Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / MS / Internal / Annotations / AnnotationMap.cs / 1305600 / AnnotationMap.cs
//---------------------------------------------------------------------------- //// Copyright(C) Microsoft Corporation. All rights reserved. // // // Description: // AnnotationMap contains the implementation of the map // map between annotation id and attached annotations used by the service // // History: // 11/11/2003 magedz: created // 10/22/2004 rruiz: Moved this class to MS.Internal namespace. // // Copyright(C) 2002 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Windows.Annotations; namespace MS.Internal.Annotations { ////// The AnnotationMap holds a map between the Id's of annotations and IAttachedAnnotations /// internal class AnnotationMap { ////// Add an IAttachedAnnotation to the annotation map. /// /// the IAttachedAnnotation to be added to the map internal void AddAttachedAnnotation(IAttachedAnnotation attachedAnnotation) { Listlist = null; if (!_annotationIdToAttachedAnnotations.TryGetValue(attachedAnnotation.Annotation.Id, out list)) { list = new List (1); _annotationIdToAttachedAnnotations.Add(attachedAnnotation.Annotation.Id, list); } list.Add(attachedAnnotation); } /// /// Remove an IAttachedAnnotation from the annotation map. /// /// internal void RemoveAttachedAnnotation(IAttachedAnnotation attachedAnnotation) { Listlist = null; if (_annotationIdToAttachedAnnotations.TryGetValue(attachedAnnotation.Annotation.Id, out list)) { list.Remove(attachedAnnotation); if (list.Count == 0) { _annotationIdToAttachedAnnotations.Remove(attachedAnnotation.Annotation.Id); } } } /// /// Returns whether or not there are any annotations currently loaded. This can be /// used to avoid costly walks of the tree. /// internal bool IsEmpty { get { return _annotationIdToAttachedAnnotations.Count == 0; } } ////// Return a list of IAttachedAnnotations for a given annotation id /// /// ///list of IAttachedAnnotations internal ListGetAttachedAnnotations(Guid annotationId) { List list = null; if (!_annotationIdToAttachedAnnotations.TryGetValue(annotationId, out list)) { // return empty list if annotation id not found return _emptyList; } Debug.Assert(list != null, "there should be an attached annotation list for the annotationId: " + annotationId.ToString()); return list; } /// /// Return a list of all IAttachedAnnotations in the map /// ///list of IAttachedAnnotations internal ListGetAllAttachedAnnotations() { List result = new List (_annotationIdToAttachedAnnotations.Keys.Count); foreach (Guid annId in _annotationIdToAttachedAnnotations.Keys) { List list = _annotationIdToAttachedAnnotations[annId]; result.AddRange(list); } if (result.Count == 0) { return _emptyList; } return result; } // hash table to hold annotation id to AttachedAnnotations list private Dictionary > _annotationIdToAttachedAnnotations = new Dictionary >(); // a readonly empty list - cached for performance reasons private static readonly List _emptyList = new List (0); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright(C) Microsoft Corporation. All rights reserved. // // // Description: // AnnotationMap contains the implementation of the map // map between annotation id and attached annotations used by the service // // History: // 11/11/2003 magedz: created // 10/22/2004 rruiz: Moved this class to MS.Internal namespace. // // Copyright(C) 2002 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Windows.Annotations; namespace MS.Internal.Annotations { ////// The AnnotationMap holds a map between the Id's of annotations and IAttachedAnnotations /// internal class AnnotationMap { ////// Add an IAttachedAnnotation to the annotation map. /// /// the IAttachedAnnotation to be added to the map internal void AddAttachedAnnotation(IAttachedAnnotation attachedAnnotation) { Listlist = null; if (!