Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / StreamGeometryContext.cs / 1 / StreamGeometryContext.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This class is used by the StreamGeometry class to generate an inlined, // flattened geometry stream. // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.Collections; using System.Collections.Generic; using System.Security; using System.Security.Permissions; #if !PBTCOMPILER using System.Runtime.InteropServices; using System.Windows.Threading; using System.Windows; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Effects; using System.Windows.Media.Imaging; using System.Windows.Media.Media3D; using System.Diagnostics; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using MS.Internal.PresentationCore; namespace System.Windows.Media #elif PBTCOMPILER using MS.Internal.Markup; namespace MS.Internal.Markup #endif { ////// StreamGeometryContext /// #if ! PBTCOMPILER public abstract class StreamGeometryContext : DispatcherObject, IDisposable #else internal abstract class StreamGeometryContext : IDisposable #endif { #region Constructors ////// This constructor exists to prevent external derivation /// internal StreamGeometryContext() { } #endregion Constructors #region IDisposable void IDisposable.Dispose() { #if ! PBTCOMPILER VerifyAccess(); #endif DisposeCore(); } #endregion IDisposable #region Public Methods ////// Closes the StreamContext and flushes the content. /// Afterwards the StreamContext can not be used anymore. /// This call does not require all Push calls to have been Popped. /// ////// This call is illegal if this object has already been closed or disposed. /// public virtual void Close() { DisposeCore(); } ////// BeginFigure - Start a new figure. /// public abstract void BeginFigure(Point startPoint, bool isFilled, bool isClosed); ////// LineTo - append a LineTo to the current figure. /// public abstract void LineTo(Point point, bool isStroked, bool isSmoothJoin); ////// QuadraticBezierTo - append a QuadraticBezierTo to the current figure. /// public abstract void QuadraticBezierTo(Point point1, Point point2, bool isStroked, bool isSmoothJoin); ////// BezierTo - apply a BezierTo to the current figure. /// public abstract void BezierTo(Point point1, Point point2, Point point3, bool isStroked, bool isSmoothJoin); ////// PolyLineTo - append a PolyLineTo to the current figure. /// public abstract void PolyLineTo(IListpoints, bool isStroked, bool isSmoothJoin); /// /// PolyQuadraticBezierTo - append a PolyQuadraticBezierTo to the current figure. /// public abstract void PolyQuadraticBezierTo(IListpoints, bool isStroked, bool isSmoothJoin); /// /// PolyBezierTo - append a PolyBezierTo to the current figure. /// public abstract void PolyBezierTo(IListpoints, bool isStroked, bool isSmoothJoin); /// /// ArcTo - append an ArcTo to the current figure. /// // Special case this one. Bringing in sweep direction requires code-gen changes. // #if !PBTCOMPILER public abstract void ArcTo(Point point, Size size, double rotationAngle, bool isLargeArc, SweepDirection sweepDirection, bool isStroked, bool isSmoothJoin); #else public abstract void ArcTo(Point point, Size size, double rotationAngle, bool isLargeArc, bool sweepDirection, bool isStroked, bool isSmoothJoin); #endif #endregion Public Methods ////// This is the same as the Close call: /// Closes the Context and flushes the content. /// Afterwards the Context can not be used anymore. /// This call does not require all Push calls to have been Popped. /// ////// This call is illegal if this object has already been closed or disposed. /// internal virtual void DisposeCore() {} ////// SetClosedState - Sets the current closed state of the figure. /// internal abstract void SetClosedState(bool closed); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This class is used by the StreamGeometry class to generate an inlined, // flattened geometry stream. // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.Collections; using System.Collections.Generic; using System.Security; using System.Security.Permissions; #if !PBTCOMPILER using System.Runtime.InteropServices; using System.Windows.Threading; using System.Windows; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Effects; using System.Windows.Media.Imaging; using System.Windows.Media.Media3D; using System.Diagnostics; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using MS.Internal.PresentationCore; namespace System.Windows.Media #elif PBTCOMPILER using MS.Internal.Markup; namespace MS.Internal.Markup #endif { ////// StreamGeometryContext /// #if ! PBTCOMPILER public abstract class StreamGeometryContext : DispatcherObject, IDisposable #else internal abstract class StreamGeometryContext : IDisposable #endif { #region Constructors ////// This constructor exists to prevent external derivation /// internal StreamGeometryContext() { } #endregion Constructors #region IDisposable void IDisposable.Dispose() { #if ! PBTCOMPILER VerifyAccess(); #endif DisposeCore(); } #endregion IDisposable #region Public Methods ////// Closes the StreamContext and flushes the content. /// Afterwards the StreamContext can not be used anymore. /// This call does not require all Push calls to have been Popped. /// ////// This call is illegal if this object has already been closed or disposed. /// public virtual void Close() { DisposeCore(); } ////// BeginFigure - Start a new figure. /// public abstract void BeginFigure(Point startPoint, bool isFilled, bool isClosed); ////// LineTo - append a LineTo to the current figure. /// public abstract void LineTo(Point point, bool isStroked, bool isSmoothJoin); ////// QuadraticBezierTo - append a QuadraticBezierTo to the current figure. /// public abstract void QuadraticBezierTo(Point point1, Point point2, bool isStroked, bool isSmoothJoin); ////// BezierTo - apply a BezierTo to the current figure. /// public abstract void BezierTo(Point point1, Point point2, Point point3, bool isStroked, bool isSmoothJoin); ////// PolyLineTo - append a PolyLineTo to the current figure. /// public abstract void PolyLineTo(IListpoints, bool isStroked, bool isSmoothJoin); /// /// PolyQuadraticBezierTo - append a PolyQuadraticBezierTo to the current figure. /// public abstract void PolyQuadraticBezierTo(IListpoints, bool isStroked, bool isSmoothJoin); /// /// PolyBezierTo - append a PolyBezierTo to the current figure. /// public abstract void PolyBezierTo(IListpoints, bool isStroked, bool isSmoothJoin); /// /// ArcTo - append an ArcTo to the current figure. /// // Special case this one. Bringing in sweep direction requires code-gen changes. // #if !PBTCOMPILER public abstract void ArcTo(Point point, Size size, double rotationAngle, bool isLargeArc, SweepDirection sweepDirection, bool isStroked, bool isSmoothJoin); #else public abstract void ArcTo(Point point, Size size, double rotationAngle, bool isLargeArc, bool sweepDirection, bool isStroked, bool isSmoothJoin); #endif #endregion Public Methods ////// This is the same as the Close call: /// Closes the Context and flushes the content. /// Afterwards the Context can not be used anymore. /// This call does not require all Push calls to have been Popped. /// ////// This call is illegal if this object has already been closed or disposed. /// internal virtual void DisposeCore() {} ////// SetClosedState - Sets the current closed state of the figure. /// internal abstract void SetClosedState(bool closed); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
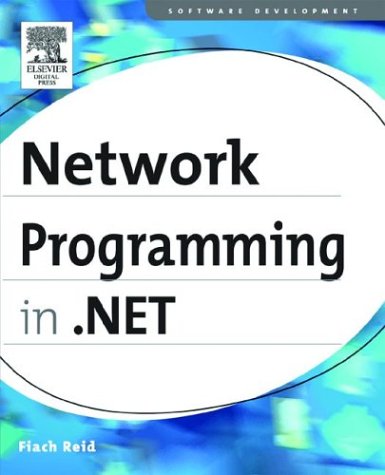
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DesignerRegionCollection.cs
- SQLBinary.cs
- SerializationHelper.cs
- HttpWriter.cs
- PageContentCollection.cs
- FixedTextPointer.cs
- TextParagraphProperties.cs
- Privilege.cs
- PerformanceCounterPermissionEntry.cs
- InternalsVisibleToAttribute.cs
- TextPenaltyModule.cs
- ToolboxItemImageConverter.cs
- Image.cs
- TextPatternIdentifiers.cs
- Number.cs
- HGlobalSafeHandle.cs
- DataExpression.cs
- RemoveStoryboard.cs
- LineUtil.cs
- CfgParser.cs
- HtmlButton.cs
- ReaderOutput.cs
- MessagePartSpecification.cs
- ProxyElement.cs
- ValueConversionAttribute.cs
- MemberAccessException.cs
- ToolStripRenderer.cs
- SchemaSetCompiler.cs
- BitmapImage.cs
- DurationConverter.cs
- LinqDataSourceStatusEventArgs.cs
- Blend.cs
- CalendarModeChangedEventArgs.cs
- Panel.cs
- ConnectionConsumerAttribute.cs
- UnsafeNativeMethods.cs
- EndPoint.cs
- SqlConnectionString.cs
- ReachDocumentPageSerializerAsync.cs
- MouseActionValueSerializer.cs
- AttachedAnnotationChangedEventArgs.cs
- MessageSecurityProtocolFactory.cs
- COM2PropertyDescriptor.cs
- TextTreeFixupNode.cs
- ConnectionOrientedTransportBindingElement.cs
- XmlILTrace.cs
- ValidatorCompatibilityHelper.cs
- CallContext.cs
- XPathArrayIterator.cs
- SeekStoryboard.cs
- ListViewContainer.cs
- DoubleUtil.cs
- RecognizedPhrase.cs
- CurrentChangedEventManager.cs
- Baml2006ReaderContext.cs
- TrackBar.cs
- RegistrySecurity.cs
- ListItemViewControl.cs
- List.cs
- httpapplicationstate.cs
- DiagnosticsConfiguration.cs
- EntityDataSourceMemberPath.cs
- UrlPath.cs
- BackgroundWorker.cs
- ContractComponent.cs
- InputScopeNameConverter.cs
- CollectionViewGroupRoot.cs
- FileUtil.cs
- Enum.cs
- OpacityConverter.cs
- StructuralType.cs
- SID.cs
- CheckBox.cs
- OneOfConst.cs
- PropertyValueUIItem.cs
- _NegoStream.cs
- SqlDeflator.cs
- JsonDeserializer.cs
- DesigntimeLicenseContextSerializer.cs
- oledbconnectionstring.cs
- TdsParserStateObject.cs
- CultureSpecificCharacterBufferRange.cs
- TreeNodeBinding.cs
- RtfNavigator.cs
- JoinElimination.cs
- CodeLinePragma.cs
- PrintEvent.cs
- MsmqIntegrationSecurityMode.cs
- ValueTable.cs
- Color.cs
- TextElementEnumerator.cs
- ScriptingSectionGroup.cs
- InterleavedZipPartStream.cs
- SqlSelectStatement.cs
- Type.cs
- SkipStoryboardToFill.cs
- SafeRegistryHandle.cs
- BitmapScalingModeValidation.cs
- SoapSchemaExporter.cs
- ISFClipboardData.cs