Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / Net / System / Net / Configuration / ServicePointManagerElement.cs / 1 / ServicePointManagerElement.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net.Configuration { using System; using System.Configuration; using System.Reflection; using System.Security.Permissions; public sealed class ServicePointManagerElement : ConfigurationElement { public ServicePointManagerElement() { this.properties.Add(this.checkCertificateName); this.properties.Add(this.checkCertificateRevocationList); this.properties.Add(this.dnsRefreshTimeout); this.properties.Add(this.enableDnsRoundRobin); this.properties.Add(this.expect100Continue); this.properties.Add(this.useNagleAlgorithm); } protected override void PostDeserialize() { // Perf optimization. If the configuration is coming from machine.config // It is safe and we don't need to check for permissions. if (EvaluationContext.IsMachineLevel) return; PropertyInformation[] protectedProperties = { ElementInformation.Properties[ConfigurationStrings.CheckCertificateName], ElementInformation.Properties[ConfigurationStrings.CheckCertificateRevocationList] }; foreach (PropertyInformation property in protectedProperties) if (property.ValueOrigin == PropertyValueOrigin.SetHere) { try { ExceptionHelper.UnmanagedPermission.Demand(); } catch (Exception exception) { throw new ConfigurationErrorsException( SR.GetString(SR.net_config_property_permission, property.Name), exception); } } } [ConfigurationProperty(ConfigurationStrings.CheckCertificateName, DefaultValue = true)] public bool CheckCertificateName { get { return (bool)this[this.checkCertificateName]; } set { this[this.checkCertificateName] = value; } } [ConfigurationProperty(ConfigurationStrings.CheckCertificateRevocationList, DefaultValue = false)] public bool CheckCertificateRevocationList { get { return (bool)this[this.checkCertificateRevocationList]; } set { this[this.checkCertificateRevocationList] = value; } } [ConfigurationProperty(ConfigurationStrings.DnsRefreshTimeout, DefaultValue = (int)( 2 * 60 * 1000))] public int DnsRefreshTimeout { get { return (int)this[this.dnsRefreshTimeout]; } set { this[this.dnsRefreshTimeout] = value; } } [ConfigurationProperty(ConfigurationStrings.EnableDnsRoundRobin, DefaultValue = false)] public bool EnableDnsRoundRobin { get { return (bool)this[this.enableDnsRoundRobin]; } set { this[this.enableDnsRoundRobin] = value; } } [ConfigurationProperty(ConfigurationStrings.Expect100Continue, DefaultValue = true)] public bool Expect100Continue { get { return (bool)this[this.expect100Continue]; } set { this[this.expect100Continue] = value; } } [ConfigurationProperty(ConfigurationStrings.UseNagleAlgorithm, DefaultValue=true)] public bool UseNagleAlgorithm { get { return (bool)this[this.useNagleAlgorithm]; } set { this[this.useNagleAlgorithm] = value; } } protected override ConfigurationPropertyCollection Properties { get { return this.properties; } } ConfigurationPropertyCollection properties = new ConfigurationPropertyCollection(); readonly ConfigurationProperty checkCertificateName = new ConfigurationProperty(ConfigurationStrings.CheckCertificateName, typeof(bool), true, ConfigurationPropertyOptions.None); readonly ConfigurationProperty checkCertificateRevocationList = new ConfigurationProperty(ConfigurationStrings.CheckCertificateRevocationList, typeof(bool), false, ConfigurationPropertyOptions.None); readonly ConfigurationProperty dnsRefreshTimeout = new ConfigurationProperty(ConfigurationStrings.DnsRefreshTimeout, typeof(int), 2 * 60 * 