Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataWeb / Server / System / Data / Services / CachedRequestParams.cs / 2 / CachedRequestParams.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Caches the value of all request headers // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services { #region Namespaces. using System; using System.Diagnostics; #endregion Namespaces. ////// Enum to represent various http methods /// internal enum AstoriaVerbs { #region Values. ///Not Initialized. None, ///Represents the GET http method. GET, ///Represents the PUT http method. PUT, ///Represents the POST http method. POST, ///Represents the DELETE http method. DELETE, ///Represents the MERGE http method. MERGE #endregion Values. } ////// Use this class to hold on to request parameters that may be inaccessible after a response writing begins. /// [DebuggerDisplay("{HttpMethod} {AbsoluteRequestUri}")] internal class CachedRequestParams { #region Fields. ///Gets the absolute resource upon which to apply the request. internal readonly Uri AbsoluteRequestUri; ///Gets the absolute URI to the service. internal readonly Uri AbsoluteServiceUri; ///Value for the Accept header. internal readonly string Accept; ///Value for the Accept-Charset header. internal readonly string AcceptCharset; ///Value of the Content-Type header. internal readonly string ContentType; ///HTTP method used for the request. internal readonly string HttpMethod; ///Value for the If-Match header. internal readonly string IfMatch; ///Value for the If-None-Match header. internal readonly string IfNoneMatch; ///Value for the MaxDataServiceVersion header. internal readonly string MaxVersion; ///Value for the DataServiceVersion header. internal readonly string Version; ///enum indicating http method used on the request, private AstoriaVerbs astoriaHttpVerb; #endregion Fields. #region Constructors. ///Initializes a new /// Value for the Accept header. /// Value for the Accept-Charset header. /// Value of the Content-Type header. /// HTTP method used for the request. /// Value for the If-Match header. /// Value for the If-None-Match header. /// Value for the DataServiceVersion header. /// Value for the MaxDataServiceVersion header. /// The absolute URI to the resource upon which to apply the request. /// URI to the service. internal CachedRequestParams( string accept, string acceptCharset, string contentType, string httpMethod, string headerIfMatch, string headerIfNoneMatch, string version, string maxVersion, Uri absoluteRequestUri, Uri serviceUri) { Debug.Assert(absoluteRequestUri != null, "absoluteRequestUri != null"); Debug.Assert(serviceUri != null, "serviceUri != null"); Debug.Assert(absoluteRequestUri.IsAbsoluteUri, "absoluteRequestUri.IsAbsoluteUri(" + absoluteRequestUri + ")"); Debug.Assert(serviceUri.IsAbsoluteUri, "serviceUri.IsAbsoluteUri(" + serviceUri + ")"); this.Accept = accept; this.AcceptCharset = acceptCharset; this.ContentType = contentType; this.HttpMethod = httpMethod; this.IfMatch = headerIfMatch; this.IfNoneMatch = headerIfNoneMatch; this.AbsoluteRequestUri = absoluteRequestUri; this.AbsoluteServiceUri = serviceUri; this.MaxVersion = maxVersion; this.Version = version; this.astoriaHttpVerb = AstoriaVerbs.None; } #endregion Constructors. #region Properties. ///instance. /// Get the enum representing the http method name. /// /// http method used for the request. ///enum representing the http method name. internal AstoriaVerbs AstoriaHttpVerb { get { if (this.astoriaHttpVerb == AstoriaVerbs.None) { if (XmlConstants.HttpMethodGet == this.HttpMethod) { this.astoriaHttpVerb = AstoriaVerbs.GET; } else if (XmlConstants.HttpMethodPost == this.HttpMethod) { this.astoriaHttpVerb = AstoriaVerbs.POST; } else if (XmlConstants.HttpMethodPut == this.HttpMethod) { this.astoriaHttpVerb = AstoriaVerbs.PUT; } else if (XmlConstants.HttpMethodMerge == this.HttpMethod) { this.astoriaHttpVerb = AstoriaVerbs.MERGE; } else if (XmlConstants.HttpMethodDelete == this.HttpMethod) { this.astoriaHttpVerb = AstoriaVerbs.DELETE; } else if (String.IsNullOrEmpty(this.HttpMethod)) { throw new InvalidOperationException(Strings.DataService_EmptyHttpMethod); } else { // 501: Not Implemented (rather than 405 - Method Not Allowed, // which implies it was understood and rejected). throw DataServiceException.CreateMethodNotImplemented(Strings.DataService_NotImplementedException); } } return this.astoriaHttpVerb; } } #endregion Properties. } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Caches the value of all request headers // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services { #region Namespaces. using System; using System.Diagnostics; #endregion Namespaces. ////// Enum to represent various http methods /// internal enum AstoriaVerbs { #region Values. ///Not Initialized. None, ///Represents the GET http method. GET, ///Represents the PUT http method. PUT, ///Represents the POST http method. POST, ///Represents the DELETE http method. DELETE, ///Represents the MERGE http method. MERGE #endregion Values. } ////// Use this class to hold on to request parameters that may be inaccessible after a response writing begins. /// [DebuggerDisplay("{HttpMethod} {AbsoluteRequestUri}")] internal class CachedRequestParams { #region Fields. ///Gets the absolute resource upon which to apply the request. internal readonly Uri AbsoluteRequestUri; ///Gets the absolute URI to the service. internal readonly Uri AbsoluteServiceUri; ///Value for the Accept header. internal readonly string Accept; ///Value for the Accept-Charset header. internal readonly string AcceptCharset; ///Value of the Content-Type header. internal readonly string ContentType; ///HTTP method used for the request. internal readonly string HttpMethod; ///Value for the If-Match header. internal readonly string IfMatch; ///Value for the If-None-Match header. internal readonly string IfNoneMatch; ///Value for the MaxDataServiceVersion header. internal readonly string MaxVersion; ///Value for the DataServiceVersion header. internal readonly string Version; ///enum indicating http method used on the request, private AstoriaVerbs astoriaHttpVerb; #endregion Fields. #region Constructors. ///Initializes a new /// Value for the Accept header. /// Value for the Accept-Charset header. /// Value of the Content-Type header. /// HTTP method used for the request. /// Value for the If-Match header. /// Value for the If-None-Match header. /// Value for the DataServiceVersion header. /// Value for the MaxDataServiceVersion header. /// The absolute URI to the resource upon which to apply the request. /// URI to the service. internal CachedRequestParams( string accept, string acceptCharset, string contentType, string httpMethod, string headerIfMatch, string headerIfNoneMatch, string version, string maxVersion, Uri absoluteRequestUri, Uri serviceUri) { Debug.Assert(absoluteRequestUri != null, "absoluteRequestUri != null"); Debug.Assert(serviceUri != null, "serviceUri != null"); Debug.Assert(absoluteRequestUri.IsAbsoluteUri, "absoluteRequestUri.IsAbsoluteUri(" + absoluteRequestUri + ")"); Debug.Assert(serviceUri.IsAbsoluteUri, "serviceUri.IsAbsoluteUri(" + serviceUri + ")"); this.Accept = accept; this.AcceptCharset = acceptCharset; this.ContentType = contentType; this.HttpMethod = httpMethod; this.IfMatch = headerIfMatch; this.IfNoneMatch = headerIfNoneMatch; this.AbsoluteRequestUri = absoluteRequestUri; this.AbsoluteServiceUri = serviceUri; this.MaxVersion = maxVersion; this.Version = version; this.astoriaHttpVerb = AstoriaVerbs.None; } #endregion Constructors. #region Properties. ///instance. /// Get the enum representing the http method name. /// /// http method used for the request. ///enum representing the http method name. internal AstoriaVerbs AstoriaHttpVerb { get { if (this.astoriaHttpVerb == AstoriaVerbs.None) { if (XmlConstants.HttpMethodGet == this.HttpMethod) { this.astoriaHttpVerb = AstoriaVerbs.GET; } else if (XmlConstants.HttpMethodPost == this.HttpMethod) { this.astoriaHttpVerb = AstoriaVerbs.POST; } else if (XmlConstants.HttpMethodPut == this.HttpMethod) { this.astoriaHttpVerb = AstoriaVerbs.PUT; } else if (XmlConstants.HttpMethodMerge == this.HttpMethod) { this.astoriaHttpVerb = AstoriaVerbs.MERGE; } else if (XmlConstants.HttpMethodDelete == this.HttpMethod) { this.astoriaHttpVerb = AstoriaVerbs.DELETE; } else if (String.IsNullOrEmpty(this.HttpMethod)) { throw new InvalidOperationException(Strings.DataService_EmptyHttpMethod); } else { // 501: Not Implemented (rather than 405 - Method Not Allowed, // which implies it was understood and rejected). throw DataServiceException.CreateMethodNotImplemented(Strings.DataService_NotImplementedException); } } return this.astoriaHttpVerb; } } #endregion Properties. } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
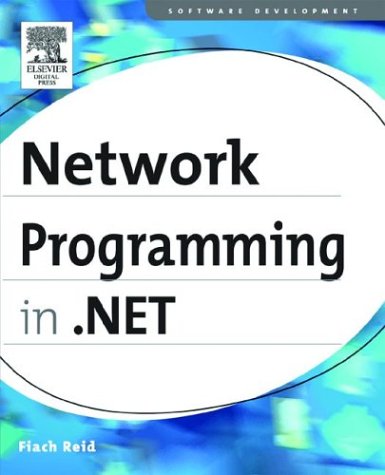
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ObjectRef.cs
- BaseParser.cs
- User.cs
- COAUTHIDENTITY.cs
- Switch.cs
- ValidationSummary.cs
- DecimalAnimationBase.cs
- EntityTypeEmitter.cs
- WebCodeGenerator.cs
- ISessionStateStore.cs
- CallbackWrapper.cs
- ShadowGlyph.cs
- LineUtil.cs
- _SafeNetHandles.cs
- ColumnBinding.cs
- PeerNeighborManager.cs
- RoutedPropertyChangedEventArgs.cs
- GroupQuery.cs
- MetricEntry.cs
- SpecularMaterial.cs
- Trigger.cs
- ADMembershipUser.cs
- SRGSCompiler.cs
- QilInvokeLateBound.cs
- DateRangeEvent.cs
- unitconverter.cs
- PrimitiveRenderer.cs
- XmlEntity.cs
- BinaryUtilClasses.cs
- RoleServiceManager.cs
- DiagnosticsConfigurationHandler.cs
- NonVisualControlAttribute.cs
- TableLayoutStyle.cs
- GridLengthConverter.cs
- StrokeSerializer.cs
- RoleManagerModule.cs
- EdmRelationshipRoleAttribute.cs
- GeneralTransform.cs
- BinarySecretKeyIdentifierClause.cs
- MemberJoinTreeNode.cs
- WizardStepBase.cs
- PropertyMappingExceptionEventArgs.cs
- MailSettingsSection.cs
- JsonReader.cs
- _Semaphore.cs
- RectangleF.cs
- WebPartPersonalization.cs
- FormatSettings.cs
- contentDescriptor.cs
- BindingListCollectionView.cs
- SqlDelegatedTransaction.cs
- Propagator.JoinPropagator.cs
- WindowVisualStateTracker.cs
- Point3DCollectionConverter.cs
- EntityTypeEmitter.cs
- XamlToRtfWriter.cs
- ProfileProvider.cs
- LicenseException.cs
- RuntimeCompatibilityAttribute.cs
- WebServiceHost.cs
- ThrowHelper.cs
- ObjectParameterCollection.cs
- SourceElementsCollection.cs
- RequestStatusBarUpdateEventArgs.cs
- ServerValidateEventArgs.cs
- ActivityDesigner.cs
- httpstaticobjectscollection.cs
- KnowledgeBase.cs
- DispatcherTimer.cs
- TextReader.cs
- NavigationProperty.cs
- VisualBasicExpressionConverter.cs
- RegexStringValidator.cs
- SafeNativeMethods.cs
- PtsCache.cs
- jithelpers.cs
- ReaderWriterLockWrapper.cs
- ProfileSettings.cs
- ListViewUpdateEventArgs.cs
- CompositeFontParser.cs
- ParallelDesigner.cs
- ContextBase.cs
- PageRequestManager.cs
- EFDataModelProvider.cs
- Process.cs
- RowVisual.cs
- RSAPKCS1KeyExchangeFormatter.cs
- PropertyDescriptor.cs
- ViewGenResults.cs
- MultiPropertyDescriptorGridEntry.cs
- PropertyDescriptorComparer.cs
- NativeMethods.cs
- SrgsOneOf.cs
- TreeNode.cs
- Thickness.cs
- GlyphingCache.cs
- MenuItemAutomationPeer.cs
- TypeElement.cs
- ScriptResourceAttribute.cs
- ValidationSettings.cs