Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / NetFx35 / System.ServiceModel.Web / System / ServiceModel / Channels / HttpStreamXmlDictionaryWriter.cs / 1 / HttpStreamXmlDictionaryWriter.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Channels { using System.Collections.Generic; using System.Collections.ObjectModel; using System.IO; using System.Net; using System.Xml; using System.Text; using System.ServiceModel.Dispatcher; using System.ServiceModel.Syndication; class HttpStreamXmlDictionaryWriter : XmlDictionaryWriter { WriteState state; Stream stream; public HttpStreamXmlDictionaryWriter(Stream stream) { if (stream == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("stream"); } this.stream = stream; this.state = WriteState.Start; } public override WriteState WriteState { get { return this.state; } } public override void Close() { if (this.state != WriteState.Closed) { this.state = WriteState.Closed; stream.Close(); } } public override void Flush() { stream.Flush(); } public override string LookupPrefix(string ns) { if (ns == string.Empty) { return string.Empty; } else if (ns == Atom10FeedFormatter.XmlNs) { return "xml"; } else if (ns == Atom10FeedFormatter.XmlNsNs) { return "xmlns"; } else { return null; } } public override void WriteBase64(byte[] buffer, int index, int count) { if (buffer == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("buffer")); } if (index < 0) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("index")); } if (count < 0) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("count")); } if (count > buffer.Length - index) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("count", SR2.GetString(SR2.SizeExceedsRemainingBufferSpace, buffer.Length - index))); } ThrowIfClosed(); this.stream.Write(buffer, index, count); this.state = WriteState.Content; } public override void WriteCData(string text) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException()); } public override void WriteCharEntity(char ch) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException()); } public override void WriteChars(char[] buffer, int index, int count) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException()); } public override void WriteComment(string text) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException()); } public override void WriteDocType(string name, string pubid, string sysid, string subset) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException()); } public override void WriteEndAttribute() { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException()); } public override void WriteEndDocument() { } public override void WriteEndElement() { ThrowIfClosed(); if (this.state != WriteState.Element && this.state != WriteState.Content) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new XmlException(SR2.GetString(SR2.EndElementWithoutStartElement))); } } public override void WriteEntityRef(string name) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException()); } public override void WriteFullEndElement() { ThrowIfClosed(); if (this.state != WriteState.Element && this.state != WriteState.Content) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new XmlException(SR2.GetString(SR2.EndElementWithoutStartElement))); } } public override void WriteProcessingInstruction(string name, string text) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException()); } public override void WriteRaw(string data) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException()); } public override void WriteRaw(char[] buffer, int index, int count) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException()); } public override void WriteStartAttribute(string prefix, string localName, string ns) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException()); } public override void WriteStartDocument(bool standalone) { ThrowIfClosed(); } public override void WriteStartDocument() { ThrowIfClosed(); } public override void WriteStartElement(string prefix, string localName, string ns) { ThrowIfClosed(); if (!string.IsNullOrEmpty(prefix) || !string.IsNullOrEmpty(ns) || localName != HttpStreamMessage.StreamElementName) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException()); } this.state = WriteState.Element; } public override void WriteString(string text) { byte[] buffer = Convert.FromBase64String(text); WriteBase64(buffer, 0, buffer.Length); } public override void WriteSurrogateCharEntity(char lowChar, char highChar) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException()); } public override void WriteWhitespace(string ws) { if (ws == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("ws"); } if (ws.Length != 0) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException()); } } protected void ThrowIfClosed() { if (this.state == WriteState.Closed) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR2.GetString(SR2.XmlWriterClosed))); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
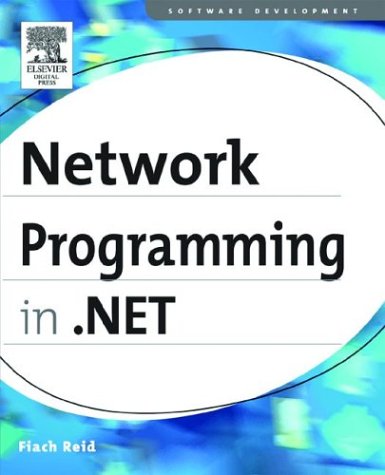
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AlgoModule.cs
- WebPartDeleteVerb.cs
- ValidatedControlConverter.cs
- DelayDesigner.cs
- Dictionary.cs
- ResourcePool.cs
- WebPartEditorApplyVerb.cs
- XmlDictionary.cs
- ConsumerConnectionPointCollection.cs
- UpdateManifestForBrowserApplication.cs
- XamlStream.cs
- ISAPIApplicationHost.cs
- LinkConverter.cs
- HtmlContainerControl.cs
- ItemContainerGenerator.cs
- DataGridSortingEventArgs.cs
- SqlDependencyUtils.cs
- OverrideMode.cs
- IdnMapping.cs
- ParallelDesigner.cs
- TableHeaderCell.cs
- WebPartEditVerb.cs
- MissingFieldException.cs
- ListViewInsertionMark.cs
- SecurityAppliedMessage.cs
- XmlSchemaExporter.cs
- ExtensibleClassFactory.cs
- DictionaryKeyPropertyAttribute.cs
- Expressions.cs
- BamlReader.cs
- FixUpCollection.cs
- ObjectDataProvider.cs
- RemoteWebConfigurationHostServer.cs
- ResourceDictionaryCollection.cs
- SByteStorage.cs
- AttributeTableBuilder.cs
- Message.cs
- PartialTrustVisibleAssembly.cs
- controlskin.cs
- WebPartVerbCollection.cs
- SortAction.cs
- CommandField.cs
- shaperfactoryquerycacheentry.cs
- XamlUtilities.cs
- WebControlsSection.cs
- PenContexts.cs
- DesignerUtility.cs
- ServicePointManagerElement.cs
- BypassElement.cs
- EventPrivateKey.cs
- WindowsMenu.cs
- PackageDigitalSignatureManager.cs
- DataGridItemCollection.cs
- loginstatus.cs
- DataReceivedEventArgs.cs
- RemoteCryptoRsaServiceProvider.cs
- StylusButtonEventArgs.cs
- UnmanagedMarshal.cs
- NetTcpBindingCollectionElement.cs
- NavigationWindow.cs
- TypeExtension.cs
- GeometryGroup.cs
- CryptoConfig.cs
- ILGenerator.cs
- filewebrequest.cs
- HtmlUtf8RawTextWriter.cs
- recordstatefactory.cs
- XmlDocumentSchema.cs
- AssociatedControlConverter.cs
- PropertyDescriptorCollection.cs
- Stopwatch.cs
- safex509handles.cs
- FormViewPageEventArgs.cs
- StateFinalizationActivity.cs
- TableLayoutStyle.cs
- BoundField.cs
- METAHEADER.cs
- ColorKeyFrameCollection.cs
- AttributedMetaModel.cs
- MultiBindingExpression.cs
- RoleGroup.cs
- ExpandoObject.cs
- SoapFault.cs
- ExtentJoinTreeNode.cs
- Ipv6Element.cs
- SortKey.cs
- XPathDocumentIterator.cs
- ContourSegment.cs
- CommandField.cs
- PrintPreviewGraphics.cs
- CompareValidator.cs
- ProcessHostMapPath.cs
- StatusStrip.cs
- OperatingSystem.cs
- Vector3dCollection.cs
- HttpStaticObjectsCollectionBase.cs
- DiscreteKeyFrames.cs
- PermissionRequestEvidence.cs
- RegexNode.cs
- FreezableOperations.cs