Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / MS / Internal / Data / DisplayMemberTemplateSelector.cs / 1 / DisplayMemberTemplateSelector.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Defines DisplayMemberTemplateSelector class. // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows; using System.Windows.Controls; using System.Windows.Data; using MS.Internal; namespace MS.Internal.Data { // Selects template appropriate for CLR/XML item in order to // display string property at DisplayMemberPath on the item. internal sealed class DisplayMemberTemplateSelector : DataTemplateSelector { ////// Constructor /// /// path to the member to display public DisplayMemberTemplateSelector(string displayMemberPath, string stringFormat) { Debug.Assert(!(String.IsNullOrEmpty(displayMemberPath) && String.IsNullOrEmpty(stringFormat))); _displayMemberPath = displayMemberPath; _stringFormat = stringFormat; } ////// Override this method to return an app specific /// The data content /// The container in which the content is to be displayed ///. /// a app specific template to apply. public override DataTemplate SelectTemplate(object item, DependencyObject container) { if (XmlHelper.IsXmlNode(item)) { if (_xmlNodeContentTemplate == null) { _xmlNodeContentTemplate = new DataTemplate(); FrameworkElementFactory text = ContentPresenter.CreateTextBlockFactory(); Binding binding = new Binding(); binding.XPath = _displayMemberPath; binding.StringFormat = _stringFormat; text.SetBinding(TextBlock.TextProperty, binding); _xmlNodeContentTemplate.VisualTree = text; _xmlNodeContentTemplate.Seal(); } return _xmlNodeContentTemplate; } else { if (_clrNodeContentTemplate == null) { _clrNodeContentTemplate = new DataTemplate(); FrameworkElementFactory text = ContentPresenter.CreateTextBlockFactory(); Binding binding = new Binding(); binding.Path = new PropertyPath(_displayMemberPath); binding.StringFormat = _stringFormat; text.SetBinding(TextBlock.TextProperty, binding); _clrNodeContentTemplate.VisualTree = text; _clrNodeContentTemplate.Seal(); } return _clrNodeContentTemplate; } } private string _displayMemberPath; private string _stringFormat; private DataTemplate _xmlNodeContentTemplate; private DataTemplate _clrNodeContentTemplate; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Defines DisplayMemberTemplateSelector class. // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows; using System.Windows.Controls; using System.Windows.Data; using MS.Internal; namespace MS.Internal.Data { // Selects template appropriate for CLR/XML item in order to // display string property at DisplayMemberPath on the item. internal sealed class DisplayMemberTemplateSelector : DataTemplateSelector { ////// Constructor /// /// path to the member to display public DisplayMemberTemplateSelector(string displayMemberPath, string stringFormat) { Debug.Assert(!(String.IsNullOrEmpty(displayMemberPath) && String.IsNullOrEmpty(stringFormat))); _displayMemberPath = displayMemberPath; _stringFormat = stringFormat; } ////// Override this method to return an app specific /// The data content /// The container in which the content is to be displayed ///. /// a app specific template to apply. public override DataTemplate SelectTemplate(object item, DependencyObject container) { if (XmlHelper.IsXmlNode(item)) { if (_xmlNodeContentTemplate == null) { _xmlNodeContentTemplate = new