyConverter.cs
- WebService.cs
- Style.cs
- NavigationHelper.cs
- cryptoapiTransform.cs
- GrabHandleGlyph.cs
- ReadOnlyNameValueCollection.cs
- UIPermission.cs
- PersonalizationProvider.cs
- BuildResultCache.cs
- OleDbConnectionFactory.cs
- TextStore.cs
- TextTreeDeleteContentUndoUnit.cs
- WebException.cs
- StylusPointProperty.cs
- FlowPosition.cs
- StyleCollectionEditor.cs
- ExpressionsCollectionEditor.cs
- GroupPartitionExpr.cs
- FlowDocument.cs
- BindingSource.cs
- Rect.cs
- PointAnimationUsingKeyFrames.cs
- SkewTransform.cs
- DragDrop.cs
- CommandCollectionEditor.cs
- NavigationEventArgs.cs
- FreezableDefaultValueFactory.cs
- SecurityHelper.cs
- ButtonBaseAdapter.cs
- EdgeModeValidation.cs
- _CommandStream.cs
- ScriptResourceMapping.cs
- PlatformCulture.cs
- ScriptingRoleServiceSection.cs
- DataControlFieldCell.cs
- QilFactory.cs
- ImageFormat.cs
- TextTreeInsertUndoUnit.cs
- WebPartPersonalization.cs
- TrackBarRenderer.cs
- TemplateBamlTreeBuilder.cs
- ImageField.cs
- BindingSource.cs
- StateRuntime.cs
- OleDbWrapper.cs
- CommonDialog.cs
- Executor.cs
- VerificationAttribute.cs
- ListItemCollection.cs
- TypeCodeDomSerializer.cs
- InkCanvasSelectionAdorner.cs
- TabControl.cs
- NumericUpDown.cs
- FocusManager.cs
- objectquery_tresulttype.cs
- FlowSwitchLink.cs
- XPathParser.cs
- LinqDataSourceView.cs
- SmiRequestExecutor.cs
- FormsAuthenticationConfiguration.cs
- WindowsScrollBarBits.cs
- OneOfTypeConst.cs
- WSSecurityOneDotOneReceiveSecurityHeader.cs