Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Net / System / Net / Mail / AttachmentCollection.cs / 1 / AttachmentCollection.cs
using System; using System.Collections.ObjectModel; namespace System.Net.Mail { ////// Summary description for AttachmentCollection. /// public sealed class AttachmentCollection : Collection, IDisposable { bool disposed = false; internal AttachmentCollection() { } public void Dispose(){ if(disposed){ return; } foreach (Attachment attachment in this) { attachment.Dispose(); } Clear(); disposed = true; } protected override void RemoveItem(int index){ if (disposed) { throw new ObjectDisposedException(this.GetType().FullName); } base.RemoveItem(index); } protected override void ClearItems(){ if (disposed) { throw new ObjectDisposedException(this.GetType().FullName); } base.ClearItems(); } protected override void SetItem(int index, Attachment item){ if (disposed) { throw new ObjectDisposedException(this.GetType().FullName); } if(item==null) { throw new ArgumentNullException("item"); } base.SetItem(index,item); } protected override void InsertItem(int index, Attachment item){ if (disposed) { throw new ObjectDisposedException(this.GetType().FullName); } if(item==null){ throw new ArgumentNullException("item"); } base.InsertItem(index,item); } } }
Link Menu
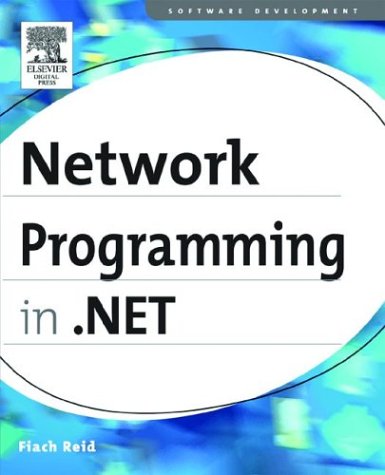
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GroupJoinQueryOperator.cs
- DefaultValidator.cs
- DataGridCommandEventArgs.cs
- ModifiableIteratorCollection.cs
- DataFieldCollectionEditor.cs
- ApplicationManager.cs
- DataSpaceManager.cs
- BitHelper.cs
- PhoneCallDesigner.cs
- GridEntryCollection.cs
- PinnedBufferMemoryStream.cs
- UrlPropertyAttribute.cs
- QuadraticBezierSegment.cs
- XPathQueryGenerator.cs
- PerformanceCounterPermissionEntryCollection.cs
- DbProviderFactory.cs
- UrlPath.cs
- PrefixQName.cs
- Stopwatch.cs
- ISCIIEncoding.cs
- AnonymousIdentificationSection.cs
- FontConverter.cs
- PrimarySelectionAdorner.cs
- CommandTreeTypeHelper.cs
- ToolboxDataAttribute.cs
- AlignmentXValidation.cs
- ActionItem.cs
- FontEmbeddingManager.cs
- VariantWrapper.cs
- SiteMembershipCondition.cs
- KeyedHashAlgorithm.cs
- COM2Enum.cs
- AuthenticationManager.cs
- SrgsElementList.cs
- BindingBase.cs
- SchemaTableOptionalColumn.cs
- HTTPNotFoundHandler.cs
- NameTable.cs
- CommandHelpers.cs
- CodeConditionStatement.cs
- StringAnimationUsingKeyFrames.cs
- BindingListCollectionView.cs
- PipeConnection.cs
- SqlGatherConsumedAliases.cs
- XmlToDatasetMap.cs
- TextTreeText.cs
- FunctionImportElement.cs
- MultitargetUtil.cs
- InkCanvasSelectionAdorner.cs
- propertyentry.cs
- ResXResourceWriter.cs
- CodeDOMProvider.cs
- FilterElement.cs
- Hex.cs
- TypeToken.cs
- CustomAttributeBuilder.cs
- DictionaryManager.cs
- HtmlControlPersistable.cs
- DocumentViewerConstants.cs
- DeclarativeExpressionConditionDeclaration.cs
- OleDbTransaction.cs
- InputScopeNameConverter.cs
- JavaScriptString.cs
- MemoryPressure.cs
- MobileControlsSection.cs
- GeneratedContractType.cs
- TrustLevelCollection.cs
- DataServiceQueryOfT.cs
- SystemFonts.cs
- PartManifestEntry.cs
- filewebrequest.cs
- AdornedElementPlaceholder.cs
- ThreadStartException.cs
- ZipIOExtraFieldElement.cs
- WebSysDefaultValueAttribute.cs
- SmiXetterAccessMap.cs
- CommonGetThemePartSize.cs
- WebPartConnectionsCancelVerb.cs
- Line.cs
- ClientRolePrincipal.cs
- ReferenceService.cs
- WebPartDisplayModeCollection.cs
- AssociationSet.cs
- PointAnimationClockResource.cs
- ExpandSegmentCollection.cs
- XmlReflectionImporter.cs
- ConnectionPoint.cs
- DependencyPropertyChangedEventArgs.cs
- QueryGeneratorBase.cs
- IgnoreFileBuildProvider.cs
- QueryReaderSettings.cs
- EditorZone.cs
- LocalizationParserHooks.cs
- WindowsTitleBar.cs
- PerformanceCountersElement.cs
- XmlQueryStaticData.cs
- NumberSubstitution.cs
- Header.cs
- TransformedBitmap.cs
- PrintingPermission.cs