DataTemplate(); FrameworkElementFactory text = ContentPresenter.CreateTextBlockFactory(); Binding binding = new Binding(); binding.XPath = _displayMemberPath; binding.StringFormat = _stringFormat; text.SetBinding(TextBlock.TextProperty, binding); _xmlNodeContentTemplate.VisualTree = text; _xmlNodeContentTemplate.Seal(); } return _xmlNodeContentTemplate; } else { if (_clrNodeContentTemplate == null) { _clrNodeContentTemplate = new DataTemplate(); FrameworkElementFactory text = ContentPresenter.CreateTextBlockFactory(); Binding binding = new Binding(); binding.Path = new PropertyPath(_displayMemberPath); binding.StringFormat = _stringFormat; text.SetBinding(TextBlock.TextProperty, binding); _clrNodeContentTemplate.VisualTree = text; _clrNodeContentTemplate.Seal(); } return _clrNodeContentTemplate; } } private string _displayMemberPath; private string _stringFormat; private DataTemplate _xmlNodeContentTemplate; private DataTemplate _clrNodeContentTemplate; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
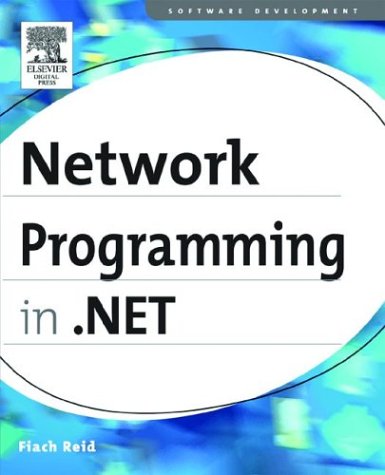
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridViewHitTestInfo.cs
- SchemaImporterExtensionsSection.cs
- HitTestParameters.cs
- GreenMethods.cs
- SafeRightsManagementEnvironmentHandle.cs
- DesignerWidgets.cs
- HtmlForm.cs
- CompiledELinqQueryState.cs
- RefExpr.cs
- WmlPanelAdapter.cs
- KnownBoxes.cs
- SmtpMail.cs
- Intellisense.cs
- RectValueSerializer.cs
- ElapsedEventArgs.cs
- StylusPointCollection.cs
- ListViewItemEventArgs.cs
- PageContent.cs
- AsyncPostBackErrorEventArgs.cs
- SecurityContextCookieSerializer.cs
- AxisAngleRotation3D.cs
- TableRow.cs
- AssertFilter.cs
- DataGridColumnHeaderItemAutomationPeer.cs
- TextClipboardData.cs
- ServiceModelActivity.cs
- AvTraceDetails.cs
- QueryStringParameter.cs
- NonClientArea.cs
- ObfuscateAssemblyAttribute.cs
- Tablet.cs
- AdornerDecorator.cs
- WebPartConnectionsCloseVerb.cs
- FilterableAttribute.cs
- WebPartsPersonalization.cs
- GPPOINT.cs
- printdlgexmarshaler.cs
- MasterPageBuildProvider.cs
- HttpCookiesSection.cs
- Label.cs
- UInt16.cs
- PropertyMetadata.cs
- BlockingCollection.cs
- SignatureResourceHelper.cs
- QilLoop.cs
- counter.cs
- TdsEnums.cs
- PersianCalendar.cs
- ReaderContextStackData.cs
- DataObjectFieldAttribute.cs
- StringUtil.cs
- SupportingTokenBindingElement.cs
- SubMenuStyleCollection.cs
- ContentType.cs
- Logging.cs
- StateDesigner.LayoutSelectionGlyph.cs
- ListViewItemCollectionEditor.cs
- AnnotationAdorner.cs
- ObjectListTitleAttribute.cs
- TreeNodeStyle.cs
- ConnectivityStatus.cs
- UnsafeNativeMethods.cs
- ProfessionalColors.cs
- UDPClient.cs
- WebReferenceCollection.cs
- HuffCodec.cs
- DataGridCommandEventArgs.cs
- IdentityNotMappedException.cs
- XPathPatternBuilder.cs
- While.cs
- AdvancedBindingEditor.cs
- UInt32Storage.cs
- OleDbTransaction.cs
- assertwrapper.cs
- StructuredType.cs
- ToolBarButtonClickEvent.cs
- CatalogPartChrome.cs
- CachedRequestParams.cs
- LockedActivityGlyph.cs
- LineBreakRecord.cs
- URLMembershipCondition.cs
- ReachFixedPageSerializerAsync.cs
- DateTimeFormatInfoScanner.cs
- ContentValidator.cs
- Converter.cs
- XmlAggregates.cs
- List.cs
- XamlTemplateSerializer.cs
- Vector3DAnimationBase.cs
- EntityClassGenerator.cs
- DependencySource.cs
- UInt64.cs
- PartManifestEntry.cs
- ViewgenGatekeeper.cs
- MultiBinding.cs
- DataSourceControl.cs
- SqlBuilder.cs
- recordstatefactory.cs
- RegisteredExpandoAttribute.cs
- ping.cs