Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media3D / AxisAngleRotation3D.cs / 1305600 / AxisAngleRotation3D.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // //--------------------------------------------------------------------------- using System; using MS.Internal; namespace System.Windows.Media.Media3D { ////// A rotation in 3-space defined by an axis and an angle to rotate about that axis. /// public partial class AxisAngleRotation3D { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Default constructor that creates a rotation with Axis (0,1,0) and Angle of 0. /// public AxisAngleRotation3D() {} ////// Constructor taking axis and angle. /// public AxisAngleRotation3D(Vector3D axis, double angle) { Axis = axis; Angle = angle; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ //----------------------------------------------------- // // Public Events // //------------------------------------------------------ //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties // Used by animation to get a snapshot of the current rotational // configuration for interpolation in Rotation3DAnimations. internal override Quaternion InternalQuaternion { get { if (_cachedQuaternionValue == c_dirtyQuaternion) { Vector3D axis = Axis; // Quaternion's axis/angle ctor throws if the axis has zero length. // // This threshold needs to match the one we used in D3DXVec3Normalize (d3dxmath9.cpp) // and in unmanaged code. See also AxisAngleRotation3D.cpp. if (axis.LengthSquared > DoubleUtil.FLT_MIN) { _cachedQuaternionValue = new Quaternion(axis, Angle); } else { // If we have a zero-length axis we return identity (i.e., // we consider this to be no rotation.) _cachedQuaternionValue = Quaternion.Identity; } } return _cachedQuaternionValue; } } #endregion Internal Properties internal void AxisPropertyChangedHook(DependencyPropertyChangedEventArgs e) { _cachedQuaternionValue = c_dirtyQuaternion; } internal void AnglePropertyChangedHook(DependencyPropertyChangedEventArgs e) { _cachedQuaternionValue = c_dirtyQuaternion; } //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ private Quaternion _cachedQuaternionValue = c_dirtyQuaternion; // Arbitrary quaternion that will signify that our cached quat is dirty // Reasonable quaternions are normalized so it's very unlikely that this // will ever occurr in a normal application. internal static readonly Quaternion c_dirtyQuaternion = new Quaternion( Math.E, Math.PI, Math.E * Math.PI, 55.0 ); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
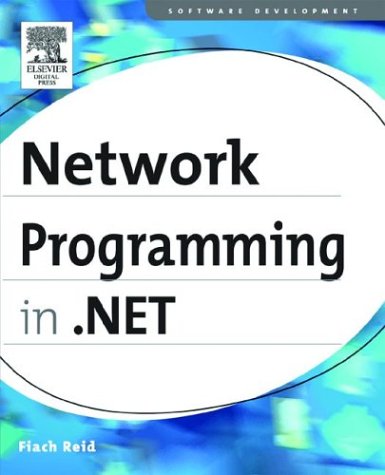
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridViewRowCollection.cs
- AnnotationAdorner.cs
- ExceptionUtil.cs
- RemotingServices.cs
- PropertyItem.cs
- PathTooLongException.cs
- ObjectIDGenerator.cs
- XslTransform.cs
- UntypedNullExpression.cs
- CustomTypeDescriptor.cs
- ParserOptions.cs
- OleDbMetaDataFactory.cs
- ClusterRegistryConfigurationProvider.cs
- PathFigureCollectionConverter.cs
- FileNotFoundException.cs
- PersonalizationProviderCollection.cs
- XmlUtil.cs
- AnnotationMap.cs
- JapaneseLunisolarCalendar.cs
- EntityDataSourceQueryBuilder.cs
- NativeMethodsOther.cs
- initElementDictionary.cs
- MimeBasePart.cs
- DeploymentSectionCache.cs
- UInt64Converter.cs
- ToolStripItemTextRenderEventArgs.cs
- TextParagraphView.cs
- Point4D.cs
- SchemaType.cs
- RemotingConfigParser.cs
- RegionData.cs
- EFTableProvider.cs
- DBConnectionString.cs
- HttpGetProtocolReflector.cs
- MediaPlayerState.cs
- Semaphore.cs
- QueryHandler.cs
- HostSecurityManager.cs
- MessageSmuggler.cs
- LocatorManager.cs
- CellConstantDomain.cs
- BuildProvider.cs
- ParagraphVisual.cs
- ListViewUpdatedEventArgs.cs
- FrameworkContentElement.cs
- EncoderBestFitFallback.cs
- PersonalizationProvider.cs
- ContextQuery.cs
- WmlCommandAdapter.cs
- SqlStatistics.cs
- SoundPlayerAction.cs
- EntryWrittenEventArgs.cs
- SqlWebEventProvider.cs
- TypeDelegator.cs
- ExpanderAutomationPeer.cs
- SqlRemoveConstantOrderBy.cs
- IpcClientChannel.cs
- TypeBuilder.cs
- InternalBufferOverflowException.cs
- SmtpFailedRecipientException.cs
- GcHandle.cs
- CellLabel.cs
- DataGridViewHitTestInfo.cs
- MetadataItem.cs
- StructuralCache.cs
- ContentElementCollection.cs
- EntityException.cs
- EmbeddedObject.cs
- PersonalizationStateQuery.cs
- TdsEnums.cs
- AutomationTextAttribute.cs
- EntityDataSourceDataSelection.cs
- Monitor.cs
- HitTestFilterBehavior.cs
- ScopedKnownTypes.cs
- DateTimeOffset.cs
- BitmapSource.cs
- PackageRelationship.cs
- DateTimeConverter2.cs
- LineInfo.cs
- LinqDataSourceEditData.cs
- ComplexTypeEmitter.cs
- TypeContext.cs
- EventProvider.cs
- StringCollectionMarkupSerializer.cs
- FunctionUpdateCommand.cs
- OutOfProcStateClientManager.cs
- AssemblyHelper.cs
- MetafileHeader.cs
- DBDataPermissionAttribute.cs
- WebBrowsableAttribute.cs
- CryptoConfig.cs
- LongValidator.cs
- _FixedSizeReader.cs
- TimeZoneInfo.cs
- XmlSchemaAnyAttribute.cs
- RootAction.cs
- TextElementEnumerator.cs
- WindowsRichEditRange.cs
- SchemaNotation.cs