_annotationIdToAttachedAnnotations.TryGetValue(attachedAnnotation.Annotation.Id, out list)) { list = new List (1); _annotationIdToAttachedAnnotations.Add(attachedAnnotation.Annotation.Id, list); } list.Add(attachedAnnotation); } /// /// Remove an IAttachedAnnotation from the annotation map. /// /// internal void RemoveAttachedAnnotation(IAttachedAnnotation attachedAnnotation) { Listlist = null; if (_annotationIdToAttachedAnnotations.TryGetValue(attachedAnnotation.Annotation.Id, out list)) { list.Remove(attachedAnnotation); if (list.Count == 0) { _annotationIdToAttachedAnnotations.Remove(attachedAnnotation.Annotation.Id); } } } /// /// Returns whether or not there are any annotations currently loaded. This can be /// used to avoid costly walks of the tree. /// internal bool IsEmpty { get { return _annotationIdToAttachedAnnotations.Count == 0; } } ////// Return a list of IAttachedAnnotations for a given annotation id /// /// ///list of IAttachedAnnotations internal ListGetAttachedAnnotations(Guid annotationId) { List list = null; if (!_annotationIdToAttachedAnnotations.TryGetValue(annotationId, out list)) { // return empty list if annotation id not found return _emptyList; } Debug.Assert(list != null, "there should be an attached annotation list for the annotationId: " + annotationId.ToString()); return list; } /// /// Return a list of all IAttachedAnnotations in the map /// ///list of IAttachedAnnotations internal ListGetAllAttachedAnnotations() { List result = new List (_annotationIdToAttachedAnnotations.Keys.Count); foreach (Guid annId in _annotationIdToAttachedAnnotations.Keys) { List list = _annotationIdToAttachedAnnotations[annId]; result.AddRange(list); } if (result.Count == 0) { return _emptyList; } return result; } // hash table to hold annotation id to AttachedAnnotations list private Dictionary > _annotationIdToAttachedAnnotations = new Dictionary >(); // a readonly empty list - cached for performance reasons private static readonly List _emptyList = new List (0); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
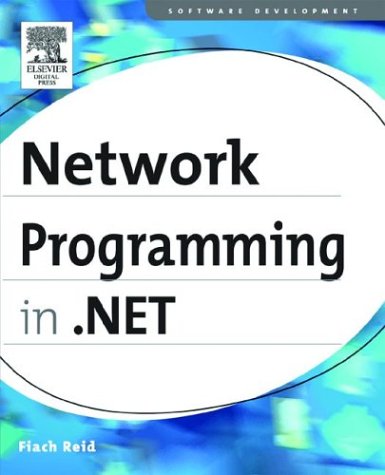
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ContextMarshalException.cs
- Int16.cs
- MD5CryptoServiceProvider.cs
- PropertyChangeTracker.cs
- WebBrowser.cs
- TrackingMemoryStream.cs
- ExpressionBuilder.cs
- PropertyCollection.cs
- DynamicDocumentPaginator.cs
- UnsafeCollabNativeMethods.cs
- PagerStyle.cs
- WindowsTitleBar.cs
- Table.cs
- DataContractFormatAttribute.cs
- ImageDrawing.cs
- precedingquery.cs
- SqlBulkCopy.cs
- PersistChildrenAttribute.cs
- ListenerAdapterBase.cs
- PipeStream.cs
- NetworkAddressChange.cs
- Panel.cs
- ExpressionHelper.cs
- PngBitmapEncoder.cs
- precedingsibling.cs
- HandledEventArgs.cs
- MorphHelper.cs
- KeyFrames.cs
- XsdDuration.cs
- AssemblyFilter.cs
- RichTextBoxAutomationPeer.cs
- ConditionalAttribute.cs
- BitmapInitialize.cs
- SqlConnection.cs
- httpapplicationstate.cs
- EventRouteFactory.cs
- InputProcessorProfilesLoader.cs
- MemberProjectedSlot.cs
- PrinterUnitConvert.cs
- ServiceSecurityAuditBehavior.cs
- SqlCommandSet.cs
- ObjectHandle.cs
- SmtpDateTime.cs
- DataGridTablesFactory.cs
- ServiceNameElement.cs
- TriggerAction.cs
- AuthenticationModuleElement.cs
- RuntimeCompatibilityAttribute.cs
- ReachDocumentReferenceCollectionSerializer.cs
- MDIWindowDialog.cs
- ProfileElement.cs
- DataControlPagerLinkButton.cs
- BitmapEffectInputData.cs
- WorkflowDesigner.cs
- MetafileHeaderWmf.cs
- SchemaNotation.cs
- DataGridViewAdvancedBorderStyle.cs
- CacheOutputQuery.cs
- XpsFilter.cs
- TimeStampChecker.cs
- DataObject.cs
- GetMemberBinder.cs
- XmlMemberMapping.cs
- ThicknessConverter.cs
- KeyedCollection.cs
- MatrixKeyFrameCollection.cs
- StateMachine.cs
- PersonalizationStateQuery.cs
- CodeIterationStatement.cs
- AsyncContentLoadedEventArgs.cs
- Expression.cs
- DataGridPageChangedEventArgs.cs
- DataSourceListEditor.cs
- SchemaHelper.cs
- BooleanToSelectiveScrollingOrientationConverter.cs
- XmlSchemaProviderAttribute.cs
- IDReferencePropertyAttribute.cs
- HttpSocketManager.cs
- BaseParagraph.cs
- Clipboard.cs
- XPathNavigator.cs
- ParseHttpDate.cs
- XmlSchemaInclude.cs
- EdmProperty.cs
- BooleanConverter.cs
- RangeValidator.cs
- AssociationTypeEmitter.cs
- XmlSchemaIdentityConstraint.cs
- EndpointInfoCollection.cs
- RequestDescription.cs
- SpeechSeg.cs
- SettingsAttributeDictionary.cs
- ProxyElement.cs
- SingleTagSectionHandler.cs
- ExpandoClass.cs
- DetailsViewUpdateEventArgs.cs
- AssociativeAggregationOperator.cs
- ClientTarget.cs
- ModelItemImpl.cs
- EncodingNLS.cs