Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Base / System / Windows / Threading / DispatcherExceptionFilterEventArgs.cs / 1 / DispatcherExceptionFilterEventArgs.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Arguments for the ExceptionFilter event. The event is raised // when a dispatcher exception has occured. This event is raised // before the callstack is unwound. // // History: // 07/22/2003 : KenLai - Created // //--------------------------------------------------------------------------- using System.Diagnostics; using System; namespace System.Windows.Threading { ////// Arguments for the ExceptionFilter event. The event is raised when /// a dispatcher exception has occured. /// ////// This event is raised before the callstack is unwound. /// public sealed class DispatcherUnhandledExceptionFilterEventArgs : DispatcherEventArgs { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- // Initialize a new event argument. internal DispatcherUnhandledExceptionFilterEventArgs(Dispatcher dispatcher) : base(dispatcher) { } //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- ////// The exception that was raised on a thread operating within /// the dispatcher. /// public Exception Exception { get { return _exception; } } ////// Whether or not the exception should be caught and the exception /// event handlers called. /// ////// A filter handler can set this property to false to request that /// the exception not be caught, to avoid the callstack getting /// unwound up to the Dispatcher. /// /// A previous handler in the event multicast might have already set this /// property to false, signalling that the exception will not be caught. /// We let the "don't catch" behavior override all others because /// it most likely means a debugging scenario. /// public bool RequestCatch { get { return _requestCatch; } set { // Only allow to be set false. if (value == false) { _requestCatch = value; } } } //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ ////// Initialize the preallocated args class for use. /// ////// This method MUST NOT FAIL because it is called from an exception /// handler: do not do any heavy lifting or allocate more memory. /// This initialization step is separated from the constructor /// precisely because we wanted to preallocate the memory and avoid /// hitting a secondary exception in the out-of-memory case. /// /// /// The exception that was raised while executing code via the /// dispatcher. /// /// /// Whether or not the exception should be caught and the /// exception handlers called. /// internal void Initialize(Exception exception, bool requestCatch) { Debug.Assert(exception != null); _exception = exception; _requestCatch = requestCatch; } //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ private Exception _exception; private bool _requestCatch; } ////// Delegate for the events that convey the state of the UiConext /// in response to various actions that involve items. /// public delegate void DispatcherUnhandledExceptionFilterEventHandler(object sender, DispatcherUnhandledExceptionFilterEventArgs e); } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Arguments for the ExceptionFilter event. The event is raised // when a dispatcher exception has occured. This event is raised // before the callstack is unwound. // // History: // 07/22/2003 : KenLai - Created // //--------------------------------------------------------------------------- using System.Diagnostics; using System; namespace System.Windows.Threading { ////// Arguments for the ExceptionFilter event. The event is raised when /// a dispatcher exception has occured. /// ////// This event is raised before the callstack is unwound. /// public sealed class DispatcherUnhandledExceptionFilterEventArgs : DispatcherEventArgs { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- // Initialize a new event argument. internal DispatcherUnhandledExceptionFilterEventArgs(Dispatcher dispatcher) : base(dispatcher) { } //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- ////// The exception that was raised on a thread operating within /// the dispatcher. /// public Exception Exception { get { return _exception; } } ////// Whether or not the exception should be caught and the exception /// event handlers called. /// ////// A filter handler can set this property to false to request that /// the exception not