Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / ReverseInheritProperty.cs / 1 / ReverseInheritProperty.cs
using System; using System.Collections; using System.Windows.Media; using System.Windows.Input; using MS.Internal; namespace System.Windows { internal abstract class ReverseInheritProperty { internal ReverseInheritProperty( DependencyPropertyKey flagKey, CoreFlags flagCache, CoreFlags flagChanged) { FlagKey = flagKey; FlagCache = flagCache; FlagChanged = flagChanged; } internal abstract void FireNotifications(UIElement uie, ContentElement ce, UIElement3D uie3D, bool oldValue); internal void OnOriginValueChanged(DependencyObject oldOrigin, DependencyObject newOrigin, ref DeferredElementTreeState oldTreeState) { DeferredElementTreeState treeStateLocalCopy = oldTreeState; oldTreeState = null; // Step #1 // Update the cache flags for all elements in the ancestry // of the element that got turned off and record the changed nodes if (oldOrigin != null) { SetCacheFlagInAncestry(oldOrigin, false, treeStateLocalCopy); } // Step #2 // Update the cache flags for all elements in the ancestry // of the element that got turned on and record the changed nodes if (newOrigin != null) { SetCacheFlagInAncestry(newOrigin, true, null); } // Step #3 // Fire value changed on elements in the ancestry of the element that got turned off. if (oldOrigin != null) { FirePropertyChangeInAncestry(oldOrigin, true /* oldValue */, treeStateLocalCopy); } // Step #4 // Fire value changed on elements in the ancestry of the element that got turned on. if (newOrigin != null) { FirePropertyChangeInAncestry(newOrigin, false /* oldValue */, null); } if (oldTreeState == null && treeStateLocalCopy != null) { // Now that we have applied the old tree state, throw it away. treeStateLocalCopy.Clear(); oldTreeState = treeStateLocalCopy; } } void SetCacheFlagInAncestry(DependencyObject element, bool newValue, DeferredElementTreeState treeState) { // If the cache flag value is undergoing change, record it and // propagate the change to the ancestors. if ((!newValue && IsFlagSet(element, FlagCache)) || (newValue && !IsFlagSet(element, FlagCache))) { SetFlag(element, FlagCache, newValue); // NOTE: we toggle the changed flag instead of setting it so that that way common // ancestors show resultant unchanged and do not receive any change notification. SetFlag(element, FlagChanged, !IsFlagSet(element, FlagChanged)); // Check for block reverse inheritance flag, elements like popup want to set this. { UIElement uie = element as UIElement; if (uie != null) { if (uie.BlockReverseInheritance()) { return; } } ContentElement ce = element as ContentElement; if (ce != null) { if (ce.BlockReverseInheritance()) { return; } } UIElement3D ui3D = element as UIElement3D; if (ui3D != null) { if (ui3D.BlockReverseInheritance()) { return; } } } // Propagate the flag up the visual and logical trees. Note our // minimal optimization check to avoid walking both the core // and logical parents if they are the same. { DependencyObject coreParent = DeferredElementTreeState.GetInputElementParent(element, treeState); DependencyObject logicalParent = DeferredElementTreeState.GetLogicalParent(element, treeState); if (coreParent != null) { SetCacheFlagInAncestry(coreParent, newValue, treeState); } if (logicalParent != null && logicalParent != coreParent) { SetCacheFlagInAncestry(logicalParent, newValue, treeState); } } } } void FirePropertyChangeInAncestry(DependencyObject element, bool oldValue, DeferredElementTreeState treeState) { // If the cache value on the owner has been changed fire changed event if (IsFlagSet(element, FlagChanged)) { SetFlag(element, FlagChanged, false); if (oldValue) { element.ClearValue(FlagKey); } else { element.SetValue(FlagKey, true); } UIElement uie = element as UIElement; ContentElement ce = (uie != null) ? null : element as ContentElement; UIElement3D uie3D = (uie != null || ce != null) ? null : element as UIElement3D; FireNotifications(uie, ce, uie3D, oldValue); // Check for block reverse inheritance flag, elements like popup want to set this. if (uie != null) { if (uie.BlockReverseInheritance()) { return; } } else if (ce != null) { if (ce.BlockReverseInheritance()) { return; } } else if (uie3D != null) { if (uie3D.BlockReverseInheritance()) { return; } } // Call FirePropertyChange up the visual and logical trees. // Note our minimal optimization check to avoid walking both // the core and logical parents if they are the same. { DependencyObject coreParent = DeferredElementTreeState.GetInputElementParent(element, treeState); DependencyObject logicalParent = DeferredElementTreeState.GetLogicalParent(element, treeState); if (coreParent != null) { FirePropertyChangeInAncestry(coreParent, oldValue, treeState); } if (logicalParent != null && logicalParent != coreParent) { FirePropertyChangeInAncestry(logicalParent, oldValue, treeState); } } } } ///////////////////////////////////////////////////////////////////// void SetFlag(DependencyObject o, CoreFlags flag, bool value) { UIElement uie = o as UIElement; ContentElement ce = (uie != null) ? null : o as ContentElement; UIElement3D uie3D = (uie != null || ce != null) ? null : o as UIElement3D; if (uie != null) { uie.WriteFlag(flag, value); } else if (ce != null) { ce.WriteFlag(flag, value); } else if (uie3D != null) { uie3D.WriteFlag(flag, value); } } ///////////////////////////////////////////////////////////////////// bool IsFlagSet(DependencyObject o, CoreFlags flag) { UIElement uie = o as UIElement; ContentElement ce = (uie != null) ? null : o as ContentElement; UIElement3D uie3D = (uie != null || ce != null) ? null : o as UIElement3D; if (uie != null) { return uie.ReadFlag(flag); } else if (ce != null) { return ce.ReadFlag(flag); } else if (uie3D != null) { return uie3D.ReadFlag(flag); } return false; } ///////////////////////////////////////////////////////////////////// internal DependencyPropertyKey FlagKey; internal CoreFlags FlagCache, FlagChanged; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections; using System.Windows.Media; using System.Windows.Input; using MS.Internal; namespace System.Windows { internal abstract class ReverseInheritProperty { internal ReverseInheritProperty( DependencyPropertyKey flagKey, CoreFlags flagCache, CoreFlags flagChanged) { FlagKey = flagKey; FlagCache = flagCache; FlagChanged = flagChanged; } internal abstract void FireNotifications(UIElement uie, ContentElement ce, UIElement3D uie3D, bool oldValue); internal void OnOriginValueChanged(DependencyObject oldOrigin, DependencyObject newOrigin, ref DeferredElementTreeState oldTreeState) { DeferredElementTreeState treeStateLocalCopy = oldTreeState; oldTreeState = null; // Step #1 // Update the cache flags for all elements in the ancestry // of the element that got turned off and record the changed nodes if (oldOrigin != null) { SetCacheFlagInAncestry(oldOrigin, false, treeStateLocalCopy); } // Step #2 // Update the cache flags for all elements in the ancestry // of the element that got turned on and record the changed nodes if (newOrigin != null) { SetCacheFlagInAncestry(newOrigin, true, null); } // Step #3 // Fire value changed on elements in the ancestry of the element that got