Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Common / internal / materialization / recordstatescratchpad.cs / 1 / recordstatescratchpad.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System.Collections.Generic; using System.Data.Metadata.Edm; using System.Linq; using System.Linq.Expressions; namespace System.Data.Common.Internal.Materialization { ////// Used in the Translator to aggregate information about a (nested) record /// state. After the translator visits the columnMaps, it will compile /// the recordState(s) which produces an immutable RecordStateFactory that /// can be shared amongst many query instances. /// internal class RecordStateScratchpad { private int _stateSlotNumber; internal int StateSlotNumber { get { return _stateSlotNumber; } set { _stateSlotNumber = value; } } private int _columnCount; internal int ColumnCount { get { return _columnCount; } set { _columnCount = value; } } private DataRecordInfo _dataRecordInfo; internal DataRecordInfo DataRecordInfo { get { return _dataRecordInfo; } set { _dataRecordInfo = value; } } private Expression _gatherData; internal Expression GatherData { get { return _gatherData; } set { _gatherData = value; } } private string[] _propertyNames; internal string[] PropertyNames { get { return _propertyNames; } set { _propertyNames = value; } } private TypeUsage[] _typeUsages; internal TypeUsage[] TypeUsages { get { return _typeUsages; } set { _typeUsages = value; } } private List_nestedRecordStateScratchpads = new List (); internal RecordStateFactory Compile() { RecordStateFactory[] nestedRecordStateFactories = new RecordStateFactory[_nestedRecordStateScratchpads.Count]; for (int i = 0; i < nestedRecordStateFactories.Length; i++) { nestedRecordStateFactories[i] = _nestedRecordStateScratchpads[i].Compile(); } RecordStateFactory result = (RecordStateFactory)Activator.CreateInstance(typeof(RecordStateFactory), new object[] { this.StateSlotNumber, this.ColumnCount, nestedRecordStateFactories, this.DataRecordInfo, this.GatherData, this.PropertyNames, this.TypeUsages }); return result; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System.Collections.Generic; using System.Data.Metadata.Edm; using System.Linq; using System.Linq.Expressions; namespace System.Data.Common.Internal.Materialization { ////// Used in the Translator to aggregate information about a (nested) record /// state. After the translator visits the columnMaps, it will compile /// the recordState(s) which produces an immutable RecordStateFactory that /// can be shared amongst many query instances. /// internal class RecordStateScratchpad { private int _stateSlotNumber; internal int StateSlotNumber { get { return _stateSlotNumber; } set { _stateSlotNumber = value; } } private int _columnCount; internal int ColumnCount { get { return _columnCount; } set { _columnCount = value; } } private DataRecordInfo _dataRecordInfo; internal DataRecordInfo DataRecordInfo { get { return _dataRecordInfo; } set { _dataRecordInfo = value; } } private Expression _gatherData; internal Expression GatherData { get { return _gatherData; } set { _gatherData = value; } } private string[] _propertyNames; internal string[] PropertyNames { get { return _propertyNames; } set { _propertyNames = value; } } private TypeUsage[] _typeUsages; internal TypeUsage[] TypeUsages { get { return _typeUsages; } set { _typeUsages = value; } } private List_nestedRecordStateScratchpads = new List (); internal RecordStateFactory Compile() { RecordStateFactory[] nestedRecordStateFactories = new RecordStateFactory[_nestedRecordStateScratchpads.Count]; for (int i = 0; i < nestedRecordStateFactories.Length; i++) { nestedRecordStateFactories[i] = _nestedRecordStateScratchpads[i].Compile(); } RecordStateFactory result = (RecordStateFactory)Activator.CreateInstance(typeof(RecordStateFactory), new object[] { this.StateSlotNumber, this.ColumnCount, nestedRecordStateFactories, this.DataRecordInfo, this.GatherData, this.PropertyNames, this.TypeUsages }); return result; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
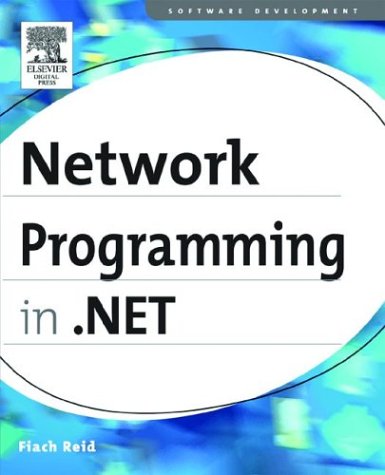
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ClientApiGenerator.cs
- BitmapPalettes.cs
- MembershipSection.cs
- odbcmetadatacolumnnames.cs
- ReaderWriterLockWrapper.cs
- MaskPropertyEditor.cs
- SQLMembershipProvider.cs
- PkcsMisc.cs
- AsyncPostBackTrigger.cs
- DataGridViewDataConnection.cs
- SelectionPattern.cs
- AuthenticationConfig.cs
- HybridDictionary.cs
- Utility.cs
- AmbientLight.cs
- ArgIterator.cs
- SyndicationSerializer.cs
- DeadCharTextComposition.cs
- RoleServiceManager.cs
- ResourceBinder.cs
- UrlMappingsSection.cs
- HMACMD5.cs
- InvalidEnumArgumentException.cs
- MetadataItemEmitter.cs
- ObjectDataSourceStatusEventArgs.cs
- ResolveCriteria11.cs
- HelpInfo.cs
- DataSourceHelper.cs
- ImageSourceValueSerializer.cs
- WebPartDescription.cs
- XmlWrappingWriter.cs
- SqlDataSourceQuery.cs
- DbParameterHelper.cs
- TimeSpanSecondsOrInfiniteConverter.cs
- HistoryEventArgs.cs
- TextRange.cs
- DictionarySectionHandler.cs
- ProvideValueServiceProvider.cs
- MessageEnumerator.cs
- SimpleApplicationHost.cs
- Formatter.cs
- WmpBitmapEncoder.cs
- DataGridViewTextBoxCell.cs
- TextServicesManager.cs
- RotateTransform3D.cs
- FunctionDetailsReader.cs
- InvalidEnumArgumentException.cs
- ListBox.cs
- ThreadWorkerController.cs
- DataSourceViewSchemaConverter.cs
- Section.cs
- TagNameToTypeMapper.cs
- TextServicesProperty.cs
- ApplicationException.cs
- ReflectTypeDescriptionProvider.cs
- DefaultPrintController.cs
- CollectionBuilder.cs
- CommentEmitter.cs
- sqlcontext.cs
- UIElementHelper.cs
- BindingOperations.cs
- PerspectiveCamera.cs
- WebPartConnectVerb.cs
- XmlQuerySequence.cs
- FormsAuthenticationCredentials.cs
- RegexGroup.cs
- AuthenticationConfig.cs
- DataBindingExpressionBuilder.cs
- AbstractSvcMapFileLoader.cs
- KeyEventArgs.cs
- GAC.cs
- LineSegment.cs
- ErrorWebPart.cs
- FontFamilyConverter.cs
- AlternateViewCollection.cs
- DecoderNLS.cs
- TraceLevelStore.cs
- RepeatBehavior.cs
- UrlRoutingModule.cs
- Header.cs
- UniqueIdentifierService.cs
- Misc.cs
- Bitmap.cs
- ItemChangedEventArgs.cs
- ACE.cs
- TemplateLookupAction.cs
- InfoCardCryptoHelper.cs
- Frame.cs
- OpacityConverter.cs
- StrongNameMembershipCondition.cs
- Geometry3D.cs
- SecurityContext.cs
- HtmlShimManager.cs
- RuleSettings.cs
- OLEDB_Util.cs
- InputMethodStateTypeInfo.cs
- StringConcat.cs
- AssemblyResourceLoader.cs
- ContainerFilterService.cs
- DBCSCodePageEncoding.cs