Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Markup / XamlTemplateSerializer.cs / 1305600 / XamlTemplateSerializer.cs
//---------------------------------------------------------------------------- // // File: XamlTemplateSerializer.cs // // Description: // Class that serializes and deserializes Templates. // // Copyright (C) 2004 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Collections; using System.Globalization; using System.IO; using System.Reflection; using System.Text; using System.Xml; using System.Security.Permissions; using MS.Utility; #if !PBTCOMPILER using System.Windows.Data; using System.Windows.Controls; using System.Windows.Documents; #endif #if PBTCOMPILER namespace MS.Internal.Markup #else namespace System.Windows.Markup #endif { ////// Class that knows how to serialize and deserialize Template objects /// internal class XamlTemplateSerializer : XamlSerializer { #if PBTCOMPILER #region Construction ////// Constructor for XamlTemplateSerializer /// public XamlTemplateSerializer() : base() { } internal XamlTemplateSerializer(ParserHooks parserHooks) : base() { _parserHooks = parserHooks; } private ParserHooks _parserHooks = null; #endregion Construction ////// Convert from Xaml read by a token reader into baml being written /// out by a record writer. The context gives mapping information. /// internal override void ConvertXamlToBaml ( XamlReaderHelper tokenReader, ParserContext context, XamlNode xamlNode, BamlRecordWriter bamlWriter) { TemplateXamlParser templateParser = new TemplateXamlParser(tokenReader, context); templateParser.ParserHooks = _parserHooks; templateParser.BamlRecordWriter = bamlWriter; // Process the xamlNode that is passed in so that the element is written to baml templateParser.WriteElementStart((XamlElementStartNode)xamlNode); // Parse the entire Template section now, writing everything out directly to BAML. templateParser.Parse(); } #else ////// If the Template represented by a group of baml records is stored in a dictionary, this /// method will extract the key used for this dictionary from the passed /// collection of baml records. For ControlTemplate, this is the styleTargetType. /// For DataTemplate, this is the DataTemplateKey containing the DataType. /// //CASRemoval:[StrongNameIdentityPermission(SecurityAction.InheritanceDemand, PublicKey = Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_STRING)] internal override object GetDictionaryKey(BamlRecord startRecord, ParserContext parserContext) { object key = null; int numberOfElements = 0; BamlRecord record = startRecord; short ownerTypeId = 0; while (record != null) { if (record.RecordType == BamlRecordType.ElementStart) { BamlElementStartRecord elementStart = record as BamlElementStartRecord; if (++numberOfElements == 1) { // save the type ID of the first element (i.e.) ownerTypeId = elementStart.TypeId; } else { // We didn't find the key before a reading the // VisualTree nodes of the template break; } } else if (record.RecordType == BamlRecordType.Property && numberOfElements == 1) { // look for the TargetType property on the element // or the DataType property on the tag or // TableTemplate.Tree or the tag break; } record = record.Next; } if (key == null) { ThrowException(SRID.StyleNoDictionaryKey, parserContext.LineNumber, parserContext.LinePosition, null); } return key; } // Helper to insert line and position numbers into message, if they are present void ThrowException( string id, int lineNumber, int linePosition, Exception innerException) { string message = SR.Get(id); XamlParseException parseException; // Throw the appropriate execption. If we have line numbers, then we are // parsing a xaml file, so throw a xaml exception. Otherwise were are // parsing a baml file. if (lineNumber > 0) { message += " "; message += SR.Get(SRID.ParserLineAndOffset, lineNumber.ToString(CultureInfo.CurrentUICulture), linePosition.ToString(CultureInfo.CurrentUICulture)); parseException = new