Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / xsp / System / Web / Extensions / ui / UpdateProgress.cs / 2 / UpdateProgress.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics.CodeAnalysis; using System.Drawing; using System.Globalization; using System.Security.Permissions; using System.Text; using System.Web; using System.Web.UI; using System.Web.Script; using System.Web.Resources; using System.Web.Util; [ AspNetHostingPermission(SecurityAction.LinkDemand, Level = AspNetHostingPermissionLevel.Minimal), AspNetHostingPermission(SecurityAction.InheritanceDemand, Level = AspNetHostingPermissionLevel.Minimal), DefaultProperty("AssociatedUpdatePanelID"), Designer("System.Web.UI.Design.UpdateProgressDesigner, " + AssemblyRef.SystemWebExtensionsDesign), ParseChildren(true), PersistChildren(false), ToolboxBitmap(typeof(EmbeddedResourceFinder), "System.Web.Resources.UpdateProgress.bmp"), ToolboxItemFilterAttribute(AssemblyRef.SystemWebExtensions, ToolboxItemFilterType.Require), ] public class UpdateProgress : Control, IScriptControl { private ITemplate _progressTemplate; private Control _progressTemplateContainer; private int _displayAfter = 500; private bool _dynamicLayout = true; private string _associatedUpdatePanelID; [ Category("Behavior"), DefaultValue(""), IDReferenceProperty(typeof(UpdatePanel)), ResourceDescription("UpdateProgress_AssociatedUpdatePanelID"), SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "ID"), TypeConverter("System.Web.UI.Design.UpdateProgressAssociatedUpdatePanelIDConverter") ] public string AssociatedUpdatePanelID { get { if (_associatedUpdatePanelID == null) { return String.Empty; } return _associatedUpdatePanelID; } set { _associatedUpdatePanelID = value; } } public override ControlCollection Controls { get { EnsureChildControls(); return base.Controls; } } [ DefaultValue(500), ResourceDescription("UpdateProgress_DisplayAfter"), Category("Behavior") ] public int DisplayAfter { get { return _displayAfter; } set { if (value < 0) { throw new ArgumentOutOfRangeException(AtlasWeb.UpdateProgress_DisplayAfterInvalid); } _displayAfter = value; } } [ Browsable(false), PersistenceMode(PersistenceMode.InnerProperty), ResourceDescription("UpdateProgress_ProgressTemplate"), ] public ITemplate ProgressTemplate { get { return _progressTemplate; } set { _progressTemplate = value; } } [ DefaultValue(true), ResourceDescription("UpdateProgress_DynamicLayout"), Category("Behavior") ] public bool DynamicLayout { get { return _dynamicLayout; } set { _dynamicLayout = value; } } private ScriptManager ScriptManager { get { ScriptManager scriptManager = ScriptManager.GetCurrent(Page); if (scriptManager == null) { throw new InvalidOperationException(String.Format(CultureInfo.InvariantCulture, AtlasWeb.Common_ScriptManagerRequired, ID)); } return scriptManager; } } protected internal override void CreateChildControls() { // Set up the progress template if (!DesignMode && _progressTemplate == null) { throw new InvalidOperationException(String.Format(CultureInfo.InvariantCulture, AtlasWeb.UpdateProgress_TemplateRequired, ID)); } else if (_progressTemplate != null) { _progressTemplateContainer = new Control(); _progressTemplate.InstantiateIn(_progressTemplateContainer); Controls.Add(_progressTemplateContainer); } } public override void