1000, null, new TimeoutValidator(true), ConfigurationPropertyOptions.None); readonly ConfigurationProperty enableDnsRoundRobin = new ConfigurationProperty(ConfigurationStrings.EnableDnsRoundRobin, typeof(bool), false, ConfigurationPropertyOptions.None); readonly ConfigurationProperty expect100Continue = new ConfigurationProperty(ConfigurationStrings.Expect100Continue, typeof(bool), true, ConfigurationPropertyOptions.None); readonly ConfigurationProperty useNagleAlgorithm = new ConfigurationProperty(ConfigurationStrings.UseNagleAlgorithm, typeof(bool), true, ConfigurationPropertyOptions.None); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net.Configuration { using System; using System.Configuration; using System.Reflection; using System.Security.Permissions; public sealed class ServicePointManagerElement : ConfigurationElement { public ServicePointManagerElement() { this.properties.Add(this.checkCertificateName); this.properties.Add(this.checkCertificateRevocationList); this.properties.Add(this.dnsRefreshTimeout); this.properties.Add(this.enableDnsRoundRobin); this.properties.Add(this.expect100Continue); this.properties.Add(this.useNagleAlgorithm); } protected override void PostDeserialize() { // Perf optimization. If the configuration is coming from machine.config // It is safe and we don't need to check for permissions. if (EvaluationContext.IsMachineLevel) return; PropertyInformation[] protectedProperties = { ElementInformation.Properties[ConfigurationStrings.CheckCertificateName], ElementInformation.Properties[ConfigurationStrings.CheckCertificateRevocationList] }; foreach (PropertyInformation property in protectedProperties) if (property.ValueOrigin == PropertyValueOrigin.SetHere) { try { ExceptionHelper.UnmanagedPermission.Demand(); } catch (Exception exception) { throw new ConfigurationErrorsException( SR.GetString(SR.net_config_property_permission, property.Name), exception); } } } [ConfigurationProperty(ConfigurationStrings.CheckCertificateName, DefaultValue = true)] public bool CheckCertificateName { get { return (bool)this[this.checkCertificateName]; } set { this[this.checkCertificateName] = value; } } [ConfigurationProperty(ConfigurationStrings.CheckCertificateRevocationList, DefaultValue = false)] public bool CheckCertificateRevocationList { get { return (bool)this[this.checkCertificateRevocationList]; } set { this[this.checkCertificateRevocationList] = value; } } [ConfigurationProperty(ConfigurationStrings.DnsRefreshTimeout, DefaultValue = (int)( 2 * 60 * 1000))] public int DnsRefreshTimeout { get { return (int)this[this.dnsRefreshTimeout]; } set { this[this.dnsRefreshTimeout] = value; } } [ConfigurationProperty(ConfigurationStrings.EnableDnsRoundRobin, DefaultValue = false)] public bool EnableDnsRoundRobin { get { return (bool)this[this.enableDnsRoundRobin]; } set { this[this.enableDnsRoundRobin] = value; } } [ConfigurationProperty(ConfigurationStrings.Expect100Continue, DefaultValue = true)] public bool Expect100Continue { get { return (bool)this[this.expect100Continue]; } set { this[this.expect100Continue] = value; } } [ConfigurationProperty(ConfigurationStrings.UseNagleAlgorithm, DefaultValue=true)] public bool UseNagleAlgorithm { get { return (bool)this[this.useNagleAlgorithm]; } set { this[this.useNagleAlgorithm] = value; } } protected override ConfigurationPropertyCollection Properties { get { return this.properties; } } ConfigurationPropertyCollection properties = new ConfigurationPropertyCollection(); readonly ConfigurationProperty checkCertificateName = new