be caught, to avoid the callstack getting /// unwound up to the Dispatcher. /// /// A previous handler in the event multicast might have already set this /// property to false, signalling that the exception will not be caught. /// We let the "don't catch" behavior override all others because /// it most likely means a debugging scenario. /// public bool RequestCatch { get { return _requestCatch; } set { // Only allow to be set false. if (value == false) { _requestCatch = value; } } } //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ ////// Initialize the preallocated args class for use. /// ////// This method MUST NOT FAIL because it is called from an exception /// handler: do not do any heavy lifting or allocate more memory. /// This initialization step is separated from the constructor /// precisely because we wanted to preallocate the memory and avoid /// hitting a secondary exception in the out-of-memory case. /// /// /// The exception that was raised while executing code via the /// dispatcher. /// /// /// Whether or not the exception should be caught and the /// exception handlers called. /// internal void Initialize(Exception exception, bool requestCatch) { Debug.Assert(exception != null); _exception = exception; _requestCatch = requestCatch; } //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ private Exception _exception; private bool _requestCatch; } ////// Delegate for the events that convey the state of the UiConext /// in response to various actions that involve items. /// public delegate void DispatcherUnhandledExceptionFilterEventHandler(object sender, DispatcherUnhandledExceptionFilterEventArgs e); } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
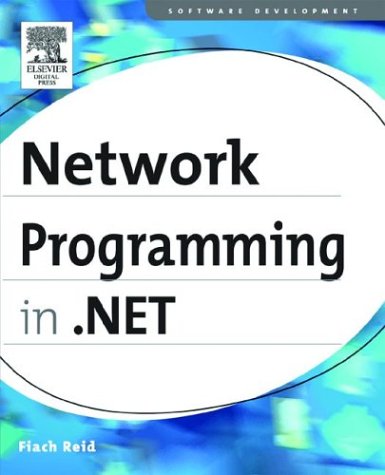
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MgmtConfigurationRecord.cs
- XmlElementAttributes.cs
- Blend.cs
- UdpAnnouncementEndpoint.cs
- MessageSmuggler.cs
- XPathParser.cs
- ICspAsymmetricAlgorithm.cs
- SpellerHighlightLayer.cs
- ReliabilityContractAttribute.cs
- ToolStripRenderEventArgs.cs
- VideoDrawing.cs
- MultipartContentParser.cs
- WindowsAuthenticationModule.cs
- BitmapCodecInfo.cs
- SmiSettersStream.cs
- Keywords.cs
- ApplicationId.cs
- LowerCaseStringConverter.cs
- RecordsAffectedEventArgs.cs
- DataList.cs
- GridLengthConverter.cs
- SolidColorBrush.cs
- SessionEndingEventArgs.cs
- Vector3dCollection.cs
- NestedContainer.cs
- ReadOnlyDataSource.cs
- UriParserTemplates.cs
- MediaContextNotificationWindow.cs
- StateWorkerRequest.cs
- DesignerHierarchicalDataSourceView.cs
- FontStretchConverter.cs
- ToolStripRenderEventArgs.cs
- ComPlusServiceLoader.cs
- HyperLinkStyle.cs
- ConfigXmlSignificantWhitespace.cs
- X509CertificateValidator.cs
- HttpCookiesSection.cs
- InvalidFilterCriteriaException.cs
- QilUnary.cs
- ConstantProjectedSlot.cs
- ChangeBlockUndoRecord.cs
- ReferenceEqualityComparer.cs
- WorkflowMarkupSerializationProvider.cs
- StylusPoint.cs
- DataGridViewButtonCell.cs
- If.cs
- SendContent.cs
- SelectionEditingBehavior.cs
- PropertyValueUIItem.cs
- PropertyGeneratedEventArgs.cs
- StreamSecurityUpgradeInitiator.cs
- COM2PictureConverter.cs
- CodeValidator.cs
- PtsContext.cs
- PolygonHotSpot.cs
- NoneExcludedImageIndexConverter.cs
- UnmanagedBitmapWrapper.cs
- DataRowCollection.cs
- DictionaryCustomTypeDescriptor.cs
- DataViewManagerListItemTypeDescriptor.cs
- HttpModulesSection.cs
- AttachedPropertiesService.cs
- TypeElement.cs
- ThreadLocal.cs
- XmlSchemaDocumentation.cs
- securitycriticaldataformultiplegetandset.cs
- HttpApplicationFactory.cs
- WebPartsPersonalization.cs
- ScaleTransform3D.cs
- CustomCategoryAttribute.cs
- PointLightBase.cs
- WebConfigurationHost.cs
- HandlerBase.cs
- TrustManagerPromptUI.cs
- DataBindingCollectionEditor.cs
- WorkflowMarkupSerializationManager.cs
- X509Extension.cs
- Events.cs
- DynamicMethod.cs
- CssStyleCollection.cs
- GPRECTF.cs
- WebPartsPersonalization.cs
- ParameterBinding.cs
- MultiView.cs
- ToolboxComponentsCreatingEventArgs.cs
- AnimatedTypeHelpers.cs
- CodeIdentifiers.cs
- LinearQuaternionKeyFrame.cs
- XamlFilter.cs
- ProcessHostServerConfig.cs
- TextOptions.cs
- SessionPageStateSection.cs
- FilterQueryOptionExpression.cs
- DispatcherSynchronizationContext.cs
- CodeArrayCreateExpression.cs
- ConfigurationSectionCollection.cs
- BreadCrumbTextConverter.cs
- CompilationSection.cs
- CodeStatementCollection.cs
- RepeatInfo.cs