DataBind() { EnsureChildControls(); base.DataBind(); } [SuppressMessage("Microsoft.Security", "CA2109:ReviewVisibleEventHandlers")] protected internal override void OnPreRender(EventArgs e) { base.OnPreRender(e); ScriptManager.RegisterScriptControl(this); } protected internal override void Render(HtmlTextWriter writer) { EnsureChildControls(); writer.AddAttribute(HtmlTextWriterAttribute.Id, ClientID); if (_dynamicLayout) { writer.AddStyleAttribute(HtmlTextWriterStyle.Display, "none"); } else { writer.AddStyleAttribute(HtmlTextWriterStyle.Visibility, "hidden"); writer.AddStyleAttribute(HtmlTextWriterStyle.Display, "block"); } writer.RenderBeginTag(HtmlTextWriterTag.Div); base.Render(writer); writer.RenderEndTag(); // div if (!DesignMode) { ScriptManager.RegisterScriptDescriptors(this); } } [SuppressMessage("Microsoft.Design", "CA1024:UsePropertiesWhereAppropriate", Justification = "Matches IScriptControl interface.")] protected virtual IEnumerableGetScriptReferences() { yield break; } [SuppressMessage("Microsoft.Design", "CA1024:UsePropertiesWhereAppropriate", Justification = "Matches IScriptControl interface.")] protected virtual IEnumerable GetScriptDescriptors() { // Don't render any scripts when partial rendering is not enabled if (Page != null && ScriptManager.SupportsPartialRendering && Visible) { ScriptControlDescriptor desc = new ScriptControlDescriptor("Sys.UI._UpdateProgress", ClientID); string updatePanelClientID = null; if (!String.IsNullOrEmpty(AssociatedUpdatePanelID)) { // Try both the NamingContainer and the Page UpdatePanel c = ControlUtil.FindTargetControl(AssociatedUpdatePanelID, this, true) as UpdatePanel; if (c != null) updatePanelClientID = c.ClientID; else { throw new InvalidOperationException(String.Format(CultureInfo.InvariantCulture, AtlasWeb.UpdateProgress_NoUpdatePanel, AssociatedUpdatePanelID)); } } desc.AddProperty("associatedUpdatePanelId", updatePanelClientID); desc.AddProperty("dynamicLayout", DynamicLayout); desc.AddProperty("displayAfter", DisplayAfter); yield return desc; } yield break; } #region IScriptControl Members IEnumerable IScriptControl.GetScriptReferences() { return GetScriptReferences(); } IEnumerable IScriptControl.GetScriptDescriptors() { return GetScriptDescriptors(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics.CodeAnalysis; using System.Drawing; using System.Globalization; using System.Security.Permissions; using System.Text; using System.Web; using System.Web.UI; using System.Web.Script; using System.Web.Resources; using System.Web.Util; [ AspNetHostingPermission(SecurityAction.LinkDemand, Level = AspNetHostingPermissionLevel.Minimal), AspNetHostingPermission(SecurityAction.InheritanceDemand, Level = AspNetHostingPermissionLevel.Minimal), DefaultProperty("AssociatedUpdatePanelID"), Designer("System.Web.UI.Design.UpdateProgressDesigner, " + AssemblyRef.SystemWebExtensionsDesign), ParseChildren(true), PersistChildren(false), ToolboxBitmap(typeof(EmbeddedResourceFinder), "System.Web.Resources.UpdateProgress.bmp"), ToolboxItemFilterAttribute(AssemblyRef.SystemWebExtensions, ToolboxItemFilterType.Require), ] public class UpdateProgress : Control, IScriptControl { private ITemplate _progressTemplate; private Control _progressTemplateContainer; private int _displayAfter = 500; private bool _dynamicLayout = true; private string _associatedUpdatePanelID; [ Category("Behavior"), DefaultValue(""), IDReferenceProperty(typeof(UpdatePanel)), ResourceDescription("UpdateProgress_AssociatedUpdatePanelID"), SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "ID"), TypeConverter("System.Web.UI.Design.UpdateProgressAssociatedUpdatePanelIDConverter") ] public string AssociatedUpdatePanelID { get { if (_associatedUpdatePanelID == null) { return String.Empty; } return _associatedUpdatePanelID; } set { _associatedUpdatePanelID = value; } } public override ControlCollection Controls { get { EnsureChildControls(); return base.Controls; } } [ DefaultValue(500), ResourceDescription("UpdateProgress_DisplayAfter"), Category("Behavior") ] public int DisplayAfter { get { return _displayAfter; } set { if (value < 0) { throw new ArgumentOutOfRangeException(AtlasWeb.UpdateProgress_DisplayAfterInvalid); } _displayAfter = value; } } [ Browsable(false), PersistenceMode(PersistenceMode.InnerProperty), ResourceDescription("UpdateProgress_ProgressTemplate"), ] public ITemplate ProgressTemplate { get { return _progressTemplate; } set { _progressTemplate = value; } } [ DefaultValue(true), ResourceDescription("UpdateProgress_DynamicLayout"), Category("Behavior") ] public bool DynamicLayout { get { return _dynamicLayout; } set { _dynamicLayout = value; } } private ScriptManager ScriptManager { get { ScriptManager scriptManager = ScriptManager.GetCurrent(Page); if (scriptManager == null) { throw new InvalidOperationException(String.Format(CultureInfo.InvariantCulture, AtlasWeb.Common_ScriptManagerRequired, ID)); } return scriptManager; } } protected internal override void CreateChildControls() { // Set up the progress template if (!DesignMode && _progressTemplate == null) { throw new InvalidOperationException(String.Format(CultureInfo.InvariantCulture, AtlasWeb.UpdateProgress_TemplateRequired, ID)); } else if (_progressTemplate != null) { _progressTemplateContainer = new Control(); _progressTemplate.InstantiateIn(_progressTemplateContainer); Controls.Add(_progressTemplateContainer); } } public override void DataBind() { EnsureChildControls(); base.DataBind(); } [SuppressMessage("Microsoft.Security", "CA2109:ReviewVisibleEventHandlers")] protected internal override void OnPreRender(EventArgs e) { base.OnPreRender(e); ScriptManager.RegisterScriptControl(this); } protected internal override void Render(HtmlTextWriter writer) { EnsureChildControls(); writer.AddAttribute(HtmlTextWriterAttribute.Id, ClientID); if (_dynamicLayout) { writer.AddStyleAttribute(HtmlTextWriterStyle.Display, "none"); } else { writer.AddStyleAttribute(HtmlTextWriterStyle.Visibility, "hidden"); writer.AddStyleAttribute(HtmlTextWriterStyle.Display, "block"); } writer.RenderBeginTag(HtmlTextWriterTag.Div); base.Render(writer); writer.RenderEndTag(); // div if (!DesignMode) { ScriptManager.RegisterScriptDescriptors(this); } } [SuppressMessage("Microsoft.Design", "CA1024:UsePropertiesWhereAppropriate", Justification = "Matches IScriptControl interface.")] protected virtual IEnumerableGetScriptReferences() { yield break; } [SuppressMessage("Microsoft.Design", "CA1024:UsePropertiesWhereAppropriate", Justification = "Matches IScriptControl interface.")] protected virtual IEnumerable GetScriptDescriptors() { // Don't render any scripts when partial rendering is not enabled if (Page != null && ScriptManager.SupportsPartialRendering && Visible) { ScriptControlDescriptor desc = new ScriptControlDescriptor("Sys.UI._UpdateProgress", ClientID); string updatePanelClientID = null; if (!String.IsNullOrEmpty(AssociatedUpdatePanelID)) { // Try both the NamingContainer and the Page UpdatePanel c = ControlUtil.FindTargetControl(AssociatedUpdatePanelID, this, true) as UpdatePanel; if (c != null) updatePanelClientID = c.ClientID; else { throw new InvalidOperationException(String.Format(CultureInfo.InvariantCulture, AtlasWeb.UpdateProgress_NoUpdatePanel, AssociatedUpdatePanelID)); } } desc.AddProperty("associatedUpdatePanelId", updatePanelClientID); desc.AddProperty("dynamicLayout", DynamicLayout); desc.AddProperty("displayAfter", DisplayAfter); yield return desc; } yield break; } #region IScriptControl Members IEnumerable IScriptControl.GetScriptReferences() { return GetScriptReferences(); } IEnumerable IScriptControl.GetScriptDescriptors() { return GetScriptDescriptors(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
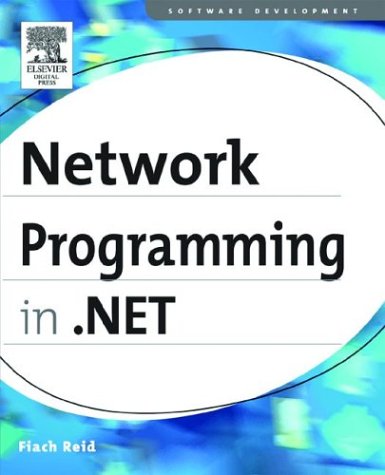
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BuildProvider.cs
- DataGridLengthConverter.cs
- OleDragDropHandler.cs
- Highlights.cs
- ResourceKey.cs
- HighlightVisual.cs
- SecureUICommand.cs
- ObjectListCommandEventArgs.cs
- VirtualizingStackPanel.cs
- DataBoundControl.cs
- Win32SafeHandles.cs
- TrustLevel.cs
- ClientOptions.cs
- XmlIncludeAttribute.cs
- WizardPanelChangingEventArgs.cs
- DecoratedNameAttribute.cs
- Tokenizer.cs
- ScriptingSectionGroup.cs
- FillErrorEventArgs.cs
- ExpressionVisitorHelpers.cs
- EdmPropertyAttribute.cs
- DupHandleConnectionReader.cs
- CacheAxisQuery.cs
- Selection.cs
- FontDriver.cs
- PolicyReader.cs
- DirectoryInfo.cs
- SafeNativeMethods.cs
- ConfigurationSectionHelper.cs
- ScrollableControl.cs
- ChannelPoolSettings.cs
- EntityClientCacheEntry.cs
- Baml2006ReaderSettings.cs
- StackOverflowException.cs
- GacUtil.cs
- QueryExpr.cs
- HttpCapabilitiesBase.cs
- _ListenerResponseStream.cs
- Debugger.cs
- XmlWriter.cs
- EventHandlersStore.cs
- DataSvcMapFile.cs
- unsafenativemethodsother.cs
- GeneralTransform3D.cs
- EntitySqlQueryState.cs
- WindowsListViewGroup.cs
- Line.cs
- XmlProcessingInstruction.cs
- FileCodeGroup.cs
- CommandEventArgs.cs
- Base64Encoder.cs
- CodeFieldReferenceExpression.cs
- RecordsAffectedEventArgs.cs
- ValidationError.cs
- AssemblyBuilderData.cs
- _IPv4Address.cs
- GridViewRow.cs
- CharConverter.cs
- WebPartCatalogAddVerb.cs
- BevelBitmapEffect.cs
- EmptyImpersonationContext.cs
- SortExpressionBuilder.cs
- RepeaterItemEventArgs.cs
- URLString.cs
- QilList.cs
- XhtmlBasicListAdapter.cs
- FixedPageAutomationPeer.cs
- DrawListViewItemEventArgs.cs
- RepeaterItemEventArgs.cs
- DataControlImageButton.cs
- ExpressionVisitor.cs
- Exceptions.cs
- xml.cs
- MobileTextWriter.cs
- InfoCardSymmetricAlgorithm.cs
- DataRelation.cs
- TableProviderWrapper.cs
- UnorderedHashRepartitionStream.cs
- MaskedTextProvider.cs
- InstanceData.cs
- ShaperBuffers.cs
- SiteMapDataSource.cs
- ApplicationFileParser.cs
- DataStreams.cs
- ListParaClient.cs
- XPathDocumentNavigator.cs
- XmlSchemaAll.cs
- SectionInput.cs
- InfoCardMasterKey.cs
- BStrWrapper.cs
- ObjectViewEntityCollectionData.cs
- Camera.cs
- WebPartManagerInternals.cs
- HwndMouseInputProvider.cs
- MatrixConverter.cs
- OperationAbortedException.cs
- messageonlyhwndwrapper.cs
- AdapterDictionary.cs
- sapiproxy.cs
- SamlAuthenticationStatement.cs