Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / UIAutomation / UIAutomationClient / System / Windows / Automation / AndCondition.cs / 1305600 / AndCondition.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // // History: // 10/14/2003 : BrendanM - Created // //--------------------------------------------------------------------------- // PRESHARP: In order to avoid generating warnings about unkown message numbers and unknown pragmas. #pragma warning disable 1634, 1691 using System; using MS.Internal.Automation; using System.Windows.Automation; using System.Runtime.InteropServices; namespace System.Windows.Automation { ////// Condition that checks whether a pattern is currently present for a LogicalElement /// #if (INTERNAL_COMPILE) internal class AndCondition : Condition #else public class AndCondition : Condition #endif { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constructor to create a condition that is true if all of the sub-conditions are true /// /// One or more sub-condition public AndCondition( params Condition [ ] conditions ) { Misc.ValidateArgumentNonNull( conditions, "conditions" ); Misc.ValidateArgument( conditions.Length >= 2, SRID.MustBeAtLeastTwoConditions ); foreach( Condition condition in conditions ) { Misc.ValidateArgumentNonNull( condition, "conditions" ); } // clone array to prevent accidental tampering _conditions = (Condition [ ]) conditions.Clone(); _conditionArrayHandle = SafeConditionMemoryHandle.AllocateConditionArrayHandle(_conditions); // DangerousGetHandle() reminds us that the IntPtr we get back could be collected/released/recycled. We're safe here, // because the Conditions are structured in a tree, with the root one (which gets passed to the Uia API) keeping all // others - and their associated data - alive. (Recycling isn't an issue as these are immutable classes.) SetMarshalData(new UiaCoreApi.UiaAndOrCondition(UiaCoreApi.ConditionType.And, _conditionArrayHandle.DangerousGetHandle(), _conditions.Length)); } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Returns an array of the sub conditions for this condition. /// ////// The returned array is a copy; modifying it will not affect the /// state of the condition. /// public Condition [ ] GetConditions() { return (Condition []) _conditions.Clone(); } #endregion Public Methods //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ #region Private Fields Condition [ ] _conditions; SafeConditionMemoryHandle _conditionArrayHandle; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // // History: // 10/14/2003 : BrendanM - Created // //--------------------------------------------------------------------------- // PRESHARP: In order to avoid generating warnings about unkown message numbers and unknown pragmas. #pragma warning disable 1634, 1691 using System; using MS.Internal.Automation; using System.Windows.Automation; using System.Runtime.InteropServices; namespace System.Windows.Automation { ////// Condition that checks whether a pattern is currently present for a LogicalElement /// #if (INTERNAL_COMPILE) internal class AndCondition : Condition #else public class AndCondition : Condition #endif { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constructor to create a condition that is true if all of the sub-conditions are true /// /// One or more sub-condition public AndCondition( params Condition [ ] conditions ) { Misc.ValidateArgumentNonNull( conditions, "conditions" ); Misc.ValidateArgument( conditions.Length >= 2, SRID.MustBeAtLeastTwoConditions ); foreach( Condition condition in conditions ) { Misc.ValidateArgumentNonNull( condition, "conditions" ); } // clone