Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / xsp / System / Web / IdleTimeoutMonitor.cs / 1 / IdleTimeoutMonitor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Request timeout manager -- implements the request timeout mechanism */ namespace System.Web { using System.Threading; using System.Collections; using System.Web.Hosting; using System.Web.Util; internal class IdleTimeoutMonitor { private TimeSpan _idleTimeout; // the timeout value private DateTime _lastEvent; // idle since this time private Timer _timer; private readonly TimeSpan _timerPeriod = new TimeSpan(0, 0, 30); // 30 secs internal IdleTimeoutMonitor(TimeSpan timeout) { _idleTimeout = timeout; _timer = new Timer(new TimerCallback(this.TimerCompletionCallback), null, _timerPeriod, _timerPeriod); _lastEvent = DateTime.UtcNow; } internal void Stop() { // stop the timer if (_timer != null) { lock (this) { if (_timer != null) { ((IDisposable)_timer).Dispose(); _timer = null; } } } } internal DateTime LastEvent { // thread-safe property get { DateTime t; lock (this) { t = _lastEvent; } return t; } set { lock (this) { _lastEvent = value; } } } private void TimerCompletionCallback(Object state) { // user idle timer to trim the free list of app instanced HttpApplicationFactory.TrimApplicationInstances(); // no idle timeout if (_idleTimeout == TimeSpan.MaxValue) return; // don't do idle timeout if already shutting down if (HostingEnvironment.ShutdownInitiated) return; // check if there are active requests if (HostingEnvironment.BusyCount != 0) return; // check if enough time passed if (DateTime.UtcNow <= LastEvent.Add(_idleTimeout)) return; // check if debugger is attached if (System.Diagnostics.Debugger.IsAttached) return; // shutdown HttpRuntime.SetShutdownReason(ApplicationShutdownReason.IdleTimeout, SR.GetString(SR.Hosting_Env_IdleTimeout)); HostingEnvironment.InitiateShutdown(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Request timeout manager -- implements the request timeout mechanism */ namespace System.Web { using System.Threading; using System.Collections; using System.Web.Hosting; using System.Web.Util; internal class IdleTimeoutMonitor { private TimeSpan _idleTimeout; // the timeout value private DateTime _lastEvent; // idle since this time private Timer _timer; private readonly TimeSpan _timerPeriod = new TimeSpan(0, 0, 30); // 30 secs internal IdleTimeoutMonitor(TimeSpan timeout) { _idleTimeout = timeout; _timer = new Timer(new TimerCallback(this.TimerCompletionCallback), null, _timerPeriod, _timerPeriod); _lastEvent = DateTime.UtcNow; } internal void Stop() { // stop the timer if (_timer != null) { lock (this) { if (_timer != null) { ((IDisposable)_timer).Dispose(); _timer = null; } } } } internal DateTime LastEvent { // thread-safe property get { DateTime t; lock (this) { t = _lastEvent; } return t; } set { lock (this) { _lastEvent = value; } } } private void TimerCompletionCallback(Object state) { // user idle timer to trim the free list of app instanced HttpApplicationFactory.TrimApplicationInstances(); // no idle timeout if (_idleTimeout == TimeSpan.MaxValue) return; // don't