turned off. if (oldOrigin != null) { FirePropertyChangeInAncestry(oldOrigin, true /* oldValue */, treeStateLocalCopy); } // Step #4 // Fire value changed on elements in the ancestry of the element that got turned on. if (newOrigin != null) { FirePropertyChangeInAncestry(newOrigin, false /* oldValue */, null); } if (oldTreeState == null && treeStateLocalCopy != null) { // Now that we have applied the old tree state, throw it away. treeStateLocalCopy.Clear(); oldTreeState = treeStateLocalCopy; } } void SetCacheFlagInAncestry(DependencyObject element, bool newValue, DeferredElementTreeState treeState) { // If the cache flag value is undergoing change, record it and // propagate the change to the ancestors. if ((!newValue && IsFlagSet(element, FlagCache)) || (newValue && !IsFlagSet(element, FlagCache))) { SetFlag(element, FlagCache, newValue); // NOTE: we toggle the changed flag instead of setting it so that that way common // ancestors show resultant unchanged and do not receive any change notification. SetFlag(element, FlagChanged, !IsFlagSet(element, FlagChanged)); // Check for block reverse inheritance flag, elements like popup want to set this. { UIElement uie = element as UIElement; if (uie != null) { if (uie.BlockReverseInheritance()) { return; } } ContentElement ce = element as ContentElement; if (ce != null) { if (ce.BlockReverseInheritance()) { return; } } UIElement3D ui3D = element as UIElement3D; if (ui3D != null) { if (ui3D.BlockReverseInheritance()) { return; } } } // Propagate the flag up the visual and logical trees. Note our // minimal optimization check to avoid walking both the core // and logical parents if they are the same. { DependencyObject coreParent = DeferredElementTreeState.GetInputElementParent(element, treeState); DependencyObject logicalParent = DeferredElementTreeState.GetLogicalParent(element, treeState); if (coreParent != null) { SetCacheFlagInAncestry(coreParent, newValue, treeState); } if (logicalParent != null && logicalParent != coreParent) { SetCacheFlagInAncestry(logicalParent, newValue, treeState); } } } } void FirePropertyChangeInAncestry(DependencyObject element, bool oldValue, DeferredElementTreeState treeState) { // If the cache value on the owner has been changed fire changed event if (IsFlagSet(element, FlagChanged)) { SetFlag(element, FlagChanged, false); if (oldValue) { element.ClearValue(FlagKey); } else { element.SetValue(FlagKey, true); } UIElement uie = element as UIElement; ContentElement ce = (uie != null) ? null : element as ContentElement; UIElement3D uie3D = (uie != null || ce != null) ? null : element as UIElement3D; FireNotifications(uie, ce, uie3D, oldValue); // Check for block reverse inheritance flag, elements like popup want to set this. if (uie != null) { if (uie.BlockReverseInheritance()) { return; } } else if (ce != null) { if (ce.BlockReverseInheritance()) { return; } } else if (uie3D != null) { if (uie3D.BlockReverseInheritance()) { return; } } // Call FirePropertyChange up the visual and logical trees. // Note our minimal optimization check to avoid walking both // the core and logical parents if they are the same. { DependencyObject coreParent = DeferredElementTreeState.GetInputElementParent(element, treeState); DependencyObject logicalParent = DeferredElementTreeState.GetLogicalParent(element, treeState); if (coreParent != null) { FirePropertyChangeInAncestry(coreParent, oldValue, treeState); } if (logicalParent != null && logicalParent != coreParent) { FirePropertyChangeInAncestry(logicalParent, oldValue, treeState); } } } } ///////////////////////////////////////////////////////////////////// void