XamlParseException(message, lineNumber, linePosition); } else { parseException = new XamlParseException(message); } throw parseException; } #endif // !PBTCOMPILER #region Data // Constants used for emitting specific properties and attributes for a Style internal const string ControlTemplateTagName = "ControlTemplate"; internal const string DataTemplateTagName = "DataTemplate"; internal const string HierarchicalDataTemplateTagName = "HierarchicalDataTemplate"; internal const string ItemsPanelTemplateTagName = "ItemsPanelTemplate"; internal const string TargetTypePropertyName = "TargetType"; internal const string DataTypePropertyName = "DataType"; internal const string TriggersPropertyName = "Triggers"; internal const string ResourcesPropertyName = "Resources"; internal const string SettersPropertyName = "Setters"; internal const string ItemsSourcePropertyName = "ItemsSource"; internal const string ItemTemplatePropertyName = "ItemTemplate"; internal const string ItemTemplateSelectorPropertyName = "ItemTemplateSelector"; internal const string ItemContainerStylePropertyName = "ItemContainerStyle"; internal const string ItemContainerStyleSelectorPropertyName = "ItemContainerStyleSelector"; internal const string ItemStringFormatPropertyName = "ItemStringFormat"; internal const string ItemBindingGroupPropertyName = "ItemBindingGroup"; internal const string AlternationCountPropertyName = "AlternationCount"; internal const string ControlTemplateTriggersFullPropertyName = ControlTemplateTagName + "." + TriggersPropertyName; internal const string ControlTemplateResourcesFullPropertyName = ControlTemplateTagName + "." + ResourcesPropertyName; internal const string DataTemplateTriggersFullPropertyName = DataTemplateTagName + "." + TriggersPropertyName; internal const string DataTemplateResourcesFullPropertyName = DataTemplateTagName + "." + ResourcesPropertyName; internal const string HierarchicalDataTemplateTriggersFullPropertyName = HierarchicalDataTemplateTagName + "." + TriggersPropertyName; internal const string HierarchicalDataTemplateItemsSourceFullPropertyName = HierarchicalDataTemplateTagName + "." + ItemsSourcePropertyName; internal const string HierarchicalDataTemplateItemTemplateFullPropertyName = HierarchicalDataTemplateTagName + "." + ItemTemplatePropertyName; internal const string HierarchicalDataTemplateItemTemplateSelectorFullPropertyName = HierarchicalDataTemplateTagName + "." + ItemTemplateSelectorPropertyName; internal const string HierarchicalDataTemplateItemContainerStyleFullPropertyName = HierarchicalDataTemplateTagName + "." + ItemContainerStylePropertyName; internal const string HierarchicalDataTemplateItemContainerStyleSelectorFullPropertyName = HierarchicalDataTemplateTagName + "." + ItemContainerStyleSelectorPropertyName; internal const string HierarchicalDataTemplateItemStringFormatFullPropertyName = HierarchicalDataTemplateTagName + "." + ItemStringFormatPropertyName; internal const string HierarchicalDataTemplateItemBindingGroupFullPropertyName = HierarchicalDataTemplateTagName + "." + ItemBindingGroupPropertyName; internal const string HierarchicalDataTemplateAlternationCountFullPropertyName = HierarchicalDataTemplateTagName + "." + AlternationCountPropertyName; internal const string PropertyTriggerPropertyName = "Property"; internal const string PropertyTriggerValuePropertyName = "Value"; internal const string PropertyTriggerSourceName = "SourceName"; internal const string PropertyTriggerEnterActions = "EnterActions"; internal const string PropertyTriggerExitActions = "ExitActions"; internal const string DataTriggerBindingPropertyName = "Binding"; internal const string EventTriggerEventName = "RoutedEvent"; internal const string EventTriggerSourceName = "SourceName"; internal const string EventTriggerActions = "Actions"; internal const string MultiPropertyTriggerConditionsPropertyName = "Conditions"; internal const string SetterTagName = "Setter"; internal const string SetterPropertyAttributeName = "Property"; internal const string