Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / Net / System / Net / Configuration / SmtpNetworkElement.cs / 3 / SmtpNetworkElement.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net.Configuration { using System; using System.Configuration; using System.Net; using System.Net.Mail; using System.Reflection; using System.Security.Permissions; public sealed class SmtpNetworkElement : ConfigurationElement { public SmtpNetworkElement() { this.properties.Add(this.defaultCredentials); this.properties.Add(this.host); this.properties.Add(this.clientDomain); this.properties.Add(this.password); this.properties.Add(this.port); this.properties.Add(this.userName); this.properties.Add(this.targetName); } protected override void PostDeserialize() { // Perf optimization. If the configuration is coming from machine.config // It is safe and we don't need to check for permissions. if (EvaluationContext.IsMachineLevel) return; PropertyInformation portPropertyInfo = ElementInformation.Properties[ConfigurationStrings.Port]; if (portPropertyInfo.ValueOrigin == PropertyValueOrigin.SetHere && (int)portPropertyInfo.Value != (int)portPropertyInfo.DefaultValue) { try { (new SmtpPermission(SmtpAccess.ConnectToUnrestrictedPort)).Demand(); } catch (Exception exception) { throw new ConfigurationErrorsException( SR.GetString(SR.net_config_property_permission, portPropertyInfo.Name), exception); } } } protected override ConfigurationPropertyCollection Properties { get { return this.properties; } } [ConfigurationProperty(ConfigurationStrings.DefaultCredentials, DefaultValue = false)] public bool DefaultCredentials { get { return (bool)this[this.defaultCredentials]; } set { this[this.defaultCredentials] = value; } } [ConfigurationProperty(ConfigurationStrings.Host)] public string Host { get { return (string)this[this.host]; } set { this[this.host] = value; } } [ConfigurationProperty(ConfigurationStrings.ClientDomain)] public string ClientDomain { get { return (string)this[this.clientDomain]; } set { this[this.clientDomain] = value; } } [ConfigurationProperty(ConfigurationStrings.TargetName)] public string TargetName { get { return (string)this[this.targetName]; } set { this[this.targetName] = value; } } [ConfigurationProperty(ConfigurationStrings.Password)] public string Password { get { return (string)this[this.password]; } set { this[this.password] = value; } } [ConfigurationProperty(ConfigurationStrings.Port, DefaultValue = 25)] public int Port { get { return (int)this[this.port]; } set { // this[this.port] = value; } } [ConfigurationProperty(ConfigurationStrings.UserName)] public string UserName { get { return (string)this[this.userName]; } set { this[this.userName] = value; } } // ConfigurationPropertyCollection properties = new ConfigurationPropertyCollection(); readonly ConfigurationProperty defaultCredentials = new ConfigurationProperty(ConfigurationStrings.DefaultCredentials, typeof(bool), false, ConfigurationPropertyOptions.None); readonly ConfigurationProperty host = new ConfigurationProperty(ConfigurationStrings.Host, typeof(string), null, ConfigurationPropertyOptions.None); readonly ConfigurationProperty clientDomain = new ConfigurationProperty(ConfigurationStrings.ClientDomain, typeof(string), null, ConfigurationPropertyOptions.None); readonly ConfigurationProperty password = new ConfigurationProperty(ConfigurationStrings.Password, typeof(string), null, ConfigurationPropertyOptions.None); readonly ConfigurationProperty port = new ConfigurationProperty(ConfigurationStrings.Port, typeof(int), 25, null, new IntegerValidator(IPEndPoint.MinPort+1, IPEndPoint.MaxPort), ConfigurationPropertyOptions.None); readonly ConfigurationProperty userName = new ConfigurationProperty(ConfigurationStrings.UserName, typeof(string), null, ConfigurationPropertyOptions.None); readonly ConfigurationProperty targetName = new ConfigurationProperty(ConfigurationStrings.TargetName, typeof(string), null, ConfigurationPropertyOptions.None); } internal sealed class SmtpNetworkElementInternal { internal SmtpNetworkElementInternal(SmtpNetworkElement element) { this.host = element.Host; this.port = element.Port; this.clientDomain = element.ClientDomain; this.targetname = element.TargetName; if (element.DefaultCredentials) { this.credential = (NetworkCredential)CredentialCache.DefaultCredentials; } else if (element.UserName != null && element.UserName.Length > 0) { this.credential = new NetworkCredential(element.UserName, element.Password); } } internal NetworkCredential Credential { get { return this.credential; } } internal string Host { get { return this.host; } } internal string ClientDomain { get { return this.clientDomain; } } internal int Port { get { return this.port; } } internal string TargetName { get { return this.targetname; } } string targetname; string host; string clientDomain; int port; NetworkCredential credential = null; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net.Configuration { using System; using System.Configuration; using System.Net; using System.Net.Mail; using System.Reflection; using System.Security.Permissions; public sealed class SmtpNetworkElement : ConfigurationElement { public SmtpNetworkElement() { this.properties.Add(this.defaultCredentials); this.properties.Add(this.host); this.properties.Add(this.clientDomain); this.properties.Add(this.password); this.properties.Add(this.port); this.properties.Add(this.userName); this.properties.Add(this.targetName); } protected override void PostDeserialize() { // Perf optimization. If the configuration is coming from machine.config // It is safe and we don't need to check for permissions. if (EvaluationContext.IsMachineLevel) return; PropertyInformation portPropertyInfo = ElementInformation.Properties[ConfigurationStrings.Port]; if (portPropertyInfo.ValueOrigin == PropertyValueOrigin.SetHere && (int)portPropertyInfo.Value != (int)portPropertyInfo.DefaultValue) { try { (new SmtpPermission(SmtpAccess.ConnectToUnrestrictedPort)).Demand(); } catch (Exception exception) { throw new ConfigurationErrorsException( SR.GetString(SR.net_config_property_permission, portPropertyInfo.Name), exception); } } } protected override ConfigurationPropertyCollection Properties { get { return this.properties; } } [ConfigurationProperty(ConfigurationStrings.DefaultCredentials, DefaultValue = false)] public bool DefaultCredentials { get { return (bool)this[this.defaultCredentials]; } set { this[this.defaultCredentials] = value; } } [ConfigurationProperty(ConfigurationStrings.Host)] public string Host { get { return (string)this[this.host]; } set { this[this.host] = value; } } [ConfigurationProperty(ConfigurationStrings.ClientDomain)] public string ClientDomain { get { return (string)this[this.clientDomain]; } set { this[this.clientDomain] = value; } } [ConfigurationProperty(ConfigurationStrings.TargetName)] public string TargetName { get { return (string)this[this.targetName]; } set { this[this.targetName] = value; } } [ConfigurationProperty(ConfigurationStrings.Password)] public string Password { get { return (string)this[this.password]; } set { this[this.password] = value; } } [ConfigurationProperty(ConfigurationStrings.Port, DefaultValue = 25)] public int Port { get { return (int)this[this.port]; } set { // this[this.port] = value; } } [ConfigurationProperty(ConfigurationStrings.UserName)] public string UserName { get { return (string)this[this.userName]; } set { this[this.userName] = value; } } // ConfigurationPropertyCollection properties = new ConfigurationPropertyCollection(); readonly ConfigurationProperty defaultCredentials = new ConfigurationProperty(ConfigurationStrings.DefaultCredentials, typeof(bool), false, ConfigurationPropertyOptions.None); readonly ConfigurationProperty host = new ConfigurationProperty(ConfigurationStrings.Host, typeof(string), null, ConfigurationPropertyOptions.None); readonly ConfigurationProperty clientDomain = new ConfigurationProperty(ConfigurationStrings.ClientDomain, typeof(string), null, ConfigurationPropertyOptions.None); readonly ConfigurationProperty password = new ConfigurationProperty(ConfigurationStrings.Password, typeof(string), null, ConfigurationPropertyOptions.None); readonly ConfigurationProperty