do idle timeout if already shutting down if (HostingEnvironment.ShutdownInitiated) return; // check if there are active requests if (HostingEnvironment.BusyCount != 0) return; // check if enough time passed if (DateTime.UtcNow <= LastEvent.Add(_idleTimeout)) return; // check if debugger is attached if (System.Diagnostics.Debugger.IsAttached) return; // shutdown HttpRuntime.SetShutdownReason(ApplicationShutdownReason.IdleTimeout, SR.GetString(SR.Hosting_Env_IdleTimeout)); HostingEnvironment.InitiateShutdown(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
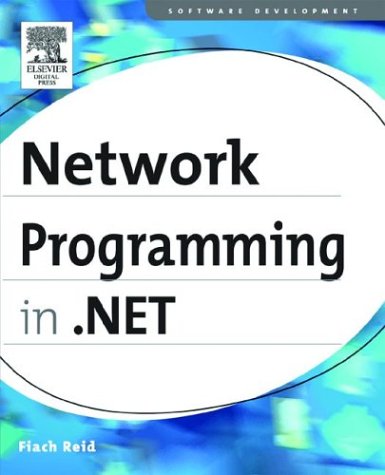
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProgressChangedEventArgs.cs
- BinaryMessageEncoder.cs
- SchemaCollectionCompiler.cs
- CustomSignedXml.cs
- Int32KeyFrameCollection.cs
- SearchForVirtualItemEventArgs.cs
- MenuItemAutomationPeer.cs
- EntityConnectionStringBuilder.cs
- Pair.cs
- MethodSignatureGenerator.cs
- ListViewAutomationPeer.cs
- CryptoConfig.cs
- SendActivityEventArgs.cs
- Control.cs
- IncrementalCompileAnalyzer.cs
- DiscoveryClientProtocol.cs
- ValidationHelper.cs
- Dictionary.cs
- ClientApiGenerator.cs
- BinHexEncoder.cs
- SettingsAttributeDictionary.cs
- RestHandlerFactory.cs
- FileDataSourceCache.cs
- XPathDocumentNavigator.cs
- CellParaClient.cs
- VideoDrawing.cs
- MarkupCompilePass1.cs
- TextParagraphView.cs
- AssemblyInfo.cs
- SystemPens.cs
- DataGridViewColumnCollectionDialog.cs
- ButtonAutomationPeer.cs
- CryptoStream.cs
- EntityDataSourceView.cs
- ToolStripRenderEventArgs.cs
- ReferencedAssembly.cs
- CodeMemberMethod.cs
- HttpResponseHeader.cs
- SqlPersonalizationProvider.cs
- TreeNodeBinding.cs
- ExpressionDumper.cs
- ToolStripProgressBar.cs
- MembershipValidatePasswordEventArgs.cs
- WebColorConverter.cs
- XmlAggregates.cs
- SiteMapDataSource.cs
- NavigationEventArgs.cs
- Bitmap.cs
- WebMessageFormatHelper.cs
- ResourceAssociationTypeEnd.cs
- NameValueSectionHandler.cs
- OperationCanceledException.cs
- TextRangeAdaptor.cs
- ProfessionalColorTable.cs
- SqlConnectionStringBuilder.cs
- HttpContextServiceHost.cs
- TextTreeInsertUndoUnit.cs
- DataGridViewTextBoxColumn.cs
- XmlLoader.cs
- WebEventTraceProvider.cs
- SchemaNames.cs
- SystemInfo.cs
- PropertyGridView.cs
- NotifyInputEventArgs.cs
- Wildcard.cs
- HttpStreams.cs
- ListViewEditEventArgs.cs
- InternalsVisibleToAttribute.cs
- XsltInput.cs
- TransactionBehavior.cs
- WizardDesigner.cs
- ViewValidator.cs
- BufferCache.cs
- BindingValueChangedEventArgs.cs
- DataViewManager.cs
- UserPreferenceChangedEventArgs.cs
- PeerDefaultCustomResolverClient.cs
- DataListItem.cs
- UDPClient.cs
- HandlerElementCollection.cs
- HtmlProps.cs
- AspNetHostingPermission.cs
- AnnotationAdorner.cs
- ProcessHostMapPath.cs
- XmlDataSource.cs
- WSSecureConversation.cs
- ConsumerConnectionPoint.cs
- HebrewNumber.cs
- EventLogPermission.cs
- FindCriteria11.cs
- ScrollEvent.cs
- DataObjectAttribute.cs
- Int32KeyFrameCollection.cs
- ElementProxy.cs
- IApplicationTrustManager.cs
- ImpersonateTokenRef.cs
- BitmapEffectDrawing.cs
- ipaddressinformationcollection.cs
- ProgressBar.cs
- AbstractSvcMapFileLoader.cs