array to prevent accidental tampering _conditions = (Condition [ ]) conditions.Clone(); _conditionArrayHandle = SafeConditionMemoryHandle.AllocateConditionArrayHandle(_conditions); // DangerousGetHandle() reminds us that the IntPtr we get back could be collected/released/recycled. We're safe here, // because the Conditions are structured in a tree, with the root one (which gets passed to the Uia API) keeping all // others - and their associated data - alive. (Recycling isn't an issue as these are immutable classes.) SetMarshalData(new UiaCoreApi.UiaAndOrCondition(UiaCoreApi.ConditionType.And, _conditionArrayHandle.DangerousGetHandle(), _conditions.Length)); } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Returns an array of the sub conditions for this condition. /// ////// The returned array is a copy; modifying it will not affect the /// state of the condition. /// public Condition [ ] GetConditions() { return (Condition []) _conditions.Clone(); } #endregion Public Methods //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ #region Private Fields Condition [ ] _conditions; SafeConditionMemoryHandle _conditionArrayHandle; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
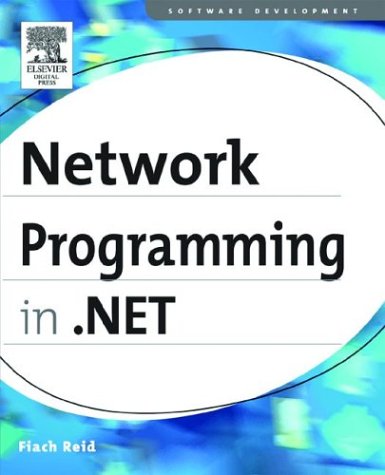
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlNodeAnnotation.cs
- ObjectStateEntryBaseUpdatableDataRecord.cs
- EntityViewGenerationAttribute.cs
- TableDetailsRow.cs
- AvTrace.cs
- OuterGlowBitmapEffect.cs
- IImplicitResourceProvider.cs
- Blend.cs
- RichListBox.cs
- CriticalExceptions.cs
- InvalidChannelBindingException.cs
- MeasurementDCInfo.cs
- SchemaImporterExtensionsSection.cs
- ReadOnlyDictionary.cs
- AsymmetricKeyExchangeDeformatter.cs
- panel.cs
- Number.cs
- DBProviderConfigurationHandler.cs
- SymbolEqualComparer.cs
- Internal.cs
- StringUtil.cs
- MediaTimeline.cs
- DecoderFallbackWithFailureFlag.cs
- ToolStripGrip.cs
- SubqueryTrackingVisitor.cs
- CodePageEncoding.cs
- ClientUtils.cs
- ListenerSessionConnectionReader.cs
- CommentEmitter.cs
- Model3D.cs
- WebPartEventArgs.cs
- KeyGestureConverter.cs
- ConditionValidator.cs
- XslTransformFileEditor.cs
- MetafileEditor.cs
- DataGridCommandEventArgs.cs
- _ListenerRequestStream.cs
- OutOfProcStateClientManager.cs
- BlurEffect.cs
- ContractMapping.cs
- EnumDataContract.cs
- LayoutEvent.cs
- XPathDocument.cs
- RadioButtonRenderer.cs
- FilteredAttributeCollection.cs
- DataContractJsonSerializer.cs
- FileDialog_Vista_Interop.cs
- ImageList.cs
- XpsS0ValidatingLoader.cs
- PrtCap_Builder.cs
- ListViewTableCell.cs
- GenericPrincipal.cs
- XmlBufferReader.cs
- SystemEvents.cs
- CharAnimationBase.cs
- TokenizerHelper.cs
- TransformPattern.cs
- PersonalizationDictionary.cs
- CodeTypeReferenceSerializer.cs
- PublisherMembershipCondition.cs
- PerformanceCounter.cs
- BitmapEditor.cs
- ContainerParagraph.cs
- PictureBox.cs
- Rule.cs
- PointHitTestResult.cs
- BufferedStream.cs
- Italic.cs
- TdsEnums.cs
- securestring.cs
- QuaternionAnimation.cs
- KeyMatchBuilder.cs
- SafeNativeMethodsMilCoreApi.cs
- QueryableDataSource.cs
- SqlUserDefinedTypeAttribute.cs
- WebSysDefaultValueAttribute.cs
- TextTreeRootTextBlock.cs
- UnionCodeGroup.cs
- EncodingNLS.cs
- Double.cs
- HtmlGenericControl.cs
- VisualBrush.cs
- Constants.cs
- SafeFileMappingHandle.cs
- XmlDataDocument.cs
- SqlGenerator.cs
- RecordBuilder.cs
- Matrix.cs
- httpserverutility.cs
- TextDocumentView.cs
- DocumentOrderComparer.cs
- UpdateCommand.cs
- manifestimages.cs
- ObjectStateManager.cs
- CodePropertyReferenceExpression.cs
- FormsAuthenticationCredentials.cs
- UIElement3D.cs
- AssertSection.cs
- DataGridState.cs
- JoinTreeNode.cs