port = new ConfigurationProperty(ConfigurationStrings.Port, typeof(int), 25, null, new IntegerValidator(IPEndPoint.MinPort+1, IPEndPoint.MaxPort), ConfigurationPropertyOptions.None); readonly ConfigurationProperty userName = new ConfigurationProperty(ConfigurationStrings.UserName, typeof(string), null, ConfigurationPropertyOptions.None); readonly ConfigurationProperty targetName = new ConfigurationProperty(ConfigurationStrings.TargetName, typeof(string), null, ConfigurationPropertyOptions.None); } internal sealed class SmtpNetworkElementInternal { internal SmtpNetworkElementInternal(SmtpNetworkElement element) { this.host = element.Host; this.port = element.Port; this.clientDomain = element.ClientDomain; this.targetname = element.TargetName; if (element.DefaultCredentials) { this.credential = (NetworkCredential)CredentialCache.DefaultCredentials; } else if (element.UserName != null && element.UserName.Length > 0) { this.credential = new NetworkCredential(element.UserName, element.Password); } } internal NetworkCredential Credential { get { return this.credential; } } internal string Host { get { return this.host; } } internal string ClientDomain { get { return this.clientDomain; } } internal int Port { get { return this.port; } } internal string TargetName { get { return this.targetname; } } string targetname; string host; string clientDomain; int port; NetworkCredential credential = null; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
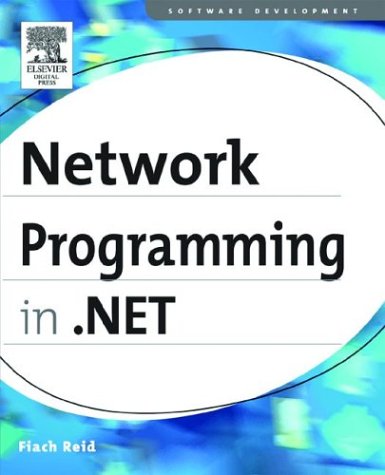
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TemplateComponentConnector.cs
- TreeBuilderXamlTranslator.cs
- AxImporter.cs
- RelationshipFixer.cs
- DelayDesigner.cs
- KeyEventArgs.cs
- ThicknessAnimationUsingKeyFrames.cs
- ModelFactory.cs
- DataGridViewEditingControlShowingEventArgs.cs
- MouseGesture.cs
- ColorAnimation.cs
- VirtualizingPanel.cs
- _ListenerAsyncResult.cs
- EntityDesignPluralizationHandler.cs
- DataGridViewColumnCollection.cs
- SoapFaultCodes.cs
- ResourceManagerWrapper.cs
- Image.cs
- ImageConverter.cs
- StandardToolWindows.cs
- MsmqAuthenticationMode.cs
- SystemEvents.cs
- DataSourceSelectArguments.cs
- GroupBoxRenderer.cs
- BamlRecordHelper.cs
- FontCollection.cs
- SourceSwitch.cs
- XmlSchemaSequence.cs
- TemplateControlCodeDomTreeGenerator.cs
- PrimitiveXmlSerializers.cs
- DataGridPagerStyle.cs
- Signature.cs
- EntityConnectionStringBuilder.cs
- Options.cs
- Button.cs
- FontClient.cs
- ToolStripSeparatorRenderEventArgs.cs
- ResourceDescriptionAttribute.cs
- MarkupCompilePass1.cs
- PrivilegedConfigurationManager.cs
- XmlDataSourceView.cs
- PtsHost.cs
- JsonEncodingStreamWrapper.cs
- SoapReflectionImporter.cs
- ValueUnavailableException.cs
- AttributeProviderAttribute.cs
- TextSpanModifier.cs
- EncoderNLS.cs
- DialogResultConverter.cs
- DataGridViewRowHeaderCell.cs
- IgnoreDeviceFilterElementCollection.cs
- TimeEnumHelper.cs
- NetworkStream.cs
- GridViewPageEventArgs.cs
- DataGridViewTextBoxColumn.cs
- NameValueFileSectionHandler.cs
- CopyCodeAction.cs
- HtmlTitle.cs
- TimersDescriptionAttribute.cs
- Int32RectConverter.cs
- RectangleF.cs
- IntPtr.cs
- CustomActivityDesigner.cs
- DataBindingCollection.cs
- SafeHandle.cs
- QueryHandler.cs
- TreeViewItemAutomationPeer.cs
- TileModeValidation.cs
- AttachInfo.cs
- Base64Encoding.cs
- ObfuscationAttribute.cs
- ListViewInsertedEventArgs.cs
- SpecularMaterial.cs
- PngBitmapDecoder.cs
- FunctionImportElement.cs
- MarkedHighlightComponent.cs
- PolyQuadraticBezierSegmentFigureLogic.cs
- TypeElementCollection.cs
- NameValueSectionHandler.cs
- ListControl.cs
- SelectionProcessor.cs
- SingleConverter.cs
- WindowsAuthenticationModule.cs
- ReturnEventArgs.cs
- Viewport3DVisual.cs
- CollectionChangedEventManager.cs
- TdsParserSessionPool.cs
- Span.cs
- ClientProxyGenerator.cs
- OperationSelectorBehavior.cs
- FileFormatException.cs
- RequestCache.cs
- ScrollableControlDesigner.cs
- AtomPub10ServiceDocumentFormatter.cs
- BooleanStorage.cs
- GeneralTransform3DTo2D.cs
- TextBoxAutoCompleteSourceConverter.cs
- WorkflowTransactionService.cs
- PKCS1MaskGenerationMethod.cs
- _HTTPDateParse.cs