SetterValueAttributeName = "Value"; internal const string SetterTargetAttributeName = "TargetName"; internal const string SetterEventAttributeName = "Event"; internal const string SetterHandlerAttributeName = "Handler"; #if HANDLEDEVENTSTOO internal const string SetterHandledEventsTooAttributeName = "HandledEventsToo"; #endif #endregion Data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.element BamlPropertyRecord propertyRecord = record as BamlPropertyRecord; short attributeOwnerTypeId; string attributeName; BamlAttributeUsage attributeUsage; parserContext.MapTable.GetAttributeInfoFromId(propertyRecord.AttributeId, out attributeOwnerTypeId, out attributeName, out attributeUsage); if (attributeOwnerTypeId == ownerTypeId) { if (attributeName == TargetTypePropertyName) { key = parserContext.XamlTypeMapper.GetDictionaryKey(propertyRecord.Value, parserContext); } else if (attributeName == DataTypePropertyName) { object dataType = parserContext.XamlTypeMapper.GetDictionaryKey(propertyRecord.Value, parserContext); Exception ex = TemplateKey.ValidateDataType(dataType, null); if (ex != null) { ThrowException(SRID.TemplateBadDictionaryKey, parserContext.LineNumber, parserContext.LinePosition, ex); } // key = new DataTemplateKey(dataType); } } } else if (record.RecordType == BamlRecordType.PropertyComplexStart || record.RecordType == BamlRecordType.PropertyIListStart || record.RecordType == BamlRecordType.ElementEnd) { // We didn't find the targetType before a complex property like // FrameworkTemplate.VisualTree or the
Link Menu
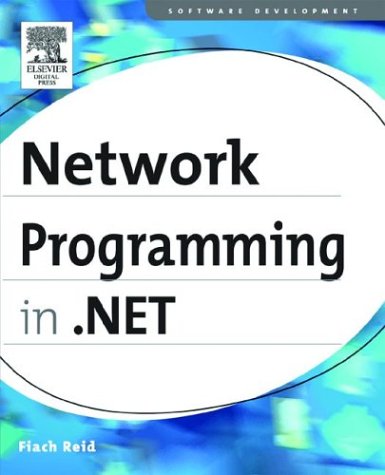
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ServiceSecurityAuditBehavior.cs
- AudioException.cs
- BezierSegment.cs
- InvalidDataException.cs
- TypeSemantics.cs
- PointAnimationBase.cs
- EdmError.cs
- NotSupportedException.cs
- RowParagraph.cs
- EventLogEntryCollection.cs
- BoundsDrawingContextWalker.cs
- ItemCheckEvent.cs
- UIElementParagraph.cs
- CalendarDateChangedEventArgs.cs
- KoreanLunisolarCalendar.cs
- ProfileServiceManager.cs
- ViewPort3D.cs
- XmlCollation.cs
- ProxySimple.cs
- SpeakProgressEventArgs.cs
- DecimalConstantAttribute.cs
- ConfigurationSectionCollection.cs
- OdbcUtils.cs
- MessagePropertyDescriptionCollection.cs
- SocketException.cs
- ManagementInstaller.cs
- ApplicationFileCodeDomTreeGenerator.cs
- NativeCppClassAttribute.cs
- FullTrustAssemblyCollection.cs
- Grid.cs
- DataRow.cs
- LocalValueEnumerator.cs
- WebPartConnection.cs
- TextSimpleMarkerProperties.cs
- SpecialFolderEnumConverter.cs
- SymmetricKeyWrap.cs
- XNodeNavigator.cs
- MeasureItemEvent.cs
- GraphicsContext.cs
- UpdatePanel.cs
- FormsAuthenticationEventArgs.cs
- BooleanProjectedSlot.cs
- DataGridViewCellCollection.cs
- List.cs
- CodeAccessPermission.cs
- ClientCredentialsSecurityTokenManager.cs
- TableStyle.cs
- CommonRemoteMemoryBlock.cs
- shaper.cs
- xmlglyphRunInfo.cs
- ProjectionCamera.cs
- AutoScrollHelper.cs
- VectorAnimationUsingKeyFrames.cs
- FixedFindEngine.cs
- Operand.cs
- XPathEmptyIterator.cs
- ObjectReferenceStack.cs
- EntityTypeEmitter.cs
- SamlSecurityToken.cs
- EncodingNLS.cs
- RtType.cs
- XmlSecureResolver.cs
- DataGridViewImageColumn.cs
- Emitter.cs
- ErrorsHelper.cs
- IMembershipProvider.cs
- QuestionEventArgs.cs
- ProtocolProfile.cs
- DispatchChannelSink.cs
- DriveInfo.cs
- XPathBinder.cs
- SettingsAttributes.cs
- XmlMemberMapping.cs
- ProgressBarBrushConverter.cs
- AuthenticationService.cs
- EventSourceCreationData.cs
- DBSchemaTable.cs
- ManifestResourceInfo.cs
- SetMemberBinder.cs
- TargetException.cs
- SoapElementAttribute.cs
- DefaultDiscoveryService.cs
- BufferedStream.cs
- RootContext.cs
- ColumnMapCopier.cs
- Inline.cs
- DataSourceCacheDurationConverter.cs
- FacetDescription.cs
- XmlHierarchicalDataSourceView.cs
- BuildResult.cs
- InputBinding.cs
- StorageConditionPropertyMapping.cs
- Control.cs
- TemplateBindingExtension.cs
- Module.cs
- BindingExpressionBase.cs
- TiffBitmapDecoder.cs
- PtsHelper.cs
- FileSystemInfo.cs
- TextRange.cs