ConfigurationProperty(ConfigurationStrings.CheckCertificateName, typeof(bool), true, ConfigurationPropertyOptions.None); readonly ConfigurationProperty checkCertificateRevocationList = new ConfigurationProperty(ConfigurationStrings.CheckCertificateRevocationList, typeof(bool), false, ConfigurationPropertyOptions.None); readonly ConfigurationProperty dnsRefreshTimeout = new ConfigurationProperty(ConfigurationStrings.DnsRefreshTimeout, typeof(int), 2 * 60 * 1000, null, new TimeoutValidator(true), ConfigurationPropertyOptions.None); readonly ConfigurationProperty enableDnsRoundRobin = new ConfigurationProperty(ConfigurationStrings.EnableDnsRoundRobin, typeof(bool), false, ConfigurationPropertyOptions.None); readonly ConfigurationProperty expect100Continue = new ConfigurationProperty(ConfigurationStrings.Expect100Continue, typeof(bool), true, ConfigurationPropertyOptions.None); readonly ConfigurationProperty useNagleAlgorithm = new ConfigurationProperty(ConfigurationStrings.UseNagleAlgorithm, typeof(bool), true, ConfigurationPropertyOptions.None); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
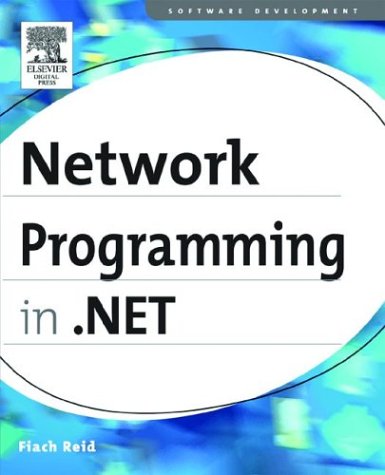
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FontNamesConverter.cs
- ApplyTemplatesAction.cs
- Menu.cs
- OpenFileDialog.cs
- SwitchAttribute.cs
- ClientBuildManager.cs
- SecurityRuntime.cs
- cache.cs
- ReverseInheritProperty.cs
- TemplateField.cs
- SignedXml.cs
- TypeBuilder.cs
- cache.cs
- TakeQueryOptionExpression.cs
- URLIdentityPermission.cs
- StorageBasedPackageProperties.cs
- DependencyPropertyConverter.cs
- MemberInitExpression.cs
- XamlTemplateSerializer.cs
- XmlDocument.cs
- ContentElement.cs
- PerformanceCountersElement.cs
- XamlLoadErrorInfo.cs
- FunctionQuery.cs
- SpeechRecognizer.cs
- HitTestFilterBehavior.cs
- ManifestResourceInfo.cs
- FloaterBaseParaClient.cs
- RepeatInfo.cs
- CompressionTransform.cs
- DataSetFieldSchema.cs
- DataGrid.cs
- ObjectStateFormatter.cs
- DataChangedEventManager.cs
- SimpleWorkerRequest.cs
- ReliabilityContractAttribute.cs
- TextServicesProperty.cs
- Attributes.cs
- DesigntimeLicenseContextSerializer.cs
- PixelFormatConverter.cs
- HttpListenerRequestTraceRecord.cs
- AesCryptoServiceProvider.cs
- CacheModeConverter.cs
- StatusBarPanel.cs
- FileDialog.cs
- WindowsGraphics2.cs
- CompiledRegexRunner.cs
- securitycriticaldata.cs
- SHA1.cs
- TextEditorParagraphs.cs
- TreeNodeStyleCollection.cs
- MetadataUtilsSmi.cs
- LoginNameDesigner.cs
- GradientSpreadMethodValidation.cs
- PartitionedDataSource.cs
- SQLBinaryStorage.cs
- GPStream.cs
- PageParserFilter.cs
- ToolStripArrowRenderEventArgs.cs
- TypeInformation.cs
- RuleAction.cs
- ScriptHandlerFactory.cs
- SimpleExpression.cs
- BrowserTree.cs
- FileEnumerator.cs
- OleDbConnection.cs
- ToolboxComponentsCreatedEventArgs.cs
- CreatingCookieEventArgs.cs
- LocatorBase.cs
- DataGridLinkButton.cs
- MetadataPropertyvalue.cs
- Pair.cs
- ToolStripProgressBar.cs
- SystemDropShadowChrome.cs
- ObjectListShowCommandsEventArgs.cs
- PageEventArgs.cs
- CompositeActivityMarkupSerializer.cs
- WinFormsSecurity.cs
- DataGridViewRowHeightInfoPushedEventArgs.cs
- WebResponse.cs
- Encoder.cs
- XmlWhitespace.cs
- DataSourceControl.cs
- MatrixTransform3D.cs
- AppModelKnownContentFactory.cs
- WindowsSlider.cs
- SqlAliasesReferenced.cs
- sitestring.cs
- RecordsAffectedEventArgs.cs
- RuntimeDelegateArgument.cs
- FloaterParaClient.cs
- CollectionBase.cs
- RectangleHotSpot.cs
- BitmapEffectDrawing.cs
- MetaDataInfo.cs
- TypeGeneratedEventArgs.cs
- InputBuffer.cs
- ContextCorrelationInitializer.cs
- SqlParameterCollection.cs
- AsyncDataRequest.cs