Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / Base / Core / PropertyEditing / PropertyValueEditor.cs / 1305376 / PropertyValueEditor.cs
namespace System.Activities.Presentation.PropertyEditing { using System; using System.ComponentModel; using System.Globalization; using System.Text; using System.Windows; using System.Activities.Presentation.Internal.Properties; using System.Activities.Presentation; using System.Runtime; ////// Container for any and all inline editor logic for properties. This class can hold /// a single DataTemplates - one for Inline editor. /// public class PropertyValueEditor { private DataTemplate _inlineEditorTemplate; ////// Creates a PropertyValueEditor /// public PropertyValueEditor() { } ////// Creates a PropertyValueEditor /// /// The DataTemplate that is used for an inline editor. /// This DataTemplate has its DataContext set to a PropertyValue public PropertyValueEditor(DataTemplate inlineEditorTemplate) { _inlineEditorTemplate = inlineEditorTemplate; } ////// Gets or sets the InlineEditorTemplate -- the DataTemplate that is used for an inline editor. /// This DataTemplate has its DataContext set to a PropertyValue /// [Fx.Tag.KnownXamlExternalAttribute] public DataTemplate InlineEditorTemplate { get { return _inlineEditorTemplate; } set { _inlineEditorTemplate = value; } } internal virtual DataTemplate GetPropertyValueEditor(PropertyContainerEditMode mode) { return (mode == PropertyContainerEditMode.Inline) ? _inlineEditorTemplate : null; } ////// Utility method that creates a new EditorAttribute for the specified /// PropertyValueEditor /// /// PropertyValueEditor instance for which to create /// the new EditorAttribute ///New EditorAttribute for the specified PropertyValueEditor public static EditorAttribute CreateEditorAttribute(PropertyValueEditor editor) { if (editor == null) throw FxTrace.Exception.ArgumentNull("editor"); return CreateEditorAttribute(editor.GetType()); } ////// Utility method that creates a new EditorAttribute for the specified /// PropertyValueEditor type /// /// PropertyValueEditor type for which to create /// the new EditorAttribute ///New EditorAttribute for the specified PropertyValueEditor type public static EditorAttribute CreateEditorAttribute(Type propertyValueEditorType) { if (propertyValueEditorType == null) throw FxTrace.Exception.ArgumentNull("propertyValueEditorType"); if (!typeof(PropertyValueEditor).IsAssignableFrom(propertyValueEditorType)) throw FxTrace.Exception.AsError(new ArgumentException( string.Format( CultureInfo.CurrentCulture, Resources.Error_ArgIncorrectType, "propertyValueEditorType", typeof(PropertyValueEditor).Name))); return new EditorAttribute(propertyValueEditorType, typeof(PropertyValueEditor)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace System.Activities.Presentation.PropertyEditing { using System; using System.ComponentModel; using System.Globalization; using System.Text; using System.Windows; using System.Activities.Presentation.Internal.Properties; using System.Activities.Presentation; using System.Runtime; ////// Container for any and all inline editor logic for properties. This class can hold /// a single DataTemplates - one for Inline editor. /// public class PropertyValueEditor { private DataTemplate _inlineEditorTemplate; ////// Creates a PropertyValueEditor /// public PropertyValueEditor() { } ////// Creates a PropertyValueEditor /// /// The DataTemplate that is used for an inline editor. /// This DataTemplate has its DataContext set to a PropertyValue public PropertyValueEditor(DataTemplate inlineEditorTemplate) { _inlineEditorTemplate = inlineEditorTemplate; } ////// Gets or sets the InlineEditorTemplate -- the DataTemplate that is used for an inline editor. /// This DataTemplate has its DataContext set to a PropertyValue /// [Fx.Tag.KnownXamlExternalAttribute] public DataTemplate InlineEditorTemplate { get { return _inlineEditorTemplate; } set { _inlineEditorTemplate = value; } } internal virtual DataTemplate GetPropertyValueEditor(PropertyContainerEditMode mode) { return (mode == PropertyContainerEditMode.Inline) ? _inlineEditorTemplate : null; } ////// Utility method that creates a new EditorAttribute for the specified /// PropertyValueEditor /// /// PropertyValueEditor instance for which to create /// the new EditorAttribute ///New EditorAttribute for the specified PropertyValueEditor public static EditorAttribute CreateEditorAttribute(PropertyValueEditor editor) { if (editor == null) throw FxTrace.Exception.ArgumentNull("editor"); return CreateEditorAttribute(editor.GetType()); } ////// Utility method that creates a new EditorAttribute for the specified /// PropertyValueEditor type /// /// PropertyValueEditor type for which to create /// the new EditorAttribute ///New EditorAttribute for the specified PropertyValueEditor type public static EditorAttribute CreateEditorAttribute(Type propertyValueEditorType) { if (propertyValueEditorType == null) throw FxTrace.Exception.ArgumentNull("propertyValueEditorType"); if (!typeof(PropertyValueEditor).IsAssignableFrom(propertyValueEditorType)) throw FxTrace.Exception.AsError(new ArgumentException( string.Format( CultureInfo.CurrentCulture, Resources.Error_ArgIncorrectType, "propertyValueEditorType", typeof(PropertyValueEditor).Name))); return new EditorAttribute(propertyValueEditorType, typeof(PropertyValueEditor)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
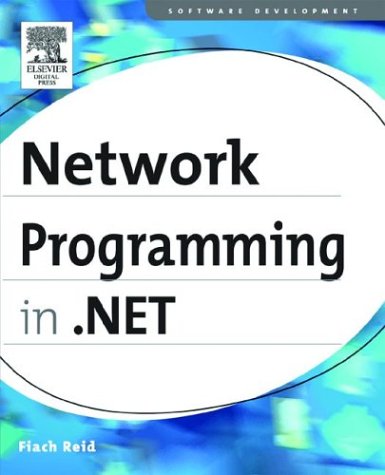
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SQLDateTimeStorage.cs
- TraceContextEventArgs.cs
- ProgressBar.cs
- recordstatescratchpad.cs
- AutoGeneratedFieldProperties.cs
- DefaultValueAttribute.cs
- UIElement3D.cs
- DataGridTableCollection.cs
- RenderDataDrawingContext.cs
- InvalidateEvent.cs
- ExtendedPropertiesHandler.cs
- BooleanFunctions.cs
- SQLMoneyStorage.cs
- ClaimSet.cs
- TableLayoutRowStyleCollection.cs
- DataSourceProvider.cs
- CodeEventReferenceExpression.cs
- OdbcDataReader.cs
- SessionState.cs
- BitmapEncoder.cs
- formatstringdialog.cs
- DecimalKeyFrameCollection.cs
- Descriptor.cs
- Propagator.JoinPropagator.JoinPredicateVisitor.cs
- TimeSpanSecondsOrInfiniteConverter.cs
- Point3DKeyFrameCollection.cs
- KeyboardDevice.cs
- WebPartAddingEventArgs.cs
- MarkerProperties.cs
- SmtpReplyReader.cs
- TransactionManager.cs
- VisualStateChangedEventArgs.cs
- WaitHandleCannotBeOpenedException.cs
- BamlWriter.cs
- NullableConverter.cs
- XmlHierarchicalDataSourceView.cs
- SingleQueryOperator.cs
- WebConfigurationHostFileChange.cs
- SparseMemoryStream.cs
- DtdParser.cs
- RegisteredHiddenField.cs
- CaseStatement.cs
- HttpApplicationStateWrapper.cs
- DataKey.cs
- AlignmentXValidation.cs
- ScrollData.cs
- AccessDataSourceView.cs
- SystemTcpStatistics.cs
- AuthorizationSection.cs
- BindStream.cs
- WmfPlaceableFileHeader.cs
- AutoResizedEvent.cs
- ControlParameter.cs
- CompiledRegexRunner.cs
- ColorConverter.cs
- XmlDataLoader.cs
- InstanceCreationEditor.cs
- ExtentCqlBlock.cs
- ProxyHelper.cs
- IItemProperties.cs
- CharAnimationUsingKeyFrames.cs
- CodeConditionStatement.cs
- DrawingCollection.cs
- ColumnHeaderConverter.cs
- BasicViewGenerator.cs
- SR.Designer.cs
- EdmType.cs
- XD.cs
- ShaderRenderModeValidation.cs
- XmlTextAttribute.cs
- ProcessHostMapPath.cs
- CodeVariableDeclarationStatement.cs
- ImageListUtils.cs
- NetNamedPipeSecurity.cs
- CapacityStreamGeometryContext.cs
- MinMaxParagraphWidth.cs
- SupportingTokenAuthenticatorSpecification.cs
- UriGenerator.cs
- DBConcurrencyException.cs
- ReservationCollection.cs
- DiagnosticTrace.cs
- StructuralCache.cs
- HttpRequestMessageProperty.cs
- BridgeDataReader.cs
- TempEnvironment.cs
- SchemaObjectWriter.cs
- TextSelectionHelper.cs
- NativeMethods.cs
- TreeNodeCollectionEditor.cs
- VisualTreeHelper.cs
- FunctionImportElement.cs
- XmlSchemaSequence.cs
- StrongName.cs
- WebPartTransformerAttribute.cs
- FieldDescriptor.cs
- PropertyMapper.cs
- MarkupProperty.cs
- CompilerWrapper.cs
- RadioButtonBaseAdapter.cs
- OpenTypeMethods.cs