SetFlag(DependencyObject o, CoreFlags flag, bool value) { UIElement uie = o as UIElement; ContentElement ce = (uie != null) ? null : o as ContentElement; UIElement3D uie3D = (uie != null || ce != null) ? null : o as UIElement3D; if (uie != null) { uie.WriteFlag(flag, value); } else if (ce != null) { ce.WriteFlag(flag, value); } else if (uie3D != null) { uie3D.WriteFlag(flag, value); } } ///////////////////////////////////////////////////////////////////// bool IsFlagSet(DependencyObject o, CoreFlags flag) { UIElement uie = o as UIElement; ContentElement ce = (uie != null) ? null : o as ContentElement; UIElement3D uie3D = (uie != null || ce != null) ? null : o as UIElement3D; if (uie != null) { return uie.ReadFlag(flag); } else if (ce != null) { return ce.ReadFlag(flag); } else if (uie3D != null) { return uie3D.ReadFlag(flag); } return false; } ///////////////////////////////////////////////////////////////////// internal DependencyPropertyKey FlagKey; internal CoreFlags FlagCache, FlagChanged; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
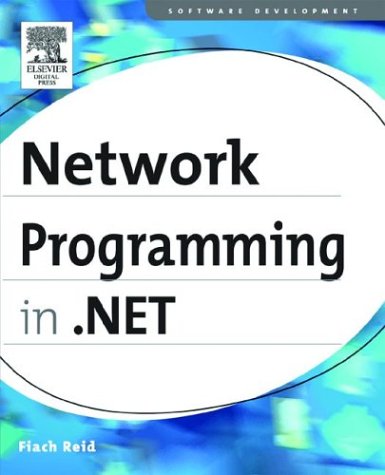
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NavigationCommands.cs
- HtmlPanelAdapter.cs
- SamlDoNotCacheCondition.cs
- TerminatorSinks.cs
- DataSourceXmlAttributeAttribute.cs
- SmiRequestExecutor.cs
- SiteOfOriginContainer.cs
- DrawingBrush.cs
- DesignerSerializationManager.cs
- Symbol.cs
- RoleManagerEventArgs.cs
- ControlBuilder.cs
- Perspective.cs
- _BaseOverlappedAsyncResult.cs
- WpfGeneratedKnownProperties.cs
- DbConnectionPoolOptions.cs
- HtmlWindowCollection.cs
- HwndSourceParameters.cs
- SoapSchemaExporter.cs
- DataGridViewCell.cs
- ToolBarPanel.cs
- ObjectSet.cs
- MembershipPasswordException.cs
- BinaryCommonClasses.cs
- ConnectionProviderAttribute.cs
- XmlnsDefinitionAttribute.cs
- WMIGenerator.cs
- CookieParameter.cs
- FilterElement.cs
- CqlBlock.cs
- RuntimeConfigurationRecord.cs
- SyncMethodInvoker.cs
- AlphaSortedEnumConverter.cs
- NullableConverter.cs
- PointAnimationClockResource.cs
- XmlSchemaFacet.cs
- Int16Storage.cs
- Condition.cs
- TypeConverter.cs
- XmlWrappingReader.cs
- SrgsGrammar.cs
- ObjectAnimationBase.cs
- HttpCookiesSection.cs
- DataServiceRequestException.cs
- ProxyWebPartConnectionCollection.cs
- CategoryEditor.cs
- ChtmlTextWriter.cs
- PageStatePersister.cs
- ResourceDisplayNameAttribute.cs
- CompositeDesignerAccessibleObject.cs
- PartialClassGenerationTaskInternal.cs
- SubclassTypeValidator.cs
- OrCondition.cs
- VectorAnimation.cs
- RegexRunnerFactory.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- BinaryMethodMessage.cs
- DataGridViewCellLinkedList.cs
- AxParameterData.cs
- AstTree.cs
- SerializationStore.cs
- ServiceHostFactory.cs
- DBBindings.cs
- NavigatorInput.cs
- CompositeClientFormatter.cs
- AsnEncodedData.cs
- XmlDocumentSerializer.cs
- ComplexBindingPropertiesAttribute.cs
- TextInfo.cs
- ZipIOLocalFileHeader.cs
- EmptyEnumerator.cs
- XmlAttributeHolder.cs
- IsolatedStorageFileStream.cs
- HtmlInputRadioButton.cs
- VirtualPathUtility.cs
- GridViewRowCollection.cs
- DataGridHeaderBorder.cs
- DataSet.cs
- BindingList.cs
- PropertyToken.cs
- AndCondition.cs
- HtmlUtf8RawTextWriter.cs
- CodeDirectiveCollection.cs
- MsmqIntegrationChannelListener.cs
- Stacktrace.cs
- XpsManager.cs
- Wizard.cs
- EtwTrace.cs
- TextRunProperties.cs
- SecurityIdentifierConverter.cs
- WebConfigurationFileMap.cs
- PlaceHolder.cs
- RangeValueProviderWrapper.cs
- WizardPanel.cs
- InternalConfigHost.cs
- DataBoundLiteralControl.cs
- CodeGeneratorOptions.cs
- FlowLayoutSettings.cs
- CacheDict.cs
- Binding.cs