Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Linq / Parallel / QueryOperators / Binary / GroupJoinQueryOperator.cs / 1305376 / GroupJoinQueryOperator.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // GroupJoinQueryOperator.cs // //[....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Threading; namespace System.Linq.Parallel { ////// A group join operator takes a left query tree and a right query tree, and then yields /// the matching elements between the two. This can be used for outer joins, i.e. those /// where an outer element has no matching inner elements -- the result is just an empty /// list. As with the join algorithm above, we currently use a hash join algorithm. /// ////// /// /// internal sealed class GroupJoinQueryOperator : BinaryQueryOperator { private readonly Func m_leftKeySelector; // The key selection routine for the outer (left) data source. private readonly Func m_rightKeySelector; // The key selection routine for the inner (right) data source. private readonly Func , TOutput> m_resultSelector; // The result selection routine. private readonly IEqualityComparer m_keyComparer; // An optional key comparison object. //---------------------------------------------------------------------------------------- // Constructs a new join operator. // internal GroupJoinQueryOperator(ParallelQuery left, ParallelQuery right, Func leftKeySelector, Func rightKeySelector, Func , TOutput> resultSelector, IEqualityComparer keyComparer) :base(left, right) { Contract.Assert(left != null && right != null, "child data sources cannot be null"); Contract.Assert(leftKeySelector != null, "left key selector must not be null"); Contract.Assert(rightKeySelector != null, "right key selector must not be null"); Contract.Assert(resultSelector != null, "need a result selector function"); m_leftKeySelector = leftKeySelector; m_rightKeySelector = rightKeySelector; m_resultSelector = resultSelector; m_keyComparer = keyComparer; m_outputOrdered = LeftChild.OutputOrdered; SetOrdinalIndex(OrdinalIndexState.Shuffled); } //--------------------------------------------------------------------------------------- // Just opens the current operator, including opening the child and wrapping it with // partitions as needed. // internal override QueryResults Open(QuerySettings settings, bool preferStriping) { QueryResults leftResults = LeftChild.Open(settings, false); QueryResults rightResults = RightChild.Open(settings, false); return new BinaryQueryOperatorResults(leftResults, rightResults, this, settings, false); } public override void WrapPartitionedStream ( PartitionedStream leftStream, PartitionedStream rightStream, IPartitionedStreamRecipient outputRecipient, bool preferStriping, QuerySettings settings) { Contract.Assert(rightStream.PartitionCount == leftStream.PartitionCount); int partitionCount = leftStream.PartitionCount; if (LeftChild.OutputOrdered) { WrapPartitionedStreamHelper ( ExchangeUtilities.HashRepartitionOrdered(leftStream, m_leftKeySelector, m_keyComparer, null, settings.CancellationState.MergedCancellationToken), rightStream, outputRecipient, partitionCount, settings.CancellationState.MergedCancellationToken); } else { WrapPartitionedStreamHelper ( ExchangeUtilities.HashRepartition(leftStream, m_leftKeySelector, m_keyComparer, null, settings.CancellationState.MergedCancellationToken), rightStream, outputRecipient, partitionCount, settings.CancellationState.MergedCancellationToken); } } //--------------------------------------------------------------------------------------- // This is a helper method. WrapPartitionedStream decides what type TLeftKey is going // to be, and then call this method with that key as a generic parameter. // private void WrapPartitionedStreamHelper ( PartitionedStream , TLeftKey> leftHashStream, PartitionedStream rightPartitionedStream, IPartitionedStreamRecipient outputRecipient, int partitionCount, CancellationToken cancellationToken) { PartitionedStream , int> rightHashStream = ExchangeUtilities.HashRepartition( rightPartitionedStream, m_rightKeySelector, m_keyComparer, null, cancellationToken); PartitionedStream outputStream = new PartitionedStream ( partitionCount, leftHashStream.KeyComparer, OrdinalIndexState); for (int i = 0; i < partitionCount; i++) { outputStream[i] = new HashJoinQueryOperatorEnumerator ( leftHashStream[i], rightHashStream[i], null, m_resultSelector, m_keyComparer, cancellationToken); } outputRecipient.Receive(outputStream); } //--------------------------------------------------------------------------------------- // Returns an enumerable that represents the query executing sequentially. // internal override IEnumerable AsSequentialQuery(CancellationToken token) { IEnumerable wrappedLeftChild = CancellableEnumerable.Wrap(LeftChild.AsSequentialQuery(token), token); IEnumerable wrappedRightChild = CancellableEnumerable.Wrap(RightChild.AsSequentialQuery(token), token); return wrappedLeftChild .GroupJoin( wrappedRightChild, m_leftKeySelector, m_rightKeySelector, m_resultSelector, m_keyComparer); } //---------------------------------------------------------------------------------------- // Whether this operator performs a premature merge. // internal override bool LimitsParallelism { get { return false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
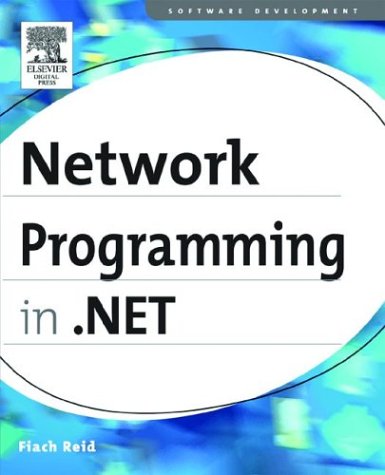
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SQLMoney.cs
- DeclaredTypeValidatorAttribute.cs
- EntityTypeEmitter.cs
- DisplayNameAttribute.cs
- QilExpression.cs
- Axis.cs
- DbReferenceCollection.cs
- SourceInterpreter.cs
- ExpressionValueEditor.cs
- XmlStringTable.cs
- BamlBinaryReader.cs
- ClassHandlersStore.cs
- SwitchAttribute.cs
- MsmqChannelFactoryBase.cs
- FixedTextContainer.cs
- ElementNotAvailableException.cs
- DefaultValueAttribute.cs
- ToolStripRenderEventArgs.cs
- VerificationAttribute.cs
- DLinqColumnProvider.cs
- EndpointDiscoveryMetadata.cs
- SqlCommand.cs
- StateBag.cs
- ReplacementText.cs
- MethodRental.cs
- SortableBindingList.cs
- HttpCacheVaryByContentEncodings.cs
- ListViewUpdatedEventArgs.cs
- Baml2006KnownTypes.cs
- NonVisualControlAttribute.cs
- TemplateKey.cs
- RuntimeHandles.cs
- SvcMapFileSerializer.cs
- GridViewPageEventArgs.cs
- SoundPlayerAction.cs
- ColorBlend.cs
- Animatable.cs
- XmlSchemaIdentityConstraint.cs
- IpcChannel.cs
- UpDownEvent.cs
- Fonts.cs
- EntityDataSourceDesigner.cs
- CodeSubDirectory.cs
- CompoundFileReference.cs
- RegexGroupCollection.cs
- Int32EqualityComparer.cs
- IdentifierElement.cs
- ExpressionBinding.cs
- HandledMouseEvent.cs
- DataGridViewRowHeightInfoPushedEventArgs.cs
- TextServicesHost.cs
- SiteMapNodeItemEventArgs.cs
- MemberPath.cs
- MatrixCamera.cs
- linebase.cs
- AudioSignalProblemOccurredEventArgs.cs
- PerformanceCounterLib.cs
- RequestResizeEvent.cs
- BamlLocalizationDictionary.cs
- WsatServiceAddress.cs
- HtmlInputPassword.cs
- autovalidator.cs
- UpDownBase.cs
- DashStyles.cs
- CompositeScriptReference.cs
- LinearGradientBrush.cs
- PrefixHandle.cs
- SizeKeyFrameCollection.cs
- FontFamilyIdentifier.cs
- RequestNavigateEventArgs.cs
- basecomparevalidator.cs
- ItemsPresenter.cs
- PlatformCulture.cs
- WebResponse.cs
- BorderSidesEditor.cs
- NativeMethods.cs
- XPathNode.cs
- AbandonedMutexException.cs
- MediaContext.cs
- OracleRowUpdatingEventArgs.cs
- CodeMethodReturnStatement.cs
- BooleanKeyFrameCollection.cs
- Process.cs
- InvalidAsynchronousStateException.cs
- CodeNamespaceCollection.cs
- WindowsFormsHelpers.cs
- ClientSponsor.cs
- XmlStreamNodeWriter.cs
- StreamWriter.cs
- _SslSessionsCache.cs
- SafeTimerHandle.cs
- AutomationPattern.cs
- RangeValuePattern.cs
- PropertyNames.cs
- OutputCacheSettingsSection.cs
- MetadataArtifactLoaderResource.cs
- ScriptBehaviorDescriptor.cs
- LinearQuaternionKeyFrame.cs
- EncoderExceptionFallback.cs
- ParentQuery.cs