Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / xsp / System / Web / UI / DataSourceControl.cs / 1 / DataSourceControl.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System.Collections; using System.ComponentModel; using System.Security.Permissions; ////// A DataSourceControl represents a data source that can be used to /// data-bind a DataBoundControl. /// DataSourceControl is an abstract base class that defines the /// interface between a DataBoundControl and its data source. /// The design of DataSourceControl enables creation of a variety of /// data controls with different underlying data sources such /// as SqlDataControl, WebServiceDataControl, XmlDataControl etc. /// The data source is implemented as a control even though it /// has no visual rendering, to allow it to be persisted /// declaratively, and to allow it to participate in state /// management should it choose to. /// In abstract terms a DataSourceControl has an underlying data source. /// This data source may contain one or more lists of data within it. /// Each list is associated with a name and at the bare minimum /// supports enumeration via the IEnumerable interface. A DataBoundControl /// is typically bound to a single list within the DataControl. /// [ Bindable(false), ControlBuilder(typeof(DataSourceControlBuilder)), Designer("System.Web.UI.Design.DataSourceDesigner, " + AssemblyRef.SystemDesign), NonVisualControl() ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public abstract class DataSourceControl : Control, IDataSource, IListSource { private static readonly object EventDataSourceChanged = new object(); private static readonly object EventDataSourceChangedInternal = new object(); [ EditorBrowsable(EditorBrowsableState.Never), ] public override string ClientID { get { return base.ClientID; } } [ EditorBrowsable(EditorBrowsableState.Never), ] public override ControlCollection Controls { get { return base.Controls; } } [ Browsable(false), DefaultValue(false), EditorBrowsable(EditorBrowsableState.Never), ] public override bool EnableTheming { get { return false; } set { throw new NotSupportedException(SR.GetString(SR.NoThemingSupport, this.GetType().Name)); } } [ Browsable(false), DefaultValue(""), EditorBrowsable(EditorBrowsableState.Never), ] public override string SkinID { get { return String.Empty; } set { throw new NotSupportedException(SR.GetString(SR.NoThemingSupport, this.GetType().Name)); } } ////// Gets or sets a value that indicates whether a control should be rendered on /// the page. /// [ Browsable(false), DefaultValue(false), EditorBrowsable(EditorBrowsableState.Never), ] public override bool Visible { get { return false; } set { throw new NotSupportedException(SR.GetString(SR.ControlNonVisual, this.GetType().Name)); } } ////// Raised internally by a DataSource when data-related state is /// changed. DataSourceViews attach to this event so that they can /// be notified when the parent data source changes. /// This event is separate from DataSourceChanged because the DataSourceView /// attaches to it and fires its DataSourceViewChanged event. We want that /// to fire before the DataSourceChanged event. /// internal event EventHandler DataSourceChangedInternal { add { Events.AddHandler(EventDataSourceChangedInternal, value); } remove { Events.RemoveHandler(EventDataSourceChangedInternal, value); } } [ EditorBrowsable(EditorBrowsableState.Never), ] public override void ApplyStyleSheetSkin(Page page) { base.ApplyStyleSheetSkin(page); } ////// Overidden to prevent child controls from being added to this control. /// protected override ControlCollection CreateControlCollection() { return new EmptyControlCollection(this); } [ EditorBrowsable(EditorBrowsableState.Never), ] public override Control FindControl(string id) { return base.FindControl(id); } ////// [ EditorBrowsable(EditorBrowsableState.Never), ] public override void Focus() { throw new NotSupportedException(SR.GetString(SR.NoFocusSupport, this.GetType().Name)); } protected abstract DataSourceView GetView(string viewName); protected virtual ICollection GetViewNames() { return null; } [ EditorBrowsable(EditorBrowsableState.Never), ] public override bool HasControls() { return base.HasControls(); } private void OnDataSourceChanged(EventArgs e) { EventHandler handler = (EventHandler)Events[EventDataSourceChanged]; if (handler != null) { handler(this, e); } } private void OnDataSourceChangedInternal(EventArgs e) { EventHandler handler = (EventHandler)Events[EventDataSourceChangedInternal]; if (handler != null) { handler(this, e); } } protected virtual void RaiseDataSourceChangedEvent(EventArgs e) { OnDataSourceChangedInternal(e); OnDataSourceChanged(e); } [ EditorBrowsable(EditorBrowsableState.Never), ] public override void RenderControl(HtmlTextWriter writer) { base.RenderControl(writer); } #region Implementation of IDataSource ////// Raised when the underlying data source has changed. The /// change may be due to a change in the control's properties, /// or a change in the data due to an edit action performed by /// the DataSourceControl. /// event EventHandler IDataSource.DataSourceChanged { add { Events.AddHandler(EventDataSourceChanged, value); } remove { Events.RemoveHandler(EventDataSourceChanged, value); } } ///DataSourceView IDataSource.GetView(string viewName) { return GetView(viewName); } /// ICollection IDataSource.GetViewNames() { return GetViewNames(); } #endregion #region Implementation of IListSource /// bool IListSource.ContainsListCollection { get { if (DesignMode) { return false; } return ListSourceHelper.ContainsListCollection(this); } } /// IList IListSource.GetList() { if (DesignMode) { return null; } return ListSourceHelper.GetList(this); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System.Collections; using System.ComponentModel; using System.Security.Permissions; ////// A DataSourceControl represents a data source that can be used to /// data-bind a DataBoundControl. /// DataSourceControl is an abstract base class that defines the /// interface between a DataBoundControl and its data source. /// The design of DataSourceControl enables creation of a variety of /// data controls with different underlying data sources such /// as SqlDataControl, WebServiceDataControl, XmlDataControl etc. /// The data source is implemented as a control even though it /// has no visual rendering, to allow it to be persisted /// declaratively, and to allow it to participate in state /// management should it choose to. /// In abstract terms a DataSourceControl has an underlying data source. /// This data source may contain one or more lists of data within it. /// Each list is associated with a name and at the bare minimum /// supports enumeration via the IEnumerable interface. A DataBoundControl /// is typically bound to a single list within the DataControl. /// [ Bindable(false), ControlBuilder(typeof(DataSourceControlBuilder)), Designer("System.Web.UI.Design.DataSourceDesigner, " + AssemblyRef.SystemDesign), NonVisualControl() ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public abstract class DataSourceControl : Control, IDataSource, IListSource { private static readonly object EventDataSourceChanged = new object(); private static readonly object EventDataSourceChangedInternal = new object(); [ EditorBrowsable(EditorBrowsableState.Never), ] public override string ClientID { get { return base.ClientID; } } [ EditorBrowsable(EditorBrowsableState.Never), ] public override ControlCollection Controls { get { return base.Controls; } } [ Browsable(false), DefaultValue(false), EditorBrowsable(EditorBrowsableState.Never), ] public override bool EnableTheming { get { return false; } set { throw new NotSupportedException(SR.GetString(SR.NoThemingSupport, this.GetType().Name)); } } [ Browsable(false), DefaultValue(""), EditorBrowsable(EditorBrowsableState.Never), ] public override string SkinID { get { return String.Empty; } set { throw new NotSupportedException(SR.GetString(SR.NoThemingSupport, this.GetType().Name)); } } ////// Gets or sets a value that indicates whether a control should be rendered on /// the page. /// [ Browsable(false), DefaultValue(false), EditorBrowsable(EditorBrowsableState.Never), ] public override bool Visible { get { return false; } set { throw new NotSupportedException(SR.GetString(SR.ControlNonVisual, this.GetType().Name)); } } ////// Raised internally by a DataSource when data-related state is /// changed. DataSourceViews attach to this event so that they can /// be notified when the parent data source changes. /// This event is separate from DataSourceChanged because the DataSourceView /// attaches to it and fires its DataSourceViewChanged event. We want that /// to fire before the DataSourceChanged event. /// internal event EventHandler DataSourceChangedInternal { add { Events.AddHandler(EventDataSourceChangedInternal, value); } remove { Events.RemoveHandler(EventDataSourceChangedInternal, value); } } [ EditorBrowsable(EditorBrowsableState.Never), ] public override void ApplyStyleSheetSkin(Page page) { base.ApplyStyleSheetSkin(page); } ////// Overidden to prevent child controls from being added to this control. /// protected override ControlCollection CreateControlCollection() { return new EmptyControlCollection(this); } [ EditorBrowsable(EditorBrowsableState.Never), ] public override Control FindControl(string id) { return base.FindControl(id); } ////// [ EditorBrowsable(EditorBrowsableState.Never), ] public override void Focus() { throw new NotSupportedException(SR.GetString(SR.NoFocusSupport, this.GetType().Name)); } protected abstract DataSourceView GetView(string viewName); protected virtual ICollection GetViewNames() { return null; } [ EditorBrowsable(EditorBrowsableState.Never), ] public override bool HasControls() { return base.HasControls(); } private void OnDataSourceChanged(EventArgs e) { EventHandler handler = (EventHandler)Events[EventDataSourceChanged]; if (handler != null) { handler(this, e); } } private void OnDataSourceChangedInternal(EventArgs e) { EventHandler handler = (EventHandler)Events[EventDataSourceChangedInternal]; if (handler != null) { handler(this, e); } } protected virtual void RaiseDataSourceChangedEvent(EventArgs e) { OnDataSourceChangedInternal(e); OnDataSourceChanged(e); } [ EditorBrowsable(EditorBrowsableState.Never), ] public override void RenderControl(HtmlTextWriter writer) { base.RenderControl(writer); } #region Implementation of IDataSource ////// Raised when the underlying data source has changed. The /// change may be due to a change in the control's properties, /// or a change in the data due to an edit action performed by /// the DataSourceControl. /// event EventHandler IDataSource.DataSourceChanged { add { Events.AddHandler(EventDataSourceChanged, value); } remove { Events.RemoveHandler(EventDataSourceChanged, value); } } ///DataSourceView IDataSource.GetView(string viewName) { return GetView(viewName); } /// ICollection IDataSource.GetViewNames() { return GetViewNames(); } #endregion #region Implementation of IListSource /// bool IListSource.ContainsListCollection { get { if (DesignMode) { return false; } return ListSourceHelper.ContainsListCollection(this); } } /// IList IListSource.GetList() { if (DesignMode) { return null; } return ListSourceHelper.GetList(this); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
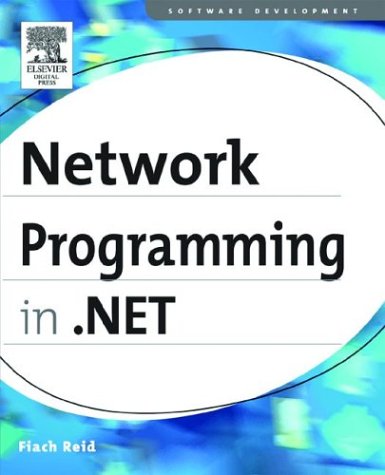
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HitTestWithGeometryDrawingContextWalker.cs
- SystemWebSectionGroup.cs
- WebServiceParameterData.cs
- SpeechDetectedEventArgs.cs
- WindowsFormsHost.cs
- WebUtility.cs
- ObjectSecurity.cs
- PropertyOverridesDialog.cs
- TabControlToolboxItem.cs
- UnSafeCharBuffer.cs
- TypeTypeConverter.cs
- SerializationFieldInfo.cs
- CodeValidator.cs
- PointAnimationClockResource.cs
- DataColumnMappingCollection.cs
- CryptoHandle.cs
- WeakHashtable.cs
- DesignerWidgets.cs
- Track.cs
- IxmlLineInfo.cs
- RuntimeResourceSet.cs
- DataGridTableCollection.cs
- TransactionTable.cs
- MissingManifestResourceException.cs
- SelectingProviderEventArgs.cs
- AttributeCollection.cs
- Int64Storage.cs
- PopOutPanel.cs
- ZoneMembershipCondition.cs
- CodeIterationStatement.cs
- SafeMILHandle.cs
- ScrollableControlDesigner.cs
- DataBinding.cs
- Bitmap.cs
- __Filters.cs
- BoundsDrawingContextWalker.cs
- EnvelopedPkcs7.cs
- SecurityChannelFaultConverter.cs
- StylusPointDescription.cs
- SerializationInfoEnumerator.cs
- WorkflowViewElement.cs
- ZipIOCentralDirectoryDigitalSignature.cs
- DataGridRowDetailsEventArgs.cs
- TimeSpanFormat.cs
- SqlRowUpdatedEvent.cs
- AbstractExpressions.cs
- RequestNavigateEventArgs.cs
- EventLogEntryCollection.cs
- BoundPropertyEntry.cs
- ControlCachePolicy.cs
- ReachDocumentSequenceSerializerAsync.cs
- EndPoint.cs
- PropertyCollection.cs
- VSWCFServiceContractGenerator.cs
- CharConverter.cs
- SrgsElementList.cs
- StringReader.cs
- SmiEventStream.cs
- TextElementEnumerator.cs
- OutputScopeManager.cs
- SizeAnimation.cs
- SendingRequestEventArgs.cs
- RotateTransform3D.cs
- BigInt.cs
- EmptyCollection.cs
- TextRangeEdit.cs
- CustomErrorsSection.cs
- ToolStripItemClickedEventArgs.cs
- OleDbError.cs
- TextSimpleMarkerProperties.cs
- MDIControlStrip.cs
- ByValueEqualityComparer.cs
- SerializationEventsCache.cs
- HttpHandlerAction.cs
- InstanceKeyView.cs
- ActivityExecutor.cs
- ShaderEffect.cs
- Thread.cs
- WebConfigurationManager.cs
- PeerChannelFactory.cs
- ValueSerializerAttribute.cs
- Membership.cs
- SoapHeaders.cs
- ResourcePool.cs
- RectangleConverter.cs
- RegexGroupCollection.cs
- ZipIOLocalFileHeader.cs
- XsdValidatingReader.cs
- RoleGroupCollection.cs
- BindableTemplateBuilder.cs
- Configuration.cs
- CustomPopupPlacement.cs
- SqlConnectionStringBuilder.cs
- DocumentViewerAutomationPeer.cs
- RawStylusInput.cs
- DynamicDiscoSearcher.cs
- SessionParameter.cs
- RawStylusInput.cs
- GenerateTemporaryTargetAssembly